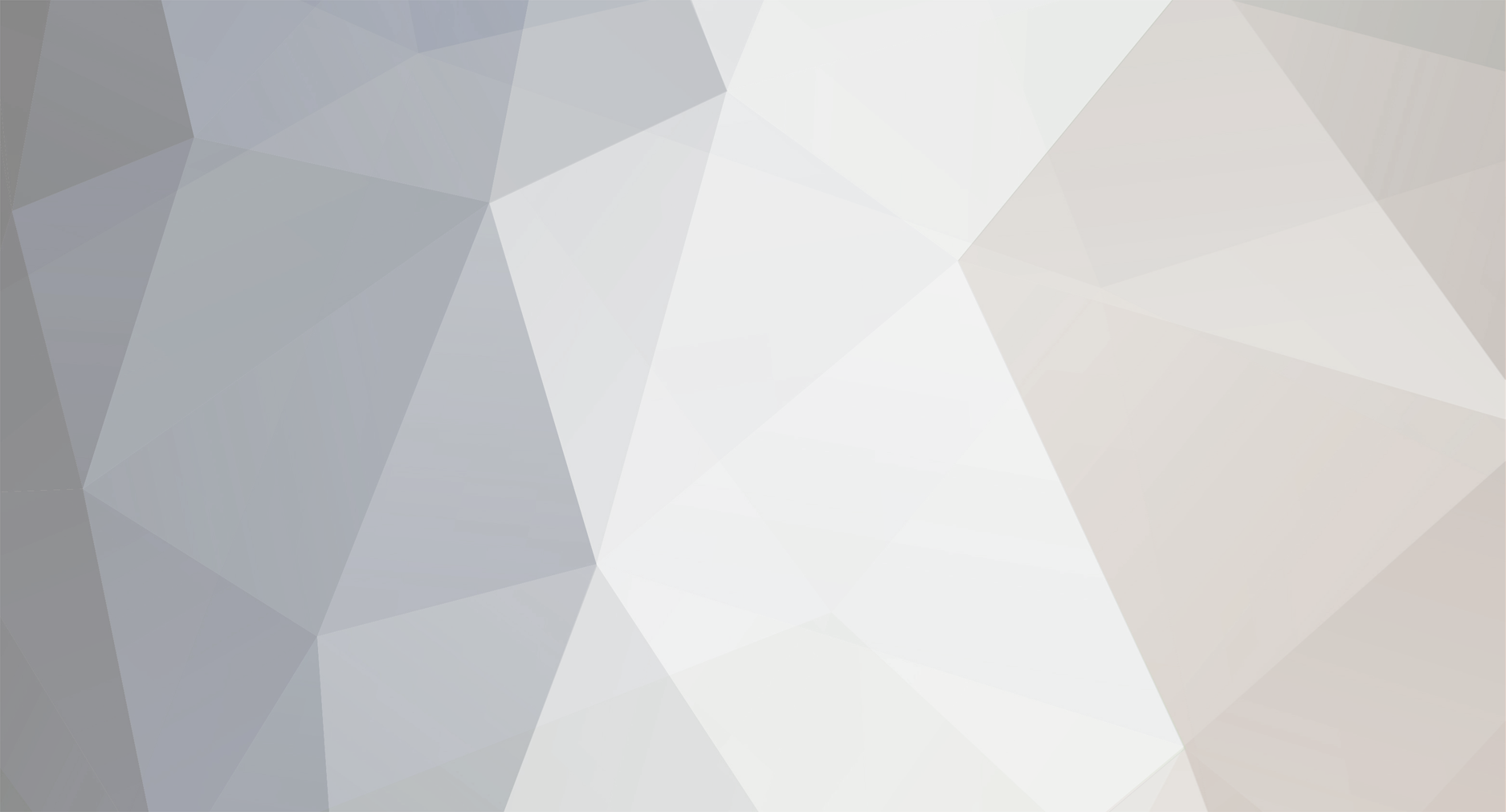
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
Or, put the regex in a variable: $priceRegEx = '/^\s*\d*\.?\d{1,2}\s*$/'; if(!preg_match($priceRegEx, $price1)) { $error[] = "Your price 1 is invalid!"; } if(!preg_match($priceRegEx, $price2)) { $error[] = "Your price 2 is invalid!"; } Even better: change the form to post the prices as an array (i.e. <INPUT type="text" name="price[]"> ... Then use RussellReal's code with a slight change: foreach ($_POST['price'] as $k => $v) { if (preg_match('/^\s*\d*\.?\d{1,2}\s*$/',$v)) continue; $errors[] = 'price is invalid!'; break; }
-
The onsubmit needs to go on the FORM tag not the INPUT tag. <form method="post" action="addMember1.php" enctype="multipart/form-data" onsubmit="return checkCheckBox(this)"> <!-- ALL THE OTHER STUFF IN YOUR FORM --> <b>By submitting, I agree to <a href="http://www.atdolls.com" target="_blank">Contract</a></b><br /> I accept: <input type="checkbox" value="0" name="agree"> <input type="submit" value="Submit form"> </form> Since this form is using METHOD="POST", you have to use $_POST[] to refer to any fields on the form when it is received by addMember1.php. $_POST fields do not magically go to the database, you would have to do that in code, so using POST should not adversely affect the database, unless you have some function that sends all POST fields to the database. One important thing to be aware of, the browser will NOT POST a checkbox if it is not checked. So, if the user has JavaScript turned off and does NOT check the box, the $_POST['agree'] element will NOT exist. You check for this using isset(): if (! isset($_POST['agree'])) { // The user did NOT check the terms box, so do not proceed } else { // OK, they agreed to the terms, continue here } Another note, I would choose a value other than zero for the checkbox. It will not matter with the code I just provided, but it can get confusing. PHP will consider a value of zero as empty and false, so if you instead did: if($_POST['agree']) ... it would be false since your INPUT specifies a value of zero. And if you use if(empty($_POST['agree'])) ... it will be true (empty). In either case, you will not know if the user checked the box or not. I usually use 1 (one) for checkboxes, unless I need a specific value from it. So, yes, you can fix this just in the HTML. But the JavaScript is NOT going to stop someone from submitting the page without checking the box IF they have JavaScript turned off. So, you ALWAYS need to validate your forms server side, no matter how much JavaScript validation you have.
