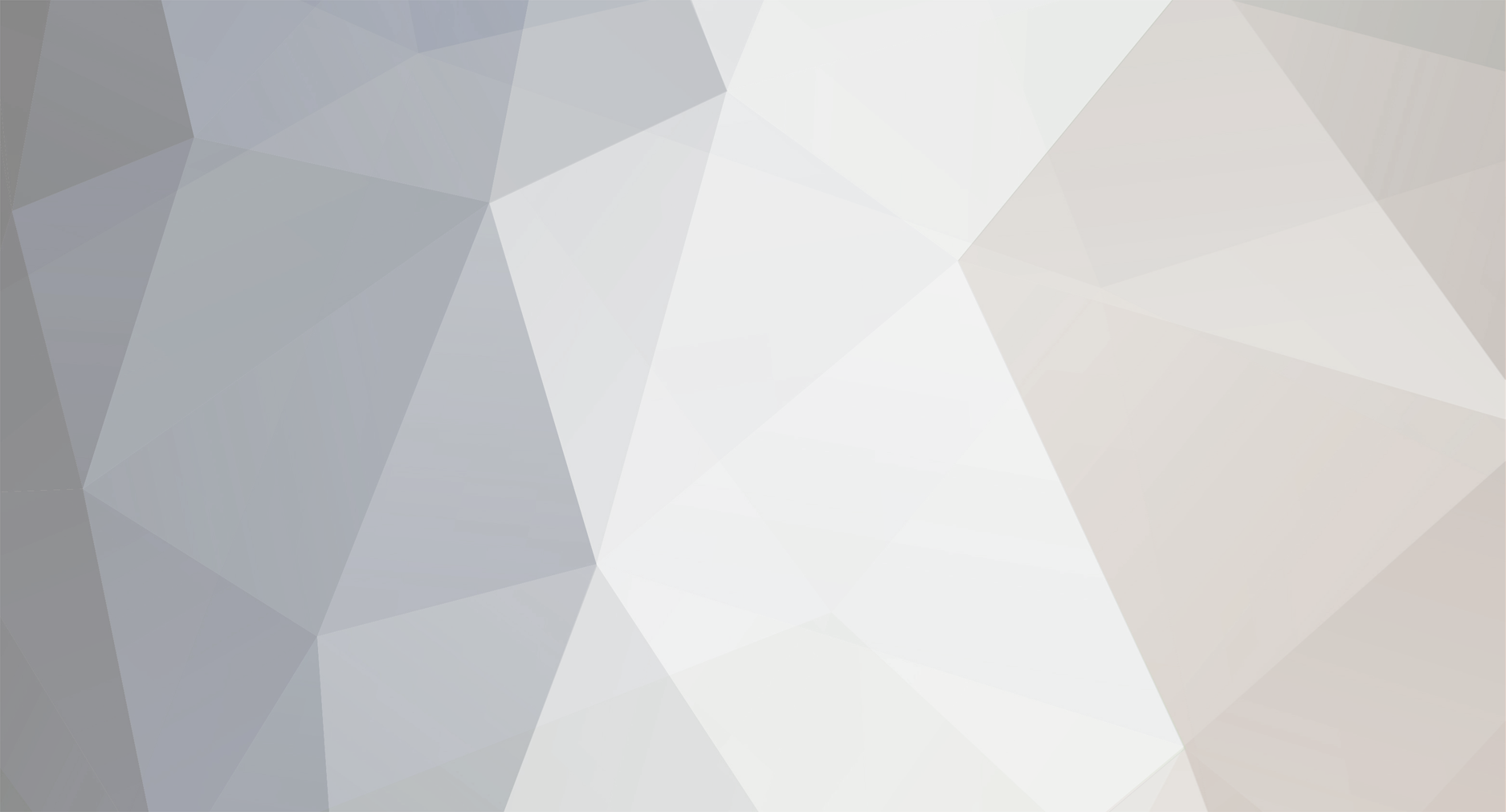
rwhite35
Members-
Posts
159 -
Joined
-
Last visited
Everything posted by rwhite35
-
Have you checked your error logs? If the script isn't behaving the way you expect, then error reporting would be the first place to start. Try locating your systems error logs at /var/log/apache/error.log or where ever httpd.conf has set that path. Alternatively, you can set error reporting locally by using the following: error_log ("custom reports here",3,"/var/www/vhosts/httpdocs/yourdomain/devlog.txt") //note path specific to your environment What this does is allow you to see how your script is behaving. devlog.txt is a text file that gets created and contains the reported text ( ie custom reports here). The '3' tell PHP to send error output to the text file (devlog.txt) instead of to system error reporting. Here is some of the best advice I have ever received, and I'll leave this thread at that: "learn how to search error logs and generate custom error reporting, it will save you years"
-
Taking another look at your code and comparing that to my response, the dataset I'm suggesting would look something like. From this multidim array you can foreach loop over each array, constructing you navigation without making additional trips to the database. In the outer loop [0]=>array('author'=>string...) the next author would be [1]=> array('author'=>"Jack Straw", 'id'=>102...). My answer doesn't address your current code, however, I suggest try a different approach altogether. $results ( [0] => array ( [author]=>"douglas adams" [id]=>101 [title]=> array ( [0]=>"Hitchhiker Guide to The Gallaxcy" [1]=>"Dirk Gently's Holistic Detective Agency" ) ) )
-
I would consider looking at your program logic. The functionality you want is mainly client side (even though the data is coming from a database query). Consider pulling all your author records on initial load. Then re develop your UI so when a web user clicks (or hover over) an authors name, it will pull the sub names from the DOM memory. That would be preferable then making another trip to the database, and will make the functionality you want easier to implement. Look into "separation of concerns" as a programming practice. That will help direct your development. Good luck.
