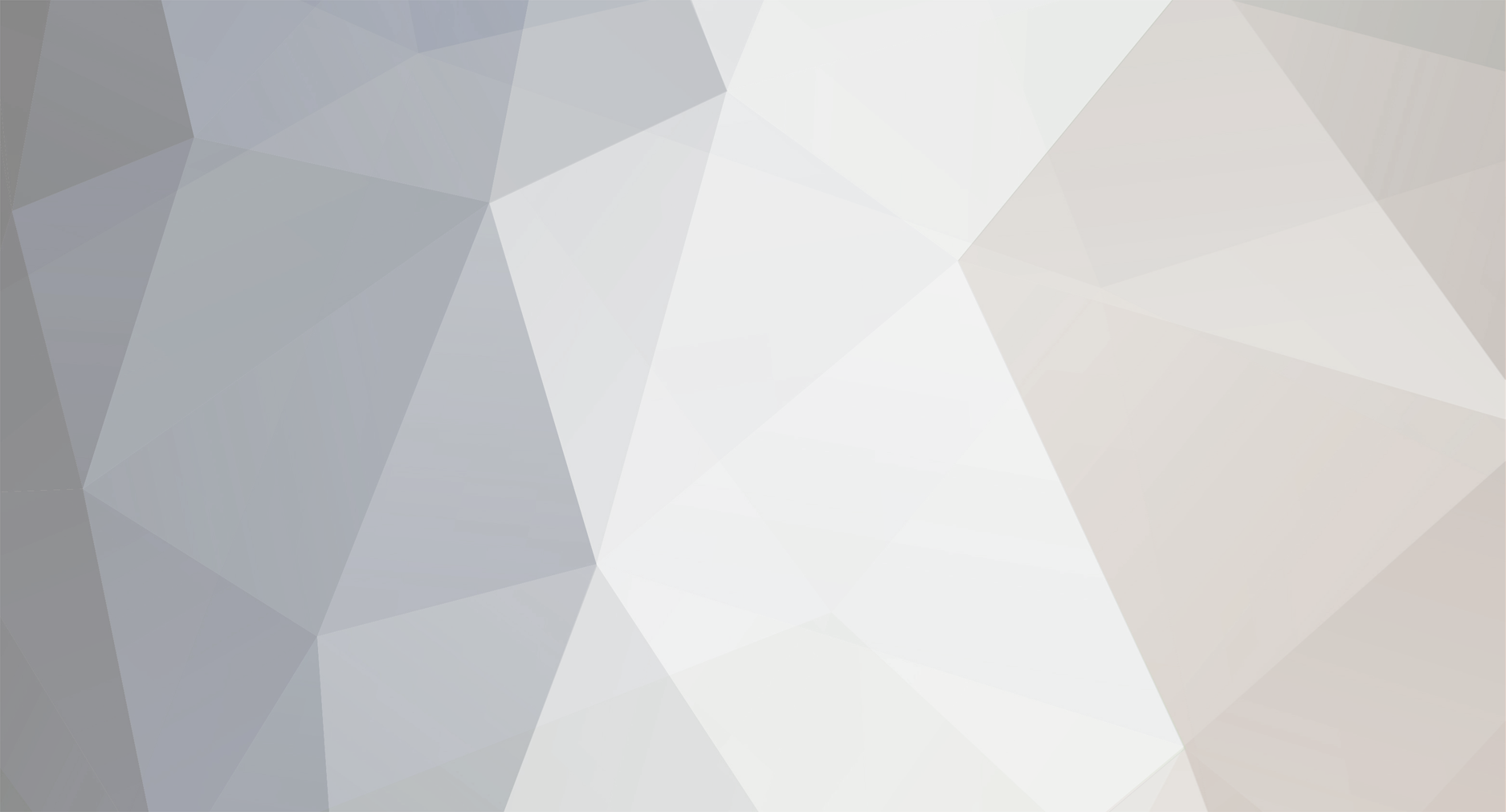
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
passing values from one php form to another and incrementing
KevinM1 replied to bumdeal2's topic in PHP Coding Help
For a table id, the best way to do it is to set the column to unsigned int auto-increment not null. This has the column start at 1, and automatically increment on each insert. -
What are $logged_in, $lang_string, and $DL_config, and where do they come from? Aside: this is a prime example of why the 'global' keyword is bad.
