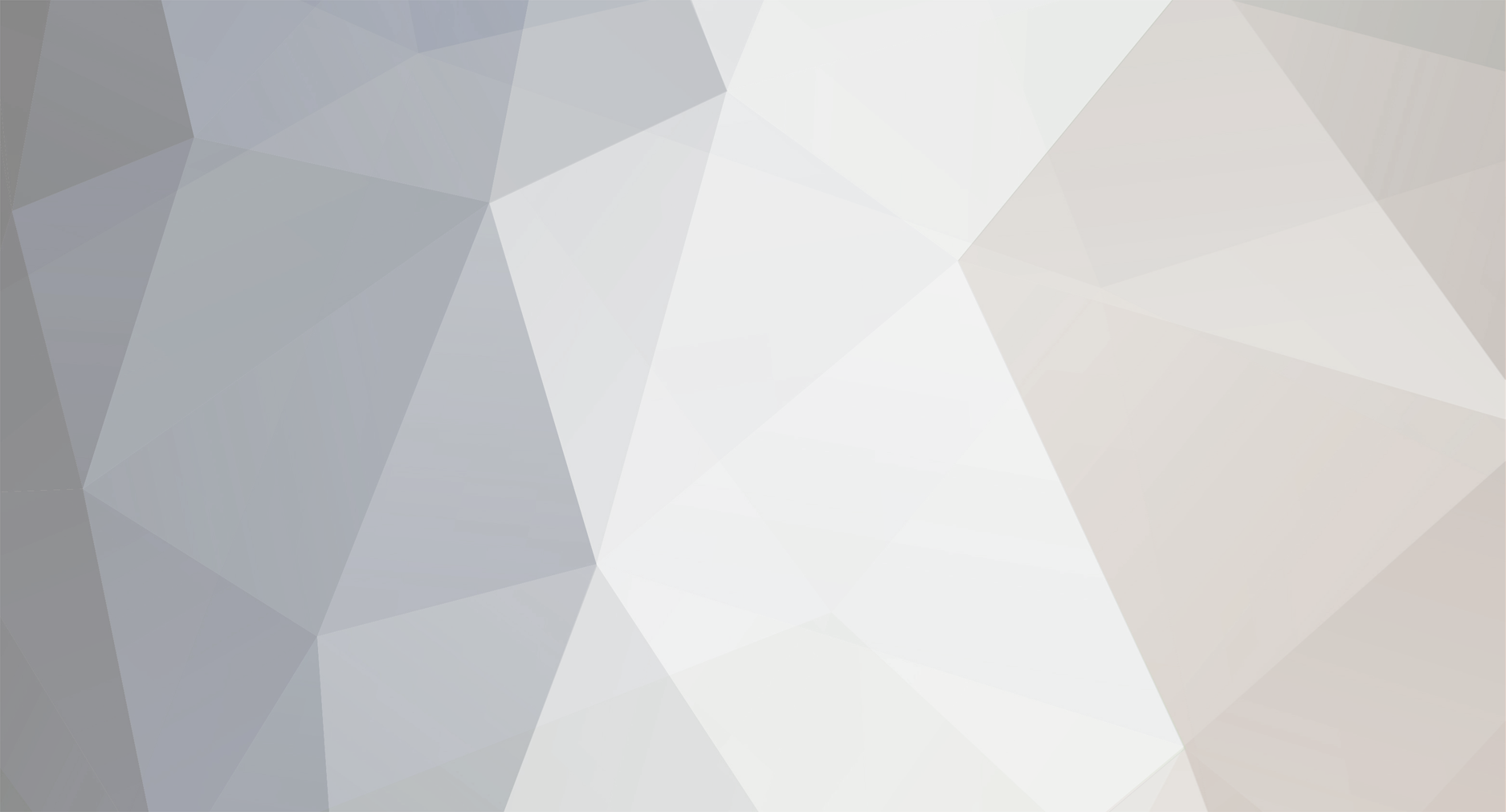
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
Problem reading array's, validation's not working.
roopurt18 replied to Ninjakreborn's topic in PHP Coding Help
Here is the relevant piece of information: [code] Array ( [classification] => Array ( [doorwarningwhichone] => [dooropeningwhichone] => [flightinstrumentwhich] => [engineinstrumentwhich] => [othertext] => ) ) [/code] Now do the same exact thing, but this time check one of the boxes associated with one of the following values: doorwarningwhichone, dooropeningwhichone, flightinstrumentwhich, engineinstrumentwhich, othertext I can almost garauntee your form validation will fail and tell you that you must check at least one box for the classification. If you didn't check any boxes, none of those keys should exist inside [i]classification[/i]. They do for some reason and I'm not gonna pick apart your code to find out why. But it just doesn't seem right to me. -
You could also look into using mod_rewrite.
