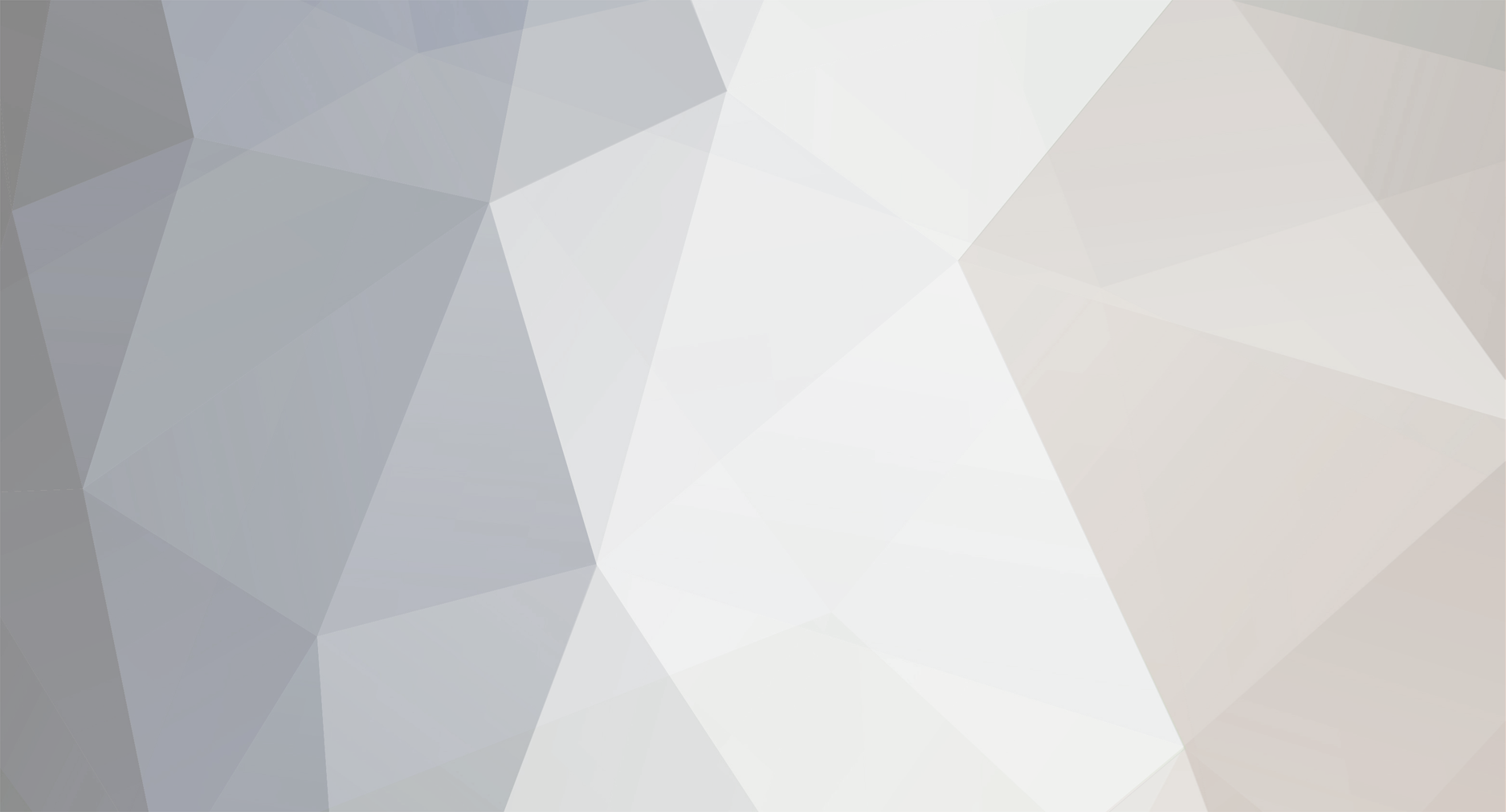
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
generate random number with variable probability
btherl replied to grilldor's topic in PHP Coding Help
You can generate the second decimal place seperately, then add them together. Alternatively you could express the probability as a function of the value being generated, and generate your array according to that function. But generating the second place seperately seems simpler :) -
Firstly, you need to tell PHP when you want to enter code, and when you want to enter HTML. For example: [code]<select name='state'> <option value='<? echo $stateArr['stateCode']; ?>'> <? echo $stateArr['stateCode']; ?> </option> </select>[/code] The '<?' says "enter php mode". The "?>" says "Finish with php mode, go back to HTML". The other style you can use is like this: [code]print "<select name='$state'>"; print "<option value='{$stateArr['stateCode']}'>{$stateArr['stateCode']}</option>"; print "</select>";[/code] In this case, you never leave PHP mode. Instead, you print out the html using commands within php itself. In both cases, the select tags must go outside the loop, as they only appear once. The option tags must be inside the loop, as they appear once for each state. Hope that helps.. feel free to ask more questions :)
-
How about [code]echo htmlentities($face, ENT_QUOTES, 'UTF-8');[/code] Or, replace 'UTF-8' with one of: BIG5 GB2312 BIG5-HKSCS
-
If you want to generate javascript using php, then yes you can. Just set your script tag to point to the php script, and have your php script output javascript. But there is no way to "include" a php file from plain javascript. Like Toon said, you can use ajax to call a php script from javascript and get the results.
-
I forgot the most obvious thing to check.. "fwrite() returns the number of bytes written, or FALSE on error." fread() "Returns the read string or FALSE in case of error." Once we're sure the calls are succeeding, then it's time to look at whether or not the packets are getting through :)
-
