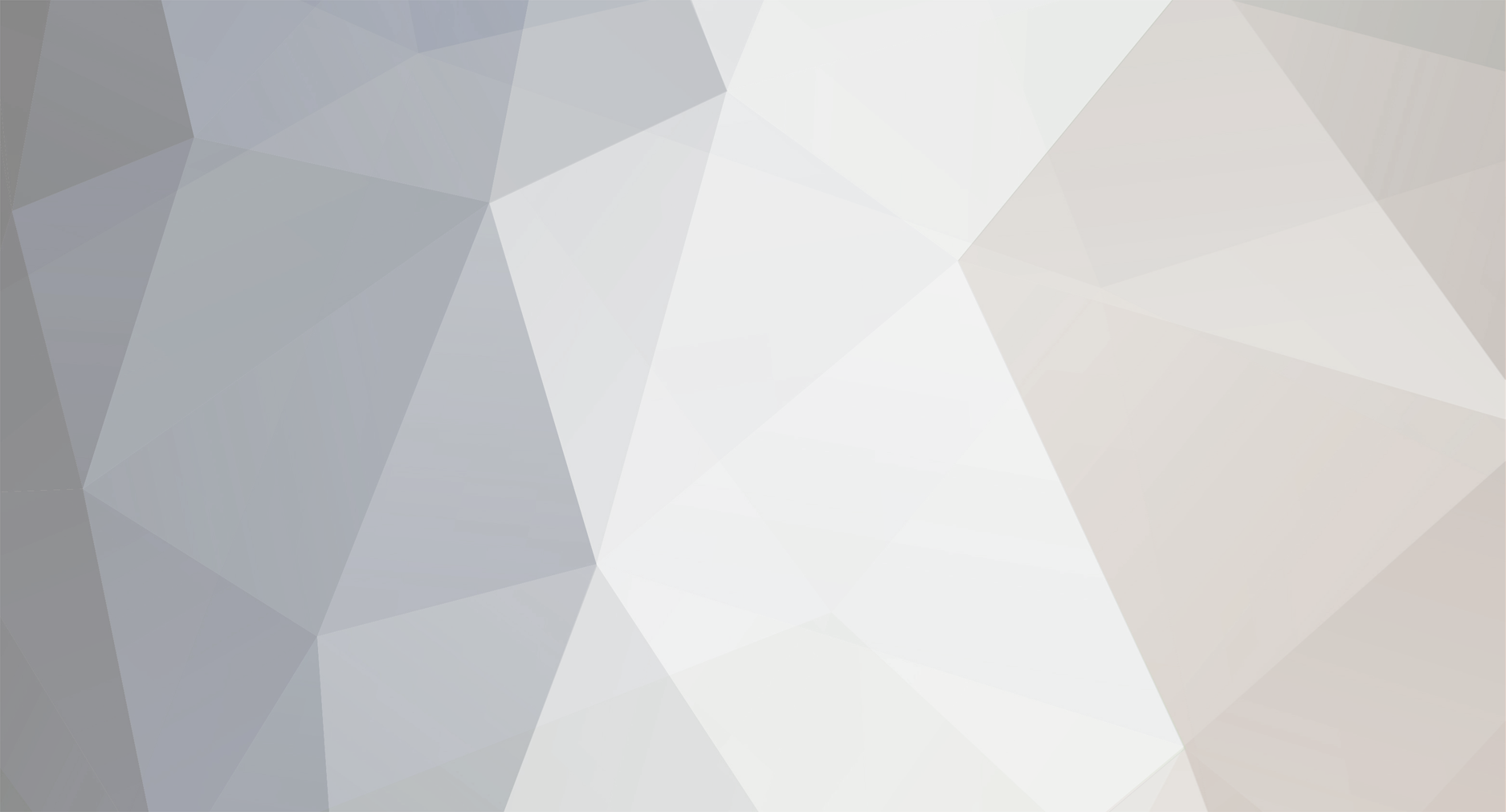
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
That is not a terrible difficult thing to do. All it requires is a form and MySQL database. It is hard enough to make a single game that is popular and people want to play. You are wanting to make at least three. I would suggest making a couple of games that you host, run, and manage before you even attempt to worry about creating a 'Game Management Software.'
