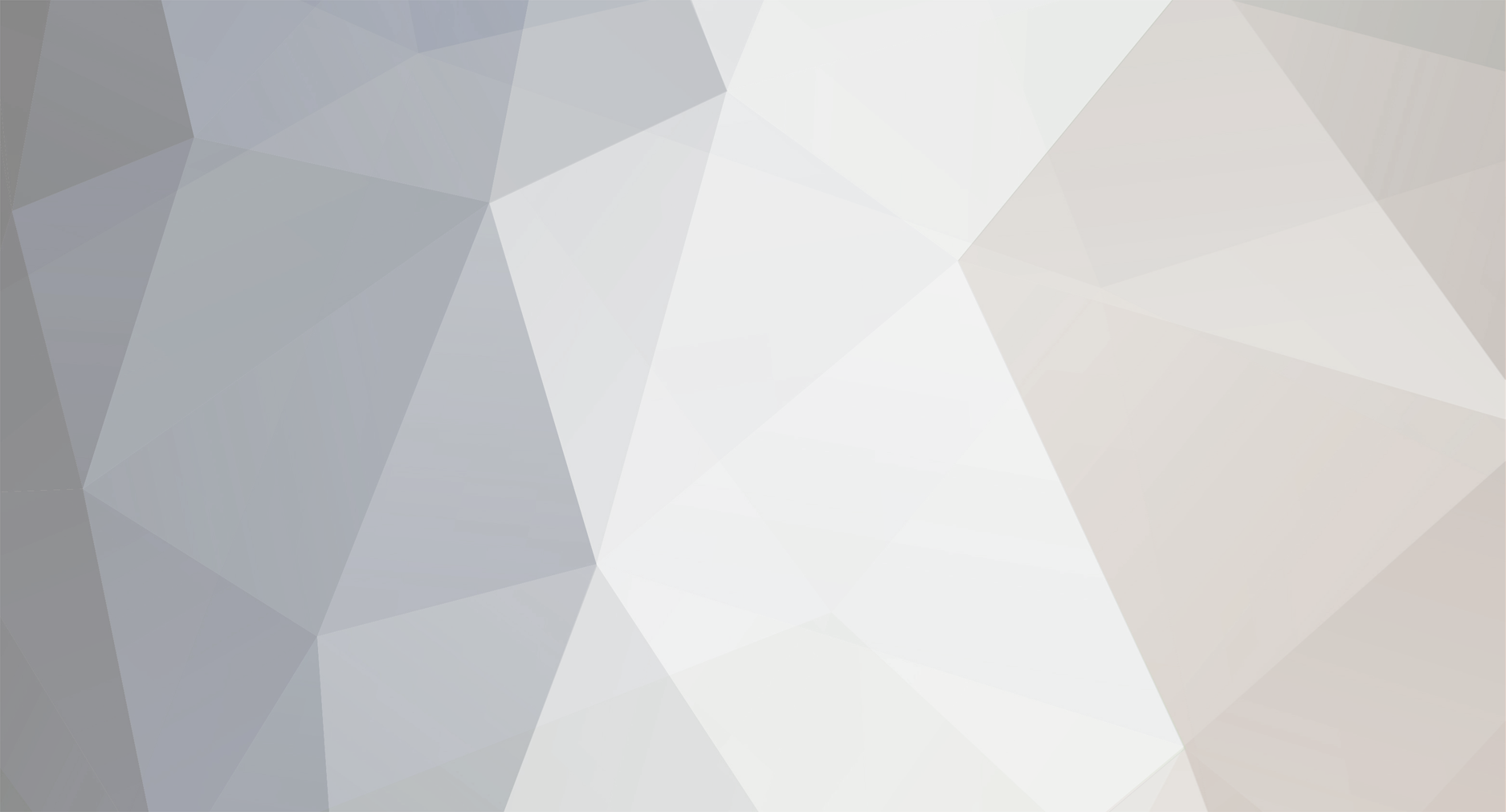
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
I, or anyone else for that matter, can submit to your forms without using their web browser. PHP has extensions that allow you to send POSTs to URLs as if you were a web browser. In that case it wouldn't matter that you told me the field was readonly; I'd just post to it anyways. Alternatively a user can open the page in their browser and then save it to their hard drive. You then edit the HTML on your local hard drive to change the form action to the appropriate URL and remove the readonly="readonly" from the input-tag. You can then open the file back up in a web browser, fill out the form, and submit it back to the originating web site. Therefore adding readonly="readonly" to the input is a good way of letting the user know they can't change it. But if you don't recheck their ability to edit the field on the page that receives the form POST, they'll just get around your readonly control in one of the manners I mentioned above.
