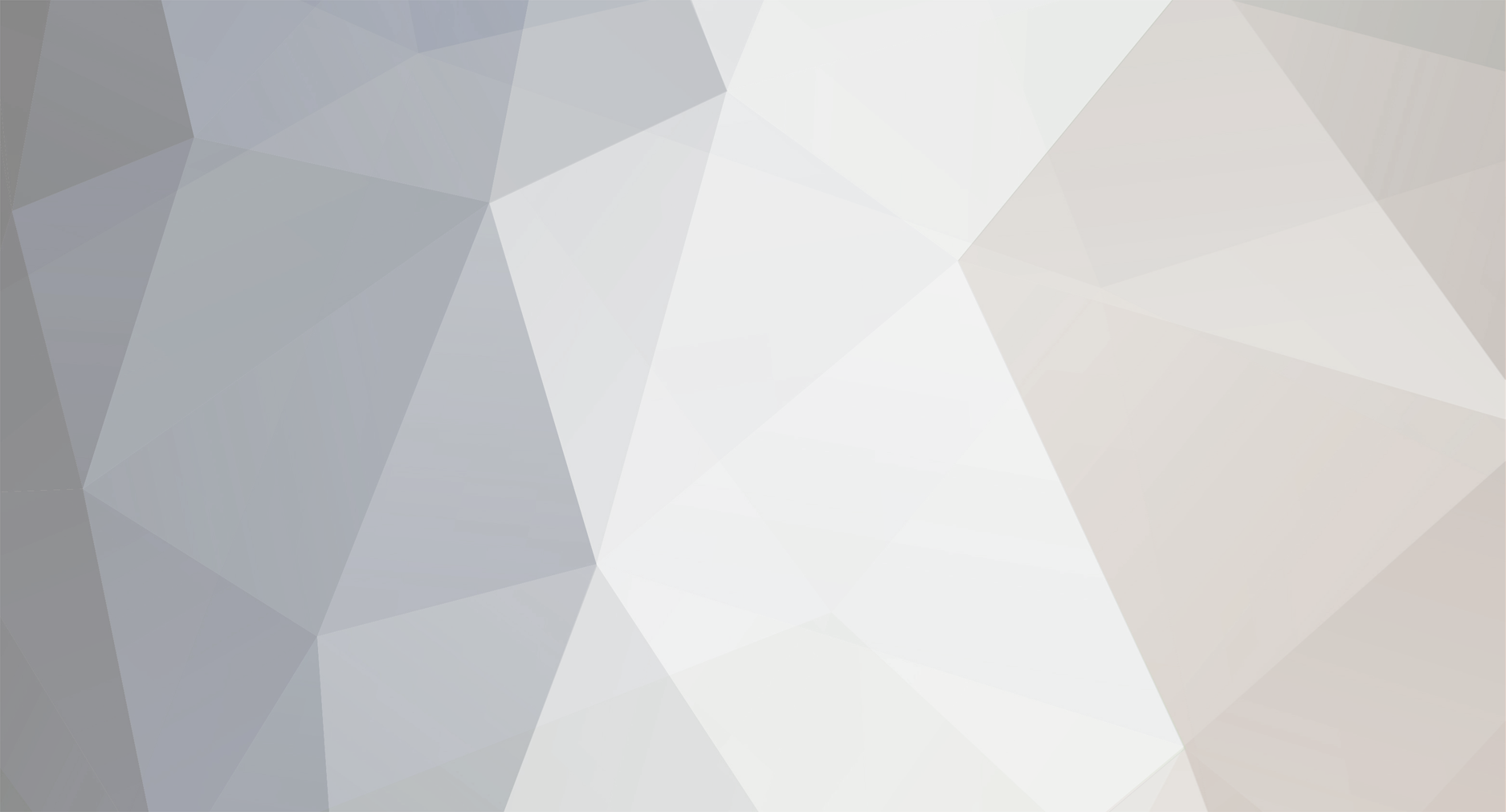
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
Searchstring missing out a row on each set of records
DavidAM replied to MargateSteve's topic in MySQL Help
// Store first three numbers of SEASON NAME into $linkrow $linkrow = mysql_fetch_array($links ); // Fetch the records of the DECADE $row while($linkrow = mysql_fetch_array($links)) { When you call mysql_fetch_array() you retrieve a row from the query result and move the pointer to the next row. So your first call gets the first row and puts it in $linkrow. When you enter the while loop for the first time, you fetch another row (it is the second row in the result set). You never did anything with the first row so it was effectively thrown away. -
You can use READONLY (instead of DISABLED) if you want the field to be visible to the user and POSTed. However, just a word of warning. Do NOT depend on the value in a hidden (or readonly) field of a form. It is a simple matter for the user to change that value to a price he likes better. When the data gets back to the server, you should lookup the correct price or carry it in a session variable.
