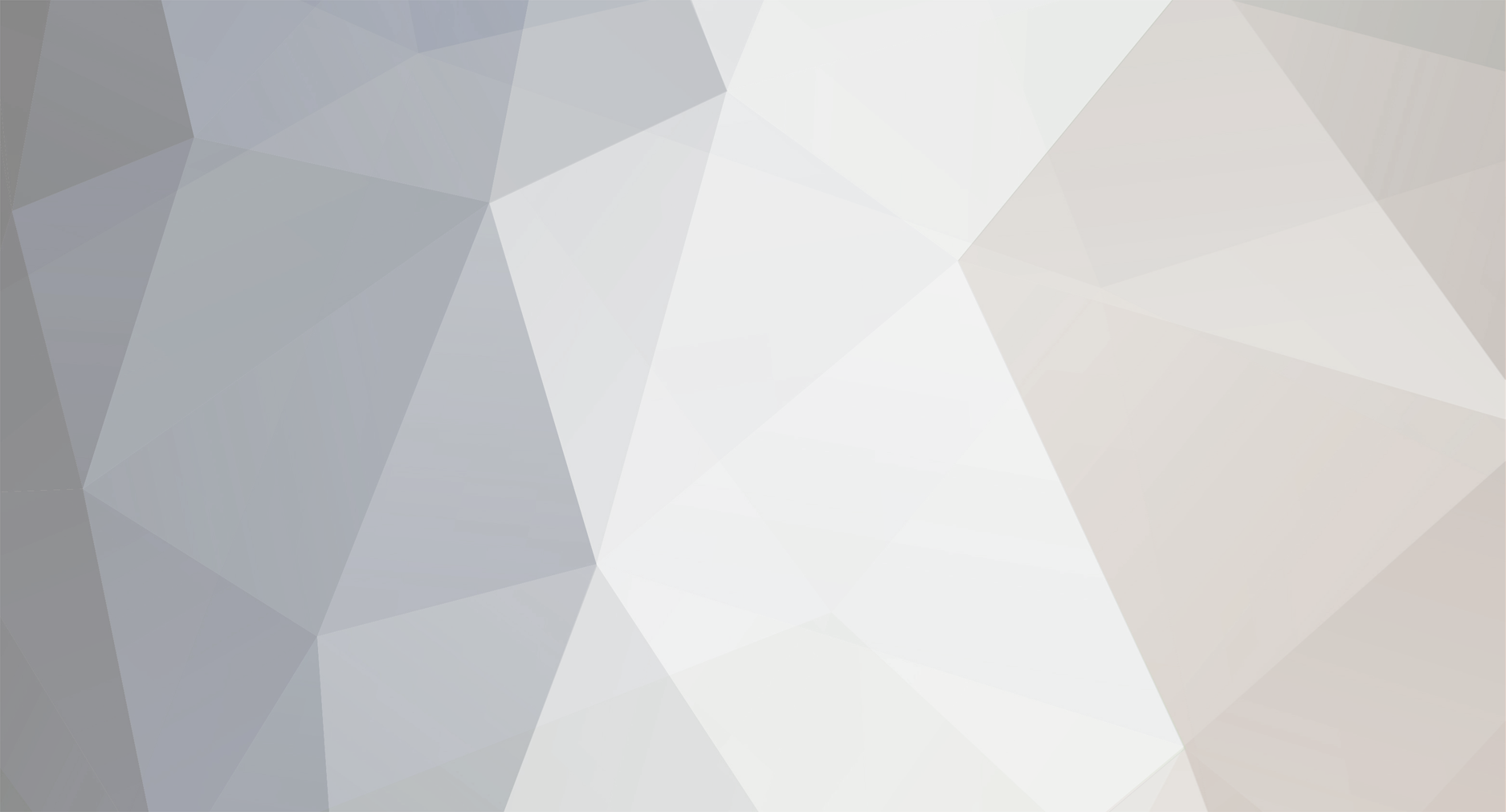
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
I believe that if the user does not provide an answer for one of the groups, that group will not post at all. So you can count the number of answers you get and compare it to the number of questions you sent. // Untested sample code if (count($_POST['answers'])) < $numberOfQuestions) { // NOT ALL OF THE QUESTIONS WERE ANSWERED } else { foreach ($_POST['answers'] as $questionID => $answerValue) { // RECORD THE answerValue FOR THE questionID } }
-
I think you are getting mixed up by the value of $monthly. Start with the calendar for, say May (month = 5). If you output the DIV for next first, your code is incrementing $monthly. So now it is month 6 (June). If you THEN output the DIV for previous, your code is operating on the NEW value (6), so you have to subtract 2 from it to get the month previous to the current one. Since the code for previous is seeing a $monthly value that is one more than the current month (or it may even be the value 1) your if ($monthly == 1) is not correct. It should actually be if ($monthly == 2). It is confusing. If you make that change it should work, but the next time you read through the code to make changes, you will wonder why you did it that way (trust me, we've all been there). The code will be more clear if you actually add a couple of variables and calculations before generating the DIV's: // untested code off the top of my head $nextMonth = $monthly + 1; if ($nextMonth > 12) { $nextMonth = 1; $nextYear = $yearly + 1; } else { $nextYear = $yearly; } $prevMonth = $monthly - 1; if ($prevMonth < 1) { $prevMonth = 12; $prevYear = $yearly - 1; } else { $prevYear = $yearly; } Or something along those lines. Then output $nextMonth and $prevMonth for the DIV events.
-
An AUTO_INCREMENT column has to be numeric (at least in mySql). Since 'T' is a character (not numeric) you cannot prepend it to the ID number in the ID column of the database. What is the datatype of your current ID column? An UNSIGNED BIG INT column can hold 18,446,744,073,709,551,615 numbers (that's a lot). If your current column is NOT a BIG INT, you can ALTER the table to change the datatype and allow for new numbers to use.
-
Do you have short tags turned off? Try changing the short tags to full tags <A href="<?php echo $address; ?>">Go To</A> Edit: Otherwise, tell us what "Does not work." means
-