-
you can try this too. echo date('Y-m-d H:i:s', $rows['last_post']);
-
How to Fire the Query on Click event on a link
rwhite35 replied to altafneva's topic in PHP Coding Help
Looking at your code, I think there might be a few other things to consider. Most important, what happens when you remove a movie from the list? The way you are "ID'ing" you movie isn't relevant to your movie list. Each movie should have its own unique id. Once you have done that, then creating links will be much simpler. And more flexible. Take another look at your program logic. But to answer your questions. If your movie has an ID, then you can simple create an anchor with that movies id. print '<a href="movie_watch.php?movie=$uniqueID" target="_blank"><img src="$movie_image_path"…>';- 6 replies
-
- sql
- dyanmic link
-
(and 3 more)
Tagged with:
-
Array to json is giving me trouble, help please...
rwhite35 replied to Rita_Ruah's topic in PHP Coding Help
I've had the most success with the following: <script src="http://ajax.googleapis.com/ajax/libs/jquery/1/jquery.js"></script> <script> //var data = <?php echo $array; ?>; var data = <?php echo htmlspecialchars(json_encode($array), ENT_NOQUOTES); ?>; $.ajax({ type: "POST", url: "process.php", data: data, success: function (msg) { console.log(msg); } }); </script> Where array is a PHP array, not encoded. BTW, you can turn on Firebug and see the POST data in the console log under the AJAX entry. -
problems parsing and dynamically writing to XML using DOM
rwhite35 replied to sunshineinabag's topic in PHP Coding Help
If you don't mean to assign $filesAttrs array (returned by getFileAttrs()) to another nested array outside your function, then remove the left/right square bracket. The current assignment is adding a new array to $testarr, which then containing the function result. Unless you call your function with different .xml files, I think you just want: $testarr = getFileAttrs(); -
php upload file not working inside a form
rwhite35 replied to mamaomphile's topic in PHP Coding Help
check the ownership and permissions of the form script or which ever script is doing the uploading. Depending on your setup, the web server (example apache) needs to own the script doing the uploading. From the command line try: chown apache:apache uploadscript.php -
If you are generating the file multiple times, you'll need a separate imagejpeg( ) for the image stream. Also, you would want the image stream for display on the last iteration of the image file. } else if($third_url1 === "products"){ $targ_w1 = $get_width; $targ_h1 = $get_height; $im1 = imagecreatetruecolor($targ_w1, $targ_h1) or die('Cannot Initialize new GD image stream'); // Background to Image $img_r1 = imagecreatefromjpeg($product_src1); //Creating Image $image1 = imagecopyresampled($im1,$img_r1,0,0,$_POST['x'],$_POST['y'],$targ_w1,$targ_h1,$_POST['w'],$_POST['h']); //here you created a file src3, no output here imagejpeg($im1, $product_src3); imagedestroy($im1); //unlink($product_src1); //unlink($product_src2); $targ_w2 = $get_width * 2; $targ_h2 = $get_height * 2; $im2 = imagecreatetruecolor($targ_w2, $targ_h2) or die('Cannot Initialize new GD image stream'); $img_r2 = imagecreatefromjpeg($product_src2); $image2 = imagecopyresampled($im2,$img_r2,0,0,$_POST['x'],$_POST['y'],$targ_w2,$targ_h2,$_POST['w'],$_POST['h']); //here you created a file with src4, no output here imagejpeg($im2, $product_src4); //now image stream to browser window imagejpeg($im2, NULL); imagedestroy($im2); } Try that. The issue has to do with headers and when they are being sent to the browser window. Your file is likely correct, its just not sending and image stream to the display.
