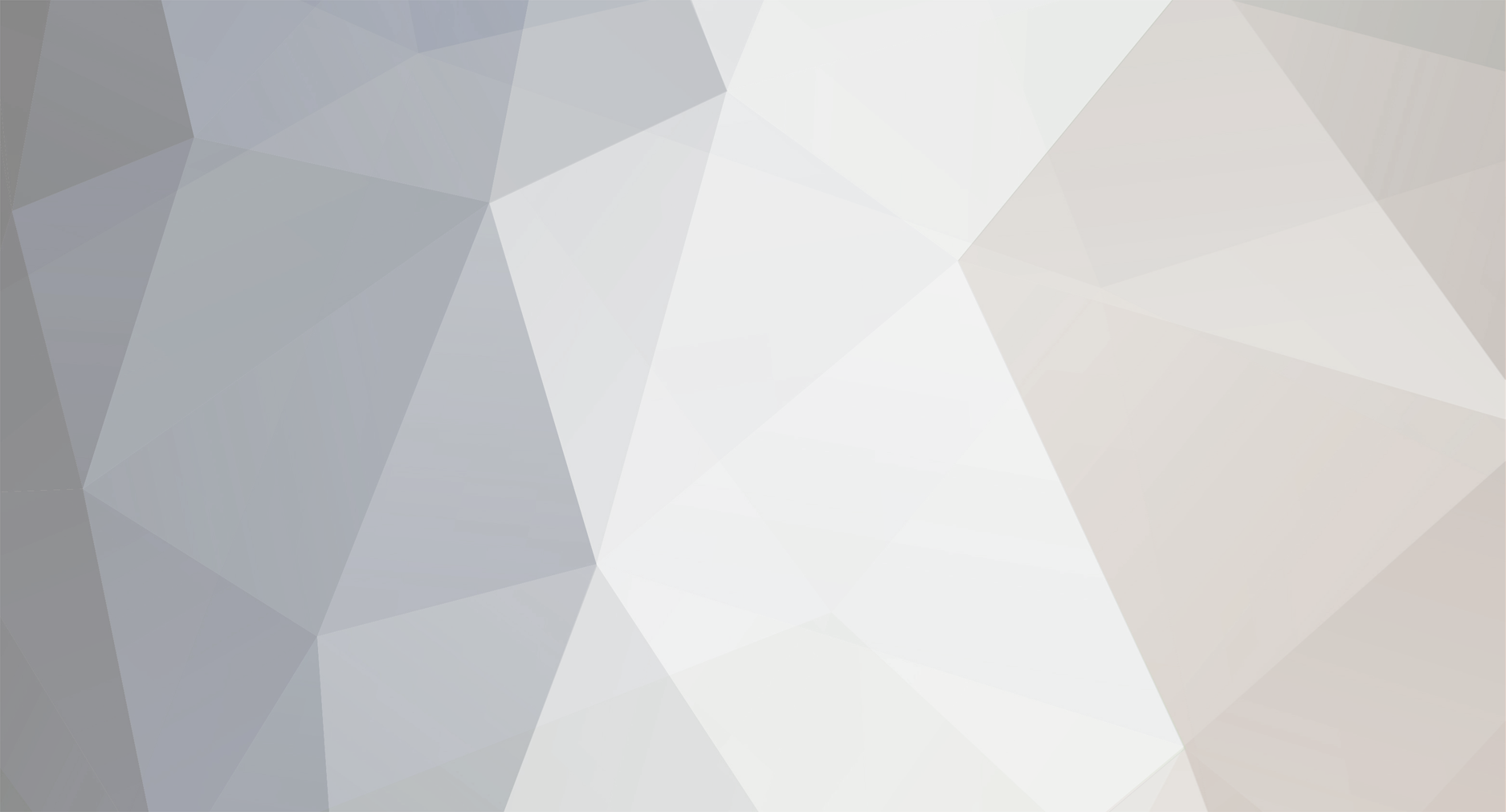
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
I guess I read the question differently. Are you trying to show the user a count-down? Something like: "This offer expires in 1 hour 12 minutes 32 seconds" -- Or are you trying to have some task performed at, say, midnight on Sunday? For the later, a cron job is the way to go for the reasons stated by others. For the former, we probably need a little more information; but JavaScript and AJAX are the direction to start.