UNION does not care about number of rows, but it DOES care about columns. All select statements in a union have to have the same number of COLUMNS and they have to be the SAME data-type (in the same order). SELECT id, Latin FROM fag_muscles WHERE Latin LIKE 'P%' UNION SELECT id, Latin FROM fag_bones WHERE Latin LIKE 'P%' Should get you the data you are looking for (as long as the datatypes match up). Of course you will not know where each entry came from, so you could add a literal into the select: SELECT id, Latin, 'Muscles' AS CameFrom FROM fag_muscles WHERE Latin LIKE 'P%' UNION SELECT id, Latin, 'Bones' FROM fag_bones WHERE Latin LIKE 'P%' That being said, I would suggest thinking about redesigning the database. It might be better with a single table and add in a column for Type (muscles or bones). Of course, that depends on how the rest of your database relates to these tables.
-
Congratulations? You did not state a problem or even ask a question, so we don't know what "help" you want.
-
Notices CAN stop a page from working. If a notice occurs before a header() or session_start() or set_cookie() or other function that wants to send a header to the browser, then that function call will fail. Of course you should get a notice or warning (I don't know which). So, look for a message about headers already sent. If you post your code and error messages, we may be able spot some potential problems, but my crystal ball is a bit foggy today.
-
First, don't design your tables with multiple columns containing the same information. This should really be two separate tables, then you could sort the results in the query. To use what you have, you could do a very convoluted IF inside the query, or collect the data in PHP and sort it: $query = "SELECT painting, date1, date2, date3 FROM paintings WHERE artist_id = '$ar' "; $result = mysql_query ($query) or die ("Invalid query: " . mysql_error ()); // collect the data $hold = array(); while ($row = mysql_fetch_assoc ($result)) { if (!empty($row['date1'])) $hold[$row['painting']][] = $row['date1'] if (!empty($row['date2'])) $hold[$row['painting']][] = $row['date2'] if (!empty($row['date3'])) $hold[$row['painting']][] = $row['date3'] } // now sort and output foreach ($hold as $painting => $dates) { sort($dates); echo "The " . count($dates) . " sale dates for ". $painting . " are " . implode(', ', $dates); echo "<br>"; } * This code is not tested and carries no warranty whatsoever.
