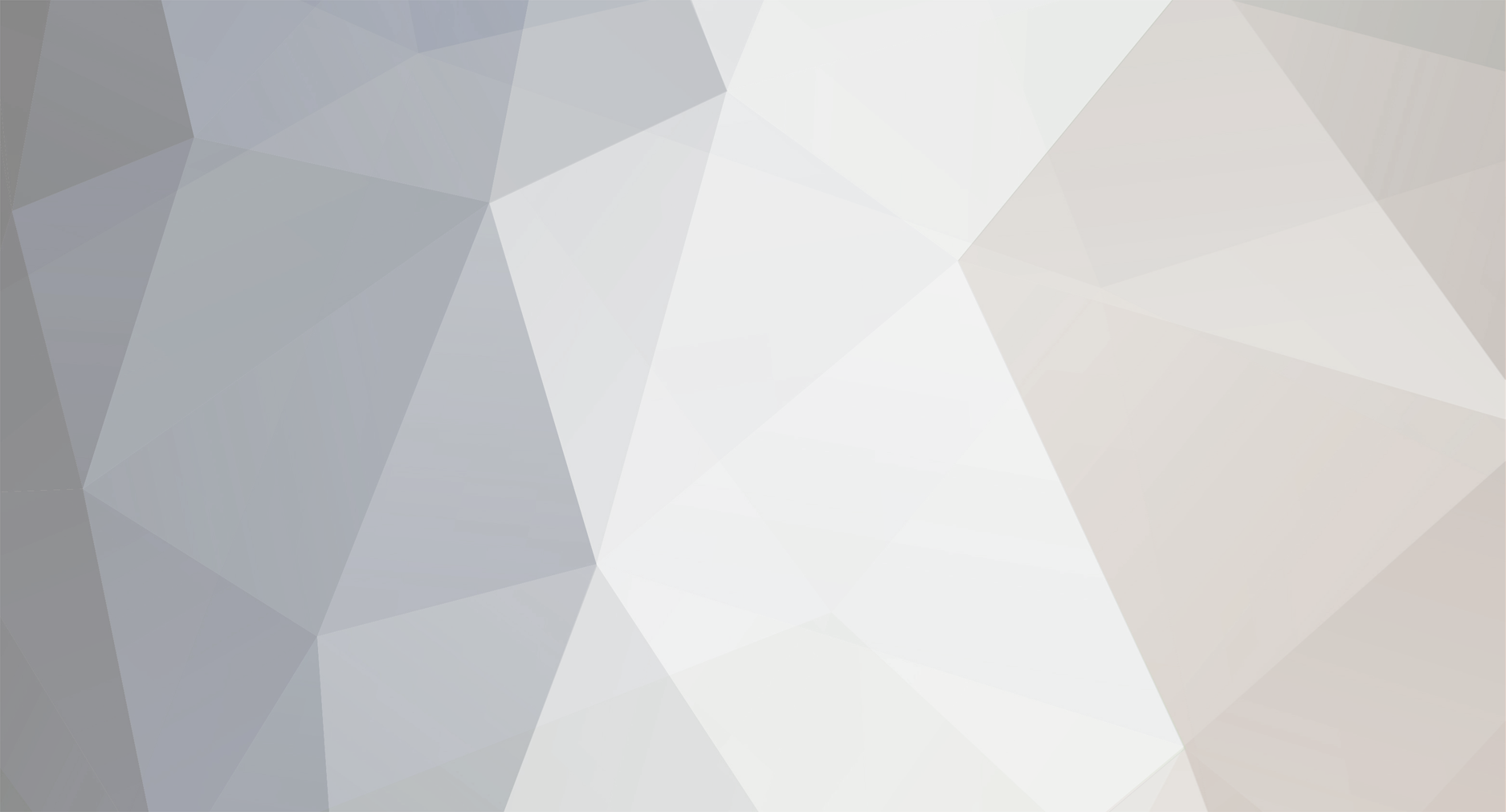
jcbones
Staff Alumni-
Posts
2,653 -
Joined
-
Last visited
-
Days Won
8
Everything posted by jcbones
-
Help with javascript gallery/lightbox with PHP and MySQL
jcbones replied to Millertime31's topic in PHP Coding Help
Are you using lightbox2? If so, you just have to give all the anchors the same name in the data-lightbox attribute. http://http://lokeshdhakar.com/projects/lightbox2/- 2 replies
-
- mysql
- javascript
-
(and 3 more)
Tagged with:
-
There are many different design patterns for MVC, and you can go as in depth into it as you want. A simple pattern would be a single entry point for the user, which determines through the controller where to route the user, grabbing the data layer from the model, and sending that data to the same place that it routed the user. <?php $controller->routeUser(); $data = $controller->model->data(); ?> **NOTE** The following is IMHO, nothing more! Now you could take it a lot further than that, but really deep architecture is to much overhead for small or medium projects. Unless you are doing a "facebook" thing, the upfront coding time outweighs the benefits. I like the way that CakePHP does things, although, even they are a little deep for smaller projects. I know that more than 10 people here would disagree with me, but that is life.