-
Welcome to phpfreaks!! In general, storing images in the database is more trouble than it is worth, and NOT what a database server is designed to do. To answer your actual question: General questions about application DESIGN should be posted in the Applications Design Forum. Questions about specific problems you are having with PHP code would go in the PHP Coding Help Forum. There are several other forums for more specific questions: Database/SQL, Client Side (CSS, HTML, JavaScript), and so forth. Just look through the list and find something specific to the question or issue you have. IMHO, choose the MORE specific forum rather than the LESS specific; as long as it fits the issue. Picking the "right" forum can help you get answers more quickly; since someone who is not highly proficient in say, JavaScript, may never look in the JavaScript forum, so they wouldn't see a question there about MSSQL.
-
Scope/Visibility Question about Child/Parent class
DavidAM replied to ballhogjoni's topic in PHP Coding Help
It has been my experience that protected methods are available up and down the inheritance tree. <?php error_reporting(E_ALL); ini_set('display.errors', 1); class A { protected function hello () { print("Hello from A\n"); } public function askMe() { print("A says:\n"); $this->hello(); $this->test(); } } class B extends A { protected function test() { print("Hello from B\n"); } public function askMe() { print("B says:\n"); $this->hello(); $this->test(); print("Ask A ..."); parent::askMe(); } } class C extends B { public function askMe() { print("C says:\n"); $this->hello(); $this->test(); print("Ask B ..."); parent::askMe(); } } print("Version: " . phpversion() . "\n\n"); $c = new C; $c->askMe(); $c->test(); $c->hello(); /* Version: 5.3.4 C says: Hello from A Hello from B Ask B ...B says: Hello from A Hello from B Ask A ...A says: Hello from A Hello from B Fatal error: Call to protected method B::test() from context '' in D:\wwwIntranet\wwwDEV\public\inhTest.php on line 46 If you comment out line 46, then you get this error: Fatal error: Call to protected method A::hello() from context '' in D:\wwwIntranet\wwwDEV\public\inhTest.php on line 47 */ From an OOP standpoint, it doesn't make sense for A to call test() since it is not defined until B; unless of course, A defines it as abstract so we know it will be there later. You cannot call hello() or test() from outside of the class, because they are protected. I think KevinM1 is answering questions in his sleep, again. -
Create Folder, Create File, Add To File, Close File
DavidAM replied to MattCoulter's topic in PHP Coding Help
1) You have not stated a question or problem. 2) Please use tags when posting code: mkdir($username); $file=fopen($username."/index.htm","w"); fwrite($file,"<html>"."</html>"); fclose($file);3) Be careful using user input for filesystem access. There are certain characters (/, \, :, <, >, $, |, <space>, etc.) that will either cause the mkdir command to fail or create problems accessing the files later (especially from the command line). If I was going to do something like this, I would use the user's ID from the database (an Integer) perhaps prepended with zeros -- so UserID # 4 might be "000000000004" for a folder name. This would allow the username to change without the complication of renaming the folder. 4) You should really test each step to be sure an error has not occurred: // Error reporting during development error_reporting(E_ALL); ini_set('display.errors', 1); if (! mkdir($username)) trigger_error('mkdir Failed', E_USER_ERROR); $file=fopen($username."/index.htm","w"); if (! $file) trigger_error('fopen Failed', E_USER_ERROR); if (! fwrite($file,"<html>"."</html>")) trigger_error('fwrite Failed', E_USER_ERROR); fclose($file); Of course, that's not any better than just using or die('xxx Failed');. You need to do something logical at any particular failure point. I would stick the whole thing in a function and handle it: error_reporting(E_ALL); ini_set('display.errors', 1); function makeUserIndex($username) { if (! mkdir($username)) { trigger_error('mkdir Failed', E_USER_WARNING); return false; } $file=fopen($username."/index.htm","w"); if (! $file) { trigger_error('fopen Failed', E_USER_WARNING); return false; } if (! fwrite($file,"<html>"."</html>")) { trigger_error('fwrite Failed', E_USER_WARNING); fclose($file); return false; } fclose($file); return true; } $user = 'DavidAM'; if (! makeUserIndex($user)) { // Something here to handle the failed attempt } (not tested) Ideally, the filename (index.htm) and the content would be passed to the function as well. -
