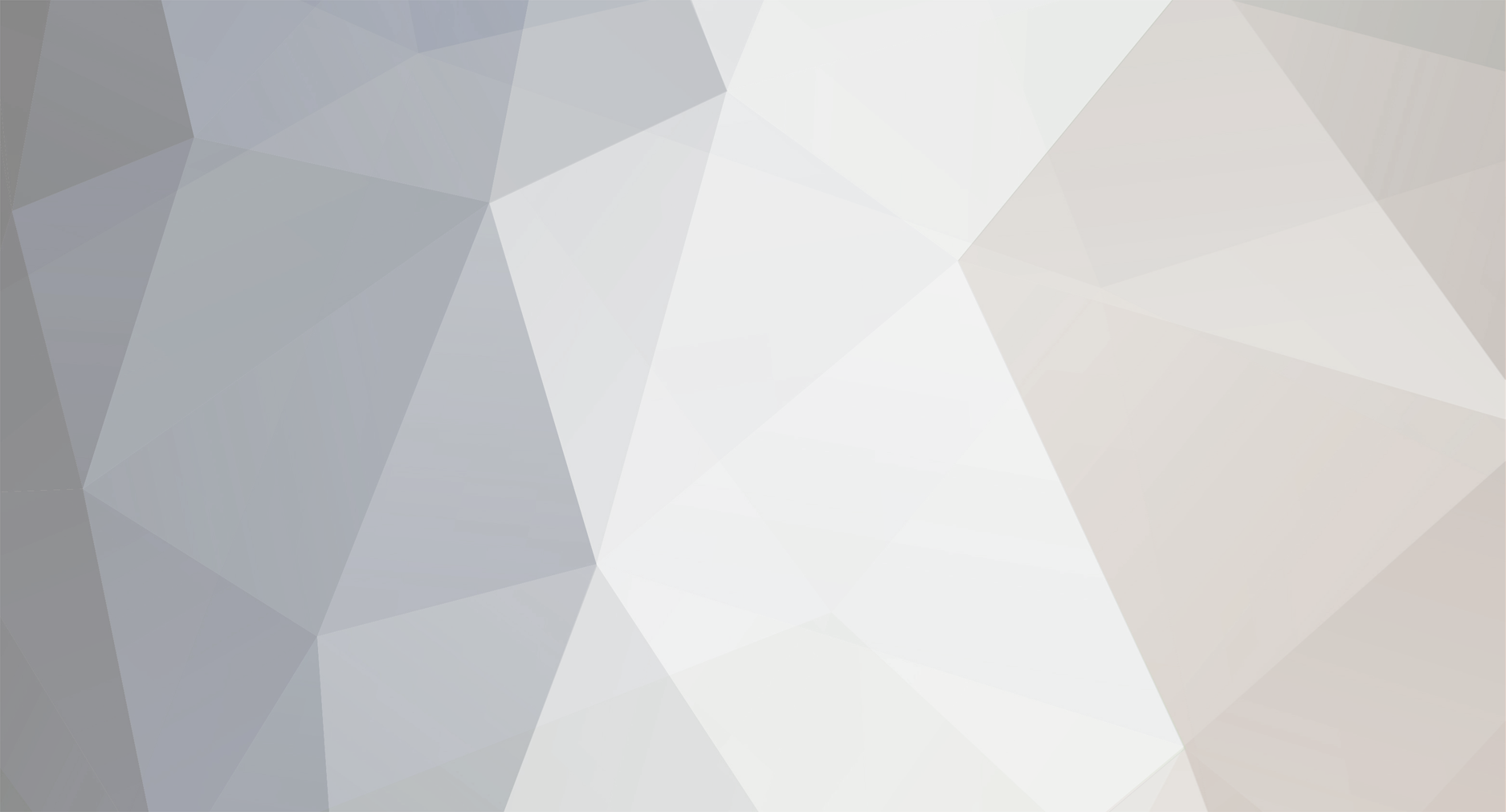
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
or save a couple of steps with: $i = 0; while ($row = mysql_fetch_assoc($result)) { echo ++$i .' CONTENT '.$row['name'].'<br />'; } // At this point, $i contains the number of rows already output while ($i < 50) { echo ++$i .' NO CONTENT<br />'; }
-
Apparently there are some typos in your original code. You retrieve the POST fields into variables, BUT then use different names for the variables in the form: /* Gathering data variables */ $nameField = $_POST['name']; // YOU CALLED THIS $name IN THE CODE BELOW $emailField = $_POST['email']; // YOU CALLED THIS $email IN THE CODE BELOW $stateField = $_POST['state']; // YOU CALLED THIS $state IN THE CODE BELOW $stylesField = $_POST['styles']; // YOU CALLED THIS $style IN THE CODE BELOW $commentsField = $_POST['comments']; // YOU CALLED THIS $comments IN THE CODE BELOW $body = <<<EOD <br><hr><br> Name: $name <br> Email: $email <br> State: $state <br> Style: $style <br> Comment: $comments <br> EOD; There's also a missing "c" in the second $success reference.