-
When you say "export" are you talking about using your browser to download the file? This line: header("Content-Disposition: attachment; filename=Export.csv"); is a suggestion to the browser to save the file as "Export.csv". However, the browser can do what it wants (usually based on your settings). My experience has been, that, in order to prevent the accidental loss of an existing file, the browser (in Windows at least) will add the "(2)", "(3)", ... to a filename when saving it. I have my browser set to always prompt me for the filename, so I can choose to overwrite, or even rename the file. Unfortunately, I don't think there is any way that you can control this from PHP (and most likely not even from JavaScript). The closest you can come would be to add something to the filename you put in that header, such as the Year-Month-Day (i.e. Export20110309.csv) so when you see the files in your download folder you know at least when each was downloaded. Your other choice is to change your browser settings to always prompt you for a filename when downloading files.
-
Storing dates as varchar or text is a bad idea. It makes it very difficult to query based on the date or manipulate the date. If possible, you should consider using the DATE datatype for date columns. However, with things the way they are, you can use DATE_FORMAT to convert the CURRENT_DATE() into a string. DATE_FORMAT(CURRENT_DATE(), '%c-%e-%Y') Unless you are using two-digits for the month and day, then it would be DATE_FORMAT(CURRENT_DATE(), '%m-%d-%Y') Those would give '1-8-2011' and '01-08-2011', respectively. To get the number of times a particular user shows up, the query would be: SELECT COUNT(*) FROM totals WHERE date = DATE_FORMAT(CURDATE(), '%c-%e-%Y') AND username = 'whatever' See the mySql manual for DATE_FORMAT() But, if at all possible, consider changing the datatype for the date column in your database. If you ever need to query for a range of dates, a string date is extremely difficult to use.
