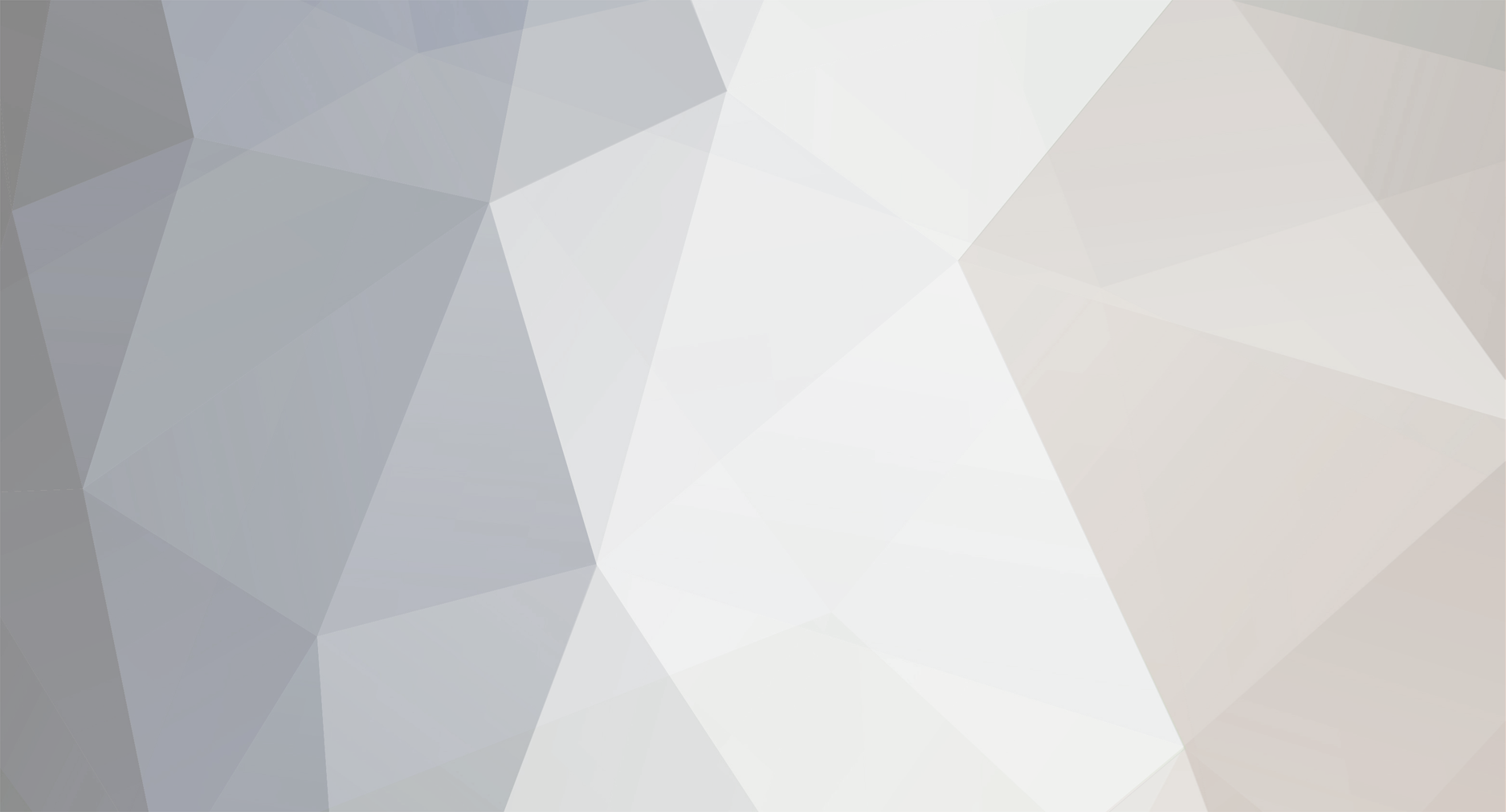
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
Let's say you had the following markup: <form id="frmExam" action="/exam.php" method="post"> <p> This is an examination of a(n) <select id="selTarget" name="selTarget"> <option value="child">child</option> <option value="adolescent">adolescent</option> <option value="adult">adult</option> </select> <select id="selGender" name="selGender"> <option value="male">male</option> <option value="female">female</option> </select> of <select class="has_other" id="selRace" name="selRace"> <option value="Caucasion">Caucasion</option> <option value="African American">African American</option> <option value="Asian American">Asian American</option> <option value="other">other</option> </select> <!-- assume class hidden means css: display: none; --> <input class="other_specifier" type="text" class="hidden" id="txtRace" name="txtRace" value="" size="10" /> descent. </p> <form> With Dojo I would do something like: // This is JavaScript /** * Event handler for elements with class="has_other" * * @param Event */ function event_HasOther_onChange( e ) { // e.target should be the SELECT element // We need the associated input var n = e.target.nextSibling; while( n && !dojo.hasClass( n, "other_specifier" ) ) { n = n.nextSibling; } // n should be the INPUT element following the SELECT element if( e.target.options[e.target.selectedIndex].value == "other" ) { dojo.removeClass( n, "hidden" ); }else{ dojo.addClass( n, "hidden" ); } } dojo.addOnLoad( function() { // Attach event handlers dojo.query( ".has_other" ).forEach( function( n, i, all ) { dojo.connect( n, "change", "event_HasOther_onChange" ); } ); } );
-
What kickstart said is correct. Your select method is only going to return one row. Also, php_query() never returns false so your loops will be infinite. echo $var['fetch']['firstName'] . $var['fetch']['lastName']; Congrats. You've just given yourself 10 extra characters to type every time you want to access a field (by having to type ['fetch']). I have to wonder why you don't just use PDO? Assuming $pdo is already an instantiated PDO object. $insert = $pdo->prepare( "insert into `yourtable` ( `first_name`, `last_name` ) values ( ?, ? )" ); if( $insert ) { $insert->execute( array( 'Larry', 'Smith' ) ); echo $insert->rowCount() . "\n"; $insert->execute( array( 'John', 'Jones' ) ); echo $insert->rowCount() . "\n"; } $q = $pdo->query( "select * from `yourtable` where 1=1 order by `last_name`" ); if( $q ) { echo $q->rowCount() . " people\n"; while( $row = $q->fetchObject() ) { echo $row->last_name . ', ' . $row->first_name . "\n"; } } Not that hard really.
-
Just remember when the user submits the form you have to check again that they have permission to update the field before you actually update it. If you send a form field to my browser disabled I can easily enable it and modify the contents.
-
Is it possible to create a bound column
