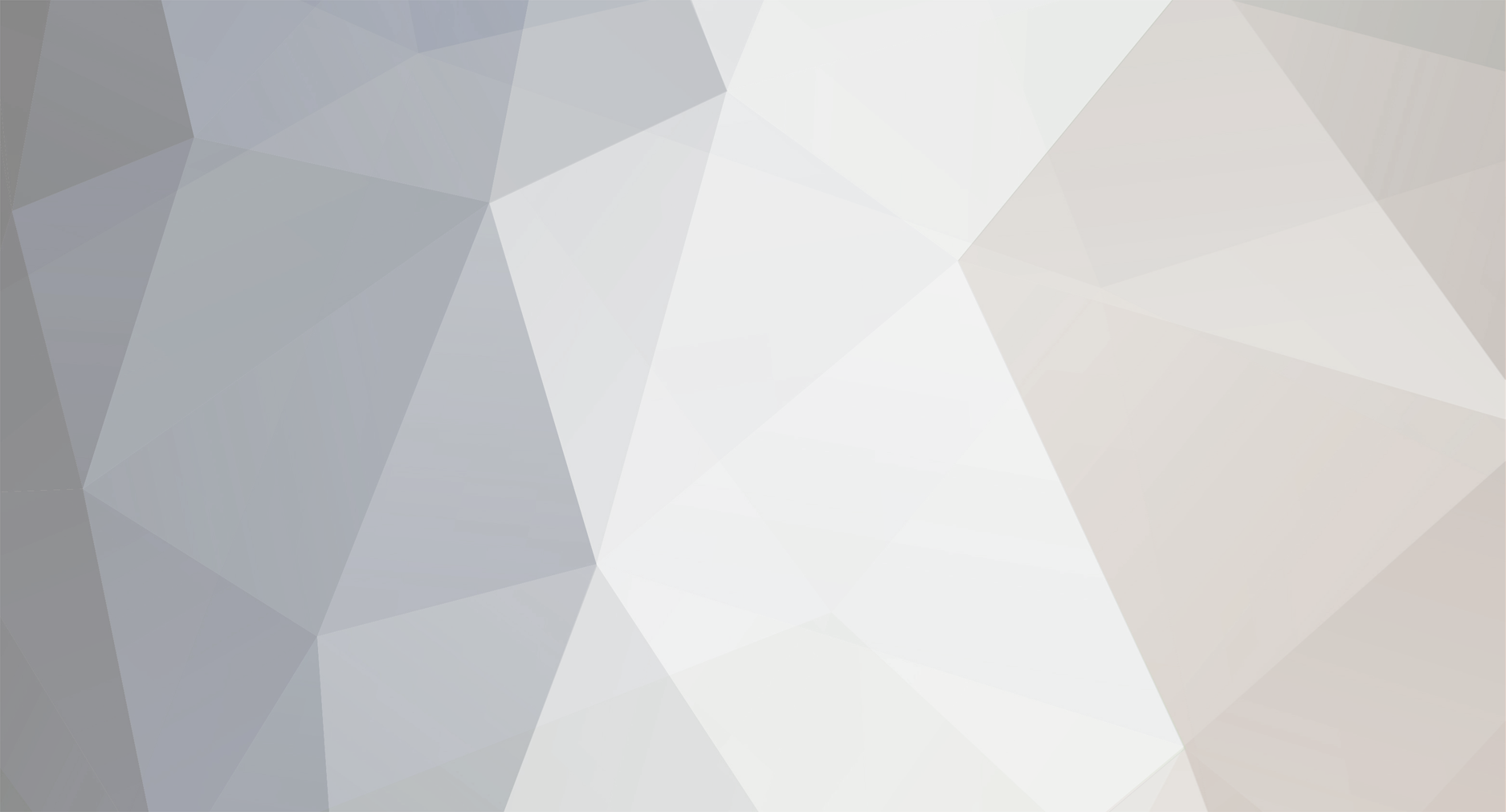
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
Are you really putting the Admin Password in a link on every page? You realize that everyone who sees that page will be able to see the password, right? <div id="globalLink"> <a href="admin.php?cmd=manage&username=admin&password=$adminpw" id="gl1" class="glink" onmouseover="ehandler(event,menuitem1);">Users</a> <a href="admin.php?cmd=editTemplate&username=admin&password=$adminpw" id="gl2" class="glink" onmouseover="ehandler(event,menuitem2);">Templates</a> <a href="admin.php?cmd=mysqlBackup&username=admin&password=$adminpw" id="gl3" class="glink" onmouseover="ehandler(event,menuitem3);">Database</a> <a href="admin.php?cmd=paymentLog&username=admin&password=$adminpw" id="gl4" class="glink" onmouseover="ehandler(event,menuitem4);">Payment</a> <a href="admin.php?cmd=profileFields&username=admin&password=$adminpw" id="gl5" class="glink" onmouseover="ehandler(event,menuitem5);">Setup</a> <a href="admin.php?cmd=changeAdminpw&username=admin&password=$adminpw" id="gl6" class="glink" onmouseover="ehandler(event,menuitem6);">Preferences</a> <a href="admin.php?cmd=logout&username=admin&password=$adminpw" id="gl7" class="glink" onmouseover="ehandler(event,menuitem6);">Logout</a> </div>
-
FRAMESET and IFRAME are two different things. A FRAMESET is the body of a document (without BODY tags) an IFRAME is an embedded element similar to an IMG.
-
Then you have to process all (both) of the result sets. The logic would look something like this: [*]mysqli_multi_query($sql) - [Returns a boolean - false on failure] [*]mysqli_store_result() -or- mysqli_use_result() [*]mysqli_more_results() [Returns a boolean - true if there are more] [*]mysqli_next_result() [*]Repeat from #2 until more_results() returns false If you need more specifics, post the code from $db->query() and $db->getOne().
-