-
It kind of depends on how your date is stored. But you'll need to use something like this: SELECT COUNT(*) FROM totals WHERE date = CURDATE()
-
Oops, good catch sasa. Those backticks always screw with my head. I usually just alias the expression and order by the alias (and I never use backticks): SELECT page, COUNT(like) AS Cnt FROM feedback GROUP BY page ORDER BY Cnt DESC LIMIT 10;
-
Your ORDER BY is ascending, so you are getting the least liked pages first. Change the ORDER BY to DESCending to get the most liked pages first. $sql = "SELECT `page`, COUNT(`like`) FROM `feedback` GROUP BY `page` ORDER BY `like` DESC LIMIT 10";
-
You are adding the click function to remove_game_type during the document ready function. However, you have not added that DIV to the document yet, so there is nothing to add the click event to. JQuery has a way to cause that event assignment to affect all elements with the specified identifier that are created later, but I don't know the correct syntax or even what it is called. I don't think I even stated that well. One thing to watch out for. The way you have that written, ALL of our "X" DIVs will have that same exact ID. This is not valid in the DOM. The IDs must all be unique. I think you want to append the count to the DIV ID like you did for the field names. If you do that, you can move the click function assignment up into the add_game_type function, so immediately after creating the element, you assign the click function to it.
-
If you are looking for the count for an individual user (say ID 3), I would use something like this: SELECT COUNT(*) AS Cnt FROM partners WHERE 3 IN (user_id, friend_id); This is going to do a table scan. If you are looking for the count for ALL users, I would try something like this: SELECT user_id, SUM(Cnt) AS Total FROM ( SELECT user_id, count(*) AS Cnt FROM partners GROUP BY user_id UNION ALL SELECT friend_id, count(*) FROM partners GROUP BY friend_id ) AS U GROUP BY user_id; This may do two table scans; or, if user_id and friend_id are both individual indexes, this may just scan the indexes. If you are looking for an individual user and don't want to do a table scan; if user_id and friend_id are indexes, use the second query but with a WHERE clause inside and no need for the GROUP BY: SELECT SUM(Cnt) AS Total FROM ( SELECT count(*) AS Cnt FROM partners WHERE user_id = 3 UNION ALL SELECT count(*) FROM partners WHERE friend_id = 3 ) AS U; Of course, this requires version 5.something of mySql to use the SELECT as a psuedo table.
-
The ONSUBMIT attribute that was in the second form tag (the one you removed), needs to be added to the first form tag (the one you kept). Also, be aware that the checkbox will now be in $_POST instead of $_GET. If that doesn't solve it, paste your code as you have it now so we can see what we are working with.
-
The code looks OK. You might want to try just using new-lines ("\n") instead of the Carriage-return--new-line pair ("\r\n"). I usually just use PHP_EOL because it is defined as the appropriate line ending based on the system (unix/linux uses just \n while Windows uses \r\n). However, I can't remember what the mail specification says (I'm thinking just new-line). So your message might be: $message = "Someone just filled out the online order form! Here's there info:" . PHP_EOL; $message .= "CUSTOMER INFORMATION" . PHP_EOL; $message .= "Renters Name - {$_POST['rname']}" . PHP_EOL; A couple of notes. [*]The variables (i.e. $_POST) will ONLY be interpreted inside a double-quoted string. The code you posted is correct, but if you are using single quotes, in the actual script, it will not work (the "\r\n" will also ONLY work in double-quoted strings); [*]Edit out your email address when you post code. I'm sure you don't want bots picking it up and spamming you; [*]The address used for the From: header REALLY needs to be a valid email address on the server sending the email. Some servers will not send an email if the From does not belong to them (it looks like someone is forging mail); and some (most) servers will mark a message as spam if the From does not match the actual sending server. [*]If the user provides an email address, and you want it in the email you get, add a Reply-To: header with their address. Then if you click reply, it will go to them. Of course, once you reply, they will have YOUR email address that you send it from.