roopurt18 replied to php_beginner_83's topic in PHP Coding Help
If you want to know what is being output by PHP it's not sufficient to examine the output as rendered by a browser. You need to use the 'View source' feature of whichever browser you're using to view the actual HTML source PHP sent to the browser. Had you done that, you would have seen: <select name="some_field"> <option value="1">Joe</option> <option value="2">Larry</option> <option value="3">John</option> <option value="4">Betty</option> </select> Now if you don't understand what that output means, there's a fine XHTML reference over at w3schools: http://www.w3schools.com/tags/default.asp http://www.w3schools.com/tags/tag_select.asp http://www.w3schools.com/tags/tag_option.asp If you understand that the text within value="" is what is sent back to PHP when the form is submitted, then it's pretty clear IDs are sent back while NAMEs are displayed. The intent of my posts was not to offend you, but to let you know there was more you could have done to check if the solution was correct yourself. Using PHP to create HTML that will then be rendered in a browser is a pretty core concept to the language; so is using the View source feature of the browser to examine the output. Now since you were articulate and asking a question most likely related to forms, I had assumed you knew how to do those things. Heck, you were even asking about a bound control, which is what other languages sometimes call such a feature. Anyways I was wrong and my apologies for offending you. -
You're 100% right. I should stop being childish and kissing up to thorpe. To the original poster, just add these two lines at the top of all your files and most of your problems will be fixed. error_reporting(0); ini_set( 'display_errors', '0' );
-
extension_dir doesn't change in php.ini
roopurt18 replied to WalterItinOrient's topic in PHP Installation and Configuration
I only bring this up because it's nailed me even when I thought I was correct. Which php.ini file does phpinfo() say it is loading? Not sure if it applies, but keep in mind the php.ini referenced by command line PHP and www PHP are not always the same. The fact that you say you edited php.ini and changed extension_dir seems to imply you got the wrong php.ini. The user account PHP (or web server) is running as doesn't have access to read the extension directory or some other such nonsense. -
Your scripts are broken on 5.2.9.7 as well. You just have a different level of error reporting set on that installation. Go on over to your 5.2.9.7 installation and at the top of a script add: error_reporting(0xffffffff); ini_set( 'display_errors', 'on' ); You should see much the same errors. The changes between PHP releases are well documented; there's no need to guess about anything. Of course guessing is never a good way to go about programming. BTW, everything thorpe said was dead on. Sensitive much?
