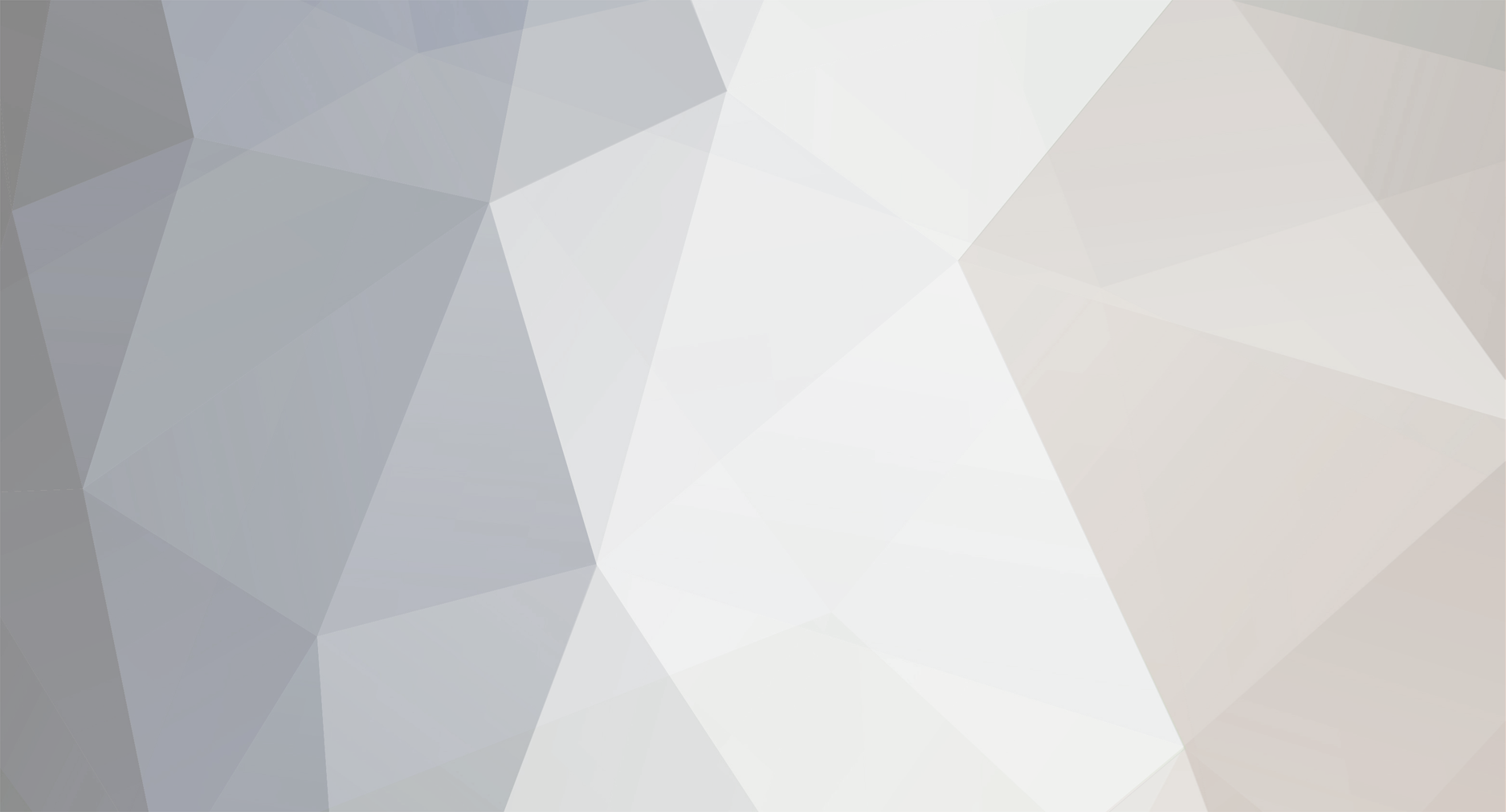
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
Some good JavaScript resources: https://developer.mozilla.org/en/JavaScript http://docs.jquery.com/Main_Page http://www.amazon.com/Pro-JavaScript-Techniques-John-Resig/dp/1590597273/ref=sr_1_2?s=books&ie=UTF8&qid=1301399245&sr=1-2
-
Don't use w3schools. Look at the link in my signature for why. You'll need to have some familiarity and comfort with a database. The one most people use is MySQL. You should learn OOP if you're going to be working on projects of moderate or larger sizes. Learn the MVC pattern, and familiarize yourself with the popular frameworks which adhere to the pattern - CodeIgniter, Zend, and/or Kohana. Get yourself quality resources. w3schools is passable as a quick refresher for syntax, but no one should use them as their primary learning source. Good books are worth their weight in gold. Above all else, remember that the syntax is only part of the equation, and not even the most important part. If you work on becoming a good programmer, the language(s) you use will be a secondary concern
-
You're missing the point thorpe is making. Your form DISPLAY script needn't be the same file as your form PROCESSING script. The processing script doesn't need to display any output and would be able to redirect the user. The user would simply see your forms, then be taken to where ever you want them to go after form submission.
