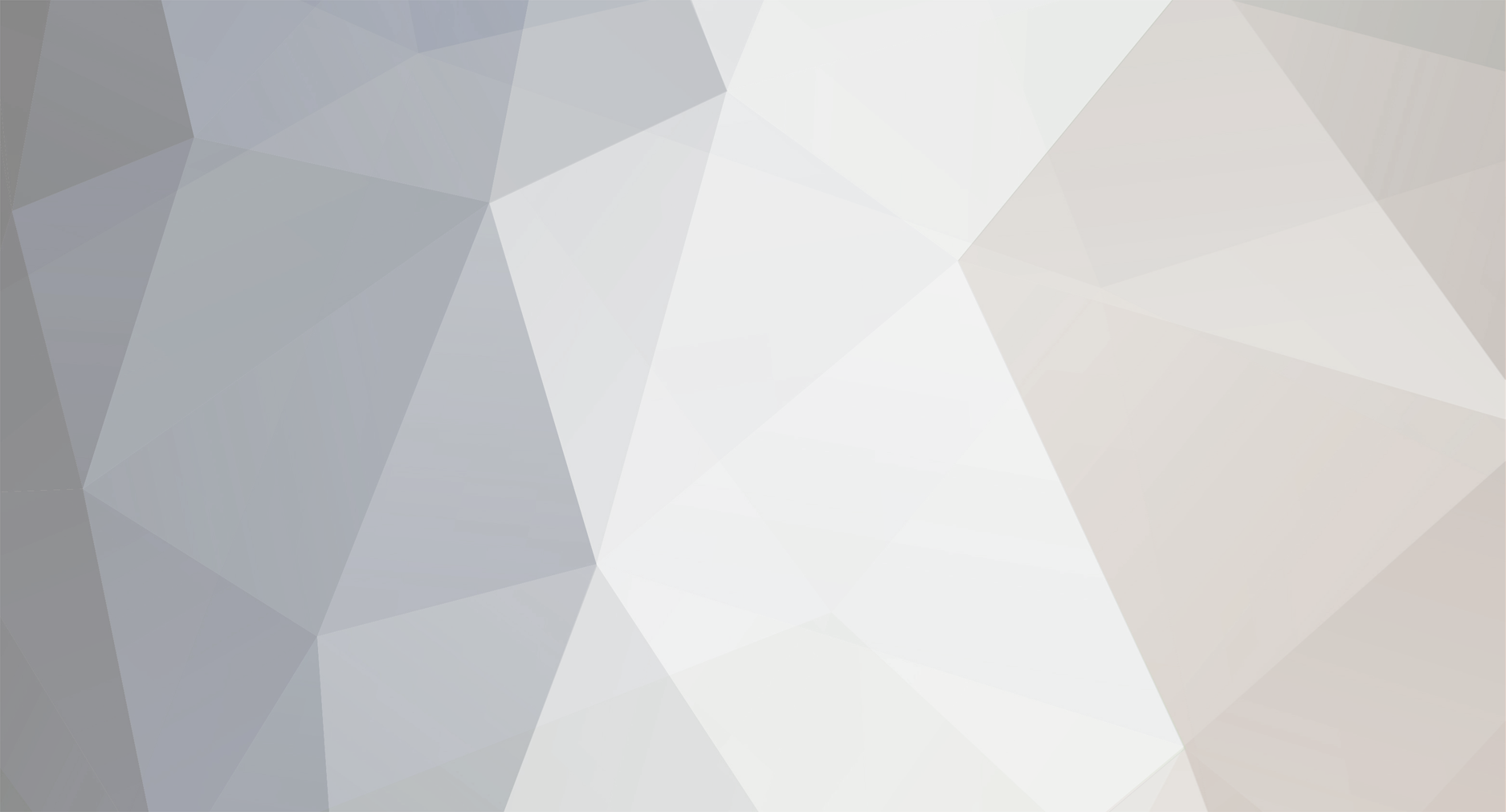
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
User management accounts - private page per user....?
roopurt18 replied to Skeleten Neteleks's topic in PHP Coding Help
Always a pleasure to help someone eager to learn. This is the foundation required to program generic pages that appear tailored to specific users. Keep in mind that as you program, many pieces of your code will require repeated functionality. When and if you find yourself retyping the same code as you did somewhere else, find a way to make it available from a central location. This type of programming is less typing for you, easier to maintain and debug, and in general more pleasant to look at 6 months later. In my opinion, the best programmers are a cross-breed between lazy and hard-working. You should always spend a sufficient amount of time designing your software and thinking about the many ways in which it will be used; this is the hard-working part of our job. The design that you come up with should enable you to implement the most common features of your project in the shortest amount of time when you need them; this is the part where we get to be lazy! -
User management accounts - private page per user....?