-
[SOLVED] Using PHP to rewrite bits of a JavaScript file
roopurt18 replied to KevinM1's topic in Application Design
Javascript can access any variables declared by other Javascript as long as they were declared before you try and access them and its within a scope you can see. You don't need to modify the existing file or bother with an AJAX approach, you can just use JSON. <?php $config = // read stuff from database $json = // convert config information into a JSON string echo <<<JS <script type="test/javascript"> var gConfig = exec({$json}); // <-- convert your JSON string into an actual object alert(gConfig.someProperty); // <-- show that we can display a property of the object, i.e. that it worked </script> JS; ?> -
Both constants.php and index.php should reside in your top-level web-accessible directory; this is usually the directory you land in when you FTP to your account. If your web host supports it, you can set up mod_rewrite via a .htaccess file; it should reside in the same directory as index.php and constants.php. I won't bother with the implementation details as there's plenty of tutorials on it and even a specific thread area on this discussion board for mod_rewrite. Depends on how you're using the images. If you're just passing URLs back to the browser then it's not the PHP interpretor that's seeing the paths but the browser, in which case '/' would refer to the directory starting from public_html (or www or whatever your web host calls it). Remember, / is the top-level directory on a *nix file system. Any time in your PHP code you try and work on a file: /path/to/some/file it will look for that file relative to the top-level directory of the file system. Basically, / on *nix is equivalent to c:\ on Windows. The reality is that you probably have an account on that file system and your account is mapped to: /home/users/your_account_name/ And finally within your directory is the one accessible via web browsers, usually named public_html or www: /home/users/your_account_name/public_html Thus in your PHP code: <?php include('/some/include/file.php'); /* Above maps to: /some/include/file.php It DOESN'T map to: /home/users/your_account_name/some/include/file.php */ ?> However if you were to do: <?php echo '<img src="/images/house.jpg" alt="A House"/>'; ?> This path is seen by the web browser. The web browser sends a request to www.yourdomain.com for the file: /images/house.jpg As far as the web browser is concerned, the path / is mapped to: /home/users/your_account_name/public_html Thus, the path: /images/house.jpg is mapped to: /home/users/your_account_name/public_html/images/house.jpg I probably didn't explain that as clearly as I could have, so here is an attempt at a short summary. A file path beginning with '/' from within PHP refers to the root directory of the server. This is not the same thing as a src attribute in the HTML that begins with '/', which simply means start the path at http://www.yourdomain.com/. The reason its not the same is http://www.yourdomain.com is not mapped to the root directory of the entire server; instead, it is mapped to the web-accessible directory of your account.
-
best way to give page wise user access/denial on my website.
roopurt18 replied to thyscorpion's topic in Application Design
Admin = 5 Staff = 4 Creator of Album = 3 Family Member = 2 Regular Old User = 1 Guest = 0 I wrote my first PHP site using that mechanism and while it's perfectly fine, it falls apart when you want to overwrite permissions for individual users. Cookies are not a good way to do this as users can manipulate them. The best form of access control is with a users / groups / permissions system. -
You may be running into problems with variable scope. The easiest way around this is to use mod_rewrite to direct all request through a single PHP script. From that script you can define constants to use throughout the site; I highly recommend using constants over a variable for what you're doing. Example directory structure: /home/username/public_html/ /home/username/public_html/index.php /home/username/public_html/constants.php /home/username/public_html/Models /home/username/public_html/Models/user.php /home/username/public_html/Models/news.php First you set up mod_rewrite to direct all requests through index.php. index.php require_once(realpath(dirname(__FILE__)) . 'constants.php'); // Examine the URL and route as necessary constants.php define( 'DIR_SRC', realpath(dirname(__FILE__)) ); // source root directory define( 'DIR_MODELS', DIR_SRC . 'Models/' ); // Model directory // define more constants as necessary random_file.php // We got here through index.php, thus all the constants in constants.php are declared include(DIR_MODELS . 'user.php'); $user = new User(); $user->throwOffCliff();
-
Because you need to pass it a string when you call it: action('text');
-
Remember that the path is relative to the file system and not the URL. So in your example: $base = '/mybaseurl/'; PHP would start at the highest directory (root) and look for the directory mybaseurl, which most likely does not exist.
-
Which will require a first query, bringing the total to two. In addition, since you're joining the table to itself you'd have to constantly generate aliases: SELECT t1.*, t2.pid AS t2pid, t3.pid AS t3pid, etc. I'm not saying it's not do-able, just that it adds a level of complexity to the PHP that may make it not worth the trouble.