-
Just set the division width, and they will automatically stack on each other.
-
You are selecting your database with a mysqli function, and using mysql on the rest of it. You cannot mix and match the database functions like that. It is either 100% mysqli, or 100%mysql. At the end of your $query string, you're missing the closing semi-colon (.
-
As Thara stated, another way (in keeping with your code) is: Edit: this forum software doesn't like linux firefox. It keeps dropping half of what I write! Change $sql = "UPDATE session_notes SET notes = '$notes', notes2 = '$notes2', notes3 = '$notes3' WHERE appointment_id = '$appointment_id'"; $sq2 = "INSERT INTO provider_submit SET provider_id = $provider_id"; if(mysql_query($sql1) && msyql_query($sql2)){
-
data is not being inserted i get query failed?
jcbones replied to jrphplover's topic in PHP Coding Help
Change this line: $result = @mysql_query($qry); To: $result = mysql_query($qry) or trigger_error(mysql_error()); Make sure your error reporting is on, if it isn't then use: $result = mysql_query($qry) or exit(mysql_error()); -
cURL and $_FILES working on Windows but not Linux...
jcbones replied to rayman0487's topic in PHP Coding Help
No, as in copy/paste it here. -
cURL and $_FILES working on Windows but not Linux...
jcbones replied to rayman0487's topic in PHP Coding Help
Can you post just the form? -
Godaddy always uses remote database connections (I hate godaddy, and this is one of the many reasons). So, assuming that you have created the database (and waited the hour or two that it needs to be active), log in to your control panel, go to databases, then actions, then details. Your hostname should be there, and that is what you should have for your DB_SERVER constant. You then need to change your file back to what it was originally, and put your changes wherever your constants are defined.
-
Seeing the error points to a class called mysqli_ext, I would say this class is dependent on that class. $foundRows should contain the resultSet from your query.
-
cakephp - get results from jquery within controller function
jcbones replied to coderhut's topic in PHP Coding Help
All Google API request are HTTP request, and returned as JSON or XML. I am assuming that $allRideOfferPlaces is an array that contains reference codes from previously pulled places? I also noticed that there is not an option to send in batches of references. Reference: Google Place API Detailed *note* There is a missing ? and a missing index in the code below. So you need to go through it before you use it. function getPlaces() { $allRideOfferPlaces = $this->rideOffer->find('all'); //set return of method to variable. $requestParams[] = 'key=myKey'; //make sure you put your key here: $requestParams[] = 'sensor=false'; //This device doesn't have GPS. foreach($allRideOfferPlaces as $place) { //foreach array value (should be references). $requestParams['reference'] = 'reference=' . $place; //assign the reference key (changes on each request). $googleApiUri = 'https://maps.googleapis.com/maps/api/place/details/json' . implode('&',$requestParams); //assign the params to the request URI $json = file_get_contents($googleApiUri); //send the request; $decoded = json($json,true); //decode the json into an array: $results[] = $decoded; //add the results to an array; //lat and long of this place: echo $decoded['name'] . ' is located at Lat: ' . $decoded['results']['geometry']['lat'] . ' and Long: ' . $decoded['results']['geometry']['lng'] . '<br />'; } echo '<pre>' . print_r($results,true) . '</pre>'; } -
how to select from 2 tables and display data
jcbones replied to hance2105's topic in PHP Coding Help
Remove this line: $row=mysql_fetch_array($query); There is no call to that line, and all it is doing is moving the internal pointer to the second row. So subsequent calls to mysql_fetch_array() will continue from the second row. Thereby making you think that the first row has been skipped. -
REMOVE these 2 lines: $row = mysql_fetch_array($result); //and $row2 = mysql_fetch_array($result2); They are moving the internal index pointer to the second row. So if you only have 1 news row, then you wouldn't see it. Also, PHP will not show MySQL errors, unless you return them from the MySQL server. $result2 = mysql_query($sql2) or trigger_error(mysql_error); Finally, for safer, more secure coding, you should migrate to PDO or mysqli classes. Edit: URL BBCODE not working???