-
This is what your output needs to look like: <input type="text" <!-- other stuff --> readonly="readonly" /> Load the page, view the HTML source, and find the input control you're trying to create. Find the part of it where you think the readonly="readonly" should have been and see what's actually there. Then change your script and try it again. Repeat this until the output (i.e. the HTML source sent to the browser) is what it's supposed to be. Here's a big hint: // You have this code in your post above...ITS NOT VALID PHP. <?php if(!$userIsAbleToEditThisBox) { echo readOnly="readOnly"; }?> // But this is: <?php if(!$userIsAbleToEditThisBox) { echo 'readonly="readonly"'; }?> And the documentation that I provided to you says it has to be: readonly="readonly" <-- That right there is what HAS to be in the HTML output readOnly="readOnly" <-- That is what you're trying to send. Why is your O capitalized? If the documentation has it lowercase, then you do it lowercase.
-
[SOLVED] Okay, I can't seem to wrap my head around this...
roopurt18 replied to applejuicerules's topic in PHP Coding Help
If I understand correctly you have a table with a DATETIME column and you want to count the number of rows entered per day? select date_format( `columnname`, 'Y-m-d' ) as `datecolumn`, count(*) as `n` from `yourtable` group by dayofyear( `columnname` ) order by `datecolumn` And you can just add a WHERE-clause for your filtering probably. -
In mathematical terms a set is a collection of items and an element is an item in the set. I could say: Let C be the set of all the items currently on my desk. Some of the elements in that set would be: a cell phone, a yellow high lighter, a quiznos cup, telephone, etc. When I use the term result-set, I mean the resulting set (or group) of records returned by the database query. Each element in the query result-set is a single database record (and each record is a collection (or set) of columns). When you do something like: $q = mysql_query( "select ..." ); // $q now contains a handle, or pointer, or reference to the RESULT-SET // mysql_fetch_assoc() returns the next ELEMENT from the result set. // Therefore if we want to loop over the result-set, we use: while( $row = mysql_fetch_assoc( $q ) ) { print_r( $row ); } This is why your code was wrong before. You were running the query and assigned the result-set to $go. Then you were calling mysql_fetch_*() and assigning the result to $fetch. In that case $fetch is a single row, and not the entire result-set. You were then returning $fetch back to the user. What kickstart is saying is you could do something like this in your function: if ( $type == 'SELECT' || $type == 'SHOW' || $type == 'DESCRIBE' || $type == 'EXPLAIN') { $go = mysql_query($query,$con) die (mysql_error() . ": " . $query); // Run the query, or echo the error. $fetch = array(); while($fetch[] = mysql_fetch_array($go)); // Take the output and create array $var[] // LOOK HERE!!!!!! // The above two lines loop over the entire result set ONE TIME $num = mysql_num_rows($go); // Count the number of affected rows. $array = array ( 'query' => $query, // $var['query'] returns the query. 'num' => $num, // $var['num'] returns the number of affected rows. // HERE YOU ARE ASSIGNING THE ARRAY YOU BUILT ABOVE (WHEN YOU LOOPED OVER THE RESULT-SET) 'fetch' => $fetch // $var['fetch'] returns the results of the query. ); So you could use your function like this: $result = pure_query( 'select', "SELECT * FROM ..." ); // REMEMBER THAT pure_query LOOPS OVER THE RESULT-SET ONCE ITSELF while( $row = $result['fetch'] ) { // AND NOW YOU ARE LOOPING OVER IT AGAIN, WASTE OF RESOURCE ON LARGE RESULT-SETS } My recommendation is to do the following: if ( $type == 'SELECT' || $type == 'SHOW' || $type == 'DESCRIBE' || $type == 'EXPLAIN') { $go = mysql_query($query,$con) die (mysql_error() . ": " . $query); // Run the query, or echo the error. $num = mysql_num_rows($go); // Count the number of affected rows. $array = array ( 'query' => $query, // $var['query'] returns the query. 'num' => $num, // $var['num'] returns the number of affected rows. // NOTICE I SET EQUAL TO $GO 'fetch' => $go // $var['fetch'] returns the results of the mysql_query(), which is the RESULT-SET ); So you could use your function like this: $result = pure_query( 'select', "SELECT * FROM ..." ); // pure_query no longer loops over the result set while( $row = mysql_fetch_assoc( $result['fetch'] ) ) { // but we have to use mysql_fetch_assoc() here }