-
Functions don't map to classes in a 1-to-1 manner. This is especially true with constructors, which are special and are supposed to be used in a particular way. Slapping functions in a class in the way that you are IS NOT OOP, and really isn't teaching you anything beyond some basic syntax. You're not gaining anything from a true methodology standpoint. You'd be much better off buying a good book on the subject and learning it the right way from the start.
-
A couple things: Since you're using the function during the submit event, you need it to return false if the error pops up. Otherwise, form submission will continue regardless of the error. Second, tie the function to the onkeyup event. Why? onkeydown fires when the button is pressed. onkeyup fires when the button is released. Your input isn't empty until after backspace is pressed. Finally, use unobtrusive JavaScript. There's no reason to have ANY JavaScript code written inline within your elements, especially if you're using jQuery. Try the following (be sure to read the two JavaScript comments I wrote): <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8" /> <link type="text/css" rel="stylesheet" href="css/style.css" /> <script type="text/javascript" src="//ajax.googleapis.com/ajax/libs/jquery/1.5/jquery.min.js"></script> <title>JQUERY</title> <style type="text/css"> .box, button { margin:5px 10px 5px 0; } p { line-height: 10px; } body { line-height: 15px; } .box { color: #FF0000; font-size: 90%; font-family: Verdana, Arial, Helvetica, sans-serif; background-color: #FFD9DA; width: 250px; border-top-right-radius: 10px; border-bottom-right-radius: 10px; padding: 10px; line-height: 15px; } .box2 { color: #00CC00; font-size: 90%; font-family: Verdana, Arial, Helvetica, sans-serif; background-color: #CEFFDB; width: 250px; padding: 10px; border-top-right-radius: 10px; border-bottom-right-radius: 10px; } </style> </head> <body> <form id="form" method="post" action="?hello=man"> <p>Name: <input type="text" id="btn1" value="" size="50" /></p> <p><div id="box1" class="box"><img src="images/icons/x.png" alt="" width="17" height="17" style="float: left; margin-right: 5px;"/>You did not enter a name!</div></p> </form> <script type="text/javascript"> $("#form").submit(check_name); // you may need to put quotes around check_name. DO NOT put parentheses at the end $("#btn1").keyup(check_name); // ditto function check_name() { if ($("#btn1").length == 0) { $("#box1").fadeIn(500); return false; } else { $("#box1").fadeOut(500); return true; } } </script> </body> </html>
-
For the correctness of terminology, HTML pages aren't a program. Neither HTML nor CSS contain any logic. Instead, they're simply documents with special formatting instructions that a browser can read and use. PHP apps are generally called scripts because they're not usually compiled. They're interpreted by the PHP engine which is running on the web server. This should help you explain your problem a bit better. Also, you may want to tell us your directory structure as changing your JS path seems to have done something.
-
At the most basic level, yes. Most frameworks are more than just a series of helper functions, however. Many form the underpinning of web applications, allowing the coder to focus on the custom work that lies on top of the framework.