In PHP 5, you can use a reference in the foreach: $cars[0]="Saab"; $cars[1]="Volvo"; $cars[2]="BMW"; $cars[3]="Toyota"; foreach ($cars as $key => &$value) // <-- $value is a reference { $value = "TEST"; echo $key . " " . $value . "<br />"; } You have to watch out though. After the loop, $value still references the last element of the array. So if you use $value again, you could overwrite the array value. It is best to unset $value immediately after the loop. $cars[0]="Saab"; $cars[1]="Volvo"; $cars[2]="BMW"; $cars[3]="Toyota"; foreach ($cars as $key => &$value) // <-- $value is a reference { $value = "TEST"; echo $key . " " . $value . "<br />"; } // Get rid of the reference // unset($value); // without this line we're borked. // more code $value = someCalculationFunction(); // NOW we have changed $cars[3]. OOPS! Your other example was running in an infinite loop because of the <= which should have been just <. for ($i=0; $i <= count($cars); $i++) { echo $cars[$i]; $cars[$i] = "2"; } The loop condition is evaluated every time through the loop. When it started count($cars) was 4. After processing $i=3 and incrementing $i; it is now 4 which is LESS THAN OR EQUAL TO 4, so it ran again. This time, we added an element $cars[4]. So the test is $i (which is 4) is less than or equal to count($cars) (which is 5 now), so it continued to run. Since the array indexes start at zero, you need to use $i < count($cars). However, using count() as the condition adds extra work since the array is counted every time the loop repeats. It is more efficient to capture the count before the loop (oh, and use less than): $times = count($cars); for ($i=0; $i < $times; $i++) { echo $cars[$i]; $cars[$i] = "2"; }
-
When you execute a Stored Procedure in mySql. It returns an "extra" resultset. If your procedure does a single SELECT to return a single resultset, mySql returns two. If your procedure just does an UPDATE and returns NO resultsets, mySql returns one. This extra resultset indicates the success (or failure) of the procedure. Basically, a return code. The standard mysql client (mysql_query(), etc) can NOT handle this extra resultset at all. Basically, if you are using the standard client, you have to close the connection after executing a stored procedure and then open a new connection for any future queries. The "improved" mysql client (mysqli object) CAN handle this extra resultset. You have to look at the multi_query method of the object (as well as more_results() and next_result()).
-
Since your first query does not SELECT the price or description, it is not surprising that it does not display the price or description. In your second query: SELECT pid, image, eid, description FROM prints, products WHERE prints.cid = 1 AND prints.pid IN (31,15,22,29,18) ORDER BY prints.pid DESC You are selecting from TWO tables, but you have not described any relation between them. As a result, you are getting a Cartesian product -- every row in your "products" table is matched to every row from "prints" that satisfies the WHERE clause. You need to JOIN the tables in some way. Without knowing your table structure and how the two relate I can only guess at the JOIN. Perhaps, if the "eid" is in both tables, you could write the query like this: SELECT pid, image, products.eid, description FROM prints JOIN products ON prints.eid = products.eid WHERE prints.cid = 1 AND prints.pid IN (31,15,22,29,18) ORDER BY prints.pid DESC But, since you did not report an error from the select, it would appear that "eid" is NOT actually in both tables. So figure out how they relate and apply the appropriate join.
-
Take a look at the first part of your code ... I did some indenting so we can see it more clearly $q = "SELECT * FROM `accountinfo_org` ORDER BY `strikeaction` ASC";//Select all from account table order by strike action ascending . $res = mysql_query($q) or die(mysql_error()); // Query $count = mysql_num_rows($res); // Count for($i=1;$i>=$count;$i++){// For loop while($player=mysql_fetch_array($res)){ // While Loop $id = securevar($player['id']); // ID $user = securevar($player['username']); // Username $q = "UPDATE `accountinfo_org` SET `strikerank` = '$i' WHERE `id` = '$id'"; // Update account set strike action equals $i where account is account. $res = mysql_query($q) or die(mysql_error()); // Get Query if(isset($res)){ // if isset result from query. echo "Strike Rank set to $user as ".number_format($i)."!"; // Echo This. } } } on the second line here, you execute a query and store the RESOURCE in $res. On line 6 (above) you start a while loop retrieving data from that RESOURCE ($res). Then, about 4 lines later, you execute a different query assigning ITS RESOURCE to $res. When the while loop repeats and tries to retrieve data from $res, it is a DIFFERENT RESOURCE and the fetch either fails or returns a row with columns that do not match what you expect in the loop. When you execute another query inside of a loop, you MUST use a DIFFERENT variable for the resource that is returned. NOTE: I did not look at the rest of the code. You need to fix this, and review the rest of your code for similar situations. Good Luck, post back if you have more trouble.
-
You need to GROUP BY category, sub-category. Add this line to the end of that query: GROUP BY c.id, sc.id
-
BelowZero: I'm sorry, I don't know why I put an INSERT statement in the code I posted, it was clear you were doing an UPDATE. I don't know how I mixed that up. I even remember thinking about mjdamato's point about running queries in a loop, but I could not see a straight-forward way to do UPDATE in a batch similar to his INSERT suggestion. The code I suggested should have been: foreach ($_POST['spread'] as $weekID => $games) { foreach ($games as $gameID => $values) { $sql = 'UPDATE schedule SET A_pt_spread = ' . $values['A'] . ', H_pt_spread = ' . $values['H'] . ' WHERE week_id = ' . $weekID . ' and game_id = ' . $gameID; mysql_query($sql); } } I apologize for sending you down that rabbit trail. As to the "never run queries in a loop" statement; you could build a single UPDATE statement from the posted values and run it after the loop. IMO that is an advanced solution and I leave it as an exercise for the user. I will say that the final query would (possibly) look something like this (for 3 games); UPDATE schedule SET A_pt_spread = CASE(game_id WHEN 1 THEN 5 WHEN 2 THEN 6 WHEN 3 THEN 7 END), H_pt_spread = CASE(game_id WHEN 1 THEN 3 WHEN 2 THEN 4 WHEN 3 THEN 5 END) WHERE week_id = 1 and game_id IN (1, 2, 3)
-
I suspect your query is not correct. SELECT * FROM business, businessCat WHERE businessCat.categoryId = '$catId' AND business.businessId = 'businessCat.categoryId' It looks like you are joining the categoryId (from businessCat) to the businessId (in business) (and what are those quotes doing in there?). Shouldn't that be something like this: SELECT * FROM business, businessCat WHERE businessCat.categoryId = '$catId' AND business.businessId = businessCat.businessId (changes in the last line) or, using the JOIN phrase: SELECT * FROM business JOIN businessCat ON business.businessId = businessCat.businessId WHERE businessCat.categoryId = '$catId' If categoryId is a number, then your page should be passing that number, not the name like "Automotive". I'm just guessing here since we don't have the table layouts, but I think this should help. If it doesn't, then post your table layouts (Table name, column names and datatypes) and possibly some sample data, so we can figure it out.
-
Oops! Yeah they should get closed right after the call to mysql_query(). foreach ($_POST['spread'] as $weekID => $games) { foreach ($games as $gameID => $values) { $sql = 'INSERT A_pt_spread = ' . $values['A'] . ', H_pt_spread = ' . $values['H'] . ' WHERE week_id = ' . $weekID . ' and game_id = ' . $gameID; mysql_query($sql); } } Unless, of course, you need to do more work in there.
-
The problem we have here, is that your input fields are not an array. (Well, they are all in the $_POST array, but they are individual entries there), so you have to process each one. To make it even more difficult, when the form is POSTed, the only way you know the week and game IDs is by picking apart the field names; this is going to be a pain. Let's see if we can offer a more flexible solution. First, let's look at what we currently have. The fields where you enter the spreads are posted (in the $_POST array) as -- assuming week 2 of the season -- w2g1Aspread, w2g1Hspread, w2g2Aspread, w2g2Hspread, w2g3Aspread, w2g3Hspread, ... and so on. Let's instead post the whole thing as an array of spreads. Hold on to your hats, this is advanced stuff ... When we generate the fields let's use this code to process the database resultset: while ($row = mysql_fetch_array($result)) { printf('<input type="text" size="4" name="spread[%d][%d][A]">', $weekID, $row['game_id']); printf(" %s vs. %s ", $row['AwayTeam'], $row['HomeTeam']); printf('<input type="text" size="4" name="spread[%d][%d][H]">', $weekID, $row['game_id']); print("<br /><br />"); } Notice the way we build up the name for the INPUT tag. Now, the %-symbols will NOT be in the name sent to the browser. The browser should actually see something like this (again, week 2): <input type="text" size="4" name="spread[2][1][A]"> Team-1 vs. Team-2 <input type="text" size="4" name="spread[2][1][H]"><br /><br /> <input type="text" size="4" name="spread[2][2][A]"> Team-3 vs. Team-6 <input type="text" size="4" name="spread[2][2][H]"><br /><br /> <input type="text" size="4" name="spread[2][3][A]"> Team-5 vs. Team-4 <input type="text" size="4" name="spread[2][3][H]"><br /><br /> Normally, when creating an array with fields, we just put the array brackets "[]" on the end of the name, and the array is sequentially numbered. However, it is permitted to put real values in the array brackets and get those values back. So, when we post this form, we get something like this for $_POST['spread']: Array ( [2] => Array ( [1] => Array ( [A] => 1 [H] => 2 ) [2] => Array ( [A] => 3 [H] => 4 ) [3] => Array ( [A] => 5 [H] => 6 ) ) ) Stick with me, we're almost there ... Notice that the first element of the array is "2", which is our week number. There are several elements of that array, keyed by our game number, and each of those has two elements for Away and Home. So, to stick this in the database, we can do something like this: foreach ($_POST['spread'] as $weekID => $games) { foreach ($games as $gameID => $values) { $sql = 'INSERT A_pt_spread = ' . $values['A'] . ', H_pt_spread = ' . $values['H'] . ' WHERE week_id = ' . $weekID . ' and game_id = ' . $gameID; mysql_query($sql); Hope this helps.
