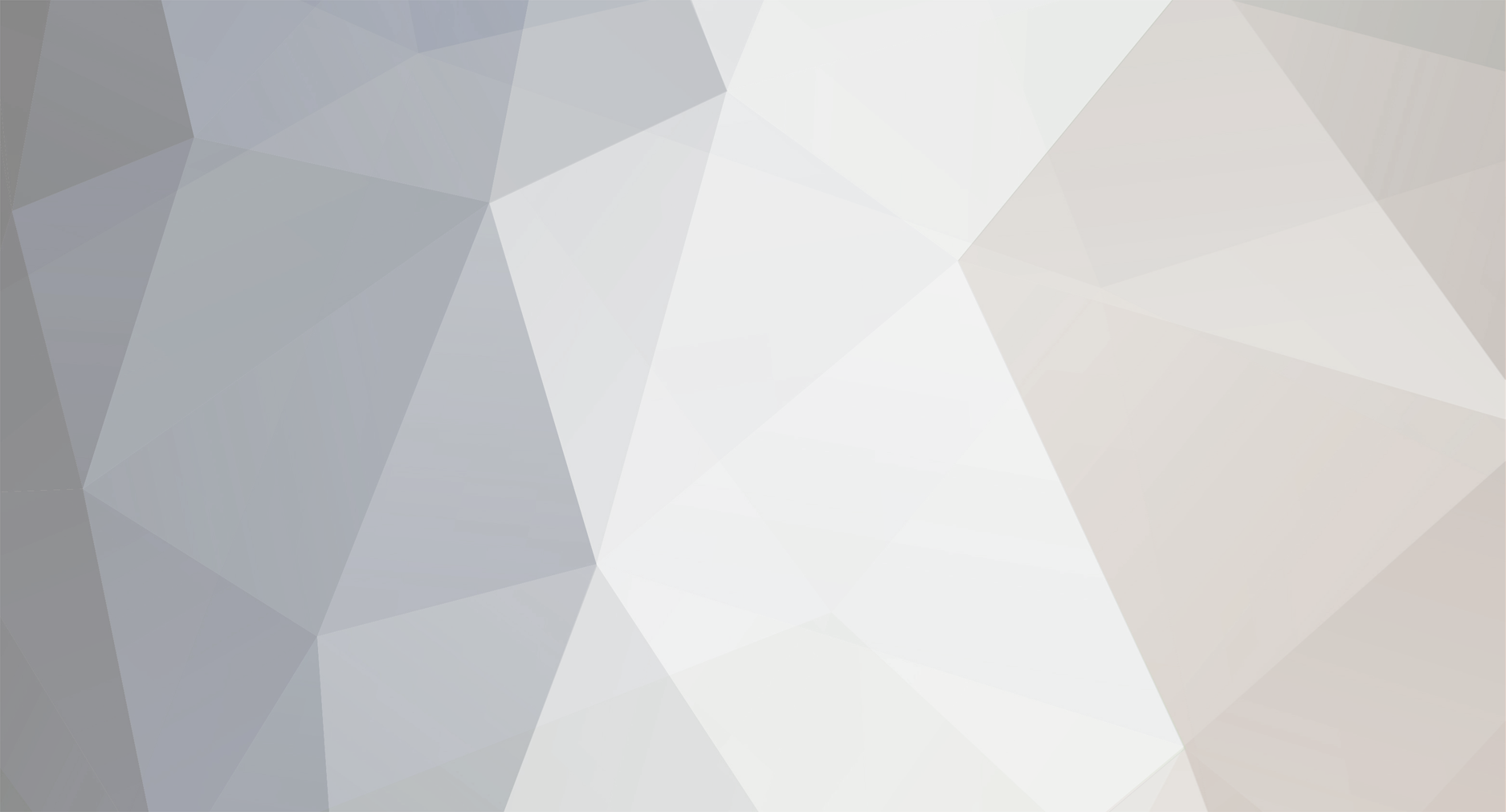
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
What are the contents of the other elements of $_FILES when this happens? Specifically, "error" and "size"? It could be that the uploaded file exceeded the server's allowed size or failed in some other way. The "error" element will give you an indication of why it failed (see the manual). You should always check the "error" element before trying to move an uploaded file. if ( (!empty($_FILES)) and ($_FILES["error"] == UPLOAD_ERR_OK) ) { If the "error" is zero and the "size" is NOT zero, I would check whether the mkdir() worked and the permissions are correct. This does not seem to be the problem since it "usually works" and tmp_name is empty, but I don't know what else could be wrong.