-
If you're just using PHP out of the box, you're not using a framework.
-
You're going about this the wrong way. Classes can't be split up like you want them to be. If you feel your class is flawed due to its length, you should look into refactoring it into other, smaller classes. In any event, you're also trying to reinvent the wheel when PHP already has several OOP database options available to use - MySQLi and PDO. Look into them to see if they'd fit your needs.
-
You have to store both the salt and the hashed salted password in the db. Why? Because when someone tries to log on, you'll need to test that password value with the pre-existing salt. So, using pseudo-code: // user enters in name and password combo $name = $_POST['name']; $pass = $_POST['password']; $query = mysql_query("SELECT password, salt FROM users WHERE username = $name"); if (mysql_num_rows($query) === 1) { $row = mysql_fetch_assoc($query); $savedPass = $row['password']; $salt = $row['salt']; if (sha1($pass . $salt) === $savedPass) // REMEMBER: $pass is the attempted password... { // valid user } else { // invalid user - figure out what to do } } else { // bad user name } Using the current time as a salt when a user registers is the way to go because the value will always be unique, and it will always be unique for that user. Make sure you do your research on how many characters the hashing algorithm you choose will generate. The more characters, the better, and you don't want to harm your own security by choosing the wrong column type. A lot of them generate more than 32 characters. EDIT: For password/salt creation, you simply need two columns - one varchar column, the length of whatever hashing algorithm you choose, and one timestamp column for the salt. When you register the user, simply use: $salt = time(); $saltedPass = sha1($pass . $salt); // insert both into the db for that user I use SHA1 as, last I read, it's better than MD5. I should brush up on that, though....
-
What are you doing when you view the HTML? Simply selecting 'View Source'? Or doing something else? Browser tools may show implied/inherited styles, depending on which you may be using. You shouldn't be having inline styles added to your actual code. I've used jQuery many times without that sort of thing happening, but have never used the cookie plugin you have, so I can't vouch for it. Can you do a simple jQuery-only test to see if it's the plugin causing the problem, if there is indeed a problem? And, have you tested your full code with other browsers?
-
The mysql_fetch functions move a read-only marker through the result set. Since you only have one result, the line of $row = mysql_fetch_array($result) which is outside the while-loop is grabbing your only result. The function call within the loop conditional can't grab anything, so the loop doesn't even execute. So, in short, remove the first $row = mysql_fetch_array($result) line.
-
Can you give a code example of a Service?
-
Well, first, if you're using PHP 5 you don't need to pass the User instance by reference. Objects are automatically passed by reference. But, yeah, your design is messed up. Your Email class shouldn't require a User to work, right? Logically, email is a utility service. It should be able to be used throughout your system. This is one of the reasons why I suggested turning it into a static class (all methods marked as static) in your previous thread on this subject. If you're determined to use composition, it makes more sense for the User to contain the Email. Why? Because it's the User which depends on the Email functionality being present. Email is a component (hence composition) of the User.
-
It was spam, which is against this site's rules
-
You may be able to break the timestamp down into its component parts, and then order the returning data according to those parts. There's a whole bunch of date/time related functions you can use: http://dev.mysql.com/doc/refman/5.0/en/date-and-time-functions.html I'm just unsure if they're all compatible with a timestamp.
-
What does your db table look like? Also, please place your code in tags.