roopurt18 replied to Skeleten Neteleks's topic in PHP Coding Help
The array that is printing is what's currently stored in the $_SESSION auto-global. I added that to illustrate that information from one page can transparently (as far as the user is concerned) carry over to another page. Notice that each index of that array is a value that was stored in index.php. There are many cases where you need to pass information from one script to another and there are several ways you can do so: 1) Using a form with method="post" or method="get" 2) Creating a link that sets URL parameters: home.php?user=john123&logged_in=true 3) With cookies 4) With sessions I use a combination of forms, urls with parameters, and sessions myself. In my sessions I store the least amount of information necessary to identify the current user. Typically this means just a username. On every page visited in my site, I use the information stored in $_SESSION to look up everything else I need from the database for that specific page. I use forms for more complicated requests such as site searches, file uploads, message board posts, etc. I use URL parameters for more simple requests such as sorting a table, pagination, and some types of filtering. There is no right or wrong way to go about any of this, it's all a matter of preference. Let's say your users have a lot of information in the DB such as their phone number, address, etc. You could load all of that information once when they log in, store it all in the $_SESSION array, and never have to access the database again. As I said, I myself prefer to store the least amount of information necessary because based on just the username I can look that information up again when necessary. My method adds a little more overhead to the DB but I feel it is more secure as there is less data floating around. Whatever you choose is a matter of preference and convenience and over time you will develop a way of doing things that you are comfortable with. -
require_once()
-
User management accounts - private page per user....?
roopurt18 replied to Skeleten Neteleks's topic in PHP Coding Help
If you are writing all of your queries like this: [code]<?php $sql = "QUERY IS IN HERE"; $var = mysql_query($sql); ?>[/code] Insert this line between the assignment to $sql and the function call: [code]<?php echo '<pre style="text-align: left;">' . wordwrap(print_r($sql, true), 80) . '</pre>'; ?>[/code] Looks like one of your sql calls is a bad query. Copy and paste the output here after having done that. -
User management accounts - private page per user....?
roopurt18 replied to Skeleten Neteleks's topic in PHP Coding Help
What is the exact error you're getting? Is it from PHP or from MySQL? -
User management accounts - private page per user....?
roopurt18 replied to Skeleten Neteleks's topic in PHP Coding Help
[code]<?php // THIS IS WHERE YOU'D PULL MORE INFORMATION FROM THE DATABASE DEPENDING // ON WHICH USER HAS LOGGED IN AND DISPLAY IT TO THEM! $sql = "SELECT * FROM Users u, Products p, UserProductLinks l WHERE " . "l.user_id = u.id AND " . "l.product_id=p.id AND " . "u.User={$_SESSION['UserClean']}"; // Notice the added condition here! ?>[/code] You changed [b]$_SESSION['UserClean'][/b] to [b]$_SESSION['UsernameClean'][/b] in index.php. You have to change it in home.php as well. -
User management accounts - private page per user....?
roopurt18 replied to Skeleten Neteleks's topic in PHP Coding Help
Copy paste your current versions of index.php and home.php. Make sure you enter them between [b]code[/b] tags and [b]make sure you remove your specific database information from the top.[/b] -
Does PHP have a preprocessor like C / C++?
roopurt18 replied to roopurt18's topic in PHP Coding Help
I need the function to use the values at the point of the function call, not within the function itself. -
I have a function that should receive as it's arguments some of the magic constants (__FILE__, __LINE__, etc.). I'd like to be lazy and not have to remember the order of the arguments. Is there any way I can set up the function call like a #define in C / C++ so that it is replaced in the PHP file but not actually called in the define()? Thanks!
-
User management accounts - private page per user....?
roopurt18 replied to Skeleten Neteleks's topic in PHP Coding Help
[code]<?php $Out .= "<p>Product Name: " . $row['Name']; . "<p>Description: " . $row['Description']; ?>[/code] Anytime you receive a programming error on line [i]x[/i], there is a very good chance it's not actually on line [i]x[/i] but some where near it. If you look at line 30 you will notice it ends in a semi-colon, which terminates a statement. This causes the beginning [b]dot[/b] on line 31 to cause an error. -
Functions are the mechanism by which you combine programming statements into conceptual wholes. Let's say that every page on your website has to start with the same 10 lines of code. Do you really want to retype that same cover over and over on every page? If your site is only 3 pages, it's not a huge deal. But if your site is 10 pages, thats 100 lines of code. Now what happens if you find an error in it? You have to change it on every single page. Sucks for you! Here comes functions to the rescue! You write that code [b]once[/b] as a function in a single file. Then you require_once that file in every page and at the very top of every page, call the function you wrote. Think of a function as a call to directory service. You dial the phone, give the operator your zip code or a company name, and they give you a phone number. You don't care [b]how[/b] they found that information, all you care about is that they gave it back to you. That's what a function is. Every page doesn't care [b]how[/b] the header is created or necessarily what's in it. All they care about is if they can call the function that creates it and all [b]you[/b] as the programmer care about is that you don't have to retype it a dozen or more times and that when you do find an error it's localized to one piece of your code.
-
User management accounts - private page per user....?
roopurt18 replied to Skeleten Neteleks's topic in PHP Coding Help
[code]<?php // First we check if the username is valid, the condition to do so varies // based on your application. A valid username might be alphanumeric only, // or alpha only, and usually they have a length restriction. It's a good // idea to test for that here // The value $user_name_is_invalid is a dummy to represent whatever check // you might actually make if(!$user_name_is_invalid){ $HadErrors = true; // Not valid, so mark that we had errors $Errors[] = "Login name appears to be invalid."; } ?>[/code] If you left the above code intact in the [b]ValidateForm[/b] function I provided, then it will always give you that error. The reason is the line: [code]if(!$user_name_is_invalid){[/code] If you read the comment above that line, you will see I'm advocating checking the format of the username provide by the user in the login form. For instance, if we know that all usernames are alphanumeric and between 3 and 10 characters in length, then we automatically know that [b]reallylongusername[/b] and [b]crap!@#[/b] are [b]invalid[/b]. The variable I used to represent that test is called $user_name_is_invalid. I included it as a reminder to you that you should check the format of the data being entered on your forms and not to take it for granted that it is correct. You can change that variable to an appropriate test (using something like preg_match) or comment out that block entirely, depending on how thorough you want to be. The exact reason your form is always giving you an error is that $user_name_is_invalid is unset, so the expression !$user_name_is_invalid evaluates as true, so the form always thinks there is an error. The morale of the story here is you can't ever just copy and paste code that is given to you or that you find on the internet and expect it to work. Look at it in detail, plug some echo "im at this point in the code" statements in various places, and play with it. That is the only way you can truly learn and understand this stuff. -
I need to enable dbase support on our new host before I can begin migrating my site from my old host. The host can / will do this for me but I'd like to tackle the problem on my own to expand my own personal knowledge. Apparently I can go about this a couple ways. I can recompile apache with a --enable dbase directive, which I'd like to avoid because I don't want to risk breaking the current apache installation. I would think I can also enable it dynamically through an .so. To that end I tried downloading a php-dbase*.rpm and installing it, but I'm getting a failed dependency stating php needs to be greater than 4.3.11. However, the new host is running php 4.4.4 so I'm confused as to why this would be the case. If anyone can provide some advice I'd be extremely grateful. The server is running some version of linux, although I'm not entirely sure which. When it comes to this environment I know enough to be dangerous, which is why I'm now turning here. Thanks!
-
You need stop relying on free tutorials and go pick up a basic PHP programming book. If you don't understand why [code] $user = 'user'; $user = 'user1'; [/code] only recognizes 'user1', then I'm sorry but you may have read the basics, but you didn't understand them.
-
After looking at your code some, you're going to take a performance hit here: [code]<?php $count = mysql_query("SELECT * FROM shows,venues,zipcodes " . "WHERE shows.show_venue LIKE venues.venue_id " . "AND venues.venue_zip LIKE zipcodes.zipcode " . "ORDER BY shows.show_start" , $db); $total_rows = mysql_num_rows($count); $page_rows = 25; if (isset($_GET['page'])) { $page = $_GET['page']; }else{ $page = 1; } $total_pages = ceil($total_rows / $page_rows); $offset = ($page - 1) * $page_rows; $result = mysql_query("SELECT * FROM shows,venues,zipcodes " . "WHERE shows.show_venue LIKE venues.venue_id " . "AND venues.venue_zip LIKE zipcodes.zipcode " . "ORDER BY shows.show_start " . "LIMIT $offset, $page_rows", $db); ?>[/code