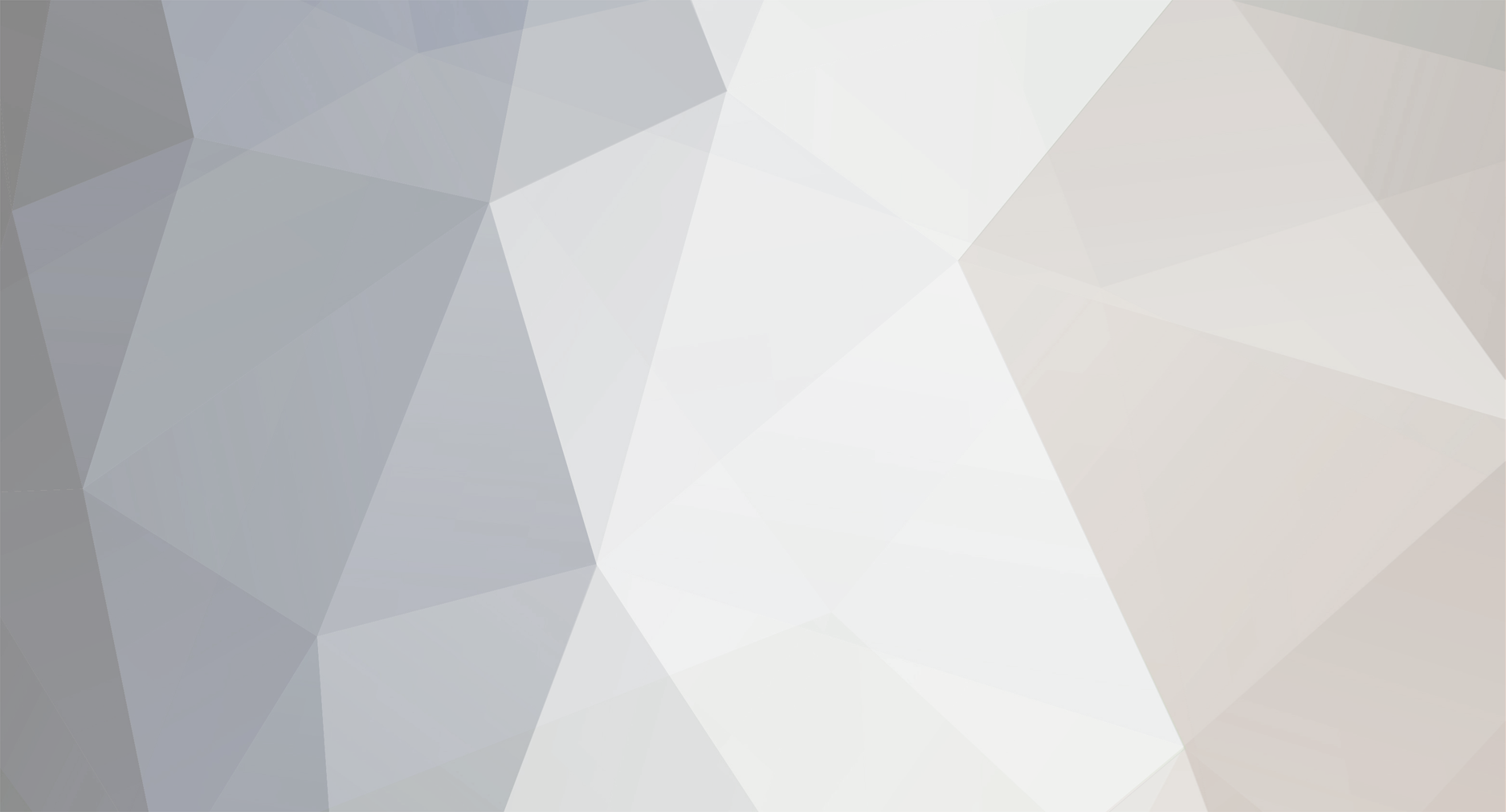
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
You have to keep track of the last one printed: $lastUser = null; while (($data = fgetcsv($handle, 1000, ",")) !== FALSE) { if ($data[0] != $lastUser) { echo "User Name: $data[0]"; $lastUser = $data[0]; } echo "Booking IDs: $data[1]"; } echo "<hr>";Of course, this would depend on the user records being grouped together in the input. If they are not, you could load all of the data into a multi-dimensional array.