-
It sounds like you want to determine if the url entered by a user is already in the database, so you don't enter a duplicate. Since domain.com and www.domain.com could, in fact, be different sites, just normalizing the url may not be the correct solution. Go to the address bar of your browser and type google.com (no http, no www, just google.com). When the page finishes loading, look at the address bar, it shows http://www.google.com/ (this is the effective url). If I was going to attack this, I would start by using curl to issue a HEAD request (is that possible? if not, use GET) for the address entered by the user. If the request fails, then the user's entry is invalid. If the request succeeds, you should be able to retrieve the EFFECTIVE URL from the curl information. This is the url I would store in the database. I would try it with HEAD first, because this will use less bandwidth on your server and the destination server. This is just out of common courtesy and so there is less returned data to deal with. If I could not get this to work with curl (and some help from phpFreaks), then I would take a look at parse_url() to break down the user's entry and normalize the url before entering it in the database.
-
I would define the checkboxes and emails as arrays using $i as the key: ."<td width='5px';><input type='checkbox' name='transcheck[".$i."]'></td>" ."<td id='parent".$i."A'>".$arr[0]." ".$arr[1]."</td>" ."<td id='parent".$i."B'>".$arr[2]."</td></tr>" ."<tr style='display:none'><input type='hidden'; name='transemail[".$i."]'; value='".$arr[9]."'></tr>"; then you can use a simple foreach loop: foreach ($_POST['transcheck'] as $key => $value) { /* We don't have to check the $value since checkboxes are only in POST if the user actually checked them */ echo $_POST['transemail'][$key]; } of course, you probably should not be putting email addresses on the form. Hidden fields are only hidden on the rendered page. If you view the source, you will see them. Bots love this stuff. It also leaves you open to XSS injection. You should really be using the unique ID from the database and then retrieve the email addresses from the database in the destination script.
-
use /C instead of /K
-
If you return a comma-separated list of ID's from, say, a function; you could then use PHP to put it into an SQL statement. $ids = '1,12,6'; $sql = "SELECT * FROM Places WHERE ID IN ($ids)"; (don't put quotes around $ids.) of course, I'm not sure how to get it sorted with an ORDER BY. I'll have to think about that.
-
Have you considered using the built-in PHP Tokenizer? It takes some work to understand the results, but it parses PHP into an array that you might be able to use.
-
On the display of the web page, the THEAD will appear at the top of the table. When the page is printed, if the browser decides to split the table across multiple pages, it will print the THEAD at the top of the TABLE on each page. By the way, there is no way you will ever determine the size that the page will be when printed. Firefox (and probably others) gives me the ability to scale the output to however I choose 50%, 80%, 120%, whatever. So, even if you figure the size of your page, you don't know the size of the printout. If you need absolute print formatting, then generate a PDF. If you just want to make a user's printing of your page more friendly, there are some (very few) HTML and CSS elements that will help. It is unfortunate that the HTML specification does not give us more print layout capabilities.
-
I recently built an internal application that shows several tables on a single web-page. Since this is an INTERNAL application, I did not test any of the following in ANY browser other than the one I use (FireFox). So, this works in FireFox, but I don't know about any other browsers. 1) If an HTML table is longer than the printed page, the "<THEAD>...</THEAD>" section is repeated on each page. HTML also has a "<TFOOT>" (I believe) that may do the same thing. I did not use it, so I can't say if it works. <TABLE border=1> <THEAD> <TR><TH colspan=2>Hours</TH><TH>Job</TH><TH>Sat</TH><TH>Sun</TH> <TH>Mon</TH><TH>Tue</TH><TH>Wed</TH><TH>Thu</TH><TH>Fri</TH> <TH>Total</TH><TH class="ot">Reg</TH><TH class="ot">OT</TH> </TR></THEAD> <TR> TABLE DETAIL GOES HERE </TR> 2) To force a page break at a specific spot (for me each table needed to start on a new page). I added a block element (I used "<H2>") to the webpage. I assigned a CSS class to this element so that it is NOT displayed on the screen; and another CSS class so that it causes a page break. <H2 class="MDnoScreen pageBreak">Hours Report: Jan 15, 2011 - Jan 28, 2011</H2> /* CSS Page Break for Printing */ .pageBreak { clear: both; page-break-before: always; } /* SCREEN */ @media screen { .MDnoScreen {display: none;} /* Do not show on screen (it is for the printer) */ I hope this helps. You can google for the "page-break-before" attribute and for the THEAD/TFOOT elements for more detail.