-
How to create an add button to add all details at once to mysql table
jcbones replied to hance2105's topic in PHP Coding Help
First you would build your form different, using arrays: <?php session_start(); include('db_connect.php'); $sql = mysql_query("select * from tblproduct"); $num = mysql_num_rows($sql); if($num>0) { echo "<form name=\"price\" method=\"post\" action=\"ret_addprod.php\">" ."<center><table bgcolor='grey' width='80%' border=0>" . "<tr bgcolor='#CCCCCC'>" . "<td><b><center>Product</td>" . "<td><b><center>Name</td>" . "<td><b><center>Brand</td>" . "<td><b><center>Price</td>" . "</tr>"; while($row = mysql_fetch_assoc($sql)){ echo "<tr>" .'<td style="text-align:center;"><img height="100" width="100" src="Images/Products/'.$row['prod_photo'].'"/></td>' . "<td style='text-align: center;'>".$row['prod_name']."</td>" . "<td style='text-align: center;'>".$row['prod_brand']."</td>" . "<td>" ."<input type=\"hidden\" name=\"prod_id[]\" value=".$row['prod_id']." />" ."<input type=\"text\" name=\"prod_price[]\" />" ."</td>"; } echo "</table>"."<input type=\"submit\" value=\"Add\" /></form>" ."<center><a href ='retailer_home.php' >Home</a>"; } else{ echo "No products found."; } ?> Then on: ret_addprod.php You would have a processing portion like: <?php include('db_connect.php'); if($_SERVER['REQUEST_METHOD'] == 'POST') { foreach($_POST['prod_id'] as $index => $id) { $sql = sprintf("UPDATE tblproduct SET prod_price = %f WHERE prod_id = %d", $_POST['prod_price'][$index], $id); if(mysql_query($sql)) continue; exit('There was an error in your syntax<br />' . mysql_error()); } } ?> I don't really like running queries in loops. The only way to make it work without looping the queries, would require a huge case statement. If there is a problem with overhead (shouldn't be, unless your server is remote from the db server) perhaps we could write that out. Edit: I'm an idiot, mixed the syntax for sprintf and mysqli->prepare -
Need Help with my code, It will not execute.
jcbones replied to newguyneedshelp's topic in PHP Coding Help
Are you trying to execute the file using a server, or just a file on your computer? -
It sounds like you are on your way to normalisation, at least, in part. Just don't forget that databases can still bog down with a normal dataset. Your queries need to be optimized, and don't forget the indexes, as both can have adverse effects.
- 10 replies
-
- databasemysql
- tables
-
(and 1 more)
Tagged with:
-
There is no way of even giving a clue without first seeing code snippet, at the very least. This is because of the vast amount of ways you could interact with databases in PHP.
-
I don't understand why this isn't doing anything.
jcbones replied to iWareWolf_'s topic in PHP Coding Help
Mysql can't return something it doesn't have. So of course it will stop at the data call. Add some test data, test it, then comw back here with any questions. -
Return error in PHP when mysql duplicate entry is found
jcbones replied to benoit1980's topic in PHP Coding Help
I would check the mysqli_errno to see if it is returning a duplicate key error. Not sure of number for that specific error, but you can find it in the mysqld_error.txt file in the docs folder of your installation. -
Correct Max, but that will not cause the query to fail. It is just bad form, and another reason that people should have already migrated to MySQLI, and/or PDO. In fact when I was a yongling, I was instructed to always insert integers as strings, to help combat injection attempts.. Being that it was a security firm requesting it, I did it that way for a few years before I was 'learned' better.
-
You need to apply CSS styles to the <td>s that need to be black. Unfortunately there is not enough info to help you further.
-
In order to implement the changes in the above post, which is the correct way to do it, then you need to set a hidden input in the form with the post id. Also, some browsers don't send submit button info the same as others, so I would suggest using $_SESSION['REQUEST_METHOD']