Another possibility is that the udp packets are being blocked by a firewall. UDP has no setup stage, so the connection will "succeed" even if there is no path for the packets to go through. Are you able to use TCP instead?
-
I think I can offer an explanation.. First, you set $sched[$team] to a scalar value (a string). Then you try to access it as an array. PHP handles that by converting your array index (such as 'week1') into an integer, and treats it as a string offset index. So: [code]$sched[$team]['week1'] = 3; # is interpreted as.. $sched[$team]{0} = 3;[/code] Hence, the first character is changed to the week3 value, since that's the last assignment you make to the first character.
-
Constraints are not always good. If your lecturers said they were ALWAYS good, they were wrong :) I would say that if you can't think of any reason to use a constraint, then you shouldn't be using one. If you can think of a reason to use one, then you should be using one. Usually you set a constraint as an aid to debugging. The assumption is that your code is not perfect, and constraints (including types, lengths, and other more complex constraints) will help you pick up bugs before they grow into horrible monsters that eat you alive. There are actually many hidden constraints, such as the character set of the database, which you are already using. And that constraint is useful if the database character set matches the display character set. Calling a column a numeric rather than a varchar is also a constraint, limiting the column to holding numeric values only. It makes a lot of sense if you want to store dollar+cent values. It ensures that you don't accidentally store something else there. But if you have no reason to constrain a value, then don't constrain it. Have you seen how much trouble airlines have because the ticket name field can't fit long names? They have to kludge around it. But in that case, it does make some kind of sense, because the name MUST be short enough to fit on the ticket. On some databases, varchar length constraints may have helped efficiency. But on postgresql, it doesn't help. Varchars with lengths are stored identically to unbounded varchars.