-
It's possible you left out a comma in the SELECT statement: SELECT id, title album_cover FROM ... this select will put the data from the column named title into a field named album_cover. However, without seeing some code, we can't really tell you what the problem is.
-
You are putting the raw image data into the SQL string. It is very likely that this data contains at least one byte that represents the single quote which you are using to delimit the string (or a NUL byte, or something else). Try wrapping the $image with mysql_real_escape_string(). It is similar to addslashes() but is specific to mysql server. So your INSERT statement would look like this (you should wrap the image name as well): $sql = "INSERT INTO imagedb VALUES ('','" mysql_real_escape_string($image_name) . "','" . mysql_real_escape_string($image) . "')"; that's kinda hard to read, which is why I use sprintf(): $sql = sprintf("INSERT INTO imagedb VALUES ('','%s', '%s')"), mysql_real_escape_string($image_name), mysql_real_escape_string($image) . "')"; Another option is to store it using base64_encode() and decode it (when you retrieve it) with base64_decode(). This will store a string representation so it will take more space. In either case, you are going to have to watch out for the size of the image data. There is a limit on how much data you can send to and retrieve from the database in a single statement. This limit is set in the database server configuration file.
-
Assuming you use POST foreach ($_POST as $fieldName => $fieldValue) { /* PROCESSING $fieldName is the dynamically generated name of the field $fieldValue is the user entered value WATCH OUT, the button you use to submit the form will be here as well */ }
-
You are also going to need parenthesis around the two OR conditions. As it is written, you will get rows that are Customer = Y and PostCode LIKE whatever; along with rows where County LIKE whatever (regardless of the customer flag). $query = "select * from FUNERAL where CUSTOMER = 'Y' AND (POSTCODE LIKE '%$trimmed%' OR COUNTY LIKE '%$trimmed%') order by POSTCODE";
-
I don't think stupid is the correct word to use here. However, eval() can be dangerous. Especially if you are running it on user supplied values. You really need to sanitize the input. Take a look at is_numeric(), intval(), floatval() for the numbers. Also test the operation string to be sure it is one you support (in_array(), strpos(), etc). ManiacDan is right in this respect. It is a trivial matter to POST anything I want to any form on the internet, and if the script does not check the inputs, your system could be compromised your system will be compromised. Having said that, the switch method is safer and probably more efficient. It also has the advantage of intrinsically validating the operation (add a default case to return "ERROR" or something). But you still should test the user inputs, regardless of which approach you take.
-
JOIN Query for 2 Tables - Cannot get a valid result w/out Error
DavidAM replied to OldWest's topic in MySQL Help
Oops. Sorry about that. Since the product_id column exists in more than on table, it has to be qualified by the table name. -
Why build the array every time you call the function, why not just use the constant() function: function blLevelName($value){ $const = 'BL_LEVEL_NAME_' . $value; if (defined($const)) return constant($const); else return 'Unknown: ' . $value; } At the very least use a switch! OP: Your post indicates you are not getting the Referrals. Do they NOT show up at all? or Are you getting the "Unknown" value? If they are not showing up, I would take a look at the INSERT statement that is putting them into the database. This SELECT query should return them if they are there. Is the Status column ever zero? and Do you have similar problems with it?