-
Text entered in form inputs disappear after page reload
roopurt18 replied to RoBiNp's topic in PHP Coding Help
You have to make sure you are setting the proper attributes for controls so that a value is assigned to them. The attributes can be any one of (and it depends on which type of input you are using): value, checked, selected -
IF( DAYOFWEEK( NOW() )=5, NOW(), NOW() - INTERVAL 7 DAY ) AS `MyValue` * Not tested...
-
eval(), but it's dangerous.
-
extension_dir doesn't change in php.ini
roopurt18 replied to WalterItinOrient's topic in PHP Installation and Configuration
I'm not sure if this is relevant at all, but how did you install PHP the first time around? Did you just extract a zip file? Or did you run some .msi or .exe installation routine? Are you sure there aren't multiple extension_dir= lines in your php.ini? It makes no sense whatsoever that you can edit php.ini and have the changes take effect but that it ignores your extension_dir= change. Unless there's some other php configuration value that could affect it, such as safemode or something. -
You can use the HttpRequest object. http://us3.php.net/manual/en/class.httprequest.php Create the object and make the initial POST request. When the request returns you'll want to find the session cookie that came with it and set that cookie value into the HttpRequest object. From then on out the HttpRequest object will send the session cookie with any future requests and the web site will think you're a regular user. <?php $http = new HttpRequest( 'http://www.somedomain.com/login.php', HTTP_METH_POST ); $http->addPostFields( array( 'username' => 'jonny5', 'password' => 't0p53c23t5' ) ); $http->send(); $cookies = $http->getResponseCookies(); foreach( $cookies as $cookie ) { $http->addCookies( $cookie->cookies ); } echo $http->getResponseBody(); // Just to see what was returned $http->setMethod( HTTP_METH_GET ); $http->setUrl( 'http://www.somedomain.com/listusers.php' ); $http->send(); echo $http->getResponseBody(); ?>
-
[SOLVED] Multiple Field Search not Working
roopurt18 replied to kingnutter's topic in PHP Coding Help
Double check your queries (i.e. echo them out) and add some calls to mysql_error() to try and get more information about why it's not working. -
Well you obviously have the hang of arrays, form processing, and database interaction. So I'll give you a tiny hint and see if that doesn't help. Say you had this HTML markup in your form: <input type="text" name="first_name[]" value="Larry" /> <input type="text" name="first_name[]" value="Bob" /> ...many more inputs <input type="text" name="first_name[]" value="Betty" /> Notice the square brackets on the name-attribute? That causes the $_POST array to contain a sub array. echo $_POST['first_name'][0]; // Larry echo $_POST['first_name'][1]; // Bob //.. more echos echo $_POST['first_name'][count( $_POST['first_name'] ) - 1]; // Betty By carefully manipulating the name-attribute of your INPUT elements you can cause $_POST to already contain a structure similar to your database layout. <input type="text" name="records[0][first_name]" value="Jon" /> <input type="text" name="records[0][last_name]" value="Jones" /> <input type="text" name="records[1][first_name]" value="Sam" /> <input type="text" name="records[1][last_name]" value="Smith" /> <input type="text" name="records[2][first_name]" value="Karl" /> <input type="text" name="records[2][last_name]" value="Kluck" /> echo '<pre>' . print_r( $_POST ) . '</pre>';
-
It's not redefining it. The [] operator causes a value to be pushed onto an already defined array. It just so happens that if the array is not defined to begin with, PHP will create an empty one for you. Relying on the language to initialize variables for you is a dangerous habit to fall into. It allows you to be a lazy programmer and lazy programmers often make more mistakes. They also have many more problems moving their script from one version of a language to another because this default behavior is not set in stone and can change at any time. And I'll repeat, I do not recommend pre-building the array in that manner there.