-
I don't see why you need a LEFT JOIN. A regular JOIN should do it. 1) Take out the first condition in the WHERE clause, you are just repeating the relationship already stated in the second JOIN ... ON; 2) is locationid a string or a number? Don't you want the name from the location table in the WHERE clause? SELECT HPhoto.filename, HPhotographer.name FROM HPhotographer JOIN HPhoto ON HPhoto.photographerid = HPhotographer.photographerid JOIN HLocation ON HLocation.locationid = HPhoto.locationid WHERE HLocation.name = 'chicago' ORDER BY HPhoto.date ASC;
-
Nothing is ever equal to NULL, not even another NULL. You have to use 'IS NULL' mysql_query(" SELECT * FROM user_post WHERE ( (postby='$cur_user_id' && postfor IS NULL) || (postfor='$cur_user_id') ) ORDER BY postdate DESC LIMIT $num_posts") or die(mysql_error());
-
I believe the manual is saying that using DISTINCT causes the engine to create a temporary table in order to collect the data and determine the unique ones. The user comments do seem to indicate that there is some problems with DISTINCT and ORDER BY. Rather than join the table to itself, I would try: select sponsor from table GROUP BY sponsor The GROUP BY should cause the returned list to be unique. However, is your table really named "table"? Is that a legal name for a table? If that is the table's name try putting it in backticks select distinct sponsor from `table` ORDER BY sponsor ASC" (well, that doesn't make sense, if it was an illegal name, the query should be failing entirely)
-
Linking two columns to the same foreign key
DavidAM replied to MargateSteve's topic in PHP Coding Help
SELECT HT.team_name as HomeTeam, VT.team_name as AwayTeam, AG.home_score, AG.away_score FROM all_games AS AG JOIN teams as HT ON AG.home_team = HT.team_id JOIN teams AS VT ON AG.away_team = VT.team_id Sorry, I have a habit of "aliasing" tables so there is less to type; plus, we had to so we could use the same table twice. I got the table and column names from your post, so they should be correct, I think. You are correct that AG.home_score is of the form table.column. But you will notice in the FROM clause we say we are going to refer to the all_games table AS AG. The 'AG' here is called an alias. Anywhere we use AG to qualify a column, we are referring to the all_games table. We can't use 'all_games' now to qualify a column name, because we said we are going to call it AG. You could take out 'AS AG' and change all of the 'AG.' references to 'all_games.' and the query will be the same. We can't do that with the other two tables though. Since we need two instances of of the teams table, we have to use an alias for at least one of them. -
How to use DATE_SUB() to perform count()'s over multiple datetime periods
DavidAM replied to jayarsee's topic in MySQL Help
Glad to help! I remember the first time (years ago) I saw a query using SUM to do a COUNT and spent a couple of days trying to figure out what it meant. It's actually pretty cool, and effecient. When working on a query, you have to think in SETs and PATTERNs not PROCEDUREs. Sometimes it's difficult to shift the mind-set back and forth between the front-end (procedural) and the back-end (sets), but it sure helps. Mark the topic SOLVED (there's a link down at the bottom of the page). That way, someone else searching will know that there's an answer here, somewhere. Happy Coding -
How to use DATE_SUB() to perform count()'s over multiple datetime periods
DavidAM replied to jayarsee's topic in MySQL Help
So what you really want to know is: For each of these events, how many times did it occur in each of the past several weeks SELECT SE.event_id, FLOOR(DATEDIFF(CURDATE(), SE.event_datetime) / 7) AS WeekNo, COUNT(*) FROM events as SE WHERE SE.event_id IN (12, 13, 14) GROUP BY SE.event_id, WeekNo The WHERE clause specifies the events that you are interested in. You could add a clause here to limit the date range you want ( AND SE.event_datetime BETWEEN '$lowDate' AND '$highDate' ). Note that the BETWEEN phrase is inclusive (includes both the Low and High value). The SELECT clause returns the event ID as well as a calculated week number (weeks ago) and