-
Thanks, ";" was it. Here's the working code then. $res = array(); $query = "SELECT COUNT(*) FROM tbl_1;"; //requires the ";" to separate query statements. $query .= "SELECT COUNT(*) FROM tbl_2"; if($mysqli->multi_query($query)){ do { if ($result = $mysqli->store_result()) { while($row = $result->fetch_row()){ $res[] = $row[0]; } } else { echo "store result returned false."; } $result->free(); } while ($mysqli->more_results() && $mysqli->next_result()); } else { echo $mysqli->error; } $mysqli->close(); print_r($res);
-
Having a tough time getting multiple select count statements to return a result. Tried a few different way, but just looking to tally individual table rows. Any thoughts? $res = array(); //output array $query = "SELECT COUNT(*) AS count FROM tbl_1"; //assign count to an alias (column), but tried it without also $query .= "SELECT COUNT(*) AS count FROM tbl_2"; if($mysqli->multi_query($query)){ do { if ($result = $mysqli->store_result()) { $row = $result->fetch(); //tried this with fetch_row() and while loop $res[] = $row['count']; } else { echo "store result returned false."; } $result->free(); } while ($mysqli->more_results() && $mysqli->next_result()); } $mysqli->close(); print_r($res); Thanks in advance.
-
Thanks TRQ, That may be true, however strict enforcement may be the difference between 5.3 and 5.4. Same scripts in 5.3 work but didn't in 5.4. It should be documented because the answer was not readily available on the net. Regards,
-
Spent the day trying to figure out why GD library 2.0 imagejpeg($resource_id,NULL,$quality); wouldn't output my images to the browser after updating PHP from 5.3 to 5.4. Allow me to hopefully save you the trouble. If you've enabled GD library and using an external script to generate image files, NO OTHER OUTPUT from the external script is permitted, except the header('Content-Type: image/jpeg'); and the image stream. This goes for any sub include scripts (like a database connection). Error reporting for the executable script should be set to 0 with error_reporting(0); OR you should absolutely make sure none of the scripts are generating any errors or any other output. Here some code: initial_script.php <!DOCTYPE html> <html lang="en"> <head><title>First in stack</title> <meta charset="UTF-8" /> </head> <body> <img src="<?php print createimage.php?imgid=$img_id&uid=$user_id; ?>" alt="red dot" > </body> </html> Next create the image createimage.php <?php include dbconnection.php; //assume db connection and data pull returns image name $image = $row['img_name']; //image create resources etc.. $file="/path/to/".$image; $file_resid = imagecreatefromjpeg($file); // first send the header header('Content-Type: image/jpeg'); // display the image to the browser $quality=100; imagejpeg( $file_resid, NULL, $quality) or die("error displaying jpg"); imagedestroy($image_t); ?> In my case dbconnection.php was spawning a low level notice which was being sent before the header and image stream. This was causing the process to return a broken image icon. Also, imagejpeg now requires NULL if you are displaying the image to the browser. Before (5.3) this parameter could be " ". Firebug was throwing this error - Image corrupt or truncated:
-
PHP login and registration formulier with a html index
rwhite35 replied to yasminlove1's topic in PHP Coding Help
Yes, and sessions are the way you would do that. But you have to register each user who logs in with a session variable. And the way to do that is by user id. In your log in process, you will define a session var like this: session_start(); /* * data base query to get user id, user name, user password * once you have returned and defined a users "credentials", * you assign their credentials to a session variable */ $_SESSION['user_id'] = $user_id; $_SESSION['user_id']['user_name'].=$user_name; $_SESSION['user_id']['user_pass'].=$user_pass; // the session variable output would look something like Array ( [135] => Array // the outer array would be the users id ( [user_name] => Jane Doe // from the data base query [user_pass] => a45t67i912b332 // from the data base query ) ) In the head of each subsequent page, define local variables to present specific content to each user. session_start(); $array = $_SESSION['user_id']; foreach ($array as $key=>$value) { ${$key} = $value; //returns two local variables, $user_name and $user_pass } echo "Hello ".$user_name.", your stable is ready for your horse.<br>\n"; Hello Jane Doe, your stable is ready for your horse. -