-
As s0c0 said, you should combine queries whenever possible. You might want to add DISTINCT to the final query in his solution though, otherwise, you will get the same user multiple times (if they have rated more than one story in the list). $q2 = "SELECT DISTINCT username FROM ratings WHERE username NOT IN ('$session->username', 'Anonymous') AND storyId IN (".implode(',',$storyArr).")"; If you are looking for people who have rated ALL (not just ANY) of the same stories as the user, I think this will do it $q2 = "SELECT username FROM ratings WHERE username NOT IN ('$session->username', 'Anonymous') AND storyId IN (".implode(',',$storyArr).") GROUP BY username HAVING COUNT(*) = " . count($storyArr); Also, just a comment on your original query. It is really NOT necessary to say WHERE username IS NOT Anonymous when you are also saying WHERE username IS (current user). Since username can only be one value, if it IS (current user) is IS NOT anonymous -- unless of course the current user IS anonymous in which case your original query will return no rows. $myself = "SELECT storyId,username FROM ratings WHERE username<>'Anonymous' AND username='".$session->username."'";
-
You are still exploding on space, where str_word_count() might give a better answer. You are looking up several words multiple times. This is a waste of resources. You can use array_unique() to get the unique words or use array_count() to get the unique words AND the number of times each word is found. Then just lookup each word once: $words=explode(" ",$msg); // $words = str_word_count($msg, 1); // seems like a better way $uWords = array_count($words); $hpos=0; $pos=0; $nf=0; $stop=0; foreach ($uWords as $word => $count) { // do your database lookup, but instead of incrementing the counters, add $count if($row[1]==1 && $row[2]==0) $hpos += $count; else if($row[1]==0 && $row[2]==0) $pos += $count; else if($row[2]==1) $stop += $count; else $nf += $count; } Also, after loading a large amount of data into the database, you might need to rebuild the indexes (I'm not sure if this is required with mySql Primary Keys or not, but it shouldn't hurt) see ANALYZE TABLE or OPTIMIZE TABLE in the mySql Documentation
-
Take the limits out of the individual queries. Then put the limit OUTSIDE the last closing parenthesis (SELECT stuff FROM somewhere ...) UNION (SELECT more_stuff FROM somewhere ...) LIMIT 10;
-
Typically you would use an array (or database) and a loop to output the options. Then you test inside the loop to see if a each option is chosen: $langList = array('English', 'French', 'German', 'Dutch'); print('<select name="var1">'); foreach ($langList as $lang) { printf('<option %s>%s</option>', ($var1 == $lang ? 'selected="selected"' : ''), $lang); } echo '</select>'; Personally, I use printf() for this kind of work. It just seems to be easier to read. However it could be done as: echo '<option'; if ($var1 == $lang) echo ' selected="selected"'; echo '>' . $lang . '</option>';
-
$x=0; $GrandTotal = 0; while ($x<$numrows){ //loop through the records // all your other code as is $totalpay = $worked*6.40; $GrandTotal += $totalpay; $x++; } } ?> <BR> Total pay so far is: <?php echo"$GrandTotal"?>
-
Since you are outputting the DOCTYPE and HTML and HEAD tags at the beginning of that script, the setcookie() and header() functions are going to fail. If you had error reporting turned on, you would get a message about headers already sent when you call those functions.