-
HELP!!! My php script is not inserting variable values into my string???
btherl replied to khopson's topic in PHP Coding Help
I hope you mean the <?php tag, not the <?>php tag :) I think it's very unlikely that the problem is with the interpreter. That output there looks like what might happen if you accidentally left php mode and returned to html mode, with a '?>' for example. Did you include the '?>' from ken's example? If so, take it out and try again. -
(SOLVED)Why wont this work ,help please
btherl replied to just me and php's topic in PHP Coding Help
Does it say "Deleted X", where X is a $del_id? If not, there are 3 ways it could fail. Either the query failed, $_POST['checkbox'] is empty or $_POST['delete'] is not true. Try this: [code]var_dump($_POST['delete']); var_dump($_POST['checkbox']);[/code] just before the if ($_POST['delete']); Also, add an "else" case to the check for query failure: [code]... } else { echo "Query $sql failed! Error was " . mysql_error(); }[/code] -
HELP!!! My php script is not inserting variable values into my string???
btherl replied to khopson's topic in PHP Coding Help
I copied and pasted your php, and it's fine.. the problem must be elsewhere. Though there is a small chance that something is odd with your php interpreter. You could make a simple form to test with: [code]<form action="http://localhost:8500/Services/getWeekNum.php" method=post> <input type=text name=year value=1066> <input type=text name=month value=1> <input type=text name=day value=1> <input type=submit name=submit> </form>[/code] -
HELP!!! My php script is not inserting variable values into my string???
btherl replied to khopson's topic in PHP Coding Help
I'm puzzled by this too.. Can you post your entire script inside code tags? [code]This is inside code tags.[/code] Click the # button above where you enter the post to insert code tags. -
HELP!!! My php script is not inserting variable values into my string???
btherl replied to khopson's topic in PHP Coding Help
Are you sure you are writing $week_str= "&weeknum=10&year=$yr&month=$month&day=$day&"; And not $week_str= '&weeknum=10&year=$yr&month=$month&day=$day&'; Notice the different quotes. Double quotes allow you to add variables in the string. Single quotes treat the string literally, and do not allow variables. -
respiritu, please move your question to another topic. Being polite wouldn't hurt either. t3od0r, the lines looking like this generate the links: [code]echo "<a href='".$filename."?from=".($i*$display_nr-$display_nr)."&".$vars."'>".$i."</a> ";[/code] The function uses "$vars" to add any additional variables, such as the category id you want to be included. Somehow you will need to ensure that $vars, when given as an argument to make_next_previous_with_number(), is set to "cat_id=x". If that makes no sense at all, post again and I'll explain further :)
-
php replacing my & with & (how do i stop this?)
btherl replied to john_6767's topic in PHP Coding Help
Where do you see it being replaced with & ? I suspect that it occurs somewhere else, not in that piece of code you have pasted. -
"undefined offset" means you are trying to access an array element that doesn't exist. It's likely that your latitude and longitude don't have the same number of elements. To check, use count() on both of them and see if the numbers match up. Are you intending to fetch several rows of data from your sql queries, or just a single row with many columns? mysql_fetch_array() will only fetch a single row. That may also be causing problems.
-
The short answer is.. your web host has configured the website so that what you are trying to do is impossible. You can either ask them to change the configuration (not likely), or you can move those images you want to display under the document root. For example, put them in /home/localfind/site/Clients , instead of /home/localfind/Clients You will need to be sure that sensitive files aren't under the Clients directory as well.. you may need to store some files in seperate locations, or add access controls to particular paths. Then your html will be [code]"<img src='/Clients/{$business[$x]}/images/{$thumb[$x]}'>"[/code] The {} syntax allows you to put variables inline into a string, even if they are things like array elements.