You have to handle NULL's in a special way. The proper SQL syntax would be: call spMyStoredProc(1,2,NULL,'username')to produce that from PHP, you have to test the parameter: $param3 = null; if ($param3 === null) { // That's a PHP null $sql = "call spMyStoredProc(1,2,NULL,'username')"; } else { $sql = "call spMyStoredProc(1,2,'" . mysql_real_escape_string($param3) . "','username')"; }I have a function for escaping that handles this: function quoteStringNull($pmValue, $psDelimiter = "'") { if ($pmValue === null) return 'NULL'; return $psDelimiter . mysql_real_escaspe_string($pmValue) . $psDelimiter; } $param3 = null; $sql = sprintf('call spMyStoredProc(1,2,%s,"username")', quoteStringNull($param3)); // call spMyStoredProc(1,2,NULL,"username") $param3 = 'Master'; $sql = sprintf('call spMyStoredProc(1,2,%s,"username")', quoteStringNull($param3)); // call spMyStoredProc(1,2,'Master',"username") (That's off the top of my head, I don't have access to my library right now). The function returns the string NULL which is sent to mySql properly; or it returns the delimited string value (including the quotes). So the function results can be dropped straight into the sql string.
- 6 replies
-
- mysql_real_escape_string
- null
-
(and 3 more)
Tagged with:
-
You'll want to urlencode the url in the query string. $source = '?source=' . urlencode($_SERVER['REQUEST_URI']); just in case there are any "special" characters in it. Do you want the url of the page that is doing the redirect (which is what Ch0cu3r's code gives you)? Or do you want the url of the the page they came from to get to the page that is doing the redirect? In the second case, use $_SERVER['HTTP_REFERER'] instead of 'REQUEST_URI'.
-
Is equivalent to $conn = mssql_connect( "SERVER", "username", "password" ) or die( "Err:conn - " . mssql_get_last_message());It is essentially the same code. On the other hand, "Err:conn - Changed database context to 'databasename'." does not look like an ERROR message to me (except that it comes from your die() statement. Is it possible you copy-pasted that or die() and forgot to change the prefix ("Err:conn")? What I am saying is that I would think that is an informational message, which should not cause the connection to fail, so $conn should have a value and the or die() should not happen. I've never used mssql, but a quick search though the PHP manual gives me the impression you may want to look at mssql_min_message_severity and/or mssql_min_error_severity or the associated php.ini settings.
-
When doing a login page (as the OP is), you usually have other pages that are restricted to logged-in users. In many cases, you want to know WHO is logged it (at some point). So the "most efficient" method would be to select the USER_ID and store it in a SESSION variable which can be retrieved by later pages as needed. Since a user's login MUST be unique, there will only be one row returned, so there is only ONE fetch. In this instance, there is no difference in performance between fetching the count column and fetching the user-id column. $sql = "SELECT user_id FROM users WHERE username = '$username' AND password = '$password'"; $query = mysqli_query($dbc, $sql); if ($query) { $row = $query->fetch_assoc(); if ($row) { // A row was returned $_SESSION['user_id'] = $row['user_id']; } else { // Invalid Username OR Password } } else { // ERROR unable to access the database } In fact, I might select the user's "Display Name" and "Access Rights" (if applicable) and then just assign the entire row to the SESSION so I don't have to hit the database to check his/her access or name on every page load. But that really depends on the application requirements.