-
If you want it in an Unordered list (which is not really a table when you are talking to HTML and SQL programmers), you want it to look something like this: <UL> <LI>Company <UL> <LI>Spec 1</LI> <LI>Spec 2</LI> </UL> </LI> <LI>Company <UL> <LI>Spec 1</LI> <LI>Spec 2</LI> </UL> </LI> </UL> The PHP to generate that is something like this (untested): $lastCompany = ''; echo '<UL>'; # Start Main List - Item per Company while($row = mysql_fetch_array($companyresult)) { if ($row['company'] != $lastCompany) { if (! empty($lastCompany)) { echo '</UL></LI>'; # Close the previous Company List } $lastCompany = $row['company']; sprintf('<LI>%s<UL>', $lastCompany); # Open a new Company list } printf('<LI>%s</LI>', $row['spec1']); } if (! empty($lastCompany)) echo '</UL>' # Close the last Company List echo '</UL>'; # Close the Main List
-
The first example from the manual for move_uploaded_file $uploads_dir = '/uploads'; foreach ($_FILES["pictures"]["error"] as $key => $error) { if ($error == UPLOAD_ERR_OK) { $tmp_name = $_FILES["pictures"]["tmp_name"][$key]; $name = $_FILES["pictures"]["name"][$key]; move_uploaded_file($tmp_name, "$uploads_dir/$name"); } } Look at line # 4. You need to use the key tmp_name not name as the source (the file to be moved).
-
How to Avoid Postback on JQuery Button click in PHP
DavidAM replied to fanboime's topic in Javascript Help
PHP is executed on the SERVER before the page gets to the user. It is not possible to execute PHP code directly from JavaScript. You would have to use AJAX to send a request to the server to execute some PHP code. -
I noticed a function show_error() in your source file. It would seem to me, you just need to call this function in the appropriate places. It will output the appropriate error message, if one exists for the specified field: function print_the_form($errors = array(), $fields = null) // Display the form { ?> <form class="contact-form" action="#contact-form" method="post" id="contact-form"> <div class="input-item"><label>NAME</label><input type="text" name="name" value="<?php inpt_value('name', $fields); ?>" id="inpt-name"></div> <?php show_error('name', $errors);?> <div class="input-item"><label>EMAIL</label><input type="email" name="email" value="<?php inpt_value('email', $fields); ?>" id="inpt-email"></div> #MAD: Show the email error here <div class="textarea-item"><label>MESSAGE</label> <textarea name="message" id="txtarea"><?php inpt_value('message', $fields); ?></textarea></div> #MAD: Show the message error here <input type="hidden" value="1" name="submit" /> <div class="send-button"><button type="submit">SEND</button></div> </form> <!-- = /contact form = --> <?php } In the code above, I put it immediately after the closing DIV tag for each item. I'm not sure if it belongs there or INSIDE the DIV - The significance (as far as I can tell) would be in how the CSS is written for this form.
-
Why? and How secure does this need to be? Why would you need to encrypt the data in the database? Just have the application NOT display it. If you have users with direct access to the database you can GRANT or REVOKE access to specific TABLES and COLUMNS to prevent the users from seeing data. If you are talking about storing credit card data, and you don't know anything about encryption, I would suggest you stop RIGHT NOW. Security is a serious, complex thing. You will need to learn about various encryption algorithyms and salts and I don't know what all. Personally, I would not attempt it without hiring an expert in the field (and buying a big fat Liability Insurance policy).
-
Let's try this again. Your original post said: You have cutoff the error message. PHP error messages give the name of the file and the line number in that file where the error occurred. Sometimes, the actual problem is a couple of lines BEFORE the line number given. This error message "... boolean given ..." indicates that the parameter passed to the mysql_fetch_array function is the (boolean) value FALSE. It is expected to be a query resource returned by mysql_query. So, the problem is a couple of lines BEFORE there error. Read the error message, find the file and line number in the error message. Post code from that file starting 5 or 10 lines BEFORE the line number in the error message, and continuing through 5 or 10 lines AFTER the error message. SURROUND THE CODE WITH TAGS. ALSO Post the FULL ERROR MESSAGE (you can X-out the path if you feel it is a security risk). If we need to see more code, we will ask. When we provide a suggestion, and it does not work; post the NEW CODE as you tried it, and tell us what EXACTLY happened. If you get ANY error messages, POST THE FULL TEXT OF THE MESSAGE. We are not sitting there looking at your screen, we have NO IDEA what is happening, so you must provide us with as much pertinent detail as possible. mac_gyver is correct. The code you posted can NOT produce the "query is emtpy" message. So either you posted the wrong code, or you put the mysql_error call in the wrong place, or something. Again, you did not post the NEW CODE so we can't tell what is happening.
-
You have passed the $errors array to the print_the_form function; but you never output the error messages anywhere in that function.
-
There is a slight problem in the index.php print <<<HERE <tr> <td> <form method="POST" action="updateform.php"> <input type="hidden" name="sel_record" value="$id"> <input type="submit" name="update" value=" Edit " </form> </td> <td><strong> Description: </strong>$descr,<p> <strong>Type: </strong>$type</p> <p><strong> Department Head: </strong>$depthead</p> <strong> Test Analyst: </strong> $person<br/></td> HERE; Look real close at line #6 above. You never closed the INPUT field, so you never closed the FORM, so the whole page is one big form and every submit button will submit all of the IDs.
