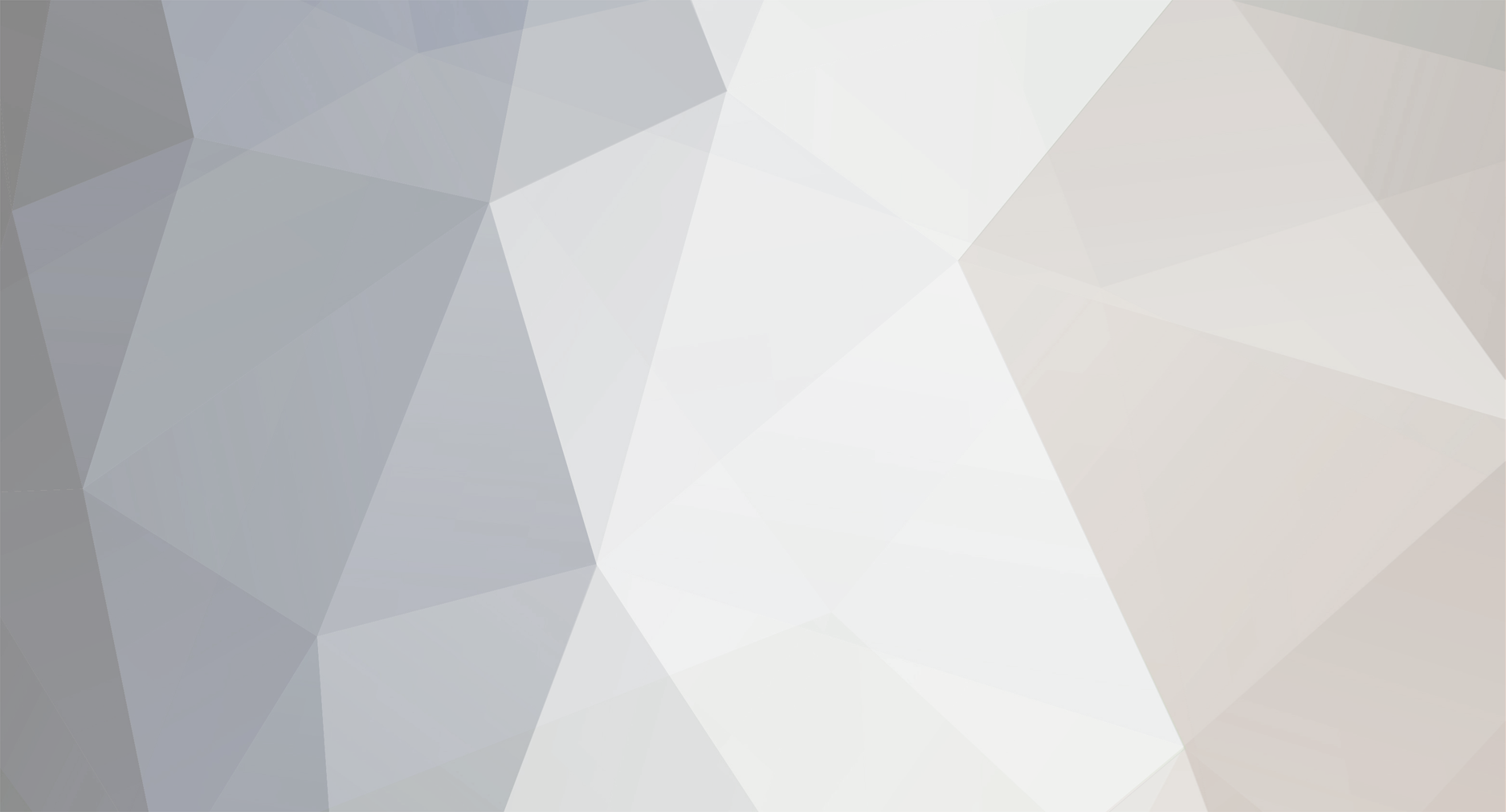
shlumph
Members-
Posts
575 -
Joined
-
Last visited
Everything posted by shlumph
-
Setting A Variable, Ternary Operator Vs. Error Control Operator
shlumph replied to jmartinsen's topic in Application Design
Also, from a readability standpoint, the @-operator makes me stop and think a little bit longer when debugging/skimming code. "Wait, what happens when..."- 6 replies
-
- ternary operator
- error control operator
-
(and 1 more)
Tagged with:
-
Keeping the active record approach it seems you're looking for, you could do something like this: <?php class User { private $_fields = array( 'id' => null, 'username' => null, 'email' => null //etc. ); public function setFields(array $data) { //Set data in $_fields array } public function save() { //save } //Other crud methods }
-
The only difference I see between the //For User block and the //For computer block is $com. So, to start, you can wrap this block into it's own function and call it twice. After that, I'd look at simplifying this new function.
-
In your userLogin() function, you can set the $userid field from whatever is returned in that row if $success is true. Edit: inline code tags.