-
JOIN Query for 2 Tables - Cannot get a valid result w/out Error
DavidAM replied to OldWest's topic in MySQL Help
You have to JOIN the two tables on a common field. Otherwise, you will get a Cartesian product (every one in each table matched with every row in the other table(s)) $query = "SELECT product_id FROM products JOIN product_descriptions ON products.product_id = product_descriptions.product_id WHERE products.status='A'"; -
Note: Since you are limiting the SELECT to one row, you don't really need to use the WHILE loop: <?php ini_set('display_errors',1); error_reporting(E_ALL|E_STRICT); include "connect.php"; $result = mysql_query("SELECT * FROM tickets ORDER BY id DESC LIMIT 1"); echo "<table cellpadding='4' cellspacing='4'><tr> <th>id</th></br> </tr>"; $row = mysql_fetch_array($result); echo "<tr><td>" . $row['id'] . "</td></tr>"; echo "</table>"; $ticketnum= $row['id']; echo "hello" . $ticketnum . "!"; ?>
-
You need another loop to walk through each field in each row. While this is not the way that I would do it, the following code adds this loop similar to the style you were using in your original post. You'll probably want to wrap TABLE tags (and TR / TD) around the output, since I did not put in any spacing (except line breaks). * This code is NOT tested function get_data($table_name){ //Query $result=mysql_query("SELECT * FROM $table_name"); // Output the field names $fldCnt = mysql_num_fields($result); for ($j = 0; $j < $fldCnt; $j++) { $field = mysql_fetch_field($result, $j); echo $field->name; } echo '<BR>'; //Count records $num=mysql_num_rows($result); $i=0; while($i < $num){ // Loop through each field in the result row for ($j = 0; $j < $fldCnt; $j++) { $data = mysql_result($result, $i, $j); echo $data; } echo '<BR>'; $i++; }; };
-
OR $weekdays = array("Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday", "Sunday"); for($i = 0; $i < count($weekdays); $i++) { echo $weekdays[$i] . "<br />"; } * Not really recommended since the count() will be executed every time the loop starts. Or, if you like writing code that is on one line and difficult to read or maintain: $weekdays = array("Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday", "Sunday"); for($i = 0; $i < count($weekdays); echo $weekdays[$i++] . "<br />") ; * Also NOT recommended by the way, if you are going to use next(), you should probably use reset() instead of current() for the first one. If you try to execute the loop a second time without a reset, the pointer will be at the end of the array and you will get an error: $weekdays = array("Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday", "Sunday"); echo reset($weekdays) . "<br />"; $i = 0; while ($i < count($weekdays)) { echo next($weekdays) . "<br />"; $i++; }
-
count($weekdays) is not going to change. It is the number of entries in the array. try using foreach instead $weekdays = array("Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday", "Sunday"); foreach($weekdays as $wkDay) { echo $wkDay . "<br />"; }
-
cannot modify header information >< need help. - cookies
DavidAM replied to Minimeallolla's topic in PHP Coding Help
1) Use code tags [ code ] when posting code 2) see the sticky HEADER ERRORS You can not send a cookie after sending any text -
see the manual for mktime(): The parameters are (in order): hour, minute, second, month, day, year
-
If you have an empty entry at the end of your file ... that is if the last line has a newline at the end of it, this line of code if(empty($entry)){exit;} is terminating your script. It will not exit the while and it will not show any HTML after the loop. An exit is an exit, quit, stop, don't do anything else. You need to break out of the while there.
-
You could use IN for the gender and BETWEEN for the age. SELECT * FROM races WHERE gender IN ($user_gender, 2) AND $user_age BETWEEN age_start AND age_end; Note that BETWEEN includes both ends of the range (so it is >= and <=) If you use the code with the OR in it, you need to put parenthesis around that group or it will be evaluated differently than you think: SELECT * FROM races WHERE (gender = $user_gender OR gender = 2) AND $user_age > age_start AND $user_age < age_end;
-
That's not valid SQL. It would be something like: $query = "select * from FUNERAL where (POSTCODE like \"%$trimmed%\" OR COUNTY like \"%$trimmed%\" ) AND CUSTOMER = 'Y' be sure to include the parenthesis around the two phrases of the OR
-
Can't understand why my function won't work...