-
[SOLVED] Multiple Field Search not Working
roopurt18 replied to kingnutter's topic in PHP Coding Help
The value in $field is the portion of the option-tag within value="". Change: if ($field=='All') To: echo $field . '<br />'; if ($field=='All') And then you'll see why $field is not equal to 'All'. -
Rather than guessing why not refer to some documentation on what is valid within an input-tag? http://www.w3schools.com/tags/default.asp http://www.w3schools.com/tags/tag_input.asp According to that it should be: <input type="text" <!-- other stuff --> readonly="readonly" />
-
While kickstart is correct in his altered version of your original function, I do not recommend doing that. The reason being that you will end up looping over your result-set an extra time. For small result sets this is no big deal. For large result sets it becomes a bottleneck in your script.
-
function to strip all non-alphanumeric characters?
roopurt18 replied to dadamssg's topic in Regex Help
You probably want to preg_replace twice. The first time as MrAdam suggested and the second time to remove consecutive spaces created by the first regexp. $str = preg_replace( '/[ ]+/', ' ', preg_replace('/[^a-z0-9]/i', ' ', $str) ); -
Well hex values typically start with 0x. What you've provided is just a series of hex digits. So based on your latest post: preg_match( '/[a-fA-F0-9]{1,8}/', $value )
-
[SOLVED] str_replace different url structures
roopurt18 replied to doa24uk's topic in PHP Coding Help
No need to provide credit. But if you feel you must you can just point back at the URL of this topic. Or my user profile. Let me know if you need my PayPal to pay royalties and such. -
Top-level documentation for PDO. http://www.php.net/manual/en/book.pdo.php The PDO class: http://www.php.net/manual/en/class.pdo.php The PDOStatement class: http://www.php.net/manual/en/class.pdostatement.php Whenever you feel like you're ready to tackle it, PDO presents a much more simple way of interacting with your database. All you do is create a new PDO object: $pdo = new PDO( $dsn ); And then run prepare() or query() calls. If you played with the examples enough you might actually be able to use it without fully understanding OOP. Anyways...if you look at your function you wrote: $go = mysql_query($query,$con) die (mysql_error() . ": " . $query); // Run the query, or echo the error. $fetch = mysql_fetch_array($go); // Take the output and create array $var[] $num = mysql_num_rows($go); // Count the number of affected rows. $array = array ( 'query' => $query, // $var['query'] returns the query. 'num' => $num, // $var['num'] returns the number of affected rows. 'fetch' => $fetch // $var['fetch'] returns the results of the query. ); You are calling mysql_fetch_array() and returning that. mysql_fetch_*() fetches a row from the result-set, not the result-set itself. So your function is running the query, fetching the first row returned, and returning that to the user. What you want to return is the result of mysql_query() (which will be the result-set). $go = mysql_query($query,$con) die (mysql_error() . ": " . $query); // Run the query, or echo the error. // DELETE THE NEXT LINE //$fetch = mysql_fetch_array($go); // Take the output and create array $var[] $num = mysql_num_rows($go); // Count the number of affected rows. $array = array ( 'query' => $query, // $var['query'] returns the query. 'num' => $num, // $var['num'] returns the number of affected rows. // NOTICE THE DIFFERENCE ON THE NEXT LINE 'fetch' => $go // $var['fetch'] returns the results of the query. );
-
[SOLVED] str_replace different url structures
roopurt18 replied to doa24uk's topic in PHP Coding Help
I don't know why I didn't just use regexp on the whole thing. <?php $urls = array(); $urls[] = 'http://www4.site2.com'; $urls[] = 'http://site3.com'; $urls[] = 'http://www15.site4.com'; $urls[] = 'http://www15.site4.com/alsjdflsjf/asdflajsfdlajsf?lsdjflj&lsdjflsf&lsjdflsjf=alsdjflasjf&lsfjlsdf'; foreach( $urls as $url ) { echo print_r( get_domain( $url ) ) . '<br />'; } /** * Extracts the domain from a URL * * @param string $url * @return string | boolean */ function get_domain( $url ) { if( preg_match( '@^http://(www[^.]+\.)?([^/]+)@', $url, $matches ) ) { return $matches[2]; } return false; } ?> -
Checking the documentation, curl_exec() returns a resource, not a string. Try casting it to a (string) when you use it.