-
How can i check if a field value contains only space characters?
roopurt18 replied to suzzane2020's topic in PHP Coding Help
You need to examine the raw output (or input) from the control to determine exactly what you need to remove. There could be empty paragraph tags, br tags, or all sorts of other crap submitted by a rich edit control that you'll have to handle. -
I knew I had a good reason for doing it that way; I had forgotten what it was though.
-
Could always change: function getPostValue( $name, $default = null ) { return isset( $_POST[$name] ) ? $_POST[$name] : $default; } // TO function getPostValue( $name, $default = null ) { return isset( $_POST[$name] ) && strlen( $_POST[$name] ) ? $_POST[$name] : $default; } Depends on your purpose.
-
Is it possible to create a bound column
roopurt18 replied to php_beginner_83's topic in PHP Coding Help
Did you look at the raw HTML that was produced? Did you submit the form and examine $_POST? That would have answered your question. -
I usually use something like this: <?php $wheres = array(); // Assume we have no wheres $driver = getPostValue( 'driver' ); $sdate = getPostValue( 'sdate' ); $edate = getPostValue( 'edate' ); if( $driver !== null ) { $driver = mysql_real_escape_string( $driver ); $wheres[] = "`driver`='{$driver}'"; } if( $sdate !== null ) { $sdate = strtotime( $sdate ); // Using strtotime gives the user more flexibility in their date formats if( $sdate !== false ) { $sdate = mysql_real_escape_string( date( 'Y-m-d H:i:s', $sdate ) ); $wheres[] = "`pu_date`>='{$sdate}'"; } } if( $edate !== null ) { $edate = strtotime( $edate ); if( $edate !== false ) { $edate = mysql_real_escape_string( date( 'Y-m-d H:i:s', $edate ) ); $wheres[] = "`pu_date`<='{$edate}'"; } } $wheres = count( $wheres ) > 0 ? (' where ' . implode( ' and ', $wheres ) . ' ') : ' '; $sql = "select * from `your_table` {$wheres}"; $q = mysql_query( $sql ); // you get the idea function getPostValue( $name, $default = null ) { return isset( $_POST[$name] ) ? $_POST[$name] : $default; } ?> And combining functions isn't any type of theory. 8P
-
Is it possible to create a bound column
roopurt18 replied to php_beginner_83's topic in PHP Coding Help
Why not try it and see? Part of programming is typing code, running code, and examining the output. You took the time to type out a well-thought question. rhodesa took the time to type out a well-thought response. You then immediately come back and ask him if it will do what you asked in the original post. This implies that: 1) You did not try it at all 2) You don't think rhodesa has the reading comprehension to understand your original post 3) (or maybe) You think your OP wasn't that clear after all. -
[SOLVED] Apostrophe Trouble - updating database
roopurt18 replied to EternalSorrow's topic in PHP Coding Help
You could have easily solved this yourself by now if you'd done a couple of things. I told you your arguments to sprintf() aren't in the right order. The best thing to do would have been to wander over to the PHP manual and read the documentation on sprintf(). By reading the documentation for the function you are calling, you determine whether or not you are using it correctly. Instead you opted to blindly remove or add things from your call to sprintf() and guess what? Your arguments to sprintf still aren't even in the right order. Also, when programs don't work you have to debug them because they have a problem. You need information to solve problems. You could easily alter your code to give you more information about what is causing the problem. Such as: $result = mysql_query($sql) or die($sql . ' resulted in error: ' . mysql_error()); Now when it dies, you'll get not only the MySQL error but the SQL statement that caused the error. Now if you actually take the time to read the SQL statement, you'll realize it's totally wrong. At that point you ask yourself, "Why is my statement wrong? Well I'm setting it with sprintf() so I better check my code against the documentation and make sure I'm using it correctly." Now don't take this the wrong way. I'm not being mean. I'm trying to teach you how to resolve your own issues. Lastly, you can remove the or die() on the sprintf() line; it's completely useless. -
[SOLVED] Apostrophe Trouble - updating database
roopurt18 replied to EternalSorrow's topic in PHP Coding Help
<?php $sql = sprintf(" UPDATE information SET author='%s', // a title='%s', // b summary='%s', // c datetime=NOW() WHERE `author`= '%s' // d AND title = '%s' ", // e mysql_real_escape_string($author), // a mysql_real_escape_string($title), // b mysql_real_escape_string($author), // c mysql_real_escape_string($title), // d mysql_real_escape_string($summary) // e ); ?> Your arguments to sprintf aren't even in the right order. You've got another serious flaw in your logic there as well that deals with setting author and title while using the same values to retrieve the record in your where clause. -
Show Specials between certain times / days of the week.