-
There's not enough information to give a complete answer. If you can specify an ORDER BY, so you get the Accounts in sequence you can just put that code in a loop until you get an indication that nothing was found. See my comments (#MAD) in the code below. Since I don't know the API, I can only guess at how to determine the exit point. public function loadAccounts() { $startid = -1; #MAD Initialize the collection of accounts first $this->accounts = Array (); while(true) { #MAD Until we issue a break; #MAD You need to check the API for an ORDER BY command - Here's my GUESS $xml = ' <queryxml> <entity>Account</entity> <query> <field>id<expression op="greaterthan">'. $startid .'</expression></field> <order direction="ascending">id</order> </query> </queryxml> '; $query = new query ( $xml ); $result = $this->client->query ( $query ); #MAD If the API returns FALSE when nothing is found ... if (!$result) break; #MAD OR test Entity to see if it has children? if (!$result->queryResult->EntityResults->Entity->haschildren) break; $laccounts = $result->queryResult->EntityResults->Entity; #MAD OR if $laccounts is an Array if (empty($laccounts)) break; foreach ( $laccounts as $account ) { $this->accounts [$account->id] = $account; $startid = $account; #MAD So we get the LAST one returned and can issue a query for next } }
-
The caret ("^") at the beginning, anchors the match to the beginning of the input string; The dollar ("$") at the end, anchors the match to the end of the input string; The parenthesis ("( )") define a capture group, not a range of characters; So try '/@[a-z]+/i' The "i" after the expression makes it case-insensitive. The "+" means one or more (the askterisk, "*", says zero or more which means an "@" alone would match). Also, this will not allow digits in the token, nor special characters, only the letters "A" - "Z" (upper- or lower- case)
-
You can't just add parameters to a function call. You need to put the CC as a header: $headers .= "Cc: <[email protected]>\r\n"; Keep in mind. When you do that, whoever you are sending the CC to will see YOUR email address as well. If you want to prevent that, send it TO: the user and BCC: to your email.
-
mysql_query returns a resource, NOT the query results. You need to fetch the data. Other comments: 1) The mysql library is deprecated, use mysqli (mysqli) 2) Don't use short tags "<?", use full tags: "<?php" 3) Use SELECT COUNT(*) if you want a count, don't return all of the data if you don't need it
-
little help please. need to make file get uploaded by form/
DavidAM replied to lovephp's topic in PHP Coding Help
Did you try closing the file? On a *nix system if would not matter, but Windows is particular about that. -
Warning: mysqli_num_rows() expects exactly 1 parameter
DavidAM replied to dojo's topic in PHP Coding Help
If the error message is: Try looking at that line: mysqli returns an object, so you don't need to pass the link, just the result (in your case $sql). So, it needs only 1 parameter (like the error message says). -
little help please. need to make file get uploaded by form/
DavidAM replied to lovephp's topic in PHP Coding Help
This is no real reason to move_uploaded_file() if you aren't going to keep it. Just open the temporary file. PHP will remove that one at the end of the script (supposedly). But maybe you need to close it before you unlink it. If this is Windows, I'm pretty sure that is the problem. As for processing ... while (($data = fgetcsv($getfile, 100000000, ",")) !== FALSE) { if (count($data) > $data = array_slice($data, 0, ; $query = "INSERT INTO details(id, student_phone, student_fname, student_lname, street_address, city, state, zip) VALUES('" . implode("','", $data) . "')"; $s=mysql_query($query); } } Actually, you would be better off using a prepared statement. And even better off by doing a multi-row insert. But since you are still using the mysql library, I'll leave it at that. Note: the mysql library has been deprecated. You need to switch to mysqli. -
little help please. need to make file get uploaded by form/
DavidAM replied to lovephp's topic in PHP Coding Help
move_uploaded_file returns TRUE or FALSE, not the name of the file. You should test if move_uploaded_file() succeeds, then open "uploads/".$file_name. -
1. Files belong in the Filesystem. Data belongs in the Database. Store the files in the filesystem, and store the path in the database. 2. Databases are designed to store, retrieve and manipulate data; and they are very good at doing that. While most (all?) databases store "BLOB" fields, they are not designed to be query-able. I mean, how many times are you going to want to find all files that contain the hex value 0xDEADBEEF, and how efficient is that query going to be? Storing files in the database does give you a single repository of all of your "data". So backups and transfers are simpler processes. However, your database backups will be huge with more potential for problems. If you dump to a set of INSERT statements, and then modify the dump file, you run the risk of damaging the file contents. Since binary files are "type" specific, a change to a single byte of an image file data, can render the entire "file" (image) invalid and unviewable. The recommended approach is to define a base path in your application specifying where these files are stored. Then include a sub-directory path in the table relative to the base path. This will permit you to move the data and files to a different server, and you only have to change the application's base path. If you are in the habit of using SELECT *, then your queries will return every column in the table. If you have files stored in the database, you will be retrieving the entire file (1K, 100K, 5MB?) with every query. If you store the path, then you get the path (VARCHAR(255)?). Note: Using SELECT * is not on the list of "best practices", and I always advocate against; but if you use it, you must be aware of the issues.
