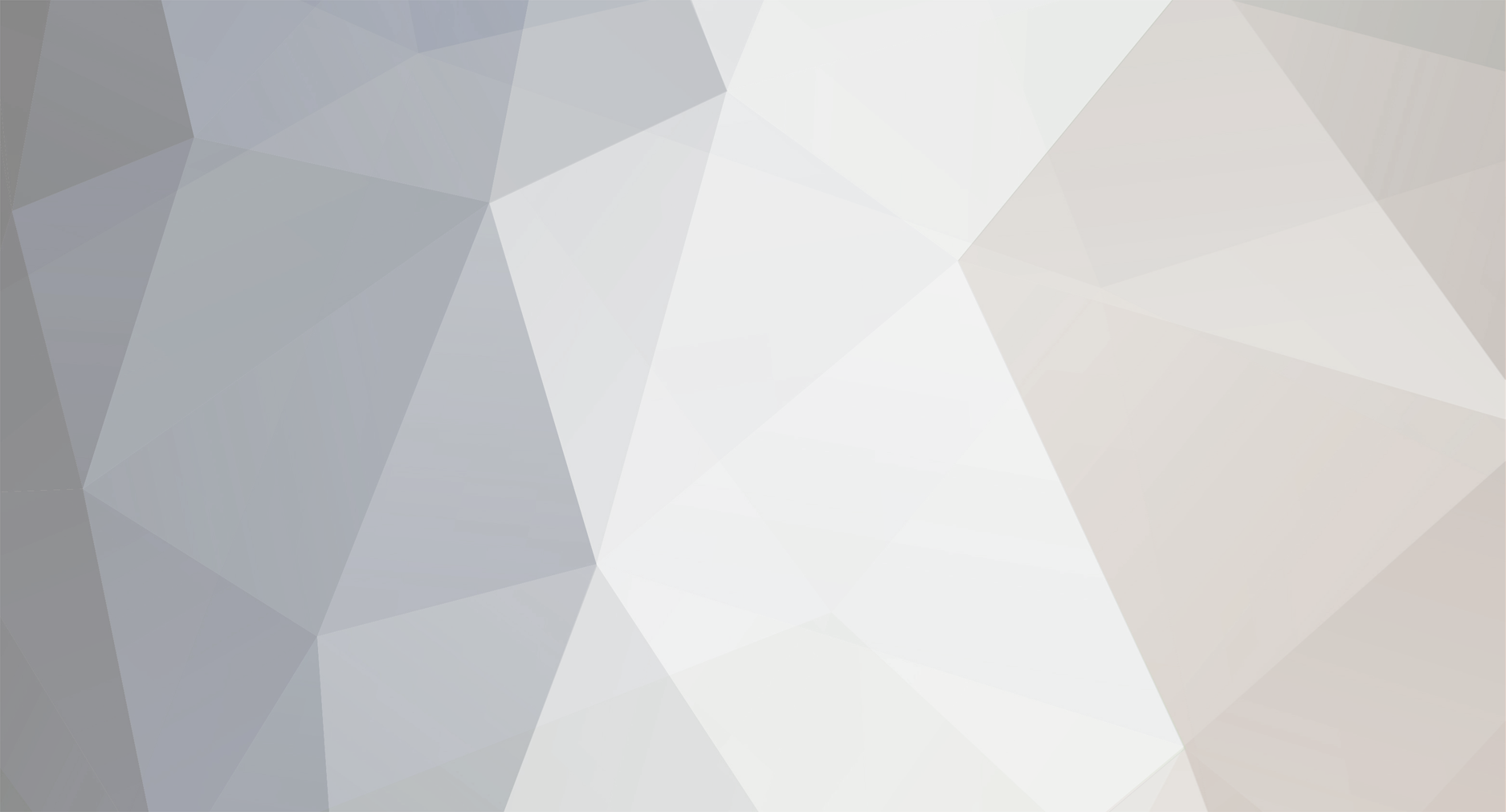
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
[SOLVED] Personal Programmer Assistant 0.2
roopurt18 replied to Ninjakreborn's topic in Beta Test Your Stuff!