-
MySQL timestamp not working with PHP strtotime :(
roopurt18 replied to junglyboi's topic in PHP Coding Help
I find that it's much easier to let the database handle this type of thing for you: [code]<?php $sql = "SELECT *, " . "DATE_FORMAT(promoter_mod, '%Y-%m-%d\t%T') AS promoter_mod_disp " . "FROM promoters ORDER BY promoter_id"; $result = mysql_query($sql); ?>[/code] -
[code] echo ('<form action="http://www.iwannabeaculjan.com/test/test2.php" method="post">'); echo ('Submitted By:<input type="text" name="submitted" size="30"><br>'); echo ('Comment:<textarea name="comment" rows=3 cols=30></textarea><br>'); echo ('<input type="submit" value="Add Comment">'); echo ('</form>');[/code] You don't need to enclose the arguments to echo in parens. This will work just fine: echo "hello, world"; [code]$submitted=$_POST['submitted']; $comment=$_POST['comment'];[/code] You probably want to clean this data before submitting it: $submitted = "'" . addslashes($_POST['submitted']) . "'"; $comment = "'" . addslashes($_POST['comment']) . "'"; [code]$time=time;[/code] It might be optional, but I'd include the parens here: $time = "'" . addslashes(time()) . "'"; [code]if (isset($comment)) { $sql="INSERT INTO piccomments (picid, comment, when, who) VALUES ('$picid', '$comment', '$time', '$submitted')"; $result=mysql_query($sql); } else { exit();[/code] Where did you set $picid? Further, picid is probably an INT column, thus single parens are not necessary. I included the single parents for the other column values in the preceding lines, so you can drop them from the SQL statement. Where is the closing curly brace for your if statement?
-
basename() extracts the file name from a string containing a directory path and a file name. 0777 is the permissions for the directory you are creating. Go to google and search for: man chmod for a better desription on file permissions.
-
User management accounts - private page per user....?
roopurt18 replied to Skeleten Neteleks's topic in PHP Coding Help
Your site is recognizing users by what's in the $_SESSION array. [b]logout.php[/b] [code]<?php // logout.php // log a user out session_start(); session_destroy(); session_unset(); $_SESSION = Array(); header("Location: index.php"); exit(); ?>[/code] -
[i]But how can i upload the file to a folder named by $_SESSION['Username'][/i] Append $_SESSION['Username'] to $file_dir [i]if that folder doesnt exist, would it auto-create the folder?[/i] Not sure if it would. But you should check if the folder exists yourself with file_exists() and is_dir(). If it doesn't exist, you can create it yourself with mkdir(). [i]when the file is uploaded successfully, i should also add entries to a sql table right?[/i] Probably, it makes life easier in the long run to have a record of what was uploaded, by who, and when. [i]to store the filename,link etc etc. this would be easier to loop through the usernames folder and display all their images right?[/i] Not necessarily. It's just about as easy to open a directory and grab a list of it's contents as it is to write a MySQL query. But having a matching table is almost always a good idea.
-
Well here goes... No one is going to write your code for you. This forum is intended to help people who are stuck on their own code and seeking help. Why don't you give it some effort on your own and post where you get stuck. Otherwise this belongs in the freelancing forum.
-
Even better!
-
[code]<?php $Colors = Array( 'cccccc', 'cdcdcd' ); $Count = 0; while( $Count < 100 ){ echo $Colors[$Count++ % 2]; // Alternates colors } ?>[/code]
-
I wouldnt do it that way though, generating variables like that is a messy thing to do IMO. I'd set it up like this: [code]<?php $Banners = Array(); // Init our banners $Banners[] = Array( 'link' => 'blah', 'graphic' => 'blah' ); $Banners[] = Array( 'link' => 'blah', 'graphic' => 'blah' ); $Banners[] = Array( 'link' => 'blah', 'graphic' => 'blah' ); $Banners[] = Array( 'link' => 'blah', 'graphic' => 'blah' ); $Banner = $Banners[(rand(1, count($Banners)) - 1]; echo $Banner['link'] . '<br />'; echo $Banner['graphic'] . '<br />'; ?>[/code]
-
$link = ${'bannerlink_' . $random};
-
How Do I Update PHP Files On My Server Using a Form?
roopurt18 replied to rushindo's topic in PHP Coding Help
You want the form to open a PHP file on your server, change the contents, and then save it? Why? -
User management accounts - private page per user....?