-
IMO, the idea of having a paid section goes against the spirit of a place like this. There shouldn't be tiers of users, and if you need a solution badly enough to pay for one, we do have a freelance sub-forum.
-
Try: echo $_GET['page'];
-
Thanks. My SQL skills, in a word, suck. Definitely my weakest area.
-
I have a many-to-many relationship in a game review site I'm working on. A review can be for a game that is available on several platforms (example: the Grand Theft Auto games), and each platform will have many reviews, one for each game reviewed on that platform. So, to represent this relationship, I have a pivot table that holds the id for both reviews and platforms. In other words, my setup is: reviews: int id, varchar title, text content, etc. platforms: int id, varchar name platforms_reviews int id, int platform_id, int review_id To figure out what platforms a particular game review is for, I have the following query: SELECT name FROM platforms WHERE id IN (SELECT platform_id FROM platforms_reviews WHERE review_id = {$review['id']}) I'm just wondering if there's a better way to do it, perhaps with a JOIN.
-
Doesn't a CSS reset mitigate most/all cross-browser rendering issues?
-
Even simpler, you can put your script at the very bottom of your markup: <!DOCTYPE html> <html> <head></head> <body> <div id="test"></div> </body> <script type="text/javascript"> var test = document.getElementById('test'); if (test) { alert('check'); } else { alert('error'); } </script> </html>
-
For displaying the db data, yeah, you'd have to do it manually. It's actually not that difficult. There's generally two areas of complexity - writing the right query and getting the results to display correctly. The first is dependent on your db structure. I can't really say more than that without seeing it. The second usually takes the form of a while-loop. Each iteration prints one row of data from the results. The issue here is related to markup - can you correctly print the results so they fit in with the rest of your layout? It sounds simple, but a lot of beginners have problems with seeing the connection between looping through data manually and displaying it just right.
-
Don't send them to me personally. Post them here in code tags.
-
Ugh.
-
It adds an escape character ('\') during data insertion/updating, but it shouldn't be visible during data retrieval. If you do see an escape character during data retrieval, chances are you have magic quotes on, which is considered a no-no (if memory serves, it should be turned off by default in PHP5, and may be completely removed from the language when version 6 is finally released). So if I type in "what/ever" it returns "what\/ever" ? Because I'm trying to implement it in my password, but the point is that my passwords get md5()'ed. And they get md5()'ed before they enter the mysql database. So should I: 1) md5() the "\" with the password, so nevertheless my passwords containing slashes work 2) leave it like this, because md5() always returns Letters and numbers... You're over thinking it. The escape character doesn't remain in the db. They're used only when the insertion/update happens, to make the query safe, and are then for all intents and purposes thrown away. For a password, something like: $pass = md5(mysql_real_escape_string($_POST['password'])); Would be fine for insertion. And when obtaining user data: $user = mysql_real_escape_string($_POST['username']); $pass = md5(mysql_real_escape_string($_POST['password'])); $query = "SELECT * FROM users WHERE user_name = $user AND password = $pass"; Should suffice. It's just like when you need to escape HTML output. For example: echo "<a href=\"http://www.google.com/\">Google</a>"; The escape characters marking the value of the href attribute aren't visible in the HTML that's generated. The same thing applies with the database - you won't see the escape characters if you attempt to retrieve data.
-
It adds an escape character ('\') during data insertion/updating, but it shouldn't be visible during data retrieval. If you do see an escape character during data retrieval, chances are you have magic quotes on, which is considered a no-no (if memory serves, it should be turned off by default in PHP5, and may be completely removed from the language when version 6 is finally released).