-
There are a couple of ways to make a multiple line message. You can just put a CRLF (or just a LF) at the end of each line: $message = "Line 1\r\n"; $message .= "Line 2\r\n"; Or you can open the quotes on one line and close them on another: $message = "Line 1 Line 2 "; You can use a HEREDOC $message = <<EOT Line 1 Line 2 $_POST['from_form'] EOT (or something like that) To add form fields, you can concatenate or place the variable inside a double-quoted string; $message = "Line1 " . $_POST['from_form'] . PHP_EOL; $message .= "Line 2 - {$_POST['fieldName']}" . PHP_EOL;
-
That is probably a mail server issue. It would make sense, to some degree, not to send extra emails. So, yeah, I could see the mail server deciding to send only one email if both addresses are the same. Since you got it to work with two different email addresses, it appears that the PHP mail() function is working as expected.
-
Dropdown has value prior to IF statement, another value within IF
DavidAM replied to PCurry's topic in PHP Coding Help
You assigned the value to it: if(($year_search="select")||($owner_search="select")) A single equal-sign "=" is assignment -- It takes two to test "==" if(($year_search=="select")||($owner_search=="select")) (or three "===" for equivalence, meaning same value and same datatype - does not apply here) -
You have two separate forms. Put everything in a single form. Then handle it in the addMember1.php page. <br/> <input TYPE="submit" name="upload" title="Add data to the Database" value="Submit Form"/> <!-- TAKE OUT THESE TWO LINES - AND THE SUBMIT BUTTON DOWN BELOW --> </form> <form action="/yourscript.cgi-or-your-page.html" method="GET" onsubmit="return checkCheckBox(this)">
-
That should work. I'm pretty sure I've used it that way before. There are a couple of things to try: 1) Remove the carriage-return - the spec requires it but there are a few mail servers that do not handle it correctly. 2) Try swapping the From and the BCC sections 3) Try putting a CRLF pair after the From header Actually, try #3 first: mail( $email_to, "Feedback Form Results", $message, "BCC: [email protected]\r\nFrom: $email\r\n" ); You know that is a backslash (before the "r" and the "n"), right? (don't mean to be insulting, just checking the obvious).
-
You can add it as a BCC header: mail( $email_to, "Feedback Form Results", $message, "BCC: [email protected]\r\nFrom: $email" ); This way the other person should not see your email address or know you received a copy of it. You could use CC: or TO: as well, but then the person you are sending it to would see your address.
-
You seem to have joined the people table twice. Try this: $query = "SELECT COUNT(*) as num FROM constructs INNER JOIN people ON (people.id = constructs.pt_id) WHERE constructs.signstat = 'y' "; $total_pages = mysql_fetch_array(mysql_query($query)); $total_pages = $total_pages[num]; Although I don't recommend combining the fetch and query in a single call. The error message indicates that mysql_query() failed and with it embedded in the call to mysql_fetch_array() you can't catch it and take action. Also, you are trying to reference the field name in the $total_pages array, but you fetched it as a numeric array. I would rewrite the whole thing like this: $query = "SELECT COUNT(*) as num FROM constructs INNER JOIN people ON (people.id = constructs.pt_id) WHERE constructs.signstat = 'y' "; $res = mysql_query($query); if ($res === false) { // In development output some stuff to help fix the problem // But don't leave this in production die(mysql_error() . PHP_EOL . 'SQL: ' . $query); } $total_pages = mysql_fetch_assoc($res); // Use fetch_assoc() so we get the column names as array keys $total_pages = $total_pages['num']; // num is a string, it needs to be in quotes EDIT: Apparently mysql_fetch_array() returns the data with both numeric indexes and column name indexes. I still recommend using mysql_fetch_assoc() specifically; but, to each his own.