-
If you are trying for sequential numbers, shouldn't that be 44.01, 44.02, 44.03 ... 44.09, 44.10, 44.11? Otherwise they are just strings.
-
To retrieve the tree levels, you have to join the table to itself. But it cannot be done in a single statement for infinite levels. To get three levels, you would do something like this: Select T1.name, T2.name, T3.name FROM table T1 JOIN table T2 on T1.parent = T2.id JOIN table T3 on T2.parent = T3.id A good way to do this is to write a stored procedure or function to retrieve the complete tree. Here is a function I wrote recently for a similar table. Using a function, you can retrieve a string containing the entire tree. DROP function IF EXISTS `get_place_text`; DELIMITER $$ CREATE FUNCTION `get_place_text`(id$ INTEGER) RETURNS varchar(1024) READS SQL DATA BEGIN DECLARE out$ varchar(1024); DECLARE pln$ varchar(1024); DECLARE pli$ integer unsigned; -- Initialize the output and loop variables SELECT '', id$ INTO out$, pli$; -- As long as we have a PlaceID get it's name and the ParentID WHILE (pli$ != 0) DO SELECT PlaceName, ParentID INTO pln$, pli$ FROM Places WHERE ID = pli$; -- Concatenate the name with the output variable SELECT CONCAT_WS(out$, pln$) INTO out$; END WHILE; RETURN out$; END $$ DELIMITER ; -- Use it like this SELECT EventDate, get_place_text(PlaceID) AS PlaceName FROM table WHERE EventID = 1;
-
Mysql import creates unwanted carriage return. How to remove?
DavidAM replied to dombrorj's topic in MySQL Help
fgets() includes the newline character at the end of the input line. You can trim() the value to get rid of it. // trim() IT HERE $line = trim(fgets($fh)); if($line){ echo "Importing value: $line <br />"; mysql_query("INSERT INTO `master` (`numberid`) VALUES ('$line')"); -
Undefined Constant.. and Undefined Variable :?
DavidAM replied to ruletka's topic in PHP Coding Help
You need quotes around the array element names. Otherwise, PHP thinks they are constants (which have not been defined). $results = mysql_query("SELECT * FROM `pets_list` WHERE `id` = '{$row['petid']}'"); $results2 = mysql_query("SELECT * FROM `users` WHERE `id` = '{$row['adopter']}'"); -
The query is executed on the first line of the code snippet: $query = mysql_query("SELECT * FROM `users` WHERE `username`='$username'"); The problem is that you are using while ($row = ...) twice on the same result set. The first WHILE loop has already processed ALL records, so the second while loop is not finding any data and is skipping the entire body of the loop. If you are expecting only ONE row from the query, you do NOT need to use a WHILE loop at all. Check to see if the query was successful, then fetch the data into $row (or whatever) and go from there.