-
I could be wrong, but I don't really think you can do all of this in a single query. What fenway is hinting at is you'll need to run two queries. The first query will be one that calculates the deepest nesting that exists, call this number X. The next query will contain X - 1 joins. You'll have to generate appropriate aliases for columns at each join which could possibly get messy. IMO, it may be easier to add a small integer column to the table, call it nesting. Items at the top have a nesting of zero, any time you insert a child item, just set it's nesting to one more than the parent's. Then you can query everything at once if you slap ORDER BY `nesting` into the query. Then with an appropriate loop, you can create the entire hierarchy in a single pass and with a single query. That may be the only way to do this without repeated database queries and without creating what could be a potentially complicated series of JOINS. (edit) I've never dealt with a table that was joined with itself to create nesting though, so someone else jump in and tell me if I'm full of crap if necessary.
-
There is also number_format()
-
[SOLVED] T_ENCAPSED_AND_WHITESPACE problem
roopurt18 replied to biggerboy's topic in PHP Coding Help
ip='$_SERVER['REMOTE_ADDR']' The above snippet is what is causing the problem. You have single quotes that denote strings to MySQL but then you have other single quotes that denote the index into the $_SERVER array. It is always recommended when embedding variables into double-quoted strings to enclose them in curly brackets. ip='{$_SERVER['REMOTE_ADDR']}' -
[SOLVED] implode multidimensional array column...
roopurt18 replied to mkosmosports's topic in PHP Coding Help
I hate when I do that. -
I changed it to 100% and it worked fine in FF. You did reload the page, right?
-
A browser can have up to two simultaneous requests to a single domain, so the answer is yes.
-
doc_table docs_to_region_table regions_table --------- -------------------- ------------- keyid <-------> doc_id +-----> keyid doc_type region_id <-----------+ region date title abstract etc.
-
Voting system... preventing duplicate votes?
roopurt18 replied to scooter41's topic in PHP Coding Help
Not to mention that a properly indexed MySQL database should be able to handle millions of records. If you did manage to have so much data that performance really became an issue, you would want to look into maybe pre-calculation or load balancing. -
Playing with it a bit more, I see that any time I specify the third argument it returns null. (EDIT: Duh, it was introduced in 5.0 /slaps_forehead) Why not just write your own version?
-
Perhaps it doesn't work with floats? Using PHP 4.4.4: <?php header('Content-type: text/plain'); $num = "4.3"; echo "hi\n"; $style = range(4.1, 5.0, 0.1); print_r($style); // We see 4.3 in the array $style echo $style === null ? "null\n" : "not null\n"; echo "bye\n"; if(in_array($num, $style)) { echo "$num Found in Array!!!\n"; } else { echo "$num Not SO MUCH \n"; // But according to in_array, $num is not in the array $style } ?> Produces hi null bye 4.3 Not SO MUCH
-
Oh but they do, just not with the same rules that you or I are used to. From a business perspective, minimal return on investment or money paid for services not rendered is illogical. Are these dealers paying your company for advertising space or exposure?
-
Need to find where to put BR tag in a WHILE loop result
roopurt18 replied to randalusa's topic in PHP Coding Help
You should be setting your styles via classes, that will get the CSS out of the HTML and make things appear more tidy. -
Need to find where to put BR tag in a WHILE loop result
roopurt18 replied to randalusa's topic in PHP Coding Help
Gah. Using tags as filler to create whitespace is like going back in time. IMO you should take the time to research CSS and use that instead. w3schools has some useful information. -
Apparently whoever the OP is working for or with disagrees.
-
Well, there truly is no such thing as random in a computer, which I'm sure you're aware. Consequently, random numbers will tend lean towards a specific area within the total range, especially when the total range is small, say 1 to 10 or 100. You could argue it any way you wanted to, but to ensure a "normal" distribution over a certain range you would have to keep track of what is being generated and occasionally seed the generator.
-
Sounds to me where they want a more controlled random sequence. As part of a game I once wrote, I had to generate a 3D scrolling landscape that would go on indefinitely. The landscape was mostly hilly with some water and once the hills got low enough it would appear to be all water. My algorithm to generate heights used a random number generator that would eventually bottom out, causing long stretches of water. In order to compensate, I kept track of how many numbers fell within a certain range and if there wasn't enough variation I would kick a set value into the system to push the terrain up.
-
You might want to encapsulate the division with a call to floor().