a count of the rows found. The GROUP BY clause causes the COUNT (in the SELECT) to be by event ID and week number. You may have to make some adjustments. Since the WeekNo calculation is based on number of days since the event occured, the count will change from day to day (not just week to week). You may want to look at the mySql WEEKOFYEAR() and WEEK() functions to adjust that. I did not use it, because there will be issues to deal with when run during the first few weeks of any given year. This query will return one row for each event and week. So with three events and a range that covers seven weeks, you could get 21 rows. Note, however, that if an event did not occur AT ALL in a given week (as calculated) you will NOT get a row for that event-week combination. You can combine these rows on the front-end and handle the "missing" row there. If you want the weekly counts in a single row per event, you could do something like this (not as pretty, but just as effective): SELECT ME.event_id, SUM(IF(DATEDIFF(CURDATE(), ME.event_datetime) < 7, 1, 0)) AS Week1, SUM(IF(DATEDIFF(CURDATE(), ME.event_datetime) >= 7 AND DATEDIFF(CURDATE(), ME.event_datetime) < 14, 1, 0)) AS Week2, SUM(IF(DATEDIFF(CURDATE(), ME.event_datetime) >= 14 AND DATEDIFF(CURDATE(), ME.event_datetime) < 21, 1, 0)) AS Week3 FROM events AS ME WHERE ME.event_id IN (12, 13, 14) GROUP BY ME.event_id This "SUMs" the value "1" for each row that fits a specific range; and "SUMs" the value "0" if the row does not fit the range. I don't know if mySql will optimize the DATEDIFF() calculation performing it once per row, or if it will do the calculation (in the example above) 5 times per row. Depending on how much data you have, this could impact performance. You could/should add a date range in the WHERE clause here, too. You might be able to "optimize" the days calculation by using a psuedo-table (depends on your version of mySql): SELECT ME.event_id, SUM(IF(DE.DaysAgo < 7, 1, 0)) AS Week1, SUM(IF(DE.DaysAgo >= 7 AND DE.DaysAgo < 14, 1, 0)) AS Week2, SUM(IF(DE.DaysAgo >= 14 AND DE.DaysAgo < 21, 1, 0)) AS Week3 FROM events AS ME JOIN (SELECT SE.event_id, DATEDIFF(CURDATE(), SE.event_datetime) AS DaysAgo FROM events AS SE WHERE SE.event_id IN (12, 13, 14) AND SE.event_datetime BETWEEN '$lowDate' AND '$highDate') AS DE ON ME.eventID = DE.eventID WHERE ME.event_id IN (12, 13, 14) AND ME.event_datetime BETWEEN '$lowDate' AND '$highDate' GROUP BY ME.event_id In this one, we build a psuedo-table of event_ids and DaysAgo (that it occured). Then join that to the main query to get the weeks as columns. I think the event id and datetime limits need to be in both places for optimum performance. You might also need an index on the datetime. You'd have to run EXPLAIN on the query to see what might improve it. -
oops, sorry. I keep forgetting that int is not a function in php. It should be intval() $chunks = intval($OrderWeight / 150); $leftover = $OrderWeight % 150; This gives you a count of the number of times your multiplier is in the total and a leftover. To output it, you would print the multiplier $chunks times and then print the leftover: $mult = 150; // Our multiple for breaking down the value $total = 340; // The value we are starting with $chunks = intval($total / $mult); // How many multiples are in the total $left = $total % $mult; // What is left over (modulus operator) for ($i = 1; $i <= $chunks; $i++) { printf('..%d..', $mult); } if ($left > 0) printf('..%d..', $left); echo PHP_EOL; ?> OUTPUT: ..150....150....40.. If you need the values in an array for some reason, you can do something like this: $mult = 150; // Our multiple for breaking down the value $total = 340; // The value we are starting with $chunks = array(); // Start with an empty array $breakDown = $total; // Just so we don't wipe out our starting value while ($breakDown > 0) { if ($breakDown >= $mult) { $chunks[] = $mult; $breakDown -= $mult; } else { $chunks[] = $breakDown; $breakDown -= $breakDown; } } printf('%d contains the following: ', $total); foreach($chunks as $chunk) printf('..%d..', $chunk); print PHP_EOL;?> OUTPUT: 340 contains the following: ..150....150....40..