I suggest you pick up a book on UML and download some UML software; StarUML is a good, free application that can help you with the design process. -
I ran into a minor problem while writing a function that does just that. The function is called [i]ajaxPrepareQS[/i] and takes as its parameter a list of form elements to build the query string out of. My Javascript knowledge and experience is limited, so I'm open to critique on my code, but here it is: [code] // ajaxPrepareQS // fields - array of form fields to encode for the AJAX request // RETURN: the data string to pass to the server function ajaxPrepareQS(fields){ var escapeFunc = escape; // Set a function pointer in case we change // later var passData = ''; for(x in fields){ if(fields[x].type == 'button' || fields[x].type == undefined){ continue; } var val = null; switch(fields[x].type){ case 'text': case 'textarea': case 'password': case 'hidden': val = fields[x].value; break; case 'checkbox': case 'radio': if(fields[x].checked){ val = fields[x].value; } break; case 'select-one': val = fields[x].options[fields[x].selectedIndex].value; break; case 'select-multiple': // This is a special case where we must set the passData // up here for each selected item in the list for(i in fields[x].options){ if(fields[x].options[i].selected){ if(passData.length != 0){ passData += '&'; } passData += fields[x].name + '=' + escapeFunc(fields[x].options[i].value); } } break; } // append the value to the full query string if(val != null){ if(passData.length != 0){ passData += '&'; } passData += fields[x].name + '=' + escapeFunc(val); } } return passData; } [/code] I used the following code to call it on all elements of my form: [code] var e = document.optbid.elements; var fields = new Array; var i = 0; for(x in e){ fields[i++] = e[x]; } var qs = ajaxPrepareQS(fields); [/code] although I guess this would work just as well: [code] var qs = ajaxPrepareQS(document.form_name.elements); [/code] When I make my AJAX calls, if the method is GET I append the returned value from [i]ajaxPrepareQS[/i] to the URL with a '?' in between them. When I use POST I send the returned value in my xmlHttp.send(). Hope this helps.
-
I was offered a 1 year free subscription, I was debating on whether or not I should bother accepting it. If someone had said, "Oh I read it and it's great yadda yadda" I probably would.
-
Just wondering if anyone reads this magazine and what your thoughts about it are.
-
Can you provide an example of doing that? Here's my actual query (with the fields removed for clarity): [code] SELECT ... FROM DocTracking t LEFT JOIN wv_user u ON (t.User=u.login) LEFT JOIN wssubc s ON (u.subname=s.subname) LEFT JOIN webview_docs d ON (t.DocID=d.doc_wvid) LEFT JOIN POOptions p ON (t.DocID=p.PONum) WHERE t.Module=2 LIMIT 0,25 [/code]
-
Make sure you're supplying the correct URL to src in the link tag.
-
Here's the scenario. There are three tables: UpDocs, POs, & DocTracking UpDocs stores information about uploaded documents. POs stores information about imported documents from another software system. DocTracking records visits by users to these pages. Within DocTracking there is a DocID (INT) field that references UpDocs.ID (INT) or POs.PONum (VARCHAR). There is a query within the site that is being dragged down by: LEFT JOIN POs p ON (DocTracking.DocID=p.PONum) Basically a VARCHAR field is being compared to an INT field. There is an index within POs on the PONum VARCHAR field. Is there any way I can force the query to use this index? I know I can use FORCE INDEX ([i]idx_name[/i]), however that doesn't appear to work due to the type differences in the fields. I can circumvent this problem by adding an INT column to POs, call it iPONum (INT), and creating an index on it. However I'd rather not add an extra column to the table if I can avoid it.
-
I'm not sure this is the right board, but it seems most appropriate. I know that flash and javascript can interact with each other. My question is, given a flash file that I'm not the author of and know nothing about, can I still enable the two to work together somehow? Basically I'm interested in displaying a flash file, having it run, and at the end of it pulling certain bits of data out of it. If anyone can point me in the right direction I'd be much obliged.
-
Store uploaded file as blob or in folder?
roopurt18 replied to Accipiter's topic in Application Design
You could store them in sub-folders based on the user that uploaded the file or a sub-folder indicating which filters will apply in displaying the image. For instance, if someone is displaying an image attached to Phase Z of Project Y within the "Plans" portion of your site, you could store them in: data/Plans/Images/Y/Z/<db_id>.<random_short_key>.<extension> Using the db_id as part, or all, of the filename will help you later if you want to write a clean up script. It makes it easier to check that every file on the disk is referenced in the DB and that every entry in the DB is on the disk. Additionally, you should store the [i]original[/i] filename, maybe even the filesize, in the DB. This way you can serve it later and have it appear to have the same name, thus hiding your renaming scheme (security, security, security!) and you can also check that it's likely the same file later. -
One of our clients is receiving an intermittent error message while trying to access our site, but apparently they're still able to access other websites while receiving this message. While on the phone with them while they were experiencing the problem, I had them ping -t www.yahoo.com and all requests timed out, which usually point at an ISP or network issue. I then tried to ping one of their machines with my own and received a bunch of "host unreachable" messages. In addition, I'm in and out of the site on a constant basis and it's always worked for me, so it's definitely not host maintenance. Below is the content portion of the image from IE. I've left off the address, menus, etc. but I did verify that the address is correct. In addition, the IE icon looks like it might be IE7 (unsure because I do not use it) or a custom IE provided by the ISP. I told them to contact their ISP and ask if it was having difficulties. Other than that, anyone have any ideas? I'm still rather new to the whole internet / networking side of things and it could very well be something I'm not familiar with. [img]http://www.ibswebview.com/test/error.jpg[/img]
-
If you only want it to be an alphanumeric field, use a regexp and preg_match to force the user to only enter that type of data. [code]<?php if(!preg_match('/^[a-zA-Z0-9\s]*$/', $_POST['Comments'])){ echo "Invalid comments!"; DisplayForm(); } ?>[/code] I went from memory so the parameters to preg_match might be in the wrong order.
