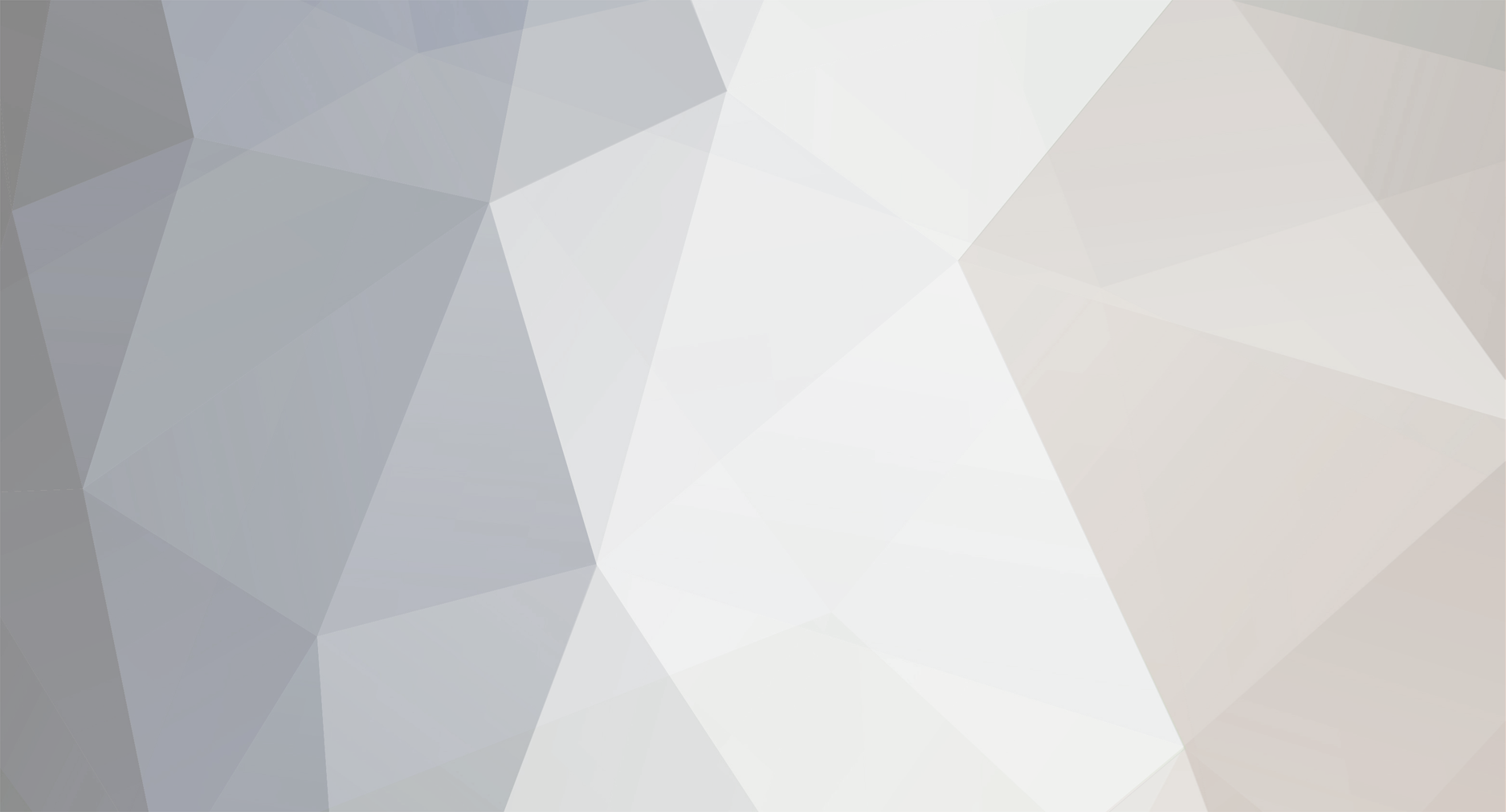
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
UPDATE auto_expire SET expire = (SELECT content_type_ads.nid, auto_expire.nid, content_type_ads.field_expire_value FROM content_type_ads) WHERE content_type_ads.nid = auto_expire.nid This is not going to work. 1) The SELECT is returning multiple values (which cannot be assigned to a single value); 2) The WHERE phrase is referencing a table that is not included in the UPDATE (the subquery does not count, although the WHERE could be moved into the subquery). It could be done using a JOIN: UPDATE auto_expire JOIN content_type_ads ON content_type_ads.nid = auto_expire.nid SET auto_expire.expire = content_type_ads.field_expire_value
-
This code is replacing the $art_id value each time through the loop. So, you are only collecting the last value. while($row = mysql_fetch_array($res)){ $art_id = $row['art_id']; } Collect the values in an array while($row = mysql_fetch_array($res)){ $art_id[] = $row['art_id']; } Of course, then this code is not going to work, since $art_id will be an array: $QUERY2="SELECT * FROM artWork WHERE art_id = '$art_id'"; You will have to use IN and implode() the array $QUERY2="SELECT * FROM artWork WHERE art_id IN (" . implode(',',$art_id) . ")"; This assumes that art_id is a numeric column. If it is a character (or varchar) you will have to provide quotes: $QUERY2="SELECT * FROM artWork WHERE art_id IN ('" . implode("','",$art_id) . "')";
-
You are referencing $user and $sessiontime BEFORE you assign values to them. Switch those lines around: $user = $_GET['user']; $sessiontime = $_GET['clock']; $query = "INSERT INTO sessions (date, user, sessiontime) VALUES ('$date', '$user', '$sessiontime')";
-
If the status column is numeric, the quotes are not required. Apparently mySql allows it though, and some people say it is safer. I'm old school, so I don't put numeric values in quotes.