-
Linking two columns to the same foreign key
DavidAM replied to MargateSteve's topic in PHP Coding Help
You have to join to the teams table twice, using a different alias for each occurrence of the table: SELECT HT.team_name as HomeTeam, VT.team_name as AwayTeam, AG.home_score, AG.away_score FROM all_games AS AG JOIN teams as HT ON AG.home_team = HT.team_id JOIN teams AS VT ON AG.away_team = VT.team_id -
$chunks = int($OrderWeight / 150); $leftover = $OrderWeight % 150;
-
If you are going to mix AND with OR you have to mark the groups that go together (using parenthesis): SELECT parts_table.*, manufacturers.* FROM parts_table, manufacturers WHERE (parts_table.ManufacturerID = manufacturers.ManufacturerID AND (parts_table.PartNumber LIKE '%siemens%' OR parts_table.PartNumber LIKE '%6SN2460-2CF00-0GB0%')) OR (manufacturers.ManufacturerID = parts_table.ManufacturerID AND (manufacturers.ManufacturerName LIKE '%siemens%' OR parts_table.PartNumber LIKE '%6SN2460-2CF00-0GB0%')) Actually, if you use a JOIN here, you can eliminate that issue since the ManufacturerID comes out of the WHERE clause: SELECT parts_table.*, manufacturers.* FROM parts_table JOIN manufacturers ON parts_table.ManufacturerID = manufacturers.ManufacturerID WHERE parts_table.PartNumber LIKE '%siemens%' OR parts_table.PartNumber LIKE '%6SN2460-2CF00-0GB0%' OR manufacturers.ManufacturerName LIKE '%siemens%'
-
MySQL strangeness, table saves intermittently...
DavidAM replied to xtian's topic in PHP Coding Help
To get the values in POST when the user submits the corrected form, they need to be in fields on the form (LABELs are not POSTed). If you want to prevent the user from changing a field, use the same field tag you did in the original form but with the READONLY attribute: <INPUT type="text" name="txtFName" value="$_POST['txtFName']" readonly="READONLY"> Or you can use a hidden field to prevent the user from even seeing it: <INPUT type="hidden" name="txtFName" value="$_POST['txtFName']"> Do not use the DISABLED attribute, since some (most?) browsers do not POST disabled fields. -
Getting a loop to simply write "static" code ??
DavidAM replied to ThunderVike's topic in PHP Coding Help
ThunderVike, I think I finally get it. You are trying to collect the code before the form is displayed because the $_POST array will not contain the checkbox name array if they are all unchecked. I'm not sure if you can make this work, since when you display the form the script ends and that code you collected will no longer exist. Maybe you can add a hidden field to the form that contains a comma-separated list of names of the checkboxes you expect to get back: <INPUT type="hidden" name="cp_checkbox_names" value="cp_checkbox_ONE,cp_checkbox_THREE,cp_checkbox_SIX"> The field is hidden so the user does not see it. Then when you get the post you can check to see if this hidden field exists, and process the names you find in it: if (isset($_POST['cp_checkbox_names'])) { $cb_names = explode(',' $_POST['cp_checkbox_names']); foreach ($cb_names as $cb_name) { if (!isset($_POST[$cb_name])) $_POST[$cb_name] = array(); } } Then the loop process we worked out before should find the checkbox array in $_POST and find it empty and clear them out. -
The difference will be in bandwidth used and browser processing. When you set the width and or height of an IMG tag, that ONLY changes the way it displays (the browser has to shrink or stretch the image) it does NOT change the amount of data sent to the browser. So, if you use a single image, and let's just say it is 1.4MB, then it will ALWAYS transfer 1.4MB from the server to the browser regardless of how big the browser displays it. This is a lot of data for slow connections, and chews up your bandwidth allowance (if your hosting plan has a limit). If you resize the images on upload, you are using server CPU time (once) and disk space for storage, but then when you send an image to the browser, you are sending less data (using less bandwidth) with the "60" size than with "800" size.