-
Record the size of the files uploaded by users and prevent them from uploading when they've reached their quota?
-
Use a separate class or namespace for interacting with the database. For instance, let's say you are designing some type of catalog. I would call the catalog class CCatalog. CCatalog would be the access point into all dealings with catalogs for the rest of your site. It would provide the methods to create, copy, move, modify, etc. a catalog. There is also a good chance that catalogs would need to interact with the database. For this I could create a separate class, or at least a namespace, called DAOCatalog or DBCatalog. This namespace / class would be a collection of functions that interact with the database. For instance, you might have a function DBCatalog::GetExistingCatalogs() that queries the database and the actual call to this function would be inside the CCatalog class. Whenever I create a site, I consider the site itself to be like a top level object. It deals with all sorts of classes CPage, CTemplate, etc. to create the content. Those classes refer to other classes CUser, CAccount, CCatalog, etc. to work with and modify the content. All of the database interactions are buried under another layer within data access objects (DAO). Basically, whatever you do, I recommend separating the database interactions underneath another layer.
-
In the PHP manual, look up the following: fopen fwrite file_get_contents implode explode More importantly, why would you not want to store this in a database?
-
I think you need to use grouping: [code]<?php $patterns = "/(\\msn.com)|(\\hotmail.com(.uk)?)/"; if (preg_match($patterns, $string)) {echo "yes";} ?>[/code]
-
NM, it's irrelevant at this point.
-
I'm still new to javascript so I may be missing something very simple, but here goes. I have a particular element that when I pull the innerHTML property in FF it shows normally. However, when I pull the innerHTML in IE6, all of the HTML tags are converted to uppercase and most of my double quotes are stripped off of attribute values. Can someone offer up an explanation for this behavior, other than IE sucks? :^]
-
Using a PHP script to do a MS Access to MySQL data move....
roopurt18 replied to pthurmond's topic in PHP Coding Help
I know you can connect to the Access DB through PHP, but I'm unsure of how exactly how that is done, so I have little advice to offer in that department. For the MySQL portion of it, I would create three .sql files. The first would be to establish and create your destination DB; all of it's tables and fields. The second would be to create a MySQL mirror of the Access DB with all of the tables prefixed by msa_. The third would be to INSERT ... SELECT from the msa_ tables to the final tables. The reason I recommend this approach is because you do not plan to need all of the data now, but that may change in the future. It is much easier to insert data across MySQL tables than it is from access to MySQL. So if it turns out you do need additional data later, you'll have easier access to it then and you'll only have to deal with the Access -> MySQL nightmare once. The other reason I recommend this approach is because of a similar situation where I work. We have a server software product that uses dbase tables and must continuously import data into a MySQL driven web application. The original developers of the web app, who are no longer with the company, designed many of the MySQL tables to be mirror images of the dbase tables. The problem is dbase != MySQL and those tables are [i]not[/i] set up to take advantage of some of the handy features MySQL has to offer. I would have much rather preferred an approach where the dbase data is imported into mirror MySQL tables and then from those tables imported into the "real" MySQL application tables so as to minimize data repitition. After enough time elapses that you are sure you no longer need the msa_ tables, you can just drop them or export them and save them as backups. -
adding more than one piece of data to a database
roopurt18 replied to jwk811's topic in PHP Coding Help
You're going to need to be more specific if you expect any help. -
Refresh automaticlly when new results come in.
roopurt18 replied to Muggin's topic in PHP Coding Help
Your solution would be AJAX with a javascript timer checking periodically for new entries in the DB. -
Help with php/mysql - If a value exists, do something.
roopurt18 replied to papaface's topic in PHP Coding Help
If you have MySQL 4.1+ you can use INSERT ... UPDATE http://dev.mysql.com/doc/refman/4.1/en/insert-on-duplicate.html -
Your regular expression will match only a single character from a-z as it is written. [b]Match any number of lower case alpha chars, including none:[/b] "/^[a-z]*$/" [b]Match any number of lower case alpha chars, at least one:[/b] "/^[a-z]+$/" [b]Match at most [i]n[/i] lower case alpha chars, including none:[/b] "/^[a-z]{[i]n[/i]}$/" [b]Match between [i]m[/i] and [i]n[/i] lower case alpha chars:[/b] "/^[a-z]{[i]m[/i],[i]n[/i]}$/" If you want to learn more: http://www.regular-expressions.info/tutorial.html
-
Either you use 2 queries for 2 result sets or you use 1 query with conditional statements in your code to display different information for each row. [nevermind - I misread something]
-
It's all a matter of preference, but I will say, if I remember correctly, that IE will choke on that empty line between your ?> and <!DOCTYPE