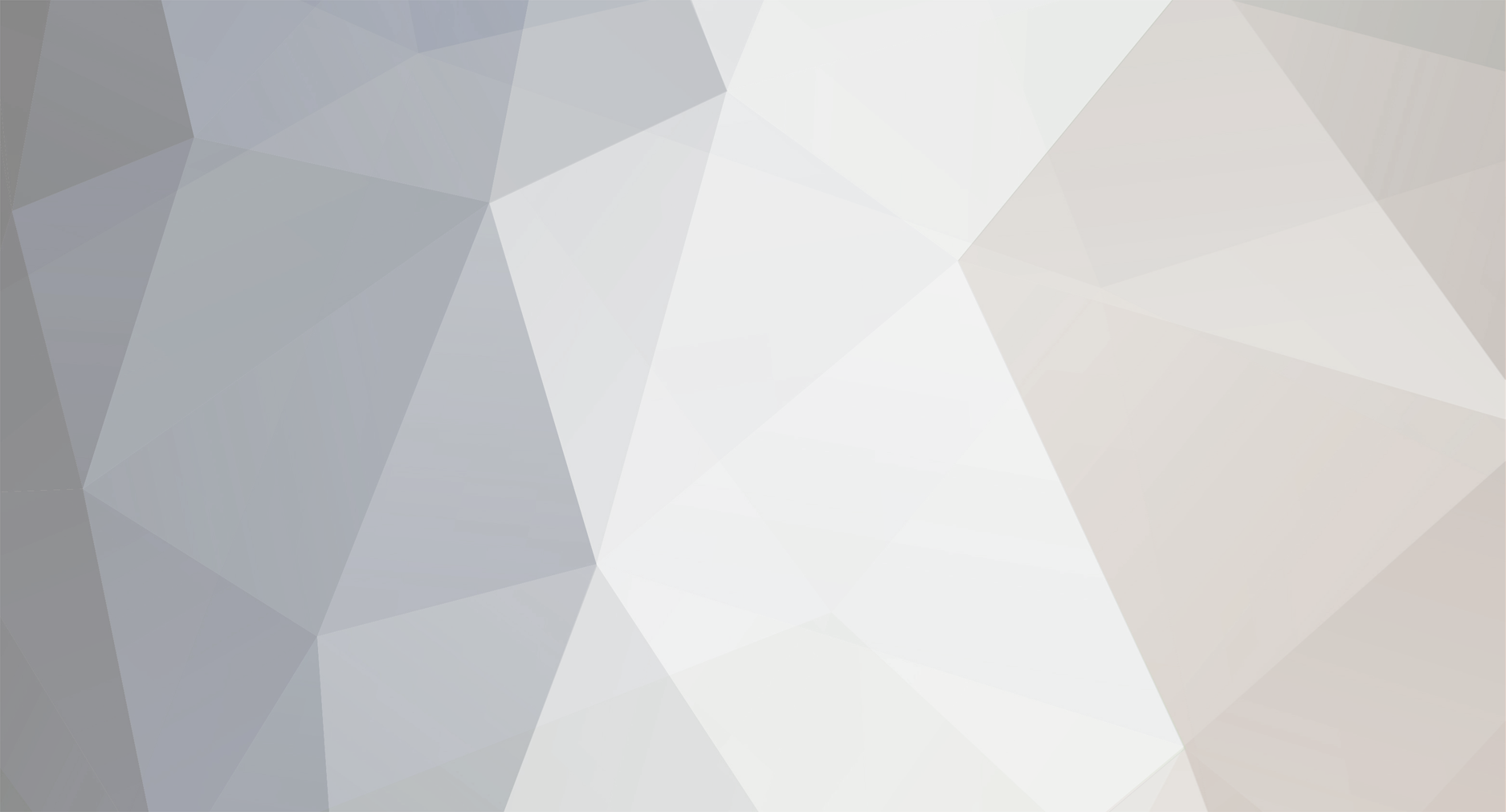
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
How would you solve this engineering problem?