PHP login and registration formulier with a html index
rwhite35 replied to yasminlove1's topic in PHP Coding Help
I don't see where loginv.php has registered anything with the session. You start the session, but do not interact with it. You could register the $_POST variables like this session_start(); if(!empty($_POST['gebruikersnaam']) AND !empty($_POST['password']) { $_SESSION['naam'] = $_POST['gebruikersnaam']; $_SESSION['pass'] = $_POST['password']; } Now any script with session_start() will have $_SESSION['naam'] and $_SESSION['pass'] available to that script. Not sure how you intend to use the session variable, but this is how you would register $_POST data to the session. I think that is what you asking. -
PHP login and registration formulier with a html index
rwhite35 replied to yasminlove1's topic in PHP Coding Help
try this. loginv.php: // replace $sql = ("SELECT gebruikersnaam, password FROM gebruikers WHERE gebruikersnaam='". $gebruikersnaam . "' AND password='" . $password . "'"); -- you are mixing string and literal quotes, nether is necessary $sql = ("SELECT gebruikersnaam, password FROM gebruikers WHERE gebruikersnaam=$gebruikersnaam AND password= $password); $controle = mysql_num_rows($uitvoer); //Uitvoer van inlog if($controle != 0) { //if $controle is >=1, nothing is happening here? Is that correct? //VERDERE VERWERKING. (DIT is aanjou, bijv. sessie ofziets? } else { //return to login with a urlencoded message $message = “Verkeerde gebruikersnaam of wachtwoord”; header('Location:login.php?mes='.$message); exit (); // Negatieve melding van ingevoerde gegevens. } -
Need help with multiple PDO queries (creating web statistic)
rwhite35 replied to tomailas's topic in PHP Coding Help
Couple things with your SQL // $sql = "SELECT SQL_CALC_FOUND_ROWS * FROM web_stats ORDER BY " . mysql_real_escape_string($order) . " LIMIT :numRows"; $sql = "SELECT * FROM web_stats ORDER BY vistedOn DESC LIMIT 1000000"; /* * Unless visitedOn and $numRows are dynamic, I wouldn't make them variables. Also, SQL_CALC_FOUND_ROWS gives you * the total rows the query would have returned if the total of db records were larger than 1000000(in your case). */ $st = $conn->prepare( $sql ); $st->execute(); Couple additional comments. PDO optimizes input for you, so if you're using the prepare, bindParam, execute machinery, also using mysql_real_escape_string isn't necessary. That part of the appeal to PDO. And this is nit pic I know, but inside the Class, what you refer to as a "function" is actually a Class Method. You can have a function inside a Method. But getWebStats is actually a Class Method. Any rate, try the edited query statement and see what errors or results you get from that. Good Luck. EDIT: I should ask, do you really mean to output 1000000 records? Because that's what you're asking the query to do. LIMIT the total records returned to N. -
Migration Complete - following these instructions Couple hick-ups, but nothing major. The main problem was that httpd.conf file re-write didn't comment out a line. I manually comment out line line 118 from: LoadModule php5_module modules/libphp5.so To: #LoadModule php5_module modules/libphp5.so When un-commented, this was causing an error on Apache restart - [warn] module php5_module is already loaded, skipping And preventing 5.4 from being the default PHP version. Changes Made to PHP.INI First, copied the php.ini-development to my default php.ini # from cmd-line (your path may be different) cd /opt/local/etc/php54/ sudo cp php.ini-development /opt/local/etc/php54/php.ini Edited the php.ini file to assign the default path for my installation of MySQL # line number directive = value 986 pdo_mysql.default_socket= /opt/local/var/run/mysql5/mysqld_socket 1135 mysql.default_socket = /opt/local/var/run/mysql5/mysqld.sock 1194 mysqli.default_socket = /opt/local/var/run/mysql5/mysqld.sock Set the correct Timezone and Long/Lat #Line number directive = value 919 date.timezone = "America/New_York" 922 date.default_latitude = 41.4994 925 date.default_longitude = 81.6956 Then saved and closed php.ini Restarted Apache2. Odd behavior, I had to restart Apache a couple before the changes actually showed up on the command line and php test page. But all seems to be working now. Now on to finding all the bugs in web apps with compatibility issues. There shouldn't be many, but I'm sure there a some.