-
grabing a single jpeg frame from mjpeg stream using PHP
DavidAM replied to fsusr's topic in PHP Coding Help
$camurl="http://127.0.0.1:8080/"; - 127.0.0.1 is the local loopback address on whatever system the code is running. If this is the address you actually used on your host, it is not going to find the web server on your home computer. $f = fopen($camurl,"r") ; - It is possible that your host does not have allow_url_fopen enabled which would cause this to fail on a url (http://). If this is the case, you will have to use sockets as in your second example, or curl. What exactly are you seeing, and what have you done to debug this? Try putting a true jpg file in the root of your home system, and change the fputs() call to "... GET /test.jpg..." or whatever name you used for the image. See if you get the image or the thing sill hangs up. Try capturing, oh say, 1000 bytes from the socket and dump it Try printing out the $str values you are sending to header(). Maybe you are getting an "error" page - does your home web server deny access to anything not on the LAN? -
Retail_Price should be a NUMERIC column (not VARCHAR), so it will not have the comma in it. Then you can use Retail_Price <= 5000. Using a string-type field for numeric data will make it virtually impossible to compare: i.e. 120 < 30 when compared as a string.
-
That would certainly prevent "record(ing) the same song twice "; however, I suspect the OP means "same song twice in a row". You need to get the title of the last song recorded: SELECT SongTitle FROM `table` WHERE pId = (SELECT MAX(pId) FROM `table`)However, I would not recommend using the pId for this. I would combine the playedDate and playedTime into a single DATETIME field, and use playedDateTime = (SELECT MAX(playedDateTime). Furthermore, if this is a continuously running job, I would just save the "last song" in a PHP variable for immediate comparison instead of going back to the database repeatedly. To prevent duplicates when the script starts (say after a reboot or whatever), load the last song from the database at startup. Oh, and give your table a meaningful name. "Table" is just not helpful, and creates the need to use the backticks whenever you query it.
-
Your original code: // Wanneer de 'id' variabel is ingesteld in de URL, weten we dat we een record moeten aanpassen if (isset($_GET['id'])) { // Wanneer de submitknop word ingedrukt word het formulier verwerkt if (isset($_POST['submit'])) { // Zorgt ervoor dat de 'id' geldig is if (is_numeric($_POST['id'])) { is checking to see if $_GET['id'] is set, then retrieving the value from $_POST['id']. These are two different resources. If the FORM method is POST and the "id" is a field in the form (which is what I see), you should be checking $_POST. If you are expecting the "id" to be part of the URL, then you need to retrieve it from $_GET. If you want to allow it to come from either, you can use $_REQUEST, however, since you have it in the form, you have to watch out for the possibility that it comes in both places AND IS DIFFERENT
-
Session Variables Being Partially Lost After Redirect
DavidAM replied to phdphd's topic in PHP Coding Help
It is possible that the "old" environment has output buffering turned on and the script in question has some output before the call to session_start(). If the "new" environment has output buffering turned off an error occurs with the call to session_start(), and the session is not actually started, so no new values can be assigned to the session. Turn on error_reporting() for the development server and fix any errors (or warnings or notices) that occur. -
New to PhP, Help setting up Header.tpl and Footer.tpl
DavidAM replied to BrandonBarker's topic in PHP Coding Help
I would add one step before that. (As long as you are learning, learn the "best practices"): <?php /* All page processing including: * Retrieve page parameters * Validate user supplied data * Retrieve data from database * Process data ... */ include '/pathtoheader/header.tpl'; /* Your page content here (HTML with a sprinkling of PHP to echo data) */ include '/pathtofooter/footer.tpl'; -
Part of the problem with obfuscated questions is that we can't really tell what you are trying to do, and it makes it difficult to understand the problem and provide an answer. I hope you have permission to scrape whatever site you are scraping and you are not violating their Terms of Service. If you are, please ignore my answer below. The problem is this line: $file = "texts/".md5($text)."-".$number.".txt";You are using THE ORIGINAL TEXT STRING FOR EVERY FILE in the md5() call. I suspect you meant to use $txt there. Which would give you a different file for each result. Also, take that first call to file_get_contents() out of there. You are wasting your resources and resources on the other site: if (! ($txt = file_get_contents(...))) { echo 'Error'; } else { # Process $txt }
-
kicken's suggestion was to have announcements_current.php generate the links the way you want them while building the page in the first place. So instead of echo $row['TextFromDB']; you would write a function to convert the links then echo ConvertLinks($row['TextFromDB']);.