-
It is an attempt to inject an iframe into your page. If this came from a user input and you put it in your database, and then write it to a page for another user, it would put an iframe on the page and make it look like it was your page (assuming it landed inside a SCRIPT tag). Seems a poor attempt, since the href is local to your site -- unless they were trying to expose some of your code to try to get your database info. Of course, it's still a poor attempt since the page specified would have been processed normally, or would have produced a 404. Or maybe it was a test to see if your site was susceptible to injection attacks. It decodes to: <iframe style="width:100%; height:820px; border:0px" scrolling="no" border="0" src="main.php"></iframe> So, did you pass the test?
-
Have you considered using an IFRAME? I don't have much experience with it, but I think it will do what you want.
-
Sorry, I don't know much about IFRAME. I played with it once, just to try to understand why the W3C would throw-away a perfectly good element (FRAMESET) and create the IFRAME. I still don't understand. Personally, I prefer the FRAMESET. I'm sure someone else with more experience will chime in here.
-
failed to open stream: No such file or directory in
DavidAM replied to ketchumdev's topic in PHP Coding Help
sorry, I didn't read it that way. -
Sorry, I should have explained more. FRAMESET (which has been depricated) actually REPLACES the body. Each FRAME in the FRAMESET creates a separate "document" to show. If the FRAMESET is shown in the browser, it will NOT also show the BODY element. In fact, I don't think it is valid to have a BODY in an HTML file with a FRAMESET (except possibly in the NOFRAMES section). If you are set on using FRAMESET, the menu stuff (that you currently have in the BODY) needs to be included using a FRAME that references a separate file. If you want to use an IFRAME, forget the whole FRAMESET thing, build the BODY as you want it using an IFRAME where you want the random site loaded - stick it in just like you would an IMG tag.
-
failed to open stream: No such file or directory in
DavidAM replied to ketchumdev's topic in PHP Coding Help
Do NOT use include('http:// ... Where is the connections folder in relation to login.php. Is it in the same directory? or is it in one of the directories above it? By putting a slash at the beginning of it, you are telling PHP to look in the root of the file system of the server. You can pretty much bet that it is NOT there. If connections is a folder in bocce (where login.php is), take the leading slash off. Otherwise, post your folder hierarchy, and we'll see what we can do. -
Insert into multiple tables linked by foreign keys (PHP, MySQL)
DavidAM replied to endouken's topic in PHP Coding Help
Do NOT duplicate data to use as a primary key, that is just a WASTE of storage and processing. Do NOT use random values for the primary key, as you said, you will eventually run into duplicate issues. Do NOT use VARCHARS for the primary key, unless you have a very compelling reason to do so. Use the auto_increment for the primary key unless the data has some unique component that will work (i.e. phone number) and even then, you would have to have a compelling reason - what do you do if someone does NOT have a phone, or they change their number? In your original code, you need to look at a few things. Why are you specifying the string "custid" for the custid? It is an integer and a string is invalid. mySql seems to just use the column's default when assigned an invalid value (a stupendously bad idea IMO). Also, you were supplying a string for the custid in the second table. Since you have defined this column as allowing NULLs, the server is using the default - which is NULL. Since this field is a foreign key, it should NOT allow NULL unless it is possible to have a customer address without a customer. Finally, use the LAST_INSERT_ID() function to get the custid when inserting the address. The two queries you want to execute are: $insertintocustomerdetail = "INSERT INTO customerdetail VALUES (NULL,'$firstname','$surname','$dob','$permissionnewsletter','$email','$userpassword','$telephone',now())"; mysql_query($insertintocustomerdetail); $addressline1=$_POST['addressline1']; $addressline2=$_POST['addressline2']; $cityortown=$_POST['cityortown']; $county=$_POST['county']; $postcode=$_POST['postcode']=strtoupper(@$_REQUEST['postcode']); $insertintoaddresstable = "INSERT INTO addresstable VALUES (NULL,LAST_INSERT_ID(),'$addressline1','$addressline2','$cityortown','$county','$postcode',now())"; mysql_query($insertintoaddresstable); By the way, you need to sanitize those inputs. You are leaving yourself open to SQL injection.