-
<?php $xml = new SimpleXMLElement( "<?xml version=\"1.0\" encoding=\"UTF-8\" standalone=\"yes\"?> <response> <results> <![CDATA[An error occured %s]]> </results> <status> <![CDATA[]]> </status> </response>" ); print_r( $xml ); ?> That works for me. Try adding a var_dump( $result ) to your code and see what $result actually is.
-
[SOLVED] str_replace different url structures
roopurt18 replied to doa24uk's topic in PHP Coding Help
Updated for what you want: <?php $urls = array(); $urls[] = 'http://www4.site2.com'; $urls[] = 'http://site3.com'; $urls[] = 'http://www15.site4.com'; $urls[] = 'http://www15.site4.com/alsjdflsjf/asdflajsfdlajsf?lsdjflj&lsdjflsf&lsjdflsjf=alsdjflasjf&lsfjlsdf'; foreach( $urls as $url ) { echo get_domain( $url ) . '<br />'; } /** * Extracts the domain from a URL * * @param string $url * @return string */ function get_domain( $url ) { if( strpos( $url, '://' ) !== false ) { list( $throwAway, $url ) = explode( '://', $url ); } if( strpos( $url, '/' ) !== false ) { $url = explode( '/', $url ); $url = array_shift( $url ); } if( preg_match( '/^www[^.]*\..*/', $url ) ) { $url = explode( '.', $url ); array_shift( $url ); $url = implode( '.', $url ); } return $url; } ?> -
[SOLVED] str_replace different url structures
roopurt18 replied to doa24uk's topic in PHP Coding Help
<?php $urls = array(); $urls[] = 'http://www4.site2.com'; $urls[] = 'http://site3.com'; $urls[] = 'http://www15.site4.com'; $urls[] = 'http://www15.site4.com/alsjdflsjf/asdflajsfdlajsf?lsdjflj&lsdjflsf&lsjdflsjf=alsdjflasjf&lsfjlsdf'; foreach( $urls as $url ) { echo get_domain( $url ) . '<br />'; } /** * Extracts the domain from a URL * * @param string $url * @return string */ function get_domain( $url ) { if( strpos( $url, '://' ) !== false ) { list( $throwAway, $url ) = explode( '://', $url ); } if( strpos( $url, '/' ) !== false ) { $url = explode( '/', $url ); $url = array_shift( $url ); } return $url; } ?> -
extension_dir doesn't change in php.ini
roopurt18 replied to WalterItinOrient's topic in PHP Installation and Configuration
Loaded Configuration File That's the file you want to be editing, which you are. Except that the "extension_dir" doesn't change, it keeps saying "C:\php5", whereas I changed that in my ini-file into "E:\php\ext". That doesn't make sense to me though. phpinfo() says the extension_dir is c:\php5? Lastly, whatever your extension directory really is, did you make sure the php_mysqli.dll is present and the proper version for your version of PHP? I know that some versions of the postgres dll I've had bundled with my installation have been faulty.