roopurt18 replied to Skeleten Neteleks's topic in PHP Coding Help
You typically create a template HTML file. This file holds the general page layout for all of your pages. Typically there is a string {CONTENT} that you replace with what actually appears on the page. [b]template.html[/b] [code] <!-- template.html - our template file !--> <html> <head> </head> <body> {CONTENT} </body> </html> [/code] [b]page.php[/b] [code]<?php // page.php - any page within our site // Build a bunch of content, storing it in $content // Now we are ready to display it $file = file_get_contents('template.html'); $file = str_replace('{CONTENT}', $content, $file); echo $file; ?>[/code] -
displaying intermediate page / message during wait times
roopurt18 replied to flyingboz's topic in PHP Coding Help
I'd look into AJAX. -
displaying intermediate page / message during wait times
roopurt18 replied to flyingboz's topic in PHP Coding Help
Well then, I'd say you're stuck. If you've already output something to the client, you can't erase it. So if the page says "Please wait..." it's not going to go away until you redirect to a new page. And since you've already output something, you can't redirect through PHP with a call to header as the headers have already been sent. -
Store the date as 'YYYY-MM-DD HH:MM:SS' format. [code]<?php $sql = "INSERT INTO table (TheDate) VALUES (" . "'" . date('Y-m-d H:i:s') . "')"; mysql_query($sql); ?>[/code] You can store current date and time using the NOW() MySQL function: [code]<?php $sql = "INSERT INTO table (TheDate) VALUES (NOW())"; ?>[/code] Pull the date out: [code]<?php $sql = "SELECT TheDate AS Unformatted, " . "DATE_FORMAT(TheDate, '%a. %b. %D, %Y') AS Formatted " . "FROM table WHERE 1"; $q = mysql_query($sql); if($q){ while($row = mysql_fetch_array($q)){ echo '<pre style="text-align: left;">' . print_r($row, true) . "</pre>"; } } ?>[/code] More information on DATE_FORMAT may be found here: http://dev.mysql.com/doc/refman/4.1/en/date-and-time-functions.html
-
displaying intermediate page / message during wait times
roopurt18 replied to flyingboz's topic in PHP Coding Help
AFAIK you can't do this without javascript (or possibly flash or AJAX). PHP runs entirely on the server which means you can't write a script that sends output to the client and then processes something on the server while updating the client page. You can only accomplish this with code that runs on the client to the best of my knowledge. You can perform a test to determine if the user has javascript enabled. Add something like: <div id="jscript_test"> <input type="hidden" name="jscript_test" value="false" /> </div> to the form. Add javascript to the page's onload function that changes the contents of that div tag to: <input type="hidden" name="jscript_test" value="true" /> The div tag's contents will only change if javascript is turned on. Then on your form handler you can check if the value of $_POST['jscript_test'] is true or not. If it's true you can use the flashy progress bar method I described above. If it's false just do all of the processing on the server and the client can wait until it finishes. -
Create a DATETIME column in your table. Store the date as 'YYYY-MM-DD HH:MM:SS' format. If you are storing the current date and time you can use the MySQL [b]NOW()[/b] function. When you pull the date out, it will be in 'YYYY-MM-DD HH:MM:SS' format, which may not be what you want to store it in. You can instead use the MySQL function [b]DATE_FORMAT[/b] to format the date to something else for display purposes.
-
You'll want to place a uniqueness constraint on the key column in the table. Any time you generate a key and it fails to insert into the DB, you'll know you need to regenerate another key.
-
displaying intermediate page / message during wait times
roopurt18 replied to flyingboz's topic in PHP Coding Help
I'll take a stab at one way of doing this. I have no idea if there is a better way though. This method depends on having PHP fire off a background script that will enable control to be returned immediately to the current script. Taken from the PHP manual: http://www.php.net/manual/en/ref.exec.php PHP at jyoppDot-com wrote: [i]Here's a function to launch a low-priority background process very simply, returning control to the foreground. I found that a lot of the other approaches shown here allow the script to continue running, and the page is served up to the user, but the PHP thread that launched the process cannot be re-used until the process has completed. Stdout and stderr need to be redirected to /dev/null to prevent this. This is for BSD/*NIX servers only (tested on Fedora Core and OSX). Win32 approaches would be necessarily very different. Omit 'nice' if you don't want to run the process with lowered priority. [/i] [code]<?php function fork($shellCmd) { exec("nice $shellCmd > /dev/null 2>&1 &"); } ?>[/code] This also depends on javascript being able to check for a file's existence on the web. Here would be the steps: 1) User fills out a form and submits with their credit card info. 2) Processing script will generate a unique ID for the request. Fire off a background script that takes the unique ID for the request in addition to any other details that actually processes the request. Control will be returned immediately to the form handler which will output a simple page with javascript. ** Now we have two separate things happening ** A) The form handler will echo out it's page containing javascript that shows some sort of progress bar. In addition, the javascript will continuously check for the existence of a file unique to this particular request on your web server. As soon as the unique file for the request is found on the webserver, redirect to the final processing / confirmation page. B) The background script is validating the credit card. As soon as it's valid and everything is ok, create the unique file so the client's javascript can terminate executing and redirect. If anyone has a better idea I'd be glad to hear it. I know nothing about it, but this might be better handled with Ajax? Again, I have no clue about that. -
Just a side note, datemod='Thursday 16th of November 2006 09:46:35 PM' You shouldn't be storing dates in that format if you can help it.
-
PHP can be used to create forms that capture data submitted by the user. PHP can be used to open other pages and / or files on the internet and pull data from them. If neither of those answer your question, then be more specific.
-
The basic MySQL syntax for what you want is: SELECT * FROM [i]table[/i] WHERE [i]condition_1[/i] AND [i]condition_2[/i] [i]condition[/i] can be things like: [i]table_column[/i] > 5 [i]table_column[/i] = 'howareyou' etc. So... SELECT * FROM table WHERE business_name LIKE '[i]name_from_form[/i]' AND business_type = [i]type_from_form[/i] You should start by finding a tutorial on writing an HTML form and just echoing out what was submitted. After you can do that you'll want to move on to using what was submitted to pull data from a database.