-
While I agree that the test should be if (!empty($row['img'])); the manual says: for both mysql and mysqli http://php.net/manual/en/function.mysql-fetch-assoc.php http://php.net/manual/en/mysqli-result.fetch-assoc.php
-
If the makeTable() function is actually making a Table (i.e. CREATE TABLE), then it can NOT be rolled back. In fact ... (emphasis added -- see http://dev.mysql.com/doc/refman/5.7/en/implicit-commit.html) Why are you creating a table in the middle of a transaction. Either create the table before you start the transaction, or redesign your application and database so you don't have to create tables on the fly. (Personally, I highly recommend the second option).
- 2 replies
-
- transaction
- ajoo
-
(and 1 more)
Tagged with:
-
SELECT ... FROM ... WHERE (num1 = 123) OR (num2 = 123) OR (num3 = 123)
-
No, you really don't want to keep them. This is how nightmares begin. If you need those values later in the code, then you want to use an array. <?php include "dbinfo.php"; $sql = 'SELECT category FROM categories WHERE id IN (1,2,3,4)'; $res = mysql_query($sql, $db); # You should switch to mysqli (improved) as the mysql library is deprecated $catList = array(); while ($row = mysql_fecth_array($res)) { $catList[] = $row['category']; } echo "<table width='100%' border='1'>"; echo "<tr>"; foreach($catList as $cat) { echo"<th width='25%' scope='col'><div align='center'>" . $cat ." </div></th>"; } echo "</tr><tr><td>"; # You didn't close the table - is there more to come? mysql_close($db); # It is usually NOT necessary to close the database connection it will close when the script exits ?>Using an array will make it much easier if you later decide to add a fifth category (or even to remove one of the four). In the code above (which does exactly the same as the original code you posted), to add or remove a category, we simply change one line, the query. With your code, you would have a lot more work. If you need to associate the category with the ID (in later code), then select the ID and the category: SELECT id, category from ..., then use the ID as the index of the array $catList[$row['id']] = $row['category'];.
-
Assign a "score" value to each of your "possible" rolls. Then calculate the "score" of each roll and compare. Hint: The "scores" do not have to be incremental, just sequential.
-
Your query gets the tweets of the the users being followed by the logged in user. We just need to add an OR clause to get the tweets of the logged in user. $sql = "SELECT * FROM tweet LEFT JOIN follow ON tweet.user_id=follow_user_id WHERE follow.user_id = " . $_SESSION['user_id'] . " OR tweet.user_id = " $_SESSION['user_id'] . " ORDER BY date_time desc";Notes:1) If the IDs (user_id, follow_user_id) are defined as integers (in the database), you do not need to wrap them in quotes (in the query). 2) Don't use SELECT *, select only the columns you actually need. 3) We need the LEFT JOIN just in case nobody is following the logged in user. 4) If the tables get really big, this may not be the most efficient query. You might have to look at a UNION query instead of the OR
-
The query is failing, probably for the reason stated by AbraCadaver. Instead of chaining all of the function calls together, you should break it up and check for failures (especially in development). // Instead of $num = mysql_num_rows($allusers = mysql_query("SELECT * FROM PMs WHERE `status`='0' AND ReceiveID='$myU->ID' ORDER BY ID")); // Use this $sql = "SELECT * FROM PMs WHERE `status`='0' AND ReceiveID='$myU->ID' ORDER BY ID"; $allusers = mysql_query($sql); if (!$allusers) { // The query failed -- do something logical here trigger_error("Query Failed: $sql -- " . mysql_error(), E_USER_ERROR); } $num = mysql_num_rows($allusers); Use this approach during development to have mySql and PHP tell you what is wrong. In production, you don't really want to just die like that - E_USER_ERROR kills the script - since the user will get a blank page (or only a partial page), and you don't want to show the error message to the user (security issue). You need to decide how to handle a fatal error so the user gets something meaningful. And yes, it is possible for a completely valid "working" query to fail after being moved to production -- The database server is down, the database server is overworked, the database itself becomes corrupt, etc. Note: I left the error in the code above so the OP can see the error message and how it will help.