-
Shouldn't that be: $result4 = mysql_query("SELECT * FROM schedule WHERE eid = '$posteid' AND (status = 1 OR status = 2)"); Otherwise you get all rows where eid == $posteid AND status == 1 along with the rows where status == 2 (regardless of the value of eid)
-
Unless this is a typo, you did not include the code to be executed in braces to be controlled by the IF statement. So, the only thing the IF is controlling is the assignment of $username. <?php if($_POST['submitted'] == 'yes') $username="*****"; Should be: <?php if($_POST['submitted'] == 'yes') { $username="*****"; // REST OF YOUR CODE HERE }
-
You have never assigned a value to $s, so it is always starting at zero. You need to retrieve the value from the $_GET array like you did with the 'p' parameter. You could do something like this: // next determine if s has been passed to script, if not use 0 if (empty($_GET['s'])) { $s=0; } else { $s = intval($_GET['s']); }
-
If you expect to have more than one friend, you can use IN instead of = : SELECT * FROM `posts` WHERE `user_id` IN (SELECT `friend_id` FROM `friend` WHERE user_id = ' #my id ')
-
In your replacement string you are referencing the first captured string, but you are not capturing any strings in your pattern. You need to put parenthesis around the pattern to be captured and you don't put them around the replacement: preg_replace('/(T[0-9]{2}Y[0-9]{2})/',"= \1",$a);
-
Multiplication of a variable - then entry into a table.
DavidAM replied to topshelfbleu's topic in PHP Coding Help
Just assign them to separate variables: $answer1 = $q1 * 3; $answer2 = $q1 * 5; $answer3 = $q1 * 10; $enter_sql= "INSERT INTO answers ($answer1, $answer2, $answer3)"; -
1) You could use non-breaking spaces: Oversize (width x length x height) OR 2) you could use the whitespace property in a span tag: Oversize <SPAN style="white-space: nowrap;">(width x length x height)</SPAN>
-
I can't really tell what your $contacts array looks like; but if it is a simple array of numeric id's you can just implode() it: $sql2="SELECT * FROM thoughts WHERE id IN (" . implode(', ', $contacts) . ") ORDER BY `thoughts`.`time` DESC";
-
Equivalence operator (3 equals signs) means the value is the same and the data type is the same. An empty string or unassigned variable does not have the same data type as a numeric zero. if($var===0){ return $var; }
-
retrieve data from form with some disabled elements
DavidAM replied to RLJ's topic in PHP Coding Help
Forms also do not send un-checked checkboxes (I wish it would -- I think it should -- but it doesn't). If your form method is POST form fields end up in the $_POST array keyed by the element's name. If the form method is GET they end up in the $_GET array. You may have seen old code that made them automatically into variables, but this feature has been depricated and is turned off by default now. While jskywalker's suggestion is correct; it will leave the variable undefined if the form field was disabled when the form was posted. So, expanding that solution you can use an else: if (isset($_POST['var1'])) { $var1 = $_POST['var1']; } else $var1 = ""; } or you can use the ternary operator: $var1 = (isset($_POST['var1']) ? $_POST['var1'] : ""); -
The literal "First Bank Corporation" needs to be in quotes not back-ticks. SELECT `employeeName` FROM `myDB`.`works` WHERE `companyName` LIKE 'First Bank Corporation';
-
You are redirecting based on the value returned by the session_id() function. That function has nothing to do with whether the login succeeded or not. Here is your code with some changes and comments - note: you should use the code ( # ) tags or php ( [ php ] ) tags when posting code on the forum, it makes it easier to read. <?php // ALWAYS USE FULL TAGS, THE SHORT TAGS WILL CREATE PROBLEMS FOR YOU LATER /*simple checking of the data*/ if(isset($_POST['login']) & isset($_POST['pass'])) { // INDENT YOUR CODE SO IT IS EASIER TO READ /*Connection to database logindb using your login name and password*/ $db=mysql_connect('servername','login','password') or die(mysql_error()); mysql_select_db('mpahost_logindb'); /*additional data checking and striping*/ // YOU SHOULD NOT NEED strip_tags() UNLESS magic_quotes IS TURNED ON $_POST['login']=mysql_real_escape_string(strip_tags(trim($_POST['login']))); $_POST['pass']=mysql_real_escape_string(strip_tags(trim($_POST['pass']))); $q=mysql_query("SELECT * FROM login WHERE login='{$_POST['login']}' AND pass='{$_POST['pass']}'",$db) or die(mysql_error()); /*If there is a matching row*/ if(mysql_num_rows($q) > 0) { $_SESSION['login'] = $_POST['login']; $login='Welcome back '.$_SESSION['login']; // DO YOUR REDIRECT HERE SINCE YOU KNOW THE LOGIN IS VALID header("Location: advocates.html"); // success page. put the URL you want // ALWAYS, ALWAYS exit() AFTER A REDIRECT exit(); } else { $login= 'Wrong login or password'; } mysql_close($db); } // ?? header("Cache-control: private"); //avoid an IE6 bug (keep this line on top of the page) // THIS WILL OVERWRITE WHATEVER YOU SET $login TO INSIDE THE IF ABOVE $login='NO data sent'; //you may echo the data anywhere in the file echo $login; ?> Generally, you should do something more for the user when the login fails, like sending them back to the login form.