DavidAM replied to ibanez270dx's topic in PHP Coding Help
Also remove the '@' sign and erase it from your memory. Hiding errors does not help you. The error you should see is that $connection is not defined. It might be defined outside your function but it is not defined inside the function. -
Hey, you found the semi-colon I lost last night. It's sitting there on your while statement. Its basically creating an empty statement, so the while runs, doing nothing until it has processed all of the rows while($row = mysql_fetch_array($result)); { // ----------------------------------^ Take this semi-colon out The echo's should still be executing after that, so you should get one recipe listed. View the source of the page and see if there is anything there. You might also turn on error reporting at the beginning of your script: error_reporting(E_ALL); ini_set('display_errors', 1);
-
Are you saying that when you receive the email sent by this code, you want to be able to click the REPLY button and send a reply to the email address entered on the form by the user? If so, you need to add a "Reply-To" header: $headers .= 'Reply-To: ' . $_POST['email'] . "\r\n"; You really need to sanitize the user entries through. Especially any that you put in the headers.
-
This might actually be a good project for PHP, but it will involve more than just questions and answers. You'll have to learn how to use sessions as well. Basically, when the script starts, if $_POST is empty, initialize the list of questions and ask the first one. If it is not empty, determine the next question and ask it. This is NOT working code! It is a basic flow (psuedo code) of how the process might work. session_start(); if (! isset($_POST['submit'])) { // First visit - initialize $_SESSIONS['questions'] = range(1, 10); // Range of question numbers shuffle($_SESSIONS['questions']); // Randomize the list $_SESSION['nextIndex'] = 0; // index of next question to ask $message = ''; } else { // user submitted an answer $thisIndex = $_SESSION['nextIndex']; // The index of the question we asked before $thisQuestion = $_SESSION['questions'][$thisIndex ]; // Question # we asked before // Get the user's answer $userAnswer = $_POST['Answer']; $dbAnswer = // SELECT ... WHERE ID = $thisQuestion // SELECT the correct answer from the database $message = ($userAnswer == $dbAnswer ? "Correct" : "Wrong"); $_SESSION['nextIndex']++; // index of next question to ask } // Select the next question $_SESSION['nextIndex'] from the database $thisIndex = $_SESSION['nextIndex']; $thisQuestion = $_SESSION['questions'][$thisIndex]; $dbQuestion = // SELECT ... WHERE ID = $thisQuestion; // Build the form to show to the user This will be a little confusing because the range() function generates an array of question numbers, in the example above, the array will contain the values 1 through 10; but the index (keys) of the array will be 0 through 9. You just have to be careful to NOT use the index as the question number or use a question number as an index. If you use meaningful names for the variables, it will help prevent that problem.
-
New to Javascript, Need help with validation script.
DavidAM replied to atrum's topic in Javascript Help
I guess you will have to use someting like: function valForm(form){ //Verify that none of the fields are blank. if(checkEmpty(form["txt_username"], document.getElementById("user_help")&& -
New to Javascript, Need help with validation script.
DavidAM replied to atrum's topic in Javascript Help
I am NOT a JavaScript expert. But I discovered something last night that might be pertinent to your problem. You are passing to your CheckEmpty() function, the INPUT element and the SPAN element. At least, you think you are. Last night I discovered that LABEL elements are NOT children of the form, they are children of the containing DIV (in my case). I suspect that the SPAN elements are NOT children of the FORM, so you are not passing in an object. Someone let me know if I am wrong here. But it appears to me, an HTML FORM is not treated as a true container. I see this when I try to validate my HTML. The form fields (INPUT, SELECT, etc) must be inside a block level element (i.e. a DIV) or it will not validate even though the FORM itself is inside a DIV, I've had to put another DIV inside the FORM tags. How does that make sense? Sorry, I didn't mean to start a rant in your topic. Try adding an alert(form["user_help"]) just inside your valForm() function and see if it shows undefined.