-
First, you will have to define "different" that string is 100% different from the one you supplied ... or it is 100% the same. Or is it somewhere in between? Once you have defined "different", you have to decide how to quantify the difference. To calculate a percentage, you have to be able to count the "differences" and divide. After you have defined and quantified "different", then we can answer the question as to whether or not we can calculate and test the amount of difference using SQL.
-
Dynamic Dropdown question, submit 2 values from dropdown to a form??
DavidAM replied to jroryl's topic in PHP Coding Help
You have all of the hidden fields INSIDE of the SELECT. This is not valid. Move the closing tag for the SELECT so it is output immediately after the last OPTION (before anything else on the form). You can have multiple fields on a form. Each field has to have a name that is unique in the form. This name is the way you refer to the field's value in the $_POST array. A field on the form can return a single value or an array of values. The values you are assigning to your OPTIONS are composed of multiple database fields. You will have to break that value up in the script that receives the POST. The "array of values" does not seem to apply here. If you wanted to allow the user to select multiple OPTIONS, you could define the SELECT to allow multiples and to return an array. -
Dynamic Dropdown question, submit 2 values from dropdown to a form??
DavidAM replied to jroryl's topic in PHP Coding Help
[*]Your combobox is NOT inside the form. Only fields that are defined between the <FORM> and </FORM> tags are POSTed. [*]Your combobox does NOT have a valid name. Only fields with a name attribute are POSTed with the form. [*]You have an extra </SELECT> at the end of the form, that has NO associated openning tag, anywhere. -
This seems to indicate that the system could not find the file that you passed to filemtime(). Since it also shows the filename it was looking for, you can see that there is no directory path specified in the value passed to filemtime(). I think you need to add the directory you are searching to that call: filemtime($dir . '/' . $file)
-
Even if you could do this, it is not a good idea. During the execution of a trigger, the tables being affected are locked (at least the pages containing the rows being modified) and will remain locked until the trigger completes. If you started an external process, and that process hung up, the server could hang. If the process you started, tried to access the data that fired the trigger, it would not be able to because the data is locked. It will wait for the lock to be released, the lock will not be released until after the process is finished, and the process can't finish until the lock is released. This is called a "Livelock", the database server cannot tell that the process will never continue, so it is not a Deadlock. The process would hang until you killed it, which would likely cause the transaction to rollback and the data will NOT be updated. Triggers were invented to insure database integrity. They should not be used for application code. They must be fast and efficient code. A cron job is your best choice. A well written, efficient script will should not be a problem for the server.
-
PHPfreaks actually has a Tutorials Section. Of course, I've never figured out how to submit one, but there are some good tutorials there.
-
When you put string data in an SQL statement, it has to have quotes around it. You put quotes around the literals here: mysql_query( "INSERT INTO $tbl_name (category_Name, ID, test) VALUES ('werwerwer', $internalId, 'werrr')" ); You have to do it here as well: mysql_query( "INSERT INTO $tbl_name (category_Name, ID, test) VALUES ('$category', $internalId, '$category2')" );
-
Oops, sorry about that, you're right the double quotes inside the replacement string did NOT belong there. Glad you figured it out.