-
using a database to store username/password combos.
DavidAM replied to amplexus's topic in PHP Coding Help
You need to assign the values to the $username, $password, and $real_name variables. When you POST a form, the fields end up in a super-global array called $_POST. The keys to the array are the field names and the values are the user entered values. There used to be a setting to have this done automatically, but that setting has been depricated, turned off by default, and will go away in the next release. There were some serious security issues with it. Also, you need to protect against SQL injections, so you need to run the POSTed values through mysql_real_escape_string(). So, before your insert statement you need something like this: $username = mysql_real_escape_string($_POST['username']); $password = mysql_real_escape_string($_POST['password']); $real_name = mysql_real_escape_string($_POST['real_name']); $query = "INSERT INTO $newdbname VALUES ('','$username','$password','$real_name')"; -
using a database to store username/password combos.
DavidAM replied to amplexus's topic in PHP Coding Help
Just before the INSERT statement: $password = md5($password); // SEE NOTE BELOW $query = "INSERT INTO $newdbname VALUES ('','$real_name','$username','$password')"; The hash in my example is NOT sufficient. You want to read up on this and pick an algorithm and a seed that will provide the security you want. On a side note. I see that you are using the same username and password variables for your database login and the insert statement. Is this what you intended? mysql_connect($servname,$username,$password); $query = "INSERT INTO $newdbname VALUES ('','$real_name','$username','$password')"; Usually, you have a single database username and database password which have no relation to the users you are allowing in the application. -
using a database to store username/password combos.
DavidAM replied to amplexus's topic in PHP Coding Help
First things first. When storing the password in a database (or a file for that matter) do NOT store it in the clear (that is, as entered by the user). Store a hash of the password. That way if someone hacks the database and reads your users table, they do not have the user's password. Why? Lots of users use the same password for many sites, if someone can get passwords from your database, they might use it to get into some other site. And if they got someone's password from another site, it should not hash to the same thing on your site. Ok. On to your question. The process is fairly straightforward: if the form was POSTed if they did not enter ALL the data (both username and password) set an error message elseif there is not a match in the database set an error message (do NOT tell the user which one is wrong) else record the fact that the user is logged in (use a session) send them off to another page endif endif display the form (with error message) When you use this approach, the form and the login code are in the same file. So the ACTION in your form tag will be the same filename (i.e. "/login.php") as the one that generates it. Do NOT use PHP_SELF or an empty string (""). The safest action value is a hard-coded absolute pathname. You need to use a session to track the fact that they are logged in when you get to the next page. filename:login.php (for instance) <?php // During development, we always want to see any errors or warnings error_reporting(E_ALL); ini_set('display_errors', 1); // If your form was POSTed, the button name will be in the POST array if (isset($_POST['Submit'])) { // Was the form posted? if ( (empty($_POST['username'])) or (empty($_POST['password'])) ) { // Is either field missing? $msg = 'Please enter your username and password'; } else { // Need to check the database include("dbinfo.inc.php"); if (! mysql_connect($servname,$username,$password)) { $msg = 'Database access error. Try Again Later'; } elseif (!mysql_select_db($database) ) { $msg = 'Database access error. Try Again Later'; } else { // OK we're connected $sql = sprintf("SELECT id FROM users WHERE username = '%s' AND password = '%s'", mysql_real_escape_string($_POST['username']), md5($_POST['password'])); // Use the same hash algorithm used to store the password $res = mysql_exec($sql); if ($res === false) { // Did the query fail? $msg = 'Database access error. Try Again Later'; // For development you can add: $msg .= mysql_error(); } elseif (mysql_num_rows($res) != 1) { // There should only be 1 matching record $msg = 'Invalid Username or Password'; } elseif (! $row = mysql_fetch_assoc($res)) { // $row is FALSE if we failed $msg = 'Database access error. Try Again Later'; } else { start_session(); $_SESSION['UserID'] = $row['id']; header('Location: /index.php'); // send them to the next page exit; // ALWAYS EXIT AFTER A HEADER() REDIRECT } } } } // Now your form - Almost exactly like you had it ?