ignace replied to yoursurrogategod's topic in Miscellaneous
RFID increases production costs, where as barcodes provide a revenue stream. Every time the barcode wears off, they'll have to buy new barcoded baseballs. IMHO though I don't see why this would matter I don't care whether I swung ball A out of the stadium or ball B, just that I did. I care more about the machine shooting the balls just like a pitcher would to practice my swing. -
<?php interface FactorRepositoryInterface { const HOUR = 'hour'; const MINUTE = 'minute'; const CELSIUS = 'celsius'; const FAHRENHEIT = 'fahrenheit'; public function getFactor($fromUnit, $toUnit); } class FactorRepository implements FactorRepositoryInterface { private $factors = array(); private function loadFactorTable() { $this->addFactor(self::HOUR, self::MINUTE, 60); $this->addFactor(self::MINUTE, self::HOUR, 1/60); $this->addFactor(self::CELSIUS, self::FAHRENHEIT, function($value) { return $value * 1.8 + 32; }); $this->addFactor(self::FAHRENHEIT, self::CELSIUS, function($value) { return ($value - 32) / 1.8; }); } public function addFactor($from, $to, $callable) { if (!is_callable($callable)) { $factor = $callable; $callable = function($value) use ($factor) { return $value * $factor; }; } $this->factors[$from] = array($to => $callable); } public function getFactor($fromUnit, $toUnit) { $this->loadFactorTable(); if (!isset($this->factors[$fromUnit], $this->factors[$fromUnit][$toUnit])) { throw new RuntimeException('Unknown unit specified'); } return new Factor($this->factors[$fromUnit][$toUnit]); } } interface FactorInterface { public function getFactor(); public function multiply($value); } class Factor implements FactorInterface { private $callable; public function __construct(callable $callable) { $this->callable = $callable; } public function getFactor() { return $this->multiply(1); } public function multiply($value) { $func = $this->callable; return $func($value); } } class UnitConverter { private $factorLookup; public function __construct(FactorRepositoryInterface $factorLookup) { $this->factorLookup = $factorLookup; } public function convert($value, $fromUnit, $toUnit) { return $this->factorLookup->getFactor($fromUnit, $toUnit)->multiply($value); } } $uc = new UnitConverter(new FactorRepository); echo $uc->convert(100, FactorRepository::CELSIUS, FactorRepository::FAHRENHEIT);The callable covers the case where you need the value to get the factor (eg celsius to fahrenheit).
-
Here is just one example of how you could turn this into functions. Also your confirmation number generation is wrong since simply generating a random number does not mean they are unique. You will need to keep track of these numbers and maybe instead use the auto-generated ID? function PrintMessage($firstName, $lastName, $totalDays, $confName, $track, $cfmNbr, $inclMealPlan, $cost) { $text = ' {first_name} {last_name} thank you for your interest. You have registered for {total_days} day(s) at our {conference_name}. You chose {interest_track} as for your track of interest. Please bring cash or your credit card the first day for payment. Your confirmation number is {confirmation_number}. Your total cost {incl_meal_plan} is ${cost}. We look forward to your participation. '; $vars = array( '{first_name}' => $firstName, '{last_name}' => $firstName, '{total_days}' => $totalDays, '{conference_name}' => $confName, '{interest_track}' => $track, '{confirmation_number}' => $cfmNbr, '{incl_meal_plan}' => ($inclMealPlan) ? '(incl. your meal plan)' : '', '{cost}' => $cost, ); $text = str_replace(array_keys($vars), $vars, $text); $text = nl2br($text); return $text; } function GenerateConfirmationNumber() { return mt_rand(1, 1000); } function CalculateCost(Array $dayPrices, Array $mealPrices, $totalDays, $inclMeals = false) { if (!isset($dayPrices[$totalDays])) { return false; } if ($inclMeals && !isset($mealPrices[$totalDays])) { return false; } $basePrice = $dayPrices[$totalDays]; if ($inclMeals) { $basePrice += $mealPrices[$totalDays]; } return $basePrice; }
-
100 form fields? The only one's who are going to fill that in is you (for testing) and web crawling bots (to ruin your life). 100 form fields is no different in OOP then 5 form fields.