-
Why do you have a meta redirect? Also, aside from the font (which, like cssfreakie says, doesn't fit what you're trying to do), you didn't really follow any of the advice I gave you last time: http://www.phpfreaks.com/forums/index.php?topic=325795.0 You really, really need to do some design homework on this. Look at bookstore and auction sites. Look at their commonalities: hardly any kind of introductory spiel, clear product descriptions, product categories, user rankings, etc. They look bright, clean, and organized. There's no reason why you couldn't do something similar, albeit on a smaller scale. The premise is sound, you just really need to put in the work to see it through. As a start, you should look at the common structural elements the other sites share. Look at how they do their navigation, their headers and footers. Pay close attention to how their products are displayed. I'd stop trying to force the young/cool/different/flippant vibe. It's not working, and it comes across as cheesy and out of place. Look at brands that actually are popular with your peers. Hell, Apple is insanely popular in your target demographic and their design aesthetic is light gray buttons with aqua highlights on a white background with clean sans-serif fonts. Here's what I'd do - white background with black text. A third, vibrant color for contrast and flair. Red, some shade of blue, orange, sage-to-dark green, maybe even violet. Sans-serif fonts. Cleaner book listings - Name, author, ISBN#, course name, department, book condition. Make this data related and searchable. Get rid of the meta refresh, shorten the opening spiel to a paragraph. Put some book listings and a search bar on the main page a la Amazon and the others. In short, get rid of the gloomy, wannabe rocker vibe and make it a clear, orderly, comfortable virtual book exchange. I'm not expecting a Fortune 500 result, but with some time and effort you can really clean things up and make a site that will entice people to use your service rather than the opposite.
-
@Destramic: Can you show us actual code? @cssfreakie: JavaScript can manipulate the DOM in its entirety - CRUD for both styles and elements.
-
I have FF4, and have not encountered the error. I just checked GMail as a test, and no problems. You may wanna reinstall if it persists.
-
D'oh, wrong thread
-
With OOP, you have to realize that inheritance is: 1. Inflexible 2. Creates is-a relationships When you extend a base class, you're saying "An object of the child class is a object of the base class, too." Your User should not be an email object. This kind of situation is why OOP stresses interfaces and composition. It's easier to say "An object of class X also implements/fulfills interface I" or, "An object of class X contains an object of class Y as a member." I'd simply turn your email class static (an email class is unlikely to retain state, so you're most likely not going to need an object) and use it directly. class Email { public static function UserMail(User $user) { // send user mail } } class User extends Base { public function EmailDetails() { Email::UserMail($this); } } It should work seamlessly if you have your autoload setup correctly. You should really learn some of the theory of OOP before you continue much further. Inheritance is a nice tool to have at times, but it's definitely not the only one, nor is it necessary a lot of the time. There are other, often better ways for objects and classes to interact with each other. Get Matt Zandstra's book on PHP OOP and the Gang of Four's book on the subject. They will fill in a lot of the gaps.
-
First application, question about design process
KevinM1 replied to kdubbie's topic in Application Design
Here's how I normally roll: 1. Figure out and design the domain. 'Domain' is what a site does. In your case, your user information form and admin functionality. I construct entities based on how the information needs to be handled, and map the relationships between them. These are my models, and any logic that isn't tied into site control or display goes in here. There are constraints here - a user shouldn't be able to do whatever they want with all the data. The models are designed to only accept functionality that falls in line with the purpose of the site. 2. I flip it around and plan/design the front end. Mockups, wireframes, etc. I try to figure out the best way for a user to view/manipulate the data on the site. 3. I make changes to both the domain model and front end mockups until they both mesh and do their collective jobs efficiently 4. Once my domain model is complete, I map it to a database. 5. I work on the site-specific code - navigation between pages, filling out templates/views, etc. 6. Release. Keep in mind, testing goes on during each phase, as does validation and security when/where needed. Websites are layered, so it makes sense that a lot of the things that don't fit into one particular step happen multiple times where needed. As far as general security, look into SQL injections, session hijacking, cross-site scripting (XSS), and cross-site request forgery. For your hosting, be sure that your files and folders have the proper permissions, and that your FTP has a strong password. P1zz@1! or P@ssW0rd doesn't cut it. Finally, there's no 100% set way on how to write an app. Most developers tend to start with the back end/business logic parts because content is the most important part of a site. A site can be well designed, or even downright pretty to look at, but the visual appeal doesn't have much of a lifespan. Repeat visitors are due to content. This isn't to say the front end isn't important - far from it. A well-designed site facilitates the consumption of content. A bad design is sure to drive visitors away. There's a reason why companies spend millions on branding, design, navigation, mobile presence, etc. But, at the end of the day, content is why people keep coming back.