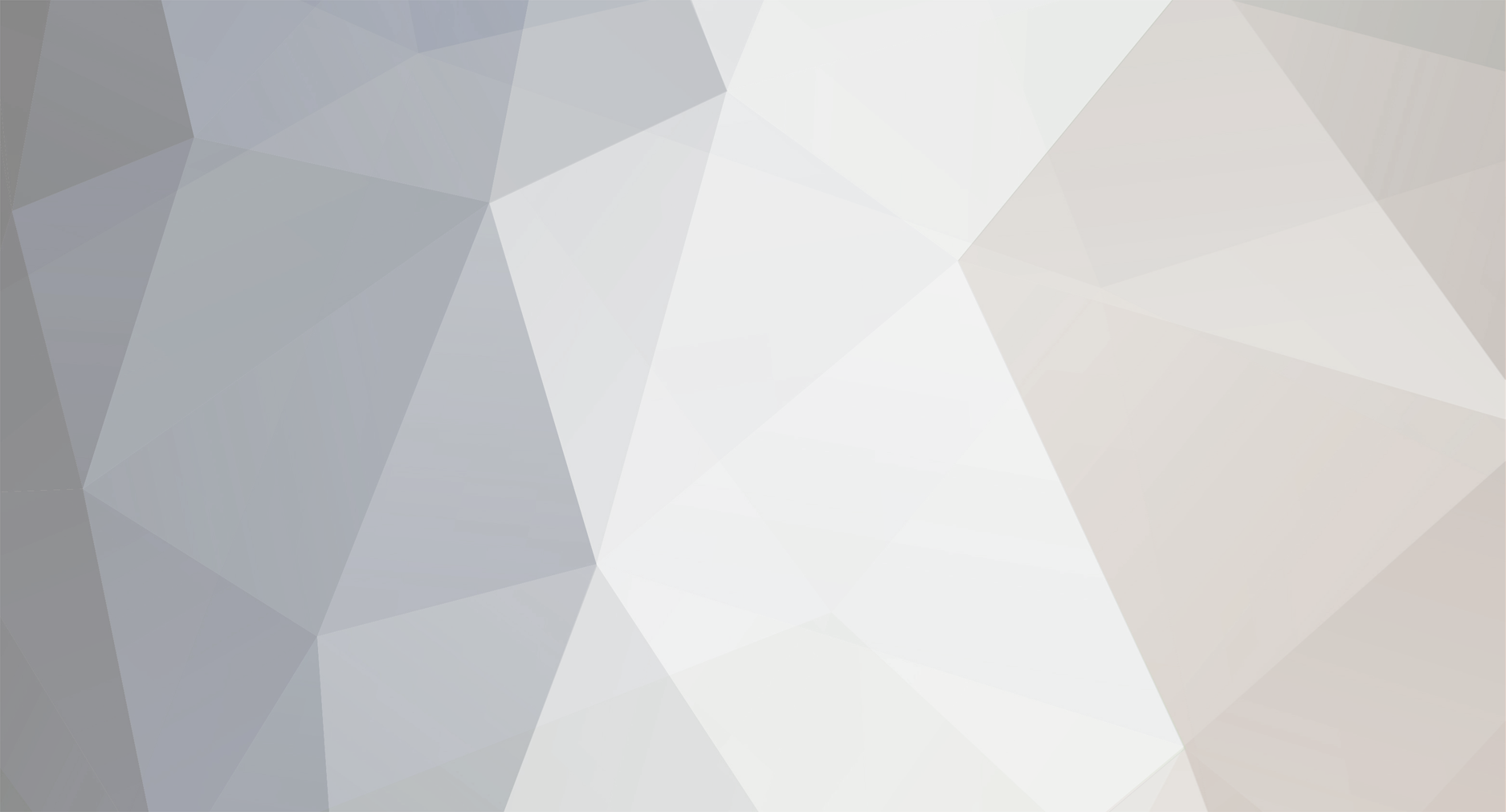
Psycho
Moderators-
Posts
12,164 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
newbie question regarding inline form validation
Psycho replied to dudleylearning's topic in PHP Coding Help
The function I provided was only for the purpose of outputting the form since there may be different branches of logic needing to output the form. There was other functionality I provided for the logic of the in-line errors. As it states in my signature, I don't always test the code I provide - it is provided as a guideline for the recipient to write their final code and properly test. So, I'm not going to test the code. But, the only thing that jumps out at me as a possibility is variable scope. Since the form.php script is included in the function, perhaps the execution of that script has the same scope as the function. If, that's the case you could resolve it by passing the $formErrors array to the function showForm($dbConnection, $formErrors) Be sure to pass the variable when calling the function and adding the parameter where you define the function. -
Opinion: strip_tags on field that will be encrypted in the database
Psycho replied to LLLLLLL's topic in PHP Coding Help
There is no good reason to use strip_tags() or any other functions that will modify the content of a password other than a valid hashing algorithm. There are very few reasons why strip_tags() needs to be used for any purposes. Data should be properly escaped for the processes it will be used for. For DB operations, prepared statements should be used. For output to a web page, htmlspecialchars() or htmlentities() should be used. For any use of data, determine how data should be escaped rather than trying to remove problematic characters. -
I see that in the inner table (that holds the form) most of the rows contain two cells (i.e. TDs). However, there are a couple rows at the end that have three cells. If you are seeing some anomaly in the layout, it could be due to this. You should use the same number of cells per row or use rowspan on certain cells within a row that won't have the maximum number. When I am using any tables on a page, I will always set them with a border when developing the layout so I can "see" the table - then remove the border when I'm done if one is not wanted.