-
Propel peer static classes: how to avoid to write duplicated code?
ignace replied to stablum's topic in Frameworks
Also, read this: http://propelorm.org/blog/2010/08/03/refactoring-to-propel-1-5-from-peer-classes-to-query-classes.html- 3 replies
-
- php
- static-methods
-
(and 3 more)
Tagged with:
-
Propel peer static classes: how to avoid to write duplicated code?
ignace replied to stablum's topic in Frameworks
We need to see real code, and what you will duplicate, to tell you how you can keep it DRY.- 3 replies
-
- php
- static-methods
-
(and 3 more)
Tagged with:
-
Because I always eat the money I earn... Coins are the hardest to digest, so always round up to a dollar. But what dale said is true, you should not be doing this for free. It depends on how you want to build an application. If you are going the OO way then starting off with the DB design is a bad idea, instead you should start out with your objects and how they will interact with each other to solve the problem domain. Then when you have something that can work, translate this to whatever DB design fits the bill. If you use Doctrine for this, it won't even be that hard. If performance is an issue, you can always adjust your OO design to accomodate for this. Indeed or whatever UML diagramming tool you favor.
-
Or simply go to http://php.net/?setbeta=1 Its an improvement (in terms of functionality, and usability) over the old website. Visually it looks better, it doesn't need much more IMO.
-
Strider, you should try to avoid setters/getters as much as you can. abstract class AbstractCalculator { protected $left; protected $right; public function __construct($left, $right) { if ($left instanceof AbstractCalculator) { $left = $left->getValue(); } if ($right instanceof AbstractCalculator) { $right = $right->getValue(); } $this->left = $left; $this->right = $right; } public function __toString() { return (string) $this->getValue(); } abstract public function getValue(); } class Sum extends AbstractCalculator { public function getValue() { return $this->left + $this->right; } } class Multiply extends AbstractCalculator { public function getValue() { return $this->left * $this->right; } } class Divide extends AbstractCalculator { public function getValue() { return $this->left / $this->right; } } echo new Divide(new Multiply(new Sum(5, 5), 2), 10);You should not be forced to edit the same class every time you want to add another operation. Your code becomes bloated because the class needs to do too many things.
-
So where exactly are you stuck?
-
Also a client is more likely to hire you if you can show an application where you have built something similar to the project you are discussing. Which is why having demo's of several different kind of applications handy a good sale strategy. Like Kevin already mentioned scout your local businesses, though far from big bucks, they are a good way to fill your portfolio and gain experience. Advertisement is as good a payment as money.
-
Is this normal or should I be concern?
ignace replied to Sam46's topic in PHPFreaks.com Website Feedback
... the one he wrote himself! -
I think you mean <?= which like you say will be always available since 5.4 and are used in Zend views. <? on the other hand won't and may even disappear in the future. http://www.php.net/manual/en/ini.core.php#ini.short-open-tag
-
It should be noted that <? is discouraged.
-
What programming language could Iron Man's Jarvis be programmed in.
ignace replied to bamfon's topic in Application Design
I think it would be written in J(arvis) -
As an example. By default all images will show a spinner and when the document has been loaded it will fire X number of ajax requests to get the twitter images. You can further improve this with a lazy-loading image script (google that) which means that only when the user scrolls down and the image is "viewed" will it fire the AJAX request. <img src="spinner.gif" alt="" class="load-author-image" data-twitter-screen-name=".."> <script> $(function() { $('.load-author-image').each(function(node) { $.ajax('get-author-image.php', { 'twitter-screen-name': $(node).data('twitter-screen-name') }, function(data) { node.src = data; }); }); }); </script>Additionally you could cache images instead of requesting them over and over again from the Twitter API. Or you could simply use a cron to get the images and store the paths in your database.
- 15 replies
-
- php
- twitterapi
-
(and 1 more)
Tagged with:
-
Need help in starting to structure code for a specific problem
ignace replied to walermo's topic in PHP Coding Help
The below code should set you off in the right direction <?php $sql = ' SELECT jobs.id, jobs.trackno, jobs.job_start, jobs.job_end, contractors.rate FROM jobs LEFT JOIN contractors ON contractors.id = jobs.contractor_id ORDER BY jobs.id ASC '; $stmt = $mysqli->query($sql); $prev = null; $diff = 0; $totalWorkedHours = 0; while ($job = $stmt->fetch_object('Job')) { if ($prev !== null && ($diff = $job->getJobStartDate()->diff($prev->getJobEndDate())->format('i')) > 30) { // process! // ... // reset! $totalWorkedHours = 0; } echo /* # */ $job->getId(), /* Job# */ $job->getTrackNo(), /* Start */ $job->getJobStartDate()->format('H:i a'), /* End */ $job->getJobEndDate()->format('H:i a'), /* diff */ $diff, /* thisjobtime */ ($workedHours = $job->getWorkedHours()), /* thisblocktime */ $totalWorkedHours, /* + difference */ ($diff / 60), /* + thisjobtime */ $workedHours, /* = thisblocktime */ $totalWorkedHours + ($diff / 60) + $workedHours; $totalWorkedHours += $workedHours; $prev = $job; } class Job { const DATETIME = 'Y-m-d H:i:s'; private $id; private $trackno; private $job_start; private $job_end; private $rate; public function __set($key, $val) { switch ($key) { case 'id': $this->id = $val; break; case 'trackno': $this->setTrackNo($val); break; case 'job_start': $this->setJobStartDate($val, self::DATETIME); break; case 'job_end': $this->setJobEndDate($val, self::DATETIME); break; case 'rate': $this->setJobRate($val); break; default: throw new DomainException; } } public function getId() { return $this->id; } public function setTrackNo($trackNo) { $this->trackno = $trackno; return $this; } public function getTrackNo() { return $this->trackno; } public function setJobStartDate($date, $format = null) { $this->job_start = ($date instanceof DateTime) ? $date : DateTime::createFromFormat($format, $date); return $this; } public function getJobStartDate() { return $this->job_start; } public function setJobEndDate($date, $format = null) { $this->job_end = ($date instanceof DateTime) ? $date : DateTime::createFromFormat($format, $date); return $this; } public function getJobEndDate() { return $this->job_end; } public function setJobRate($rate) { $this->rate = $rate; return $this; } public function getWorkedHours() { return $this->getJobEndDate()->diff($this->getJobStartDate())->format('s') / 3600; } } -
I knew a guy once who used the JB signature everywhere. Jason Bannerman?
-
Unless it's a porn site. Then it's all about the eye-candy. But I guess the design is then considered a feature.
-
A framework essentially takes out boilerplate code (session, authentication handling, db "abstraction", ..) and allows you to focus on writing the actual application. Some frameworks are really good at this, others just try to get in your way as much as possible just to make sure your really annoyed when working with it. I don't like Laravel, never did, never will. They have a DI container, yet they promote calling all your classes using their static operator, yeah... no! Symfony components are really wasted on them.
-
There is nothing complex about a forum. At the bare basic you have a Topic and a Post. Everything else are just categories, which in turn can have categories. Instead of starting out with a database schema, try it out in code and populate simple array's, something like this: namespace Forum; class Topic implements Countable, IteratorAggregate { private $posts = array(); public function __construct(Post $firstPost) { $this->addPost($firstPost); } public function addPost(Post $post) { $this->posts[] = $post; } public function receivedReplies() { return ($this->count() - 1) > 1; } public function count() { return count($this->posts); } public function getIterator() { return new ArrayIterator($this->posts); } public function lastReceivedReplyAt() { if (!$this->receivedReplies()) { return null; } $date = end($this->posts)->getPostDate(); reset($this->posts); return $date; } } class Post { private /** @var User */ $author; private /** @var string */ $subject; private /** @var string */ $text; private /** @var \DateTime */ $postDate; private /** @var \DateTime */ $modifyDate; public function __construct($author, $subject, $text, $postDate = null) { $this->author = $author; $this->subject = $subject; $this->text = $text; $this->postDate = $postDate ?: new DateTime; } } class User { private $alias; }
-
Not exactly. It's a way to separate concerns, your code can still be shitty.
-
Please consider using spl_autoload_register instead of using __autoload.
-
Indeed output buffering is not a bad coding practice at all. That said certain PHP installations even have automatic output buffering enabled without having to write ob_start() anywhere. http://be2.php.net/manual/en/outcontrol.configuration.php#ini.output-buffering
-
You need composer Then run: $ php composer.phar installIf that doesn't work then find out where in C:\wamp your php.exe resides copy the path and run it like: $ C:\wamp\php\bin\php.exe composer.phar install