-
See my comments in your code: /* You are only selecting "id" here, so the other fields are not available Also, you are comparing the database (hashed) password against a hash of the (clear text) password so if you get ANY rows at all, then the user is validated */ $query = "SELECT `id` FROM `cysticUsers` WHERE `Email` = '$email' AND `Password` = 'SHA1(CONCAT(`salt`,'$password'))' AND Status = 'active' LIMIT 1"; $request = mysql_query($query,$connection) or die(mysql_error()); /* Take out the "@" - it may be hiding errors. Don't use @ to hide errors, let the errors show IN DEVELOPMENT and fix them */ if(@mysql_num_rows($request)) { /* The only thing that will be in $row now, is "id", that is ALL that you selected if you want more data, add it to the SELECT statement */ $row = mysql_fetch_assoc($request); /* You have already done this comparison in the SELECT statement. If you get here, then the user has been validated - this IF is NOT needed Also, you did NOT select "salt" or "Password" so those elements are NOT in the $row array, you should be getting error (warning) messages here if you have error reporting turned on. */ if (sha1($row['salt'] . $_POST['password']) === $row['Password']) { /* Indent your code consistently, so you can see what is supposed to happen */ $_SESSION['CLIFE']['AUTH'] = true; $_SESSION['CLIFE']['ID'] = $result['id']; // UPDATE LAST ACTIVITY FOR USER $query = "UPDATE `cysticUsers` SET `LastActivity` = '" . date("Y-m-d") . " " . date("g:i:s") . "' WHERE `id` = '" . mysql_real_escape_string($_SESSION['CLIFE']['ID']) . "' LIMIT 1"; mysql_query($query,$connection); if(!empty($_POST['return'])) { header("Location: " . $_POST['return']); /* ALWAYS put an exit() statement immediately after a header() redirect. otherwise, the script will continue to execute until the browser acts on the redirect */ }else{ header("Location: CysticLife-Dashboard.php?id=" . $_SESSION['CLIFE']['ID']); /* ALWAYS put an exit() statement immediately after a header() redirect.*/ } } }else{ $_SESSION['CLIFE']['AUTH'] = false; $_SESSION['CLIFE']['ID'] = false; } } Note: I did NOT make any changes to your code except to add comments about what you need to change. If you copy & paste this code, it will NOT behave any differently.
-
Use a different name for one of the variables. Since all we can see is this small segment of code, I would suggest changing the name of the query result: if (isset($_POST['submitmenuitem'])) { $menuid = (int) $_POST['menuid']; $itemname = trim($_POST['itemname']); $itemnameSQL = mysqli_real_escape_string($dbc, $itemname); $itemurl = trim($_POST['itemurl']); $itemurlSQL = mysqli_real_escape_string($dbc, $_POST['itemurl']); $sortorder = (int) $_POST['sortorder']; $contentpage = mysqli_real_escape_string($dbc, $_POST['contentpage']); $newscategory = mysqli_real_escape_string($dbc, $_POST['newscategory']); $application = mysqli_real_escape_string($dbc, $_POST['application']); $query = 'SELECT *' . ' FROM menuitems' . ' WHERE menu_id = ' . $menuid . ' AND (itemname = "' . $itemnameSQL . '"' . ($itemurlSQL ? ' OR itemurl = "' . $itemurlSQL . '"' : '') . ($contentpage ? ' OR contentpage_id = ' . $contentpage : '') . ($application ? ' OR application_id = ' . $application : '') . ($newscategory ? ' OR newscategory_id = ' . $newscategory : '') . ')'; # CHANGE THE NAME HERE $Qresult = mysqli_query ( $dbc, $query ); // Run The Query echo $query; # AND CHANGE THE NAME HERE if (mysqli_num_rows($Qresult) == 0) { $query = "INSERT INTO `menuitems` (menu_id, itemname, itemurl, sortorder, contentpage_id, newscategory_id, application_id, creator_id, datecreated, enabled) VALUES ('".$menuid."', '".$itemname."', '".$itemurl."', '".$sortorder."', '".$contentpage."', '".$newscategory."', '".$application."', 1, NOW(), 0)"; mysqli_query($dbc, $query); $result = "good"; } else { $result = ''; # AND CHANGE THE NAME HERE while ($row = mysqli_fetch_array($Qresult)) { if ($row['itemname'] == $itemname) {$result .= 'bad1';} if ($itemurl && $row['itemurl'] == $itemurl) {$result .= 'bad2';} else if ($newscategory && $row['newscategory_id'] == $newscategory) {$result .= 'bad3';} else if ($application && $row['application_id'] == $application) {$result .= 'bad4';} else if ($contentpage && $row['contentpage_id'] == $contentpage) {$result .= 'bad5';} else if ($itemurl && $row['itemurl'] == $itemurl && $itemname && $row['itemname'] == $itemname) {$result .= 'bad6';} else if ($contentpage && $row['contentpage_id'] == $contentpage && $row['itemname'] == $itemname) {$result .= 'bad7';} else if ($application && $row['application_id'] == $application && $row['itemname'] == $itemname) {$result .= 'bad8';} else if ($newscategory && $row['newscategory_id'] == $newscategory && $row['itemname'] == $itemname) {$result .= 'bad9';} } } } You will have to review the rest of your code to see if anything else needs to be changed
-
Look more closely at the example, it does NOT escape the entire SQL statement, only the values being put into the statement. To correct this, you need to build your command something like this: $Command = "INSERT INTO user (Name,Address1,Address2,City,Zip,Country,Phone,Email,AcceptedRules,CreditBalance,Referee1,Referee2,Admin,Pwd,DateOfBirth,DefaultLanguage,SeeSpecialStats) VALUES ("; $Command .= "'" . mysql_real_escape_string($_REQUEST['Name']) . "',"; // Name $Command .= "'" . mysql_real_escape_string($_REQUEST['Address1']) . "',"; // Address1 # AND SO FORTH and then remove the mysql_real_escape_string() function call inside the Execute() method.