-
Hello All, Plan to migrate PHP 5.3 to 5.4 this week using MacPorts on a MacOSX 10.6 (Lion) server. Plan to go through this gist as my reference. Who has made this migration already, using MacPorts (version 2.1.3) and were there any "gotcha" I need to know about in advance? Also, I'm using a number of PEAR libraries, I don't suppose PEAR is bundled with the PHP54 port? Any thoughts or advice is appreciated. Thanks in advance.
-
mysqli prepare fails with generic error: mysql: 5.5.27
rwhite35 replied to JA12's topic in PHP Coding Help
Take a look at my code block. I believe it does what you want it to do. I simple pass the table name and array of input values, the method constructs the query and executes the query against the table. For the most part, the code is complete. You would have to write your own getdbcols method which would query the db for the column names. -
mysqli prepare fails with generic error: mysql: 5.5.27
rwhite35 replied to JA12's topic in PHP Coding Help
I second that last statement. -
mysqli prepare fails with generic error: mysql: 5.5.27
rwhite35 replied to JA12's topic in PHP Coding Help
Hmm, I'm still trying to understand what you ultimately want to do. If the user can input variable data, then you will have to match up the inputs values with the appropriate columns. That could be tricky, but not impossible. If you just want to dynamically build query string, you'll want to do this in a multi-step process. Starting with getting the table columns names, then syncing those with the data input. The code below is a method in a much larger class, but it builds dynamic query string. Also, it implements PDO and not MySQLi, but you'll get an idea of the sub routines that went in to generating the query when all that's know if the table name. /* * CLASS METHODS ADDRECORD * Add a record to the target db table, We only need to know the table name * sub routine 1 constructs the insert statement by calling getdbcols method * sub routine 2 binds the post array value to the alias(?) positions. * note - each position MUST have a value, even if the value is null (ie string_id) */ private function addnew($array,$tbl,$_conn){ // MAKE PDO DB CONNECTION try{ self::$_conn = new PDO('mysql:host='.DB_HOST.';dbname='.DB_NAME.'', DB_UNAME, DB_UPWORD); } catch (PDOException $e){ print "Error: ".$e->getMessage()."<br>"; die(); } //SUB ROUTINE 1 $tblarr = $this->getdbcols($tbl); //returns array of db table column names $query = "INSERT INTO $tbl SET "; //generate a query string foreach ($tblarr as $key){ //iterate over the cols names array $query .= ($key."=?, "); //using ? placeholder for bindParam } $query = rtrim($query,", "); //remove EOL whitespace and comma //Prepare the statement, self refers to the sub class itself. $stmt = self::$_conn->prepare($query); //SUB ROUTINE 2 :construct the bindParam statement $arcount=count($array); //max loop count foreach($array as $key=>$value){ $stmtarr[]=$value; //convert assoc to numerated array } //re index array so array count, placeholder count and incrementor will sync up $stmtarr = array_combine(range(1, count($stmtarr)), array_values($stmtarr)); for($i=1;$i<=$arcount;$i++){ //bind values to placeholder, one for each array element $stmt->bindParam($i,$stmtarr[$i]); } //Execute the statement $stmt->execute(); if($stmt==true){ return $stmt; //returns true on success } else { echo "There was an error in the query."; } self::$_conn->close(); } Also, this isn't the complete code, so you won't be able to cup/paste this into you own source. But hopefully it give you some idea on how to proceed with your code. -
mysqli prepare fails with generic error: mysql: 5.5.27
rwhite35 replied to JA12's topic in PHP Coding Help
Crongrats on migrating to a better db process! I think you have a few issues here, but the one critical to your questions is here: $bind = new BindParam; You're trying to mix PDO with MySQLi and its not going to work. Additionally, you're using MySQLi procedural style (which is fine) however, PDO is ONLY object oriented. The binding method you want to use for MySQLi procedural would be something like: $stmt = mysqli_prepare($link, "INSERT INTO tb_test VALUES (?, ?, ?)"); mysqli_stmt_bind_param($stmt, 'ssi', $value1, $value2, $int); Good luck, hope that helps move you in the right direction. -
Both great suggestions, keep them coming. This has been going on for a week or so now and efforts to distract myself don't seem to be getting me back on track. I think this is typical of any creative process, be it development, song writing or painting. You get to this place where the "technician" seems to be doing the same sorts of things over and over again. Maybe a better questions would have been how do you keep development fresh and new, when in reality the algorithms, routines, processes and methodologies are pretty much the same.
- 5 replies
-
- programming
- applications
-
(and 1 more)
Tagged with: