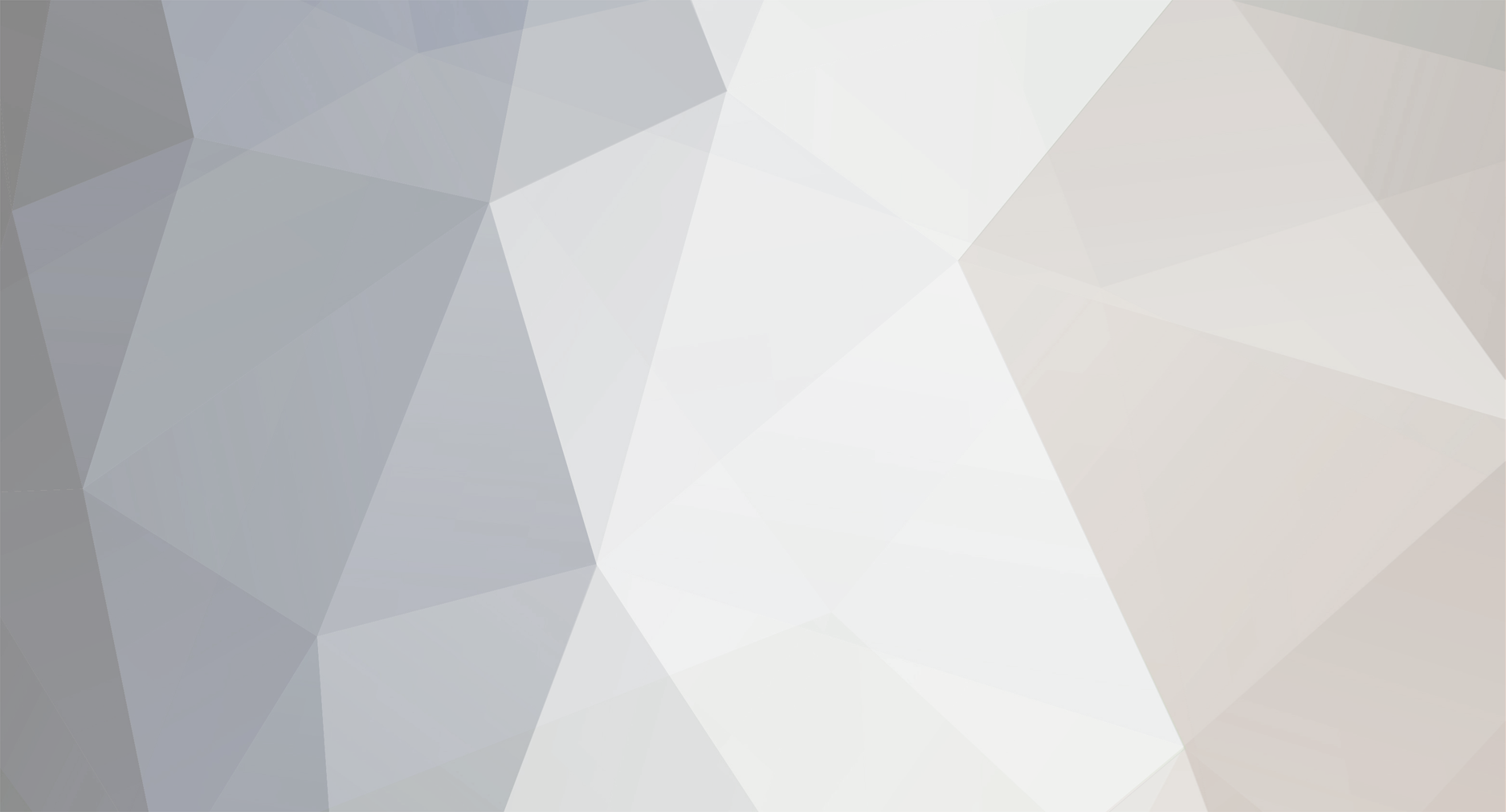
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
I don't want to insult you, but sometimes I ask very basic questions just to make sure. * How are you viewing the file and seeing the slashes? Is it possible that your viewer is adding them? Probably not, but let's just eliminate the possibility. * That's all of the code? You are not using mysql_real_escape_string() somewhere, right? * Try a liberal case of var_dump() to see where the data is getting changed. I usually wrap the var_dump() display in PRE tags just so it is clear in the browser. Try this: echo '<PRE>'; var_dump($_POST); echo '</PRE>'; // ADD THIS LINE $code = $_POST['code']; echo '<PRE>'; var_dump($code); echo '</PRE>'; // ADD THIS LINE // i also tried with w+b and w+t but same $file = "file.php"; $ourFileHandle = fopen($file, 'w+') or die("can't open file"); fwrite($ourFileHandle,$code); echo '<PRE>'; var_dump($code); echo '</PRE>'; // ADD THIS LINE (JUST TO DOUBLE CHECK) fclose($ourFileHandle); } You might also look at the "View Source" of the browser to see if there is anything special in the HTML. ** Post the dump results and let's see if we can nail this one down. * What version of PHP are you running?
-
So, this data is coming from a FORM? Does your site have magic_quotes_gpc turned on? If so, then you need to stripslashes() when you retrieve the data from $_POST (or $_GET). You said you tried stripslashes() so I did not mention it before. Try echoing out the value at various places to see where the slashes are coming from. If magic_quotes are on and you may be double escaping the input somewhere (you're not using addslashes() somewhere, right?). Can you post all of the code from where you capture the $_POST through the fwrite()?
-
Rather than screw with the data to try to include the ID, change the way you define the input fields. <input type="text" name="values[<?php echo $packet['piid']; ?>]" value="<?php echo $packet['value']; ?>" /> Here we put the piid value as the index to the array of values. When you process the POST values from the form we can do something like this: foreach ($_POST[values] as $piid => $newValue) { // $piid is the database index we need to update // $newValue is the value entered by the user } By the way, in your original code, you are creating a FORM tag after the input fields inside your loop. This is going to create all kinds of problems. The FORM tag has to come before any of the INPUT fields. Its not clear if you want a single FORM for the entire page, or separate FORMs for each package. But you need to review the way you are creating them and do it appropriately.
-
printf("<p>Comment by <a href=\"%s\">%s</a> @ %s</p>", stripslashes($row['url']), stripslashes($row['name']), $timestamp); The printf() function replaces placeholders in the first argument with data from the remaining arguments. In this statement there are three placeholders (they are the "%s" codes in the first argument). You have only supplied two values to replace these three, so you have provided "Too few arguments" (i.e. not enough). Since you removed the timestamp at the end, I guess you want to remove the "@ %s" in the format string (the first argument). printf("<p>Comment by <a href=\"%s\">%s</a></p>", stripslashes($row['url']), stripslashes($row['name']), $timestamp);
-
Are you running in a Windows environment? From the php manual for fwrite() Personally, I had thought this only applied to the CR-LF line endings, but you might try adding the "b" flag to the fopen $ourFileHandle = fopen($filefile, 'w+b');
-
Display files in array from end to beginning
DavidAM replied to franzwarning's topic in PHP Coding Help
Try rsort() instead of sort()? -
NOT ME!! Well ... not today at least. --- OK, actually, not since lunch.
-
Using "SELECT *" and then mysql_num_rows() to get a count, is a bad idea. Understand that the database is going to send all the data from every row that matches the query to the PHP client. This takes up bandwidth, memory and time. It is MUCH more efficient to use COUNT(*) and then retrieve the value that was counted. For instance: $sql = "SELECT COUNT(*) FROM myTable WHERE someCondition = 'whatever'"; $res = mysql_query($sql); if ($res !== false) { $row = mysql_fetch_row($res); echo "There are " . $row[0] . "entries."; } Note: Since we are only expecting a single row to be returned, it is not necessary to use a while loop to process the results. The code in your original post was not too far off from that. However, inside the loop you were referring to $row['COUNT(username)'] and $row['date']. These are not selected in your query, so they do not exist and are output as an empty string. If you are looking for the result for a single user and a single date, the original code would be something like this: $username = "bayyari"; $date = "3-16-2011"; $query = "SELECT COUNT(*) FROM totals WHERE date = '$date' AND username = '$username'"; $result = mysql_query($query) or die(mysql_error()); // Print out result while($row = mysql_fetch_array($result)){ echo "There are ". $row[0] ." ". $date ." items."; echo "<br />"; echo $result; }
-
I looked at all my Facebook cookies, and my phpFreaks cookies. And I don't see my login name or my password in there anywhere. I used to store the user's Login Name in a cookie and use that for authentication. But after reading another thread here on phpFreaks, I switched to using a unique id. As I said, I don't know exactly how Facebook does it - I've never seen the code behind their pages. It is not really that complicated. You design it once and code it once as a function or part of a class, and then you just call the function/method from every page. In my case it is part of a class I use to manage SESSION data.
-
Facebook does not actually store your password or your login name in a cookie. Have a look at the cookie and you will see. The way this is done - well, the way I do it and I suspect they do it (since I have not seen their code) - is to store a unique ID in the cookie. This unique id is also stored in the database. When you arrive at the site, and they receive your cookie, they lookup the unique id from the cookie and retrieve your login name from the database.
-
First, you should not store the user's password in a cookie. Cookies are not secure, so someone can either read them from the computer or intercept them when sent to the site. Since many users use a common password for multiple sites, a password stored in a cookie could compromise the user on more than one site (not just your). As to staying logged in, when a user comes to your site, the cookies are sent. You can decide in the PHP script what to do with those cookies. If the cookie has the appropriate information (appropriate to your script, that is), you can decide that the user is logged in and not bother them with the login page.
-
First Question: The session_start() call must occur before ANY output is sent to the browser. Since the DOCTYPE is output to the browser, the call has to occur BEFORE the DOCTYPE is sent. Second Question: You can set the session time limit, but not the way you want. Once the user closes the browser, the session cookie is destroyed. So, you cannot EXTEND a session across browser "sessions".
-
inserting id into another table as a foreign id: how?..
DavidAM replied to peppericious's topic in PHP Coding Help
GET and POST are useful when the user is sending you data. But when you want to pass data from one script to another, or maintain a value between outputting the form and receiving the POST, SESSION variables are the way to go. Hidden form fields do have their uses. I place a hidden field on each form with a value from uniqid() (with a little obfuscation), and store the value in a session variable. If I receive a form without this id or with an id different from the session value, then someone is not playing nice, and I kick the post back. I cringe whenever I read a post here of someone who is storing the price of the product they are selling in a hidden field so they can have it on the POST. A determined user could easily change the price and buy the item cheaper if the script does not verify the price on POST. (Maybe "determined" is not the right word here. It would take less than 60 seconds to do it and very little effort). -
Something like this if (! $result) { // Query Failed } elseif (mysql_affected_rows() == 0) { // No such book } else { // Book Deleted }
-
mysql_query() will only return false if the query fails - i.e. if it is invalid. A DELETE (or UPDATE) statement that does not affect any rows, is valid. Use mysql_affected_rows() to see how many rows were deleted.
-
You only have one capture there, so it should be $1 RewriteRule ^logger/(.*)/$ index.php?adm=logger&id=$1
-
Have a look at your generated HTML (view page source). Your page starts with a DOCTYPE, HTML open tag and HEAD section, then there is another DOCTYPE, HTML open tag and HEAD section INSIDE the BODY. In fact, there is a second BODY inside that BODY as well. The form you are having trouble with appears AFTER the closing BODY and closing HTML tags. I'm sure this confuses the browser no end. Clean up the code you generate and see if that fixes the form.
-
You are missing the CR-LF pair after the From: header. $headers = "From: Klone slikbestilling\r\n" . "MIME-Version: 1.0\r\n" . "Content-Type: text/html; charset=ISO-8859-1\r\n"; Also, the value of the From: header has to be a valid email address on the sending server. (I'm guessing you took it out for this post, but just make sure it is there when you send it).
-
What does "nothing happens" mean? Does the browser attempt to load the PHP script? Do you get any error messages? Do you get a blank screen? I do see two lines in that code that could be causing problems: $headers .-"Content-type: text\html\r\n"; $success - mail($webMaster, $emailSubject, $body, $headers); Shouldn't that be: $headers .= "Content-type: text\html\r\n"; $success = mail($webMaster, $emailSubject, $body, $headers);
-
inserting id into another table as a foreign id: how?..
DavidAM replied to peppericious's topic in PHP Coding Help
Actually, while you should be able to pass it in GET, using a SESSION variable is more secure. Accepting it through GET, or even POST (as a hidden field) is not safe. The user can easily modify the form and send a different ID which would either cause your database INSERT/UPDATE to fail, or even worse, succeed but associate the image with the wrong news item. -
inserting id into another table as a foreign id: how?..
DavidAM replied to peppericious's topic in PHP Coding Help
You also need to turn off magic_quotes_runtime There are three magic_quotes settings: [*]magic_quotes_gpc - Affects the super globals: GET, POST, COOKIES, ENV [*]magic_quotes_runtime - Affects most functions the "return data from any sort of external source including databases and text files" [*]magic_quotes_sybase - Affects how the above settings behave, as well as stripslashes() and addslashes See the manual for magic_quotes_runtime Basically, these should all be OFF -
Use number_format()
-
Move your FORM open tag outside of the WHILE loop. if (mysql_num_rows($messages_query) > 0) { echo "<form method='POST'>"; while ($messages_row = mysql_fetch_array($messages_query)) { if ($messages_row['message_read'] == 0) { echo "<div style='background-color:#FFCCCC;'>"; } $message_id = $messages_row['id']; echo "<a href='message.php?id=$message_id'>"; echo "From: " . $messages_row['sender']; echo "Subject: " . $messages_row['subject'] . "<br />"; echo "</a>"; echo "<input type='checkbox' name='message[]' value='1' />"; if ($messages_row['message_read'] == 0) { echo "</div>"; } } } But with this code you cannot tell WHICH checkbox was checked. Change the CHECKBOX line to : echo "<input type='checkbox' name='message[]' value='$message_id' />"; Then you can walk the array of checkboxes with something like this: psuedo-code foreach ($_POST['message'] as $msgID) { DELETE FROM messages WHERE id = $msgID; }
-
No. You cannot delete a file on the client machine from the server (PHP). That would be a huge security issue. You can't even delete a file using JavaScript on the client side. CSV stands for "Comma Separated Values". A CSV file is only meant to contain data, it cannot hold any formatting. If you want to add formatting, you would have to build an Excel (or other type of formatted) file instead. It seems to me that I have seen references to a PHP library that allows you to read and write XLS (native Excel) files. But I don't know anything about it as I have never used it.
-
Sessions & Mails... Major problems in one thread.
DavidAM replied to Bencori's topic in PHP Coding Help
Also, on the mail() issue, did you change the from address that you use in your code? The mail clients (Gmail, etc) will probably consider a mail as spam if the from address does not match the server actually sending the mail. Did you check the junk/spam folders in your inbox to see if the mail is there?