-
You will need to take the password out of the WHERE clause. Since you have now hashed them, the password they typed (which is in clear text) will not match the "password" in the database (which is actually a hash of the original password). Take the "password = ..." out of the WHERE clause and check it in PHP (which you are already doing). Your problem is that no rows are being returned by the query.
-
Actually, the problem is here: $result = ''; while ($row = mysqli_fetch_array($result)) { You have assigned an empty string to $result. It is not a query result anymore, so you can't use the mysqli functions against it. It looks like you are trying to use the same variable name for two different purposes. That's a bad idea, and it is impossible when the lifetime of the values overlap.
-
Extracting keyword information from Google cookie
DavidAM replied to MortimerJazz's topic in PHP Coding Help
Perhaps my previous post was not entirely correct. I rarely use scanf(), but I use sprintf() frequently. The documentation for sscanf() says that it uses the same format specifiers as sprintf(). And those "wildcard" expressions and not part of the sprintf() specification. However, I have just read through all of the user comments on sscanf() and they indicate that such expressions are usable; although I cannot find any documentation on what these "wildcard" specifiers are or how they are interpreted. If your cookie value is what you showed (earlier) in the image, then the print_r() output indicates that the sscanf() format is not being interpreted as you expect it to be. I don't have an environment here at work to do any testing, but I think the sscanf() specification is the culprit here. -
Extracting keyword information from Google cookie
DavidAM replied to MortimerJazz's topic in PHP Coding Help
I don't think this is correct: $values = sscanf($cookie, '%d.%d.%d.%d.utmcsr=%[^|]|utmccn=%[^|]|utmcmd=%[^|]|utmctr=%[^|]'); sscanf() parses a string based on placeholders. The "%d" placeholders indicate you are looking for integers there - that's fine. However, "%[^|]" is not a sscanf() placeholder. That looks more like a regular expression, which is not valid in a sscanf() call - well, at least not to my knowledge. You need to either change those, or change the first part and use preg_match(). -
According to the manual, empty() will not generate an error if the variable is not set (and I have never seen it generate one):
-
"table" is a reserved word in mySql. To use it as a column name, you need to surround it with back-ticks: AND `table` = 'basic'
-
Since you want the last purchase date for each customer, using LIMIT is not the way to go. Try a GROUP BY - and you don't need LEFT joins here SELECT b.book_title AS Title, b.book_price AS Price, c.customer_name, MAX(p.purchase_date) AS LastPurchase FROM purchases p JOIN customers c ON (p.customer_id = c.customer_id) JOIN books b ON (p.book_id = b.book_id) WHERE p.book_id = $id GROUP BY b.book_title, b.book_price, c.customer_name
-
Yeah, HTTP does not post checkboxes that are not checked. I guess the easiest thing to do is to delete the entries that are not in your posted array: $allTags = array(); foreach($_POST['tag'] as $k=>$l){ $tag = mysql_real_escape_string($_POST['tag'][$k]); // Collect all the tags - escaped and quoted - in an array // this just simplifies the implode() later $allTags[] = "'$tag'"; $check_tags = mysql_query("SELECT * FROM file_cats WHERE category='$tag'") or die(mysql_error()); $num_tags = mysql_num_rows($check_tags); if($num_tags==0){ $add_tag = mysql_query("INSERT into file_cats (fileID, category) VALUES('$fileID', '$tag')") or die(mysql_error()); } } // Delete all tags EXCEPT the ones we just added if (! empty($allTags)) { $sql = "DELETE FROM file_cats WHERE fileID = $fileID AND category NOT IN (" . implode(','$allTags) . ")"; mysql_query($sql); } NOTE: Your SELECT query seems to be missing AND fileID = $fileID - maybe you were just typing fast for the post, or maybe your code is not checking what you think it is checking.