-
I think you need a space between the minutes and the meridiem. You definitely need to use upper-case "H" for the 24-hour format: $start3='07:30 AM'; $finish3='05:15 PM'; $tstart1 = date( 'H:i', strtotime($start3) ); $tfinish1 = date( 'H:i', strtotime($finish3) );
-
Also, consider the third parameter to explode(). It can be used to specify the number of elements to be generated. $parts = explode(' ', 'Who am I', 2); // $parts[0] will be 'Who' // $parts[1] will be 'am I'
-
send_attendance(this.value); Is sending the VALUE of the SELECT tag to your function. It is NOT sending the SELECT tag itself, so you can't get the ID (the VALUE has no ID). Simply send "this" to the function and use this.value in the function as well as this.id onchange="send_attendance(this); I'm no Javascript expert, but that is my opinion on the matter.
-
Could anyone give me some exercides / challenges?
DavidAM replied to Darkelve's topic in PHP Coding Help
@j.smith1981 Not sure how the printf function works exactly, never really used it. The most basic way to create objects! Not sure where you got the weight from, since you never assigned it. But printf() is a handy tool for writing PHP code that is easier to read, and does not require as much quote escaping. // Which is easier to read to you? printf("The ball's colour is %s, and its weight is %d kilos.",$ball->colour, $ball->weight); // or echo "The ball's colour is {$ball->colour}, and its weight is {$ball->weight}."; // or echo "The ball's colour is " . $ball->colour . ", and its weight is " . $ball->weight . "."; Anyway it's for printing formatted statements. The first argument is the format, and the remaining arguments are replacement variables. Each percent symbol ( '%' ) in the format is a formatting command which is replaced by the next argument in the list. So, in the example '%s' (the 's' indicates a string) is replaced by the value of $ball->colour; and '%d' (the 'd' stands for integer) is replaced by the value of $ball->weight. The formatting commands can have specifications between the '%' and the type ('s') such as '%20s' which will use (at least) 20 character positions for the value. So, in this example, with '%20s', you would get "is green , and". I use the sister function, sprintf(), to build my sql statements. sprintf() returns the string instead of printing it: $sql = sprintf('SELECT product, description, cost FROM products WHERE product = "%s" AND isActive = 1 AND cost BETWEEN %d AND %d', mysql_real_escape_string($_POST['product'], intval($_POST['lowCost']), intval($_POST['hiCost'])); I can look at that code, and read the complete SQL statement at a glance. I can also see all of the variables going into the statement in one place. I just makes the code cleaner (IMHO). [That code is over simplified and assumes an integer cost, but sprintf does support floating point as well]. I also use printf() and sprintf() for outputting HTML code when I need to include variable values in it. Another nice thing about these functions, is that you can reference the replacement variables by an ordinal number, so you can use the same variable in multiple replacements: $sql = sprintf('SELECT product, description, cost FROM products WHERE ( product = "%1$s" OR description LIKE "%%%1$s%%") AND isActive = 1 AND cost BETWEEN %2$d AND %3$d', mysql_real_escape_string($_POST['product'], intval($_POST['lowCost']), intval($_POST['hiCost'])); Here we used the "product" value in two places, without having to supply it to the funtion twice (and we didn't have to escape it twice - saving valuable CPU cycles). You'll also notice the double-percent signs in the LIKE phrase. That basically escapes the '%' so it is placed into the string literally as a percent sign. So the value of $sql from this code, might be: SELECT product, description, cost FROM products WHERE ( product = "computer" OR description LIKE "%computer%") AND isActive = 1 AND cost BETWEEN 500 AND 900 -
$str = require_once('includes/websiteKeywords.txt'); echo $str; // For some reason, the number 1 is added at the end of this string require_once, include_once, include, and require do NOT return the contents of the included file. They output the contents of the included file. So this first line is OUTPUTing to the browser, the text (keywords) contained in that include file. Since the require_once() was successful, it returns true which you have assigned to the variable $str. You are putting the 1 at the end of your keywords, when you echo $str since it contains the value true.