-
Question about how to track and display time correctly
DavidAM replied to JeremyCanada26's topic in PHP Coding Help
Personally, I think it best to store all times as GMT in the database. Then if you change servers (and the timezone changes) you don't have a mixed base for time data in the database. To get the GMT value, use the time() function in php. In mysql you can use FROM_UNIXTIME(), or UTC_TIMESTAMP() for inserting/updating data. When retrieving data use UNIX_TIMESTAMP() to convert a DATE/DATETIME column to a unix timestamp. Use date_default_timezone_set() to set the user's timezone, then use the date() function (in PHP) to format. -
Actually, for the record, these are one-to-many relationships. I'm not trying to be negative here, just want to make sure you identify the data model correctly. The standard way to handle this is to keep track of the repeating fields and just not print them if the value is the same as the last record. If setting rowspan is a requirement, you're going to have to use multiple queries, one to get the number of rows and then queries to retrieve and display the data. However, mysql has a function that could help and might produce acceptable results. GROUP_CONCAT(). SELECT id, name, GROUP_CONCAT(child) AS ChildList ... GROUP BY id Which would return a single row for the id (and name) and separate all of the "child" names with a comma in a single field. However, you can specify the separator. If you use the HTML linebreak (<BR>) it would put each child name on a separate line in the (HTML) table when displayed in the browser: SELECT id, name, GROUP_CONCAT(child SEPARATOR '<BR>') AS ChildList ... GROUP BY id
-
The entire script is executed whenever the page is requested. So when the user visits the page for the first time, the form is displayed and your registration script at the bottom is executed even though the form has not been submitted yet. To prevent this, you should check to see if the form was submitted: if (isset($_POST['submit'])) { // test the name of the submit button // Registration code here }
-
You could, but there is no reason to. The name $myArray will only exist inside the method. Once the return is executed and you leave the method, that name would no longer exists.
-
I don't think so. The action attribute tells the BROWSER where to send the data. Without it, the browser would not know where to submit the form. If you leave the action field blank, the browser will submit to the same page as it is already on. You could try to hide it using javascript to submit the form, but then the url would be openly visible in the javascript code; plus some users might have javascript off. The only suggestion I can offer is to submit it to a script that somehow forwards the submission to a different page, perhaps with an additional "secret" value (to prevent people from directly accessing the page). Of course, that page's address will be visible in the browser, unless you post the data using curl and send the user somewhere else. What are you hoping to accomplish and why? We might be able to offer alternatives if we know this.
-
You can accomplish it using GROUP BY but you still need to know how many Q's you have: SELECT cid, SUM(IF(`Q#`=1, marks, 0)) AS Q1, SUM(IF(`Q#`=2, marks, 0)) AS Q2, SUM(IF(`Q#`=3, marks, 0)) AS Q3 FROM tableName GROUP BY cid You could use PHP and another query to determine the number of Q's and dynamically build the SELECT statement.
-
That looks good (if start and end are column names in your table and are defined as DATE or DATETIME). Note that the $date (PHP) variable is not being used and is not the same value as you put in the query. You could add code to print mysql_error() if the query fails so you can see why $date = "2010-11-25"; $query = "SELECT * FROM `Events` WHERE date('$date') BETWEEN start AND end"; $result = mysql_query($query); if ($result === false) { printf("Query Failed:\n%s\nError: %s\n", $query, mysql_error()); } else { // Looks Good, move on
-
This: if (strstr($SQL2,$email)) { echo 3; } else { is checking to see if the email address is in the SELECT statement. It is in the SELECT statement because you put it there with: $SQL2 = "SELECT * FROM newsletter WHERE email = '$_GET[email]'"; You need to check the result of the execution of that query to see if any rows were found. Something like: $SQL2 = "SELECT * FROM newsletter WHERE email = '$_GET['email']'"; $result = mysql_query($SQL2); $emailCount = mysql_num_rows($result); mysql_close($db_handle); } if ($emailCount > 0) { echo 3; } else {
-
You don't need foreach, you just need a straight for: <?php $month = "December"; $days = 31; for($day = 1; $day <= $days; $day++){ echo "<div id='day_block'> ".$day." </div>"; } ?>
-
While servicesid can appear in the table more than once; and articleid can appear in the table more than once; I suspect that the combination of servicesid and articleid needs to be unique. You can create a unique index on these two fields (combined) - in addition to your existing primary key. Then INSERT IGNORE DUPLICATE KEY should work. It is my understanding that these phrases do not care which unique key is being duplicated. On removing rows: If what you have is the articleid's that need to be left in the table you can $list = implode(',', $_POST['articleIDs']); $sql = "DELETE FROM services WHERE servicesid = 5 and articleid NOT IN ($list)"; or something along those lines