-
Cannot display a link or image with absolute url in autoresponder
btherl replied to pan1138's topic in PHP Coding Help
Does "hangs up on the HTML" means it doesn't send the email and doesn't display any errors? If so, you probably have another syntax error in there. Are you able to use a shell to check the syntax of your script? If not, can you set the display_errors configuration variable to true? Then any syntax errors will be shown in the browser. If there's no syntax errors, then add print statements throughout your script to see how far it gets before dying. -
The illegal character there is the single quote: ' Imagine you do this: [code]$string = "'); DROP TABLE tab; SELECT ('"; $sql = "INSERT INTO tab (col) VALUES ('$string')";[code] Then your sql statement will be this: [code]INSERT INTO tab (col) VALUES (''); DROP TABLE tab;SELECT ('')[/code] The key to this is that the single quote within the string lets you get out of the string.. after that, you will usually get a syntax error in your SQL. But someone crafty can start running his own sql commands. If you escape the string first, then the quotes will be escaped, so you get this: [code]INSERT INTO tab (col) VALUES ('\'); DROP TABLE tab;SELECT (\'')[/code] which will insert the string properly. The \' is treated as a normal character, instead of ending the string.[/code][/code]
-
Cannot display a link or image with absolute url in autoresponder
btherl replied to pan1138's topic in PHP Coding Help
I checked the syntax, and I think you need to change to this: [code]$body = <<<EOF <html><body><br>Thank you for your submission!<br> <br>Click on the image below for your confirmation!<br> <br><a href="http://www.yoursite.com/thankyou.html"><img src="http://www.yoursite.com/thanks.jpg" alt="Thanks!" width="244" height="165"></a><br></body> </html> EOF;[/code] The final EOF must be at the start of a line, and the beginning EOF syntax is not exactly like some other languages (perl I think allows more flexibility with the starting marker) Btw, knowing that the email was never sent and that the meta refresh doesn't display is why I knew to look at the syntax first.. otherwise I would have no idea where to start debugging. Which is why I asked all those questions :) -
Cannot display a link or image with absolute url in autoresponder
btherl replied to pan1138's topic in PHP Coding Help
Yes, that's helpful :) Just a few more questions - how doesn't it work? Does the image appear, but nothing happens when you click on it? Or is there no image appearing? And in which email program does it not work? The program you are testing with is relevant to diagnosing the problem. Or does the email simply not get sent? Or not arrive? Spam filters can sometimes cause non-arrival even when an email is being sent. Your html looks ok.. an img inside an "a href" should make a clickable image. -
When inserting your data, use mysql_real_escape_string() on the url first. Otherwise the quotes will alter your SQL statement. This is also a security issue, and allows someone to run arbitrary commands on your database, if they know you are crawling this site. eg [code]$escaped_url = mysql_real_escape_string($url); $sql = "INSERT INTO urls (url) VALUES '$escaped_url'"; $result = mysql_query($sql) or die("Query failed: $sql\n with error " . mysql_error());[/code]
-
You need to expicility add any variable you want to access after the form submit to the form itself. In this case, I think you need: [code]<form> ... <input type=hidden name=itemId value="<? echo $itemId ?>"> .. </form>[/code] Then when that form is submitted, $_POST['itemId'] will be available. The point here is that variable available the first time your script is run (in response to a GET request), are NOT available the second time, unless you explicitly make them available. You could also use sessions to store the values you want to keep between script requests.
-
Cannot display a link or image with absolute url in autoresponder
btherl replied to pan1138's topic in PHP Coding Help
What are you expecting to happen, and what is actually happening? Also, which program are you viewing the email in? -
Since you're using supplierid as the identifier, it'll make life easier if you keep that value in a variable and use it for your database queries. I would suggest making a function which first tries to update the supplierimage. If that update affects 0 rows, then do an insert. That means you need only two queries. [code]UPDATE supplierimage SET image1='$image' WHERE supplierid = $supplierid -- Or, you can do UPDATE supplierimage SET image1 = '$image' WHERE supplierid = (SELECT supplierid FROM suppliers WHERE username = '$username')[/code] Notice how the queries are simpler if you know the supplierid already. Then, if mysql_affected_rows($result) == 0 for the update, do this: [code]INSERT INTO supplierimage (supplierid, image1) VALUES ($supplierid, '$image')[/code] Or if you only have username available, replace $supplierid with the subquery above: [code]INSERT INTO supplierimage (supplierid, image1) VALUES ((SELECT supplierid FROM suppliers WHERE username = '$username'), '$image')[/code] To fetch data [code]SELECT * FROM suppliers JOIN supplierimage USING (supplierid)[/code]