-
Terminator, The Girl W/ The Dragon Tattoo, War Games, Live Free or Die Hard all have programmer related stuff in them but aren't necessarily themed about programmers. Except maybe War Games
-
Alright, you said it's going into the MP3 folder, right? So all you need to do is include the category folder in the path. $destination = 'mp3/' . $cat . '/' . $filename . '-' . $_SESSION['user_id'] . '.mp3'; You might want to make sure the directory exists, and if it doesn't, create it: if(!is_dir(dirname($destination))) { mkdir($destination, 0777, true); //Change 0777 to whatever permission you're comfortable with } move_uploaded_file($tmp, $destination);
-
That's not good! Lol. I'd look into the logout script. Make sure the identity is cleared via Zend_Auth::getInstance()->clearIdentity() and even destroy the session as well if it's necessary; Zend_Session::destroy() Depends on the storage set for Zend_Auth, the default is Zend_Auth_Storage_Session.
-
You'll have to specify the category as part of the path name when moving the uploaded file. How do you think this line should be modified? move_uploaded_file($tmp, 'mp3/' . $filename . '-' . $_SESSION['user_id'] . '.mp3');
-
That looks like valid syntax to me. Are your variables set as what they are supposed to be? If so, perhaps your problem is related to something else.
-
Another reason to have multiple database is to set up a master/slave environment, where you do reads from the slave(s) and writes to the master. The slave(s) then get updated from the master every so often. Whether or not you'll get a performance boost depends on your application, if it's more write intensive, more read intensive, etc.
- 7 replies
-
- database
- phpmyadmin
-
(and 2 more)
Tagged with:
-
There are times when you hit performance limits due to transaction volume, and would like to partition or shard your database. Search database sharding for more info, there's plenty out there.
- 7 replies
-
- database
- phpmyadmin
-
(and 2 more)
Tagged with:
-
If the storage engine supports transactions, I would recommend starting one at the beginning of your script, committing at the end and rolling back if there's any errors. This should make the script perform faster and gracefully make sure that all or nothing goes through.
-
How To Share A Logged In Session Between To Web App?
shlumph replied to silvercover's topic in PHP Coding Help
Are they on the same server? If they are, and they are under different subdomains or domains, you'll have to set the session cookie domain to be the same in both projects. If they are on different servers with different IP addresses, you'll probably have to create some sort of web service to interface the two projects. -
Don't forget to clear the $_SESSION variable(s) out after you're done needing them.
-
1) Variables stored in Zend Registry are not persistent throughout HTTP requests. They are not stored in $_SESSION or cookies, it's more of a singletonish pattern. 2) You authenticate the user with Zend_Auth. There's different storage mechanisms, but the default is using $_SESSION or Zend_Auth_Storage_Session. If you don't know if the user has an identity yet, you should probably be using Zend_Auth::getInstance()->hasIdentity() before trying to get the actual user identity.
-
There's more than one way to skin this cat: htmlspecialchars(), strip_tags(), htmlentities(), etc. would all do the job. Some people like to do it before inserting into DB, other like to do it while printing the content out. http://www.php.net/strip_tags http://www.php.net/htmlspecialchars http://www.php.net/htmlentities
-
That's because the function has no idea where $CategoriesMajor came from.
-
And might as well use a framework to make it easier for you; jQuery or Dojo Toolkit are a couple options.
-
The Singleton pattern is considered an anti-pattern now by most programmers. Static creation makes it difficult to test and, if suddenly the class doesn't need to be a singleton anymore, requires lots of changes. Dependency injection is the way to go instead. Leaving the object creation strategy to a Dependency injection container makes it easy to inject mock version of the dependencies and test classes under isolation. And with DI, you can still maintain a single instance of the class.
-
From what I'm understanding, I think you want this: <?php session_start(); // start session $_SESSION['people'] = $_POST['people']; //post number of tanks from form ?> And then adjust your select box, by getting rid of if ($i==$totalpeople)
-
Where/when do you set $_SESSION['people']?
-
If you're a talented web designer and not only learn some programming (any web language), but learn how to program well, it will be beneficial to you. I think that's an obvious fact. Most web designers come across clients that need some sort of custom programming. In that case, they have to budget some of their income to hire a programmer. The thing is, most people aren't efficient in both web design and programming. I could spend 8 hours trying to make a logo. And it would look like crap. I could also spend $150 and have a talented graphic designer make one in two hours. For me, it's more wise to hire the graphic designer because I make more then $150 in 8 hours. Not sure if I'm going off in the wrong direction, but hopefully this will give you some insight. In the end, it's up to you and how efficient you could become at both.
-
Zend Guard is one option: http://www.zend.com/en/products/guard/
-
Are you saying that by the time your page loads, some of the data on the page no longer exists?
-
You probably want a left join: SELECT pet.active, pet_id, activation_code, pet.user_id, pet_name, pet_type, status, DATE_FORMAT(pet.registration_date, '%M %d, %Y') AS rd FROM pet LEFT JOIN users ON pet.user_id = users.user_id WHERE activation_code LIKE '%%' && pet_id LIKE '%%' && users.email LIKE '%%' ORDER BY pet.pet_id DESC LIMIT 700, 100