roopurt18 replied to hinchcliffe's topic in PHP Coding Help
Oh. I missed the assignment operator misuse in your conditional test; shame on me! Anyways you can always rewrite: if( $c ) { if( $a && $b ) { } } as if( $c && $a && $b ) { } They're logically equivalent. -
The whole point of your $picked variable is to optimize out repeated executions of that first line in the loop, right? If that's the case I'd say don't bother. The loop executes 12 times and doesn't do anything so computationally expensive that you need to optimize out a big chunk. Anyways I'd just write it like this: <?php $select = ' <select name="Month"> <option value="">Month</option>'; $selectedMonth = isset( $ymd ) ? $m : date( 'm' ); for( $i = 1; $i <= 12; $i++ ) { $select .= sprintf( '<option value="%s"%s>%s</option>', $i, $i == $selectedMonth ? ' selected="selected' : '', date( 'F', mktime( 0, 0, 0, $i ) ) ); } ?>
-
Show Specials between certain times / days of the week.
roopurt18 replied to hinchcliffe's topic in PHP Coding Help
It doesn't echo the special because his variable $special is empty. It's empty because his if conditions are all written incorrectly and none of them are passing or the wrong one(s) are passing. If you want to check if $time is between, for example, 9 and 15 inclusive, you'd use: if( $time >= 9 && $time <= 15 ) { -
"Trying to get property of non object" means you are using -> on a variable that is not an object. Since you are using $xml->METADATA that means $xml is not an object like you expected it to be. Why wouldn't it be an object you ask? Because simplexml_load_file() probably returns FALSE when it fails. Why would simplexml_load_file() fail you ask? Because the file you told it to open doesn't exist. And why doesn't it exist? Because you didn't escape your backslashes in the string. $xml = simplexml_load_file("C:\\greta05\\bin\\METADATA-TABLE.xml");
-
WEird Combination of fwrite and simplexml
roopurt18 replied to cardio5643's topic in PHP Coding Help
Open your php.ini and enable the php_curl extension. Make sure you restart your web server too. -
WEird Combination of fwrite and simplexml
roopurt18 replied to cardio5643's topic in PHP Coding Help
Change: error_reporting(0); to: error_reporting(0xffffffff); -
WEird Combination of fwrite and simplexml
roopurt18 replied to cardio5643's topic in PHP Coding Help
Well I guess it may be, though it should have the xml opening tag and version information as well. You are right about the casting. SimpleXML also has an asXML() method you might look into. -
You're going to annoy the moderators if you keep creating new topics. <?php $xmlFile = "../etc/someXMLfile.xml"; // line 1 $xmlHandle = fopen($xmlFile, "w"); // line 2 $flashXML = simplexml_load_string('<root><number>1</number></root>'); // line 3 fwrite($xmlHandle, 'test'); // line 4 fclose($xmlHandle); // line 5 ?> The reason that creates but does not write to the file is simple. Lines 1 and 2 are executed, line 3 is crashing and terminating the program. <?php $xmlFile = "../etc/someXMLfile.xml"; $xmlHandle = fopen($xmlFile, "w"); fwrite($xmlHandle, 'test'); fclose($xmlHandle); $flashXML = simplexml_load_string('<root><number>1</number></root>'); ?> Try that and you're file will be created. Now do like I said in your other-other thread and validate your XML before sending it to SimpleXML.
-
WEird Combination of fwrite and simplexml
roopurt18 replied to cardio5643's topic in PHP Coding Help
I've used PHP's XMLWriter, XMLReader, and SimpleXML libraries. They all work and some work better for some purposes than others. SimpleXML is sufficient for what you're trying to do. But you must remember that SimpleXML requires the received XML to be well-formed and syntactically valid. If those conditions aren't met you'll just be in a world of hurt. You also have to remember that elements and attributes in SimpleXML are always returned as SimpleXMLElement objects and not native data types.