> <h1>Login</h1> <form name="form" method="post" action="/login.php"> <p><label for="txtUsername">Username:</label> <br /><input id="txtUsername" type="text" title="Enter the new Username" name="username" /></p> <p><label for="txtpassword">Password:</label> <br /><input id="txtpassword' type="password" title="Enter the user password" name="password" /></p> <p><input type="submit" name="Submit" value="Login" /></p> </form> <?php if (!empty($msg)) { echo '<P>' . $msg . '</P>'; } Notes: I added the message at the bottom (below the form) In your INPUT tags, you needed an ID attribute with a value that matches the FOR attribute in the LABEL I guessed at the name for your user's table, as well as the columns in that table. Now you have let the user login. On any page that requires a logged-in user, you have to add (near the top of the file): session_start(); if (empty($_SESSION['UserID'])) { header('Location: /login.php'); exit(); } That checks to see if they have logged in and will send them to the login page if they have not. Disclaimer: This code is not tested. I typed it off the top of my head. It may contain syntax errors, typographical errors, logic errors or alzhiemers errors. The code's not pretty, and may not be the most efficient. Even so it is worth at least twice what you paid for it. -
using 'order by' to order data from two text columns
DavidAM replied to theanteater's topic in MySQL Help
If it is just the names you want, I think this would work: SELECT name_col1 AS name_col FROM table WHERE a=$b UNION SELECT name_col2 AS name_col FROM table WHERE a=$b ORDER BY 1 You will not know which is which. Of course you could add a couple of fields: SELECT name_col1 AS name_col, id, 'col1' AS which_name FROM table WHERE a=$b UNION SELECT name_col2 AS name_col, id, 'col2' AS which_name FROM table WHERE a=$b ORDER BY 1 -- Orders by the first column -
using 'order by' to order data from two text columns
DavidAM replied to theanteater's topic in MySQL Help
You just separate them with a comma ORDER BY name_col1, name_col2 -
trying to add time stamp to file name to make it unique
DavidAM replied to scmeeker's topic in PHP Coding Help
I just glanced through the code quickly. I see two lines that need to be changed: imagejpeg( $desired_gdim, "image_files/".'$timestamp'.$_FILES["thumb"]["name"] ); // AND A FEW LINES BELOW THAT ... $item_image = '"$timestamp"'.$_FILES['thumb']['name']; If you put single quotes around a variable name, it is NOT interpreted as a variable -- you get the variable name NOT the value. If you put double quotes around a variable name, it is interpreted and you get the value. You are NOT REQUIRED to but quotes around variable names -- the double-quote interpretation is for convience in building strings. The two lines I mentioned could be re-written as: imagejpeg( $desired_gdim, "image_files/" . $timestamp . $_FILES["thumb"]["name"] ); // AND A FEW LINES BELOW THAT ... $item_image = $timestamp .$_FILES['thumb']['name']; Also just above that second line, you have changed $timestamp to the current time again. This could be a different value from the one you retrieved at the top of the script, so your filenames may not match up anymore. Take this second assignment out unless you are intentionally trying to change the filename - which is not going to work if the script runs in under 1 second. -
I don't know of any other way to do it that does not involve javascript. And you shouldn't use JS for required page behavior since users may turn it off, or use a browser that does not support it. When you enter your image src url in the browser address bar, what exactly happens? Do you get an error message, blank screen, or what (if you get a blank screen, view the page source and see if it is blank as well). Also, for testing purposes, take the '@' out of the function calls ($img = @imagecreate ...) it could be hiding errors. If it works without the wordpress validation, add those lines back one at a time and see where it is failing. add these two lines to the top of this script, immediately after the openning php tag: error_reporting(E_ALL); ini_set('display_errors', 1); just to make sure we can see any errors.
-
Oops! Sorry, I forgot about the hours thing. Looks like you fingured it out though. By the way, click the "Solved" button at the bottom of the post so others can see that there is a solution to the question. It's almost at the bottom of the page.
-
You are talking about the "Query String". Everything after the ? in the url is a query string that provides parameters to your script. You get a "super-global" array called $_GET. In your example: http://www.facebook.com/home.php?sk=h $_GET['sk'] would contain the value 'h'. Multiple values can be provided by separating them with an ampersand ('&') http://www.facebook.com/home.php?sk=h&id=123 In this case $_GET['sk'] is 'h' and $_GET['id'] is 123. Your script can make decisions based on the values in the $_GET array.
-
If the user column is not a number type, you have to put the string you are looking for in quotes: SELECT * FROM projects WHERE user = '{$user}'