-
OK, there are two problems with this line data: $('form').serialize, As requinix said, serialize is a function not a property. Function are called with parens: e.g. serialize(). Also, you cannot call 'form' since you have three forms on your page. Even if you correct the serialize function it will always pick the last form (i.e. the 2 value). Fortunately, jquery gives you a way to use the data of the form that called the function. And, requinix gave the the proper way to call it in step #1 of his first response.
-
Display Data from phpMyAdmin Database to php Table
Psycho replied to WyvernFrog's topic in PHP Coding Help
And what are the results of your script? Do you get errors, a blank page, or what? -
I would comment on what the OP is wanting to implement. But, then I would have to ban myself for language.
-
newbie question regarding inline form validation
Psycho replied to dudleylearning's topic in PHP Coding Help
You should create functions (or classes) rather than putting everything in-line. function showForm($dbConnection) { //Get list of authors try { $result = $dbConnection->query('SELECT id, name FROM author'); } catch (PDOException $e) { $error = 'Error fetching list of authors.' . '<br />' . $e -> getMessage(); include '../includes/error.php'; exit(); } //Put values into array foreach ($result as $row) { $authors_in_db[] = $row; } //Call the form script include 'form.php'; exit(); } Now, wherever you need to include the form call the function above. -
newbie question regarding inline form validation
Psycho replied to dudleylearning's topic in PHP Coding Help
Lot's of ways to solve this. With the current configuration you have here is one: Instead of saving errors to the string variable $texterror, save the validation errors for each field to an array such as $formErrors using the field name as the index. E.g. $formErrors = array(); if ($_POST['joke_text'] == '') { $formErrors['joke_text'] = '* Joke text field cannot be empty.'; } if ($_POST['joke_text'] == '') { $formErrors['author'] = '* Author field cannot be empty.'; } Then, after all validations are completed, check to see if $formErrors is empty. If no, continue with saving the data. If yes, include the form script. Lastly, on the form page, add logic on each field to check for any validation errors and show any errors in-line with the fields <label for="joke_text">Type your joke here:</label> <textarea id="joke_text" name="joke_text" rows="3"></textarea> <span class="error"><?php echo $formErrors['joke_text'];?></span> Note: since you don't want to show the asterisk if there is no error, include it as part of the error text or add logic to dynamically add it if there is an error for a particular field. The former is much easier to implement. Also, be sure to trim() inputs before checking if they are empty. Else, someone can enter only a space (or other non-printable characters) and the value will pass validation. -
Aside from Barand's response, I would add the following comments on your code. In the first code you posted, the conditions in the if() clause was wrong. Each condition joined with AND/OR clauses must be independent. if( strtotime($my_date) > strtotime('-7 day') && < strtotime('-14 day') ) { The red condition is incomplete. On your second posted code, the conditions are impossible! A date cannot be greater than yesterday (-1) AND also be less than 7 days ago. The operators need to be reversed.
-
@fivestringsurf: How long does this process take? I can't imagine why an AJAX process to report on the status would lock up the browser. I'm guessing you are unnecessarily passing extraneous data back and forth and the browser is failing at some limit. Or, perhaps this part of the process "The js would receive the id and keep polling the DB to check" is to blame. What does the query look like that is poling the database and how often are you poling? That query could be a resource hog and causing the system to hang. You should make sure you have relevant indexes in your tables and are using efficinet queries (e.g. use a COUNT() in the SELECT statement as opposed to querying all of the rows and then calculating how many rows were returned) Kicken's and Jacques1's suggestions are viable alternatives, but I see no reason why your current approach would not work - if implemented appropriately.