-
Sorry about that, Steve. It's funny that once we find something great we forget that it didn't exist before. Honestly, though, I did not realize VIEWs did not exist in version 4. You can also use a query as a psuedo-table in a query (I think this requires mySql 5 as well). For instance: SELECT * FROM (SELECT * FROM player_season, players, player_positions WHERE player_season.season = '104' AND player_season.player = players.player_id AND player_positions.player_position_id = players.position ORDER BY player_positions.position_order, players.position, players.surname ASC ) AS stafflist WHERE date_left IS NOT NULL which might be better than creating a VIEW. I suggested a VIEW in the first place, because your original question seemed to be talking about a static (non-changing) query. But looking at the followup posts, it looks like your "VIEW" is going to change on every page load. Different page users hitting the page at the same time are going to have a problem trying to create a view that already exists; or dropping the VIEW that is being used by another user.
-
Displaying a price based on drop down selections`
DavidAM replied to craigtolputt's topic in PHP Coding Help
Almost ... $row1 = mysql_num_rows($sql1); //if there is a result it will be either 1 or higher if($row1 > 1) { $price = $_GET['price']; } should probably be $row1 = mysql_num_rows($sql1); //if there is a result it will be either 1 or higher if($row1 >= 1) { // ONE OR MORE ROWS $dbData = mysql_fetch_assoc($sql1); // GET THE DATA FROM THE FIRST ROW // IF $row1 IS GREATER THAN 1, THIS MAY NOT BE THE CORRECT PRICE $price = $dbData['price']; } Also, you are executing the query with the user's data BEFORE you retrieve that data into your variables. The query is going to fail. Move the assignment block above the query. -
@rwwd: I hope you meant that he could use single quotes inside of the double quotes, like this: $sql2 = "insert into att_log (attendee_id, reason, absent_date, call_time, log_date, log_time, logger) values ('$attendee_id', '$reason', '$absent_date', '$call_time', '$log_date', '$log_time', '$login_id') "; Of course, any of those that are numeric do not need the quotes; but the strings and dates DO need them. @New Coder It is possible that the Staff email succeeds, then the INSERT succeeds, then the Attendee email FAILS and the user re-enters the record with basically the exact same data except they put the attendee's email in correctly (or whatever), and post the form again. Look for patterns in the duplicate entries: All the attendees logged have an apostrophe in their name (O'Roark, O'Rielly, O'Niel) or something unusual in the reason or name fields. You are not consistent in the way you collect the POST data. Does the email get sent twice when the INSERT happens twice? I don't see any reason why the INSERT would happen twice unless the entire script runs twice. Could it be that the user is clicking the submit button a second time because it is taking a while and they think that it is not running? Do these duplicate records have the same logtime? If the times are different, then the script must have started a second time because you set that value at the top. You're only logging the hour and minute, so this might not be definitive. If possible, add seconds to that. Of course, it could be happening so quickly that that value might not change either. You might try logging some of your error conditions to a flat file, with a date-time stamp. Then review this log when you find duplicate records. Maybe a particular error is occurring, and the user "knows" that they just have to hit refresh and it will work the second time (or something). Also, review your close_all.php include file. Make sure you are not processing $sql2 in some way under some condition that is causing that query to execute again.
-
It is true that you can specify GET for a FORM action which sends the form fields through the url instead of as POST data. However, I don't see a FORM in your code. It looks like you are trying to submit the form data using a link (<A href="...">). This cannot be done. (well, unless you use javascript). You have to use a submit element to send the form fields either through GET or POST. <FORM method="POST" action="<?php echo "basket.php?action=add&id=1&qty=1" ?>"> <!-- Your table here --> <INPUT type="submit" name="btnAdd" value="Add to Basket"> Note: This example sends the existing paramaters (action, id, and qty) in $_GET and sends the initials (initial_1, initial_2, and initial_3) as $_POST parameters. I just did it that way, because it is cool that you can. You should probably use GET for the form method instead of POST in this particular case, just so you don't get too confused about what's where.