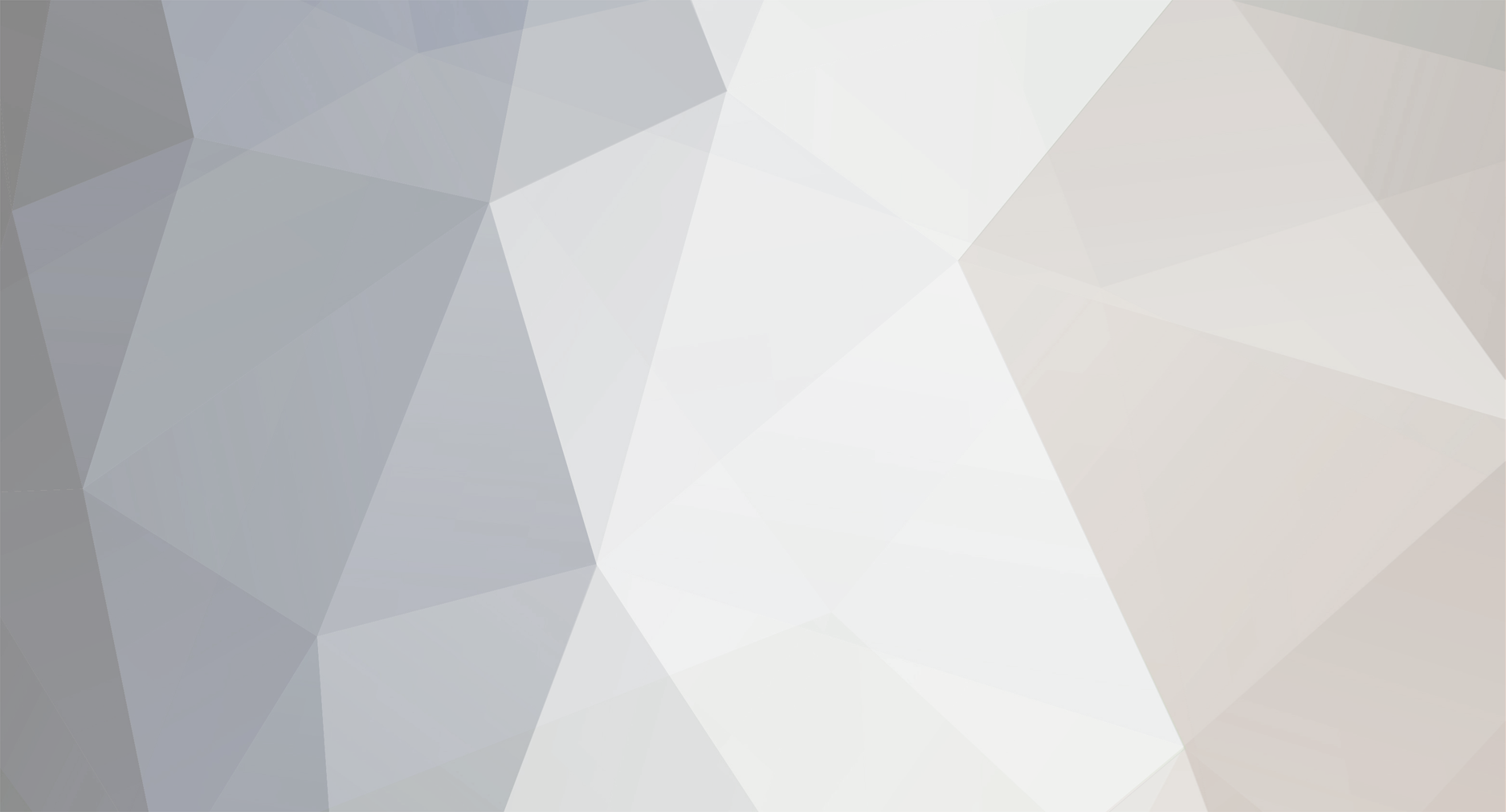
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
You really only need to store a single value in the $_SESSION global, and that is the username after they have logged in. I.E., for guests your $_SESSION will look like this: Array ( ) For logged in users, your $_SESSION will look like: Array ( [Username] => 'TheirUserName' ) From that alone you can look up everything else you need about the user in the DB as they access pages. As for the cookie to provide an auto-login for return visitors, you will need to store at least their username. However it's not enough to store just their username as anyone could duplicate the cookie on their machine with a different user's name and log in as that person. In addition to the username, you should store a unique key in the cookie as well and also store that key for the user's record in the database. When anyone visits the site, check for a username in the cookie. If it exists, check the unique key in the cookie to see if it matches what's in the database for that user. If it all checks out, auto-log them in; otherwise they're an imposter.
-
[code]<?php $arr = array( 1, 2, 3); test($arr); function test($an_array){ print_r($an_array); } ?>[/code]
-
How to use folder vars like "/500" instead of get vars like "?id=500"?
roopurt18 replied to ultrus's topic in PHP Coding Help
mod_rewrite http://www.phpfreaks.com/forums/index.php/board,50.0.html -
Only checked boxes will appear in $_POST, unchecked boxes will not. If you want to determine if the box was checked: if(isset($_POST['check_name'])){ // checked }else{ // unchecked }
-
You're not going to have two users logging in to your site through the same browser on the same PC. If they do, they're just screwing around and it's no big deal. #1 logs in and views welcome page #2 logs in and views welcome page #1 refreshes welcome page and now sees #2's welcome page This is in no way a threat or security violation to your site since the two users are sitting right next to each other. Get over it.
-
You're sure your webserver is set up and running correctly and that it is processing php pages? If you're not, create the following file: [b]phpinfo.php[/b] [code]<?php phpinfo(); ?>[/code] and point to that in your browser.
-
AFAIK, you are correct. You use sessions to transmit data from page to page [b]while[/b] the user is browsing your site. You use cookies for any information you want to persist between browser instances. Also, keep in mind it is not enough to store only the user's name in the cookie to grant them automatic login on their next visit to the site; I've seen that method before and it is [b]highly insecure[/b].
-
If that's all in the same file you are creating an html page that looks like: [code] <html> <head></head> <body></body> </html> <html> <head></head> <body></body> </html> [/code] which is not valid HTML.
-
How to develope my communication skill ???
roopurt18 replied to sivanath.nagendran's topic in Miscellaneous
Get a job as a waiter then learn how to sell, sell, sell. -
You probably also want to surround [i]details[/i] with single quotes: $adedt['details']
-
Are you calling htmlentities on the form field before inserting it into the DB?
-
Nevermind, I got it. On the source machine, I was using a command like so: tar -cf ~/host_move/the_dir.tar ~/domain/data/the_dir Changing the command to: tar --directory=/dir1/dir2/dir3/dir4/user/domain/data/ -cf ~/host_move/the_dir.tar the_dir solved the problem. I post this for anyone that runs into a similar situation.
-
Weird variable declaration. will this work?
roopurt18 replied to pakenney38's topic in PHP Coding Help
You have to use $date[[b]$[/b]x] = ... Notice the $ sign you had left out. Here is why the arrays are easier, you could output all of your inputs like so: [code]<?php if(count($date)){ // $date array has members in it foreach($date as $key => $val){ echo '<input name="date' . $key . '" type="text" id="date' . $key . '" value="' . $val . '" />'; } } ?>[/code][size=10pt][/size] -
I'm trying to tar a directory on one machine to move it to another. On the starting machine the directory is something like: /dir1/dir2/dir3/dir4/user/domain/data/[i]the_dir[/i] The target destination on the new machine is /home/user/public_html/data/[i]the_dir[/i] Try as I might, I keep getting a result like this on the target machine: /home/user/public_html/data/dir1/dir2/dir3/dir4/user/domain/data/[i]the_dir[/i] I'll keep reading documentation until I get it, but if anyone wants to help me along with this I'd greatly appreciate it!
-
Weird variable declaration. will this work?
roopurt18 replied to pakenney38's topic in PHP Coding Help
You can do what you want with: [code]<?php for($i = 1; $i <= $rowcount; $i++){ ${'date' . $i} = $_POST['date' . $i]; } ?>[/code] However, I [b]highly[/b] recommend not doing it this way and using an array as Orio suggested. -
I guess I should have been more specific. I may be mistaken, but I believe all operators of the form: $var1 [i]op[/i]= $var2; are equivalent to: $var1 = $var1 [i]op[/i] $var2; I.E: $var1 = $var1 + $var2 is equivalent to $var1 += $var2 $var1 = $var1 . $var2 is equivalent to $var1 .= $var2
-
It's string concatenation. $var = 'Hello'; $var .= ', World!'; echo $var; Outputs: Hello, World!
-
I thought there was a better way to do it than with DATE_FORMAT but for some reason I missed both DAY and DAYOFMONTH when glancing at the MySQL documentation. I'd use one of those!
-
Problem reading array's, validation's not working.
roopurt18 replied to Ninjakreborn's topic in PHP Coding Help
Place this code somewhere in the area where the form is being processed: [code]<?php echo '<pre style="text-align: left;">' . print_r($_POST, true) . '</pre>'; ?>[/code] Then submit the form without checking any of the boxes and paste the contents of the $_POST array here. -
I prefer to let the database handle things such as this. SELECT DATE_FORMAT([i]timestamp[/i], '%e') AS DayFromTS FROM [i]table[/i] WHERE 1 [i]timestamp[/i] is the name of the column containing the timestamp value, '%e' is the format to return (Day of the month, numeric (0..31)), DayFromTS is the name that it will return it as. MySQL is a very, very powerful langugae. Before you go off writing bits of PHP code to accomplish some tasks, I would look around in the MySQL manual at the [b]Functions and Operators[/b] found here: http://dev.mysql.com/doc/refman/4.1/en/functions.html
-
I think you should pick up a PHP programming book and start there.
-
Problem reading array's, validation's not working.
roopurt18 replied to Ninjakreborn's topic in PHP Coding Help
[code]<?php // test.php // Display a form that uses checkboxes as an array // Validate that at least one selection was made if(count($_POST)){ echo "<pre>" . print_r($_POST, true) . "</pre>"; // Form was submitted if(is_array($_POST['checks']) && count($_POST['checks'])){ foreach($_POST['checks'] as $check){ echo "You selected: {$check}<br />"; } }else{ echo "You did not make any selections!<br />"; } }else{ // Displaying form $form = <<<THEFORM <form name="theform" method="post" action=""> <input type="checkbox" name="checks[]" value="opt1" />Option 1<br/> <input type="checkbox" name="checks[]" value="opt2" />Option 2<br/> <input type="checkbox" name="checks[]" value="opt3" />Option 2<br/> <input type="checkbox" name="checks[]" value="opt4" />Option 2<br/> <input type="submit" name="submit" value="Submit" /> </form> THEFORM; echo $form; } ?>[/code] count() doesn't default to anything, it counts the number of elements in an array and that's it. Picking an arbitrary number, such as 5, and calling that ground zero is not necessarily reliable. -
Problem reading array's, validation's not working.
roopurt18 replied to Ninjakreborn's topic in PHP Coding Help
If it's an array, use count(); -
number_format(3.947368, 2);
-
I tend to limit my use of OOP in PHP to general mechanisms within my site. I.E. typical objects within my site tend to be CPage, CForm, CAccount, CUser, CComponent, etc. I put as much base functionality into each of these objects as necessary and use them as a base class when I find a situation in which a object needs a more specific functionality. As an example, my last CForm class allowed me to set the fields of the form, which routines would validate each field, the creation of all form related HTML, etc. Then when I needed to create a new form in my site, I'd derive a new class from CForm, specify in the constructor which fields the new form contained and which routines to use to validate it, and just like that I have a new form on my site. It automatically knows how to display itself, how to display default values when re-displaying itself because of a form error, etc. I tend to not use objects when pulling data from a database. 9 times out of 10 when I pull data from a database it's merely for displaying and I find the arrays returned by the MySQL functions sufficient for this task.