-
truncate a RSS-feed with PHP string functions like substr
Psycho replied to Maze's topic in PHP Coding Help
I'm not going to read through your entire post. But, based on what I understand, I would create a function to output the limited string. Then when you need to output the title either use the title directly (if you want to use the whole thing), or use a function to limit the output accordingly. I have an old function for just this purpose. e.g. //Return a string up to a certain number of characters //but will break on a space function truncateString($string, $length, $ellipse='…') { //Return truncated string w/ ellipse (if >$length) return substr($string, 0, stripos($string, ' ', $length)) . $ellipse; } $string = "This is a string with a lot of words that may need to be limited in some places in the output"; echo "<b>Output the full string:</b><br>\n"; echo $string . "<br><br>\n"; echo "<b>Output the limited string:</b><br>"; echo truncateString($string, 25); Output: -
Here is a break down of the expression. #^[a-z] ?\d{6}$#i The hashmarks are used to delineate the beginning and ending of the expression. The 'i' after the ending of the expression definition indicates that the expression should be case-insensitive. #^[a-z] ?\d{6}$#i The "^" and "$" indicate that the match must start from the beginning and end of the string (i.e. the match can't be inside the string) #^[a-z] ?\d{6}$#i The first character must match a character within the character class of "a-z" #^[a-z] ?\d{6}$#i The next character to match is a space, but the "?" indicates that it is optional #^[a-z] ?\d{6}$#i The "\d" is shorthand for any character that is a digit. The "(6)" following it means that there must be 6 instances of the preceding match.
-
RegEx is complicated. There is no way to boil down to a single page in the manual. You will need to learn it if you want to understand it. The \p is a delimiter for certain programming languages. It is not used in PHP. $values = array( 'a123456', 'A123456', 'a 123456', 'A 123456', '1234567', //no letter 'a12345', //less than 6 digits 'a1234567' //greater than 6 digits ); foreach($values as $value) { $match = preg_match("#^[a-z] ?\d{6}$#i", $value) ? "Valid" : "Invalid"; echo "{$value}: {$match}<br>\n"; } Output a123456: Valid A123456: Valid a 123456: Valid A 123456: Valid 1234567: Invalid a12345: Invalid a1234567: Invalid
-
No. Any auto-generated ID would be 1 more than the current highest number. I thought that was what you wanted, but apparently not. I agree with the others that what you are trying to achieve is not worthwhile. Requirements should be based on a legitimate business need, not because someone wants the data in the database to be neatly organized how they *think* it should be organized. What do you want to happen if an item is ever deleted? Should that ID be reused? What you want can be achieved pretty easily, but I'm not going to provide a solution when I feel it is counter to good practices.
-
The easiest solution that comes to mind is to use an auto-increment integer id field for the records. Then do not use the "MM" before the id value (in the database). you can always append the "MM" when selecting the records or when displaying them. When a user manually enters an ID you can choose whether to allow them to enter with or without the "MM" (or both) and just strip the MM before inserting. Using an auto-increment ID field will work without any special logic. You can add a new integer ID that does not already exist and if you try to insert a new record without the ID a new one will be generate automatically that is +1 from the highest existing value.
-
I think the only solution is to use JavaScript. I would change the configuration so that the style properties are applied to a class defined in the input field instead of the id of that element. Then have an onchange event for the field to change the class accordingly. Working example: <html> <head> <script> function updatePlaceholderClass(fldObj) { //Set fieldClass based on whether the field has a value var fieldClass = (fldObj.value=='') ? 'placeholderInput' : ''; fldObj.className = fieldClass; return; } </script> <style> .placeholderInput { background-color: green; color : yellow; } .placeholderInput:focus { background-color: #FFFFFF; color: #000000; } .placeholderInput:hover{ background-color: white; } .placeholderInput:hover::-webkit-input-placeholder{color:yello;} </style> </head> <body> <input type="text" id="Editbox4" class="placeholderInput" style="position:absolute;left:50px;top:458px;width:328px;height:30px;line-height:26px;z-index:4;" name="phone" value="" placeholder="Enter Phone Number" onchange="updatePlaceholderClass(this);"> </body> </html>
-
Regular Expression for currency number format replacement
Psycho replied to tezuya's topic in Regex Help
Hmm, OK I'm probably more confused now. If you have a string that contains a numeric value you should either convert the string to a numeric variable using something like floatval() if the string can be converted or parse the string to a value that can be interpreted as a numeric value. THEN, use the PHP functions to determine how the value would be displayed. RegEx has it's place, but with respect to this problem, I don't think it is a good solution. If your input is in the European format of 123,456.78 you can use a simple str_replace() to convert it to a string that can be interpreted as a numeric value. Then you can use number_format() to output the numeric calue. In this case, the conversion of the string to a numeric value will put it in the format that you want. But, I would still use the function number_format() to define how the number should display. Don't combine the processes of interpreting the number within a string and the format for the output of the string. //Input value $input_str = "234.454,54"; //Convert to a numeric variable $number_float = floatval(str_replace(array('.',','), array('','.'), $input_str)); //Create number output_add_rewrite_var $number_output = number_format($number_float, 2, '.', ''); echo "<br>Input string:\n"; var_dump($input_str); echo "<br>Number float:\n"; var_dump($number_float); echo "<br>Number output:\n"; var_dump($number_output); -
Regular Expression for currency number format replacement
Psycho replied to tezuya's topic in Regex Help
I am unclear on what the exact input looks like. Can you provide an example? The regular expression you have above looks to be way over-complicated. As for formatting the value - do not use regular expression. PHP has a couple of built in functions for formatting numbers and currency. Use RegEx to extract the data and use those functions to format the output. EDIT: After looking at your RegEx closer I *think* that the input ($num) is only the number that you are trying to format. I was first thinking that the 'number' was included in other text in the input. Again, if you confirm what the input looks like we can provide a better solution. -
Converting arrays into a multi-dimensional array
Psycho replied to LTMH38's topic in PHP Coding Help
Well, what do you want the format of this multi-dimensional array to be? I typically use something like this 'folder1name' => array( 'files' = array(list of files in folder1name), 'folders' = array( 'subfolder1name' =>array( 'files' => array(list of files in folder1name), 'folders' = array( //Same format if there are more subfolders ) ), 'subfolder2name' =>array( 'files' => array(list of files in folder1name), 'folders' = array( //Same format if there are more subfolders ) ) ) ) -
The closest industry standard title I can think of is: Graphic designer
-
I don't need to know anything. I'm not going to do any research on this. I'm just giving you a suggestion to try and get a good response. But, I would expect more information. Do you need authentication by the users, is there an approval process needed, what administrative function are needed and what can be done by "users"? E.g. edit/delete reservations. Do you need an approval process or is it first-come/first-serve? Can a user book as many hours and days as they want? Etc., etc.
-
A simple google search of "PHP Booking application" brought up many results. You would need to review them to see if they meet your needs. Since we don't know your specific requirements, it's kind of hard for anyone to recommend anything.
-
why my explode and str_split code is not working?
Psycho replied to Michael_Baxter's topic in PHP Coding Help
In addition to what cyberRobot stated: 1. You are trying to split the records based on spaced - but your example shows them being split by line breaks. 2. You are getting the points using the last three characters of a string. If you were getting the player records correctly, this would fail for any point values with 1 digit or more than three digits You should: 1. Split the records based on line breaks. Use the PHP constant PHP_EOL so it woill work on any server (Windows vs. Linux) 2. Get the score based on the characters after the last space (although you should trim each record first) //Get each record based on line breaks $records = explode(PHP_EOL, $_POST['points']); //Trim each array value $records = array_map('trim',$records); //loop thorough each record foreach($records as $record) { //Get position of last space $lastSpace = strrpos($record, ' '); //Get player name and points $player = trim(substr($record, 0, $lastSpace)); $points = trim(substr($record, $lastSpace+1)); //Output for debugging, Do whatever you need with the data // e.g. put into array, run query, etc. echo "Player Name: '{$player}', Points: {$points}<br>\n"; } -
First a tip: Create your logic (i.e. PHP ) at the top of the script and create variables for the output. Then put the presentation (i.e. HTML) at the bottom of the script using just echo's for the applicable output content. For your issue, do a check to see if the value was submitted before trying to access the POST variable. <?php $output = ''; $miles = ''; if(isset($_POST['mile'])) { // How many km is one mile? $onemile = 1.609344; $miles = floatval($_POST['mile']); $totalkm = $miles * $onemile; $output = "<p>$miles miles is $totalkm kilometers.</p>"; } ?> <h3 class="section-heading">Convert Miles to Kilometer II.</h3> <hr class="primary"> <form method="post" action="<?php echo $_SERVER['PHP_SELF']; ?>" style="margin-top: 20px;"> <p>Add Miles: <input type="text" class="form-control" placeholder="miles" name="mile" size="2" value="<?php echo $miles; ?>" /> </p> <p><button type="submit" class="btn btn-primary">Submit</button></p> </form> <?php echo $output; ?>