-
Malicious code can be inserted in image files. Be sure that things like image.php.gif and the like can't get through. Also, strip tags doesn't do anything to protect your database. Don't know where you got that idea. It's useful in ensuring that user submitted data (like a blog comment or forum post) doesn't contain malicious code, but that's it. Again, always validate and escape user input, even if it's just you logging in. This means: 1. Checking to make sure that user submitted input is well formed. A field that requires an integer should only contain an integer. A field for an e-mail address should only accept a legit address. RegEx and other checks (is_integer, for example) should be used here. 2. Escaping all data that will interact with your db. This will prevent injection attacks. And, no, addslashes won't cut it. You need to use the escape function that's related to the db you're using (like, say, mysql_real_escape_string). This is internet security 101. If you don't learn it now, your bigger projects will inevitably suffer.
-
You might want to give us some examples.
-
I don't think you can nest forms.
-
Not really. You're 'safe' right now as, presumably, you're not using your $_GET data to access a database, or anything critical (file upload page). If your site grows, you should definitely consider changing navigation strategies. The risk of SQL injection or a user with bad intent gaining access to a critical area of your site is too great not to. Ideally, for something this small, you'd simply have a PHP page for each main component of your site. So, one for your index, one for displaying the photos, etc. From there, you could use $_GET to retrieve the correct batch of data (even better to use $_POST, as at least the address bar couldn't be used as an avenue of attack), but only after validating it. You never, ever, ever, ever trust user input, regardless of whether or not it comes from $_GET, $_POST, $_COOKIE, or the catch-all $_REQUEST. Code defensively. Validate all input, even if you think it's safe.
-
First, I wouldn't suggest storing the photos in the db. Instead, store them in a folder(s), and have the db simply hold the paths to the photos instead. It'll make your life far easier. Second, who will be able to obtain these images? Anyone? Only registered users? Will there be a limit on how many can be 'bought' at any one time? The answers to these (and other) questions will inform your design. I'm not sure about a zipcode lookup script/db set up. I've never worked on a project that required something like that. I'm sure a Google search would point you in the right direction. I'm also not sure what you mean by 'Is their a simplier way to do this in DW that I am just not getting?' PHP is simply code. It can be written with any text editing program. I actually use Notepad++ for my coding needs. So, it's not a Dreamweaver issue, rather it's a lack of experience issue. Don't feel bad, we've all been there. Given your lack of experience with PHP, you should at least research what carts are available to you. While it's not necessary to have one for this (in fact, something like this isn't all that difficult to do, if you know how), if you're looking for fast results, it may be your best bet.
-
When I was 3-4, waaaaayyy back in the early 80's, my family had a Commodore VIC-20. It was essentially a computer in a keyboard. It was designed to plug into a TV, so we used it mostly to play games in the living room (yay Gorf!). When there wasn't a cartridge plugged into it, it was essentially a BASIC interpreter, so my brothers and I used to write some small, simple programs on it. I didn't get into programming for real until I went to college. I was a computer science major, learning C/C++. I did alright until I got to the assembly language course. I just have a hard time visualizing how to handle low level, binary stuff. Unfortunately for me, that was a required course, so I failed out of the program. I graduated with an essentially worthless Bachelor's in communication. Yay. At around the same time, one of my friends, who not coincidentally also failed out of CS, was making some money doing LAMP work for clients. I was intrigued, and started teaching myself the same skill set. And, there you go.
-
Addslashes doesn't escape everything like mysql_real_escape_string does. Any time you want to escape data, you should use the escape function related to the kind of database your using, or prepared statements, which automatically escape data inserted into a query (not available with MySQL, instead use the MySQLi extension. See: mysqli
-
Instead of addslashes, I'd use mysql_real_escape_string.
-
Could be a DRM issue. I had the same problem with Silent Hunter 3.
-
I didn't even see this line That's okay... I still have the injected linebreak there.
-
You can also accomplish this by using CSS. Give each div a class so they all have the same width and add float: left; If the total size of your divs is greater than it's containing div then it will be automatically be pushed to the next line. That's true. I tend to add the linebreak just for completeness' sake.
-
Can you elaborate on how I can pass it to the constructor? class Email { private $db; public function __construct($db) { $this->db = $db; } } $mysqli = new mysqli(/* connection string */); $email = new Email($mysqli);