-
include (and it's siblings) does NOT return the content of the file. It includes the file in the source code as if it was typed there. You cannot "include" inside a string. Assigning "include(filename.php)" to a string does NOT put the code from that file into the string, and it does NOT put any output from that file (i.e. echo, print, or stuff outside of php tags) in the string. Any code will be parsed and executed and any output will be outputted. From the looks of the code you posted, you need to wrap the stuff inside countertest.php in a function, change the echo (at the end) to a return, include the file early in your script, and call the new function in the string assignment.
-
Have a look at the "e" modifier for replacement strings. function Replace_BB($text) { $bb = array( '@\[u\](.*?)\[\/u\]@ise', // ADDED THE e MODIFIER TO THIS ONE '@\[i\](.*?)\[\/i\]@is', '@\[b\](.*?)\[\/b\]@is', '@\[img\](.*?)\[/img\]@is', '@\[url\](.*?)\[/url\]@is', '@\[url=http://(.*?)\](.*?)\[/url\]@is' ); $html = array( '"checkU(\'$1\')"', // CHANGED THE REPLACEMENT WITH A FUNCTION CALL '<em>$1</em>', '<strong>$1</strong>', '<img src="$1" />', '<a href="$1">$1</a>', '<a href="$1">$2</a>' ); return preg_replace($bb, $html, $text); } /* ADDED Callback function for preg_replace() */ function checkU($text) { if ($text == 'something') return '<u>' . $text . ' Error</u>'; else return '<u>' . $text . '</u>'; } print_r (Replace_BB($_POST['data']));
-
I'm not at the top of my game right now, but if I understand, you only want catID if it contains ALL of the answerID's in the list. Right? Try adding a count: $sql = "SELECT catID FROM $mytable WHERE answerID IN (" . implode(',' , $answerIDArray) . ") GROUP BY catID HAVING COUNT(answerID) = " . count($answerIDArray); If mySQL complains, add COUNT(answerID) to the SELECT list as well; but I don't think mySQL requires that.
-
You could get all of the matchups using a single query and a PHP loop. The query would look something like this: SELECT S.game_id, TH.team_name AS HomeTeam, TA.team_name AS AwayTeam FROM schedule AS S JOIN teams AS TH ON S.H_team = TH.team_id JOIN teams AS TA ON S.A_team = TA.team_id WHERE S.week_id = '1' ORDER BY S.game_id; The key is joining the same table (teams) twice to the schedule; and using an Alias to reference each of those in other parts of the query. So then the PHP code could look something like this: <?php include("opendatabase.php"); ?> <FORM Method = "POST" action ="insert_spreads.php"> <?php $weekID = 1; $result = mysql_query(" SELECT S.game_id, TH.team_name AS HomeTeam, TA.team_name AS AwayTeam FROM schedule AS S JOIN teams AS TH ON S.H_team = TH.team_id JOIN teams AS TA ON S.A_team = TA.team_id WHERE S.week_id = '$weekID' ORDER BY S.game_id;"); while ($row = mysql_fetch_array($result)) { printf('<input type="text" size="5" name="w%dg%dAspread">', $weekID, $row['game_id']); printf(" %s vs. %s", $row['AwayTeam'], $row['HomeTeam']); printf('<input type="text" size="5" name="w%dg%dHspread">', $weekID, $row['game_id']); print("<br /><br />"; } mysql_close($con); ?> <br /><br /> <input type="Submit" value="Submit Spreads"> This is completely untested. It gives you one trip to the database and a tight loop for output. I like to use printf() and sprintf() for HTML (and SQL) strings. But there are other ways to do it.
-
Just to be sure we are all on the same page ... The TIMESTAMP datatype in mySql is different from the DATETIME datatype. The TIMESTAMP appears to be a UNIX timestamp "under the hood". It has the same range limitation as an unsigned time() value, and stores the UTC time. It can be set in the CREATE TABLE statement to automatically update on INSERT or UPDATE or both -- well, the first one in the table can be. The DATETIME datatype does NOT have the unix range limitation, and seems to be based on storing the actual digits entered rather than a "real" time stamp. The manual states: