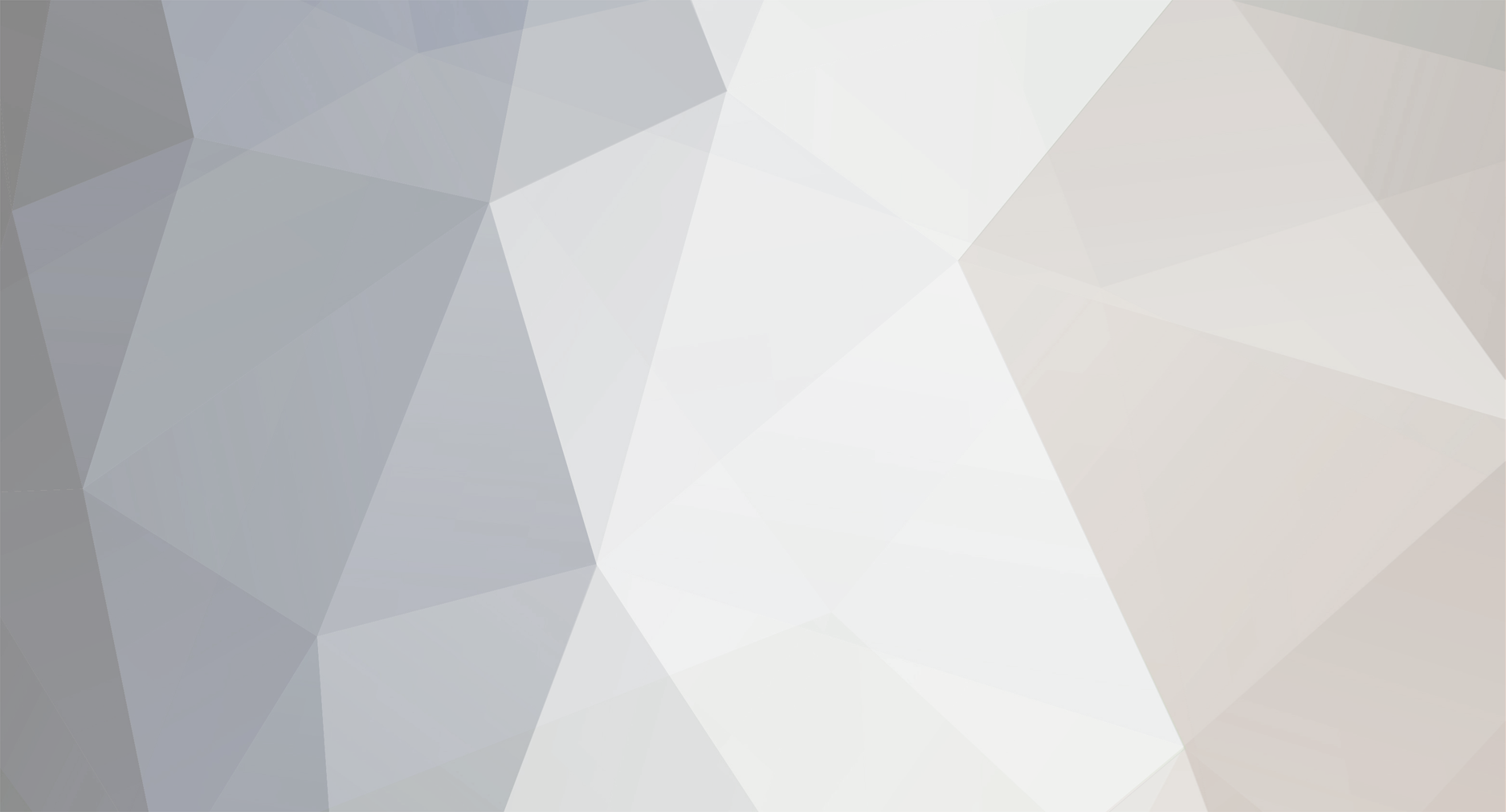
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
I still don't see how it's pass by reference. By the time you make the assignment you are working on what's already a local value to the function. Perhaps I'm missing something here.
-
Make sure you initialize $sum before you start the loop to zero!
-
[code]<?php if ($log->IsLoggedIn() == true) { } else { ?> <div align="left"> <p align="center"><span class="style45"><br> <span class="style46">Username:<br> </span></span> <input name="txt_Username" type="text" class="style15" id="txt_Username"> <br> <span class="style47">Password:</span><br> <input name="txt_Password" type="password" class="style15" id="txt_Password"> <br> <br> <input name="frmLogin_Submit" type="submit" class="style11" id="frmLogin_Submit" value="Login"> <br> <span class="style47"><a href="forgot_password.php" style="color:#ffffff">Forgot your password? </a></span></p> </div> <? } ?>[/code] You can jump in and out of PHP mode. For the record, I don't particularly like this approach, but you can do it!
-
[code]<?php function foo($args) { if (array_key_exists('dbh', $args)) $dbh = $args['dbh']; if (array_key_exists('result', $args)) $result = &$result; # pass by reference } ?>[/code] Perhaps it's because I'm just tired, but how is that pass by reference?
-
Chances are you're off by 1.
-
I like to set my default parameters to NULL instead of '' or 0. Not sure if it makes any big difference though.
-
line 151 broke.....please help spot bad code
roopurt18 replied to boostboards's topic in PHP Coding Help
$mov is used in line 151 as if it is an object. Your code is breaking because what you're passing to the function for $mov is not an object. Try posting the line that calls the function as well as the previous 10 lines or so. And did you read anything previously posted over at http://www.codeguru.com/forum/showthread.php?t=319571 ? -
User management accounts - private page per user....?
roopurt18 replied to Skeleten Neteleks's topic in PHP Coding Help
Try a table set up like the following: [size=10pt][pre]Users +-----------+--------------------+ | id | int | | username | varchar(30) | | password | int | +-----------+--------------------+ Products +-------------+-------------------+ | id | int | | name | varchar(50) | | description | tinytext | +-------------+-------------------+ UserProductLinks +--------------+---------------+ | user_id | int | | product_id | int | +--------------+---------------+ [/pre][/size] Some queries... [b]Get all users from the system:[/b] [code]SELECT * FROM Users WHERE 1[/code] [b]Get all products from the system:[/b] [code]SELECT * FROM Products WHERE 1[/code] [b]Get all products who have user links (Here we join the three tables):[/b] [code]SELECT * FROM Users u, Products p, UserProductLinks l WHERE l.user_id = u.id AND l.product_id=p.id[/code] This will limit the number of columns you have to put into your Users table. You don't really want to put your product information into the User table. The only stuff that should go into the User table is User information. The only thing that should go into the Product table is product information. The third table is so you can link the two tables together. Note that this also prevents you from having the same product entered all over in your database. With your current database design, imagine if you have 100 users with the product "Peanut Butter" but now you have to change it to "Peanut Butter Nutty." Would you rather change it one time in one table or change it all over the place? As for your specific problem, you need to loop the statement: $row = mysql_fetch_array( $result ); Try this: [code]<?php // store the record of the "example" table into $row while($row = mysql_fetch_array( $result )){ // Print out the contents of the entry echo "Company: ".$row['CompanyID']; echo " Username: ".$row['Username']; echo " Product 1: ".$row['Product1']; echo " Description: ".$row['Description1']; echo " Password: ".$row['Password']; } ?>[/code] Also, while it doesn't matter [i]that[/i] much, I recommend making only a single echo statement for all of your pages. So instead of [code]<?php echo $string1; echo $string2; echo $string3; ?>[/code] Do this: [code]<?php $out = ''; // some code $out .= $string1 . $string2; // more code $out .= $string3; $out .= SomeFunctionToCreateHTML(); // Now we output echo $out; ?>[/code] -
Use method="post" and the $_POST array instead of $_GET, although you might get the same thing. For my own pages, I use method="post" for all form submissions and reserve using $_GET only for when I want to create dynamic links that pass very little data, maybe only a product or user ID but nothing more.
-
Determine which .php files are in use by a site?
roopurt18 replied to roopurt18's topic in PHP Coding Help
Thorpe, as bqallover mentioned most webserver logs don't include included files; so unless you are speaking of something else I'm not sure that'd help. Also, I'm not sure how much outside my own personal directory on the server I can browse and look at as I've never really tried. -
Enclose your last value for the sql statement in single quotes. Don't forget to escape the string.
-
Look up isempty or is_empty in the PHP manual. I forget the exact name.
-
how to check a particular field from a query
roopurt18 replied to pouncer's topic in PHP Coding Help
Then it's obviously not activated. [code]<?php $sql = "SELECT * FROM users WHERE username='$Username' AND password='$Password'" $q = mysql_query($sql); if($q){ while($row = mysql_fetch_assoc($q)){ if($row['Activated'] == 1){ // It is activated } break; } }else{ // No record found echo 'You need to register!'; } ?>[/code] -
My current project is something I've inherited from other developers. One of my ongoing goals is to clean up a lot of the old code and I've made pretty good progress on that front. However, there are a large number of .php files just floating around that I'm not even sure are being used and others that I know are being used but would like to phase out. To that end I'm looking for a solution to determine which files are still in use by the site. I've thought about adding a small routine that I can call at the top of each file which would insert a record into a DB table with path information about the file, the name of the file, and the date it was accessed on. If the record already exists for that file I'd just update the timestamp for the record; in other words, just one record per file. I figure after a couple months of recorded data here I can go back and look at which files are good candidates to remove from the webhost. Would this be the best way to go about doing this? Or is there an easier method or reporting tool that I can use? I have shell access to the web server, which is running FreeBSD 4.11. Thanks in advance!
-
how to check a particular field from a query
roopurt18 replied to pouncer's topic in PHP Coding Help
[code]<?php $sql = "SELECT * FROM users WHERE username='$Username' AND password='$Password'" $q = mysql_query($sql); if($q){ while($row = mysql_fetch_assoc($q)){ if($row['Activated'] == 1){ // It is activated } break; } } ?>[/code] -
Applying my approach to your direct problem: [code] <?php /*-------------------------------------------------*/ /*--------------START DISPLAY RESULTS--------------*/ $group = isset($_GET['group']) ? "'" . addslashes($_GET['group']) . "'" : NULL; // Clean your data before you // give it to a database -- ALWAYS!!! $priority = isset($_GET['priority']) ? "'" . addslashes($_GET['priority']) . "'" : NULL; $type = isset($_GET['type']) ? "'" . addslashes($_GET['type']) . "'" : NULL; // Now each of $group, $priority, and $type are an escaped string surrounded // with single quotes or the value NULL // We can now build our dynamic WHERE clause $add_wheres = Array(); if($group){ $add_wheres[] = "`group`={$group}"; } if($priority){ $add_wheres[] = "`priority`={$priority}"; } if($type){ $add_wheres[] = "`type`={$type}"; } $rs = @mysql_select_db("project") or die(mysql_error()); $sql = "SELECT * FROM `project_general`"; if(count($add_wheres)){ $sql .= " WHERE " . implode(" OR ", $add_wheres); } $sql .= " ORDER BY `rec_num` ASC"; /* Previously you had the following lines, but it would seem $_GET['hidden'] is no longer needed with my method if(isset($_GET['hidden'])) { $sql = "SELECT * FROM `project_general` WHERE `group`=\"$group\" OR `priority`=\"$priority\" OR `type` =\"$type\" ORDER BY `rec_num` ASC"; } else { $sql = "SELECT * FROM `project_general` ORDER BY `rec_num` ASC"; } */ ?> [/code] (EDIT) Note that I've cleaned the data you're giving to the database; I've assumed it's text data, you would use a slightly different process for numeric data. In addition, as recommended by btherl, you should break that page down into smaller functions, it makes it easier to really follow what's going on.
-
No. Every time he appends a "WHERE" to the query he sets a variable to true. Next time he's about to add another "WHERE" he checks if it's already been added and if it has, uses "OR" instead. The method I typically use for dynamic WHEREs is: [code]<?php $add_wheres = Array(); // Set to empty if($condition1){ $add_wheres[] = "table1.fld1=table2.fld2"; } if($condition2){ $add_wheres[] = "$table2.fld3=table3.fld4"; } $sql = "SELECT ..."; // There is no WHERE in here yet if(count($add_wheres)){ // We have a dynamic WHERE $sql .= " WHERE " . implode(" AND ", $add_wheres ); }else{ // No dynamic WHERE, possibly set a default WHERE or do nothing $sql .= " WHERE something=something"; // Used a default } ?>[/code]
-
User management accounts - private page per user....?
roopurt18 replied to Skeleten Neteleks's topic in PHP Coding Help
Are you running on some sort of a time line or is this a casual project you're working on? If it's more casual I would instead start asking for advice on how to set up a MySQL database and the basics of table design. It will make your life much, much easier in the long run. -
If the fields that contain the newlines can also contain a semi-colon then you're really in trouble. I recently had to battle importing dbase memo fields from Visual FoxPro into MySQL text fields. The solution there was to use more complicated delimiters. I separated fields with [<>] and records with <[]>. So: fld1[<>]fld2[<>]fld3[<>]memo field that has hard returns in it<[]> fld1[<>]fld2[<>]fld3[<>]another memo field with hard returns <[]> For my import code, I did something like: [code]<?php $file = file_get_contents('the/file/path.txt'); if(strlen($file)){ $Records = explode( '<[]>', $file ); if(is_array($Records) && count($Records)){ foreach($Records as $Key => $Record){ $Fields = explode( '[<>]', $Record ); if(is_array($Fields) && count($Fields)){ foreach($Fields as $FieldKey => $FieldVal){ $Fields[$FieldKey] = trim($FieldVal); } $Records[$Key] = $Fields; }else{ unset($Records[$Key]); } } } } ?>[/code] The fun part about that was our FoxPro programmer, who has at least 5 years experience, couldn't figure out how to export the memo fields intact. I sat down and figured it out in 3 hours on a language I'd never even heard of until I started working here. Go figure! Hope that helps you out.
-
Then leave off the else part as it's optional. I would suggest going to google and searching for: php if and following the first link that points to the PHP manual.
-
User management accounts - private page per user....?
roopurt18 replied to Skeleten Neteleks's topic in PHP Coding Help
You'll want to look up any and all functions starting with mysql_ in the php manual then. -
User management accounts - private page per user....?
roopurt18 replied to Skeleten Neteleks's topic in PHP Coding Help
You typically don't want to write a unique page per user when creating a site, especially if you are expecting a large amount of users. It is better to filter everyone through a single entry point and have them land in one, or at least one of very few pages. It is the page that they land on that determines what user type they are (admin, moderator, regular, etc.) and displays the appropriate information. Could you possibly give an example of the type of output you're wanting to display on these unique user pages? -
Would it be extremely hard if I wanted to do this....
roopurt18 replied to drcdeath's topic in PHP Coding Help
I would think most users after logging in would need the same sort of functionality. If you're expecting to have more than say, 10 users for this site, you might want to consider a different approach. Otherwise you're going to be creating dozens or hundreds of .php files that are most likely all the same or very similar. -
Usernames and passwords should be stored inside a database. You should be using the username and password from the submitted form to look up the corresponding row in the database table. If found, the user exists and if not found, the user does not exist.
-
Got it. Since I'm a noob, what's so bad about the 3 ports? Just curious...