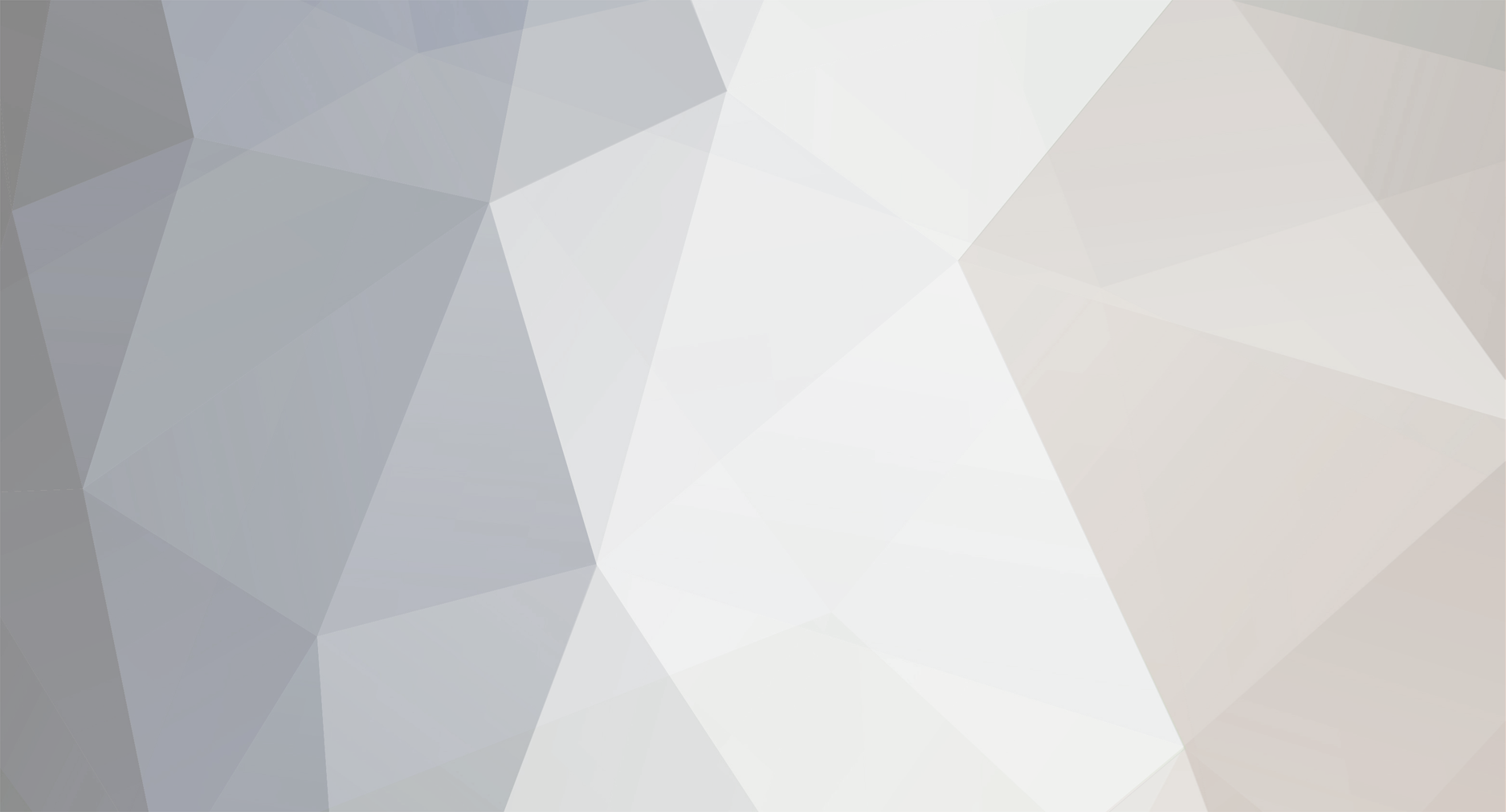
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Mathematical formula to calculate spikes in line graph data?
Psycho replied to D.Rattansingh's topic in PHP Coding Help
Yes, this can be done, but there is no absolute solution. You will need to determine what constitutes a "spike". For example, what if you had the following data: A: 20 B: 95 C: 25 D: 16 E: 88 F: 29 G: 18 H: 91 Would you consider B, E & H as spikes? There are so many of the "high" values that they could be considered normal. There are a few ways I could see achieving this: Determine the average or median, then any point that is X% off from the average/median would be considered a spike. Or, get really geeky and calculate the standard deviation and use that as a measure of determining what a 'spike' is. -
OK, I got that backwards. But, the same statement holds true - you never mentioned this in your previous posts. You can't do that. PHP code is executed on the server. JavaScript code is executed in the browser. They can interact only through client-server communications (e.g. AJAX). I think you are making this way more difficult than it needs to be. I would make your edit button call a JavaScript function that simply opens a web page - adding the ID of the record to the parameter list of the URL for that web page: <button onclick="showForm(15);">Edit</button> //15 is the ID of the record to be edited function showForm(id=false) { var url = 'http://mysite.com/user_form.php'; if(id!==false) { url += '?id=' + id; } window.open(url); } Then build the page user_form.php to check the value of $_GET['id'] when the page loads. If there is no value, then show the form in the "add" scenario. If there is a value, then get the data associated with the record with that ID and populate the form fields accordingly.
-
Regex for letters, hyphens, space, and periods only. (No Numbers)
Psycho replied to AJinNYC's topic in Regex Help
Good thing my last name isn't "O'Reilly" - else you would tell me my last name is invalid. EDIT: when validating if something like this, I find it easier to check for any that are NOT within the acceptable list rather than checking that all are valid. I would use this: function validName($name) { return !preg_match("#[^\p{L}. -]#u", $name); } -
Hmm, I think the error is an indication that the JS file is not being loaded correctly. You are apparently using a custom function (addJSFile) to load your JS files. I don't think that is part of the JQuery framework (at least I can't find it) so I can't say if you are using it correctly or not. Also, No where in your previous posts do you mention or show any code where you have PHP code within JavaScript code. You cannot execute PHP code within JavaScript (you can make a call to PHP code via AJAX). However, you can have PHP code that is used to create/modify JavaScript before it is sent to the user. For example function foo() { if(foo == true) { <?php callPhpFunction(); ?> } } The PHP function would be run at the time the page is requested from the server. Not when the JavaScript function foo() is called by the client.
-
This works for me: test.htm <html> <head> <script src="myscript.js"></script> </head> <body> <button onclick="sayHello();">Click Me</button> </body> </html> myscript.js function sayHello() { alert('Hello'); } Note that the js page needs to be in the same directory as the htm page. If not, the path to the js file will need to be modified in the script tag.
-
How, exactly, are you including the js file in the main page?
-
100% agree with trq. But, to add broader answer. You should never have an "business logic" in JavaScript - or at least you should never rely upon it. You cannot control anything that is done in JavaScript. Since JavaScript is executed client-side, it is a simple matter for someone with a modicum of knowledge to pass malicious data. For example, if you have a form that requires an email address. You absolutely need to do that validation in PHP code. However, it wouldn't be a bad idea to also add some JavaScript code to do that validation to give the user some immediate feedback. Just know that you cannot rely upon the JavaScript validation. Think of the browser as an input method for the user to pass/request information from the server. You can never trust anything coming from the browser. It doesn't matter if it is a form post of an AJAX request. If you have a form with "hidden" fields, a user can manipulate those. If you have a select list in a form, a user can pass any value for that field - not just the ones you put in the list. All business logic must be performed on the server. Only use JavaScript to enhance the user experience.
-
@Requinix, those requirements are for Volume License licenses. For a retail license there are allowances for selling the license: This was from a document for Win Home Basic, but I imagine the Win 7/8 licenses are the same. But, I'd verify the others as well.
-
preg_match_all("#<span style=\"display: inline\"[^>]*>(\d*)#is", $subject, $matches, PREG_OFFSET_CAPTURE); echo "<pre>" . print_r($matches, 1) . "</pre>"; Output: Array ( [0] => Array ( [0] => Array ( [0] => 113 [1] => 0 ) [1] => Array ( [0] => 111 [1] => 68 ) ) [1] => Array ( [0] => Array ( [0] => 113 [1] => 30 ) [1] => Array ( [0] => 111 [1] => 98 ) ) )
-
Well, to start, the database is not set up correctly and you are making it harder than it needs to be. You should not store 'calculated' data such as this. Instead, you create your queries to calculate the data dynamically. In fact, your explanation doesn't make sense to me. If the table is 'games' wouldn't you store the data for each individual game in that table?
-
Except for the fact that it leaves the '<' at the beginning. Not to mention there is a lot of wasted code. There is no reason to do array_pop() to remove the other values from the array since $a2 would be redefined on the next iteration of the loop.
-
$string = "<214.27080, 204.10100, 2500.51300>"; //Convert string into array - splitting on the commas $parts = explode(',', $string); //Iterate over each value in the array foreach($parts as &$part) { //Trim spaces and "<>" from each value and return the rounded down value $part = floor(trim($part, " <>")); } //Convert the parts of the array to a string using '/' as delimiter $newstring = implode('/', $parts);
-
Hmm, my reading of that code is that it would create a folder for every instance in the result set which does not exist. The only thing that is out of place is the elseif() which does absolutely nothing. But, it wouldn't make the code exit the process early. The code shoudl look like this //Query DB $sql = "SELECT DISTINCT tn from tic"; $result = mysql_query($sql) or die(mysql_error()); $loc = "../tickets/"; while ($row = mysql_fetch_assoc($result)) { $dir = $loc . $row['tn']; if(!is_dir($dir)) { mkdir($dir); } } exit(); Have you verified the results of your query to see that it contains what you think it should?
-
Any PHP is executed on the server before the page is sent to the user. If $username or $email are not defined at the time the page is first requested, then there will be no values put into the JavaScript. But, I am confused by what you are trying to do. If the user is already logged in, and you have these values in PHP, then why are you populating the values into JavaScript to then use JavaScript to populate them into the fields. Why not use PHP to directly populate the values into the fields when the page is created. In any event, when trying to debug PHP / JavaScript issues you need to do some simple debugging to see where the problem lies. Otherwise you are just stumbling in the dark. View source on the page to see if the values are even getting populated in the page. If not, then you have a PHP problem and most likely the variables are not getting defined. If the values are in the page, the there is an error in the JavaScript. [EDIT: Good catch Ch0cu3r - I missed that. But, my previous response stands, the logic doesn't make sense]
-
A US phone number has a 3 digit area code and a 7 digit local number. So, you would want to validate that the value contains 10 digits. There are many ways that the value can be formatted: 123.456.7890 (123) 456-7890 123-456-7890 etc. Plus, some 3 digit combinations are not valid as area codes - but I'm not sure what the full list is. I would just strip out everything that is not a number and verify the result is 10 characters long.
-
The syntax looks to be JQuery - which is a JavaScript framework. My guess is that you are not including the JQuery library and the core Javascript interpreter is reading that as syntax errors.
-
You can use Full Text searching - preferred method. This involves more than I would be willing to try and explain in a forum post. there are plenty of tutorials out there you can look up. Or, you can roll your own. Take the input and split it by spaces, then use those values to build the query. $searchString = $_POST['search_string']; $searchWords = array_filter(explode(' ', $searchString)); $WHERE_PARTS = array(); foreach($searchWords as $word) { $WHERE_PARTS[] = "content LIKE '%{$word}%'"; } $query = "SELECT * FROM table_name WHERE " . implode (' AND ', $WHERE_PARTS);
-
<?php $conn = mysql_connect("localhost","root","") or die(mysql_error()); mysql_select_db("regis") or die(mysql_error()); $query = "SELECT * FROM admin_page"; $result = mysql_query($query) or die(mysql_error()); echo "<table width='500' cellspacing='0' cellpadding='0' border='0'>\n"; echo "<tr align='center' colspan='1' rowspan='1'>\n"; echo "<th>p_name</th><th>p_link</th><th>p_content</th>\n" echo "</tr>"; while($row = mysql_fetch_assoc($result)) { echo "<tr>\n"; echo "<td><a href='show_page.php?p_id={$row['p_id']}'>{$row['p_name']}</a></td>\n"; echo "<td>{$row['p_link']}</td>\n"; echo "</tr>\n"; } echo "</table>\n"; echo "<pre>" . print_r($_GET, 1) . "</pre>"; ?>
-
I think a maximum of 20 is way too short. A longer length will allow pass phrases such as "I live in a green house" which add much more complexity yet are easier to remember.
-
Kind of hard for us to provide any real help without knowing where the first name and last name are defined. All I see is a variable called $name. Are you concatenating the first and last name fields before doing the validation? Also, I would ask why you care what data is in the name fields? Do you realize that you would potentially restrict valid names? For example, what about letters with accents such as "Muñoz". It gets even more complicated when dealing with character sets that are not Latin based. Now, there could be valid reasons to limit the valid characters in the name fields. But, if you don't have a valid business reason for doing so, it is not worth the effort - in my opinion.
- 2 replies
-
- string
- form validation
-
(and 2 more)
Tagged with:
-
Off topic: If you find yourself writing what appears to be repetitive code - such as in your series of ifelse() statements to define $p2 - stop - you're doing it wrong. You can replace all of that code with the code below. I didn't test it thoroughly, but I think it's correct. $p2List = array( "-9", "-8", "-7", "-6", "-5", "-4", "-3", "-2", "-1", "base1", "base2", "1", "2", "3", "4", "5", "6", "7", "8", "9" ); $p2ListCount = count($p2List); if($placeCount<2 || $placeCount>$p2ListCount) { die("Invalid number of characters: {$placeCount}!"); } $startIndex = ceil(($p2ListCount-$placeCount) / 2); $p2 = array_slice($p2List, $startIndex, $placeCount);
-
<?php function subarray_search($searchArray, $searchParam) { $searchKey = key($searchParam); $searchValue = current($searchParam); foreach($searchArray as $index => $subArray) { if(isset($subArray[$searchKey]) && $subArray[$searchKey]=$searchValue) { return $index; } } return false; } $findErrorStatus = subarray_search($myArray, array('status'=>'error')) if($findErrorStatus !== false) { echo "A status is in error"; } else { echo "None of the statses are in error"; } ?>
-
No It's not (at least not with a single built-in function) If you are going to go to the trouble of building something, might as well build it to be extensible.
-
@Requinix, I think his problem is he doesn't know how to deal with the exponential returned value @Cascadia11, the value you state is being returned is the wrong value. You're getting back .000005 - which makes no sense. Once you are getting the right value, try using this to format it: http://php.net/manual/en/function.number-format.php