-
java script to validate and accept only specific words
DavidAM replied to cainam29's topic in Javascript Help
Why use TEXT AREA field if the input is restricted to one word out of a specific list. Event if you have 10 or so allowed words, you should probably be using a SELECT element. Then the JavaScript is not really even needed. It is true that you can NOT rely on JavaScript validation to prevent faulty input. However, it can improve the user's experience to verify the data in JavaScript. The user gets immediate feedback on potential problems, and you save your server the extra load of processing bad data. However, you MUST validate at the server since the user CAN disable JAVASCRIPT, or re-write the form entirely. -
You are only fetching and echo'ing ONE row, so that is all you see. You will need to loop through the returned rows: if (mysql_num_rows($result)) { echo "<TABLE>"; while ($row = mysql_fetch_array($result)) { echo " <tr> <td>" .$row["tipo"]. "</td> <td>" .$row["ciudad"]. "</td> <td>" .$row["sector"]. "</td> </tr>"; } echo "</TABLE>"; }
-
1) Turn on error reporting (at least in development) so PHP can tell you about problems error_reporting(E_ALL); ini_set('display.errors', 1); 2) Test the results of your queries. I suspect the first one is failing miserably - the second parameter (if provided) should be the connection resource, not a string variable. You are missing the WHERE clause and should (probably) be concatenating: "." instead of "," if ($duperaw === false) { // The query failed, do something reasonable) In your second call to mysql_query you put the link resource first (it should be second if you provide it, but it is not required if you have only one open connection). 3) You cannot embed an associative array element in a double-quoted string unless you use curly-braces $sql = "INSERT INTO bx_sites_main (URL) VALUES ('{$link['link']}')" if (mysql_query($sql)) echo "Data Inserted"
-
Need Help With Loop of Results After Join Query
DavidAM replied to spock9458's topic in PHP Coding Help
Yeah, every now and then I like to bring back some of the classic (meaning old) terminology. @spock9458 I should have said, it is better to use something guaranteed to be unique (like the Company ID or primary key), otherwise, you might run into a problem with two companies having the same name in the database. -
@fatsol What code are you reading? The submit button does have a name, it is "submit". The problem is the single quotes inside the query: @failchicken $sql = "INSERT INTO gallery_category (category_id,category_name) VALUES (''',$_POST[cat]')"; If you look close you see three quotes before the comma and no quote after the comma before the category value. Of course, the debug message is showing nothing after the comma and only two single quotes before it, so somthing was changed before that run.
-
Need Help With Loop of Results After Join Query
DavidAM replied to spock9458's topic in PHP Coding Help
Good old-fashioned "control break" processing $lastCompany = ''; # Track the "last" company we processed while ($row = ...) { if ($lastCompany != $row['Company']) { // OK we have a new Company to output $lastCompany = $row['Company']; # Track the "last" company we processed # output company data } # output Contact Data } -
The query is invalid: if (! mysql_query($sql, $con)) die('Query Failed: ' . mysql_error($con) . ' -- ' . $sql); to find out why
-
How do you know which MANU_ID you want associated with this "model"? There really is not enough information in your question to provide the answer you seek. Is the Manufacturer specified on the form along with the model? Are you holding the ID in a session? $manuID = [Whatever the value should be] $model = $_POST['model']; # THIS NEEDS TO BE ESCAPED FOR YOUR DATABASE ENGINE $sql = "INSERT INTO Models (MANU_ID, MOD_Name) VALUES( $manuID, '$model')"; Note: You need to validate and escape all input from the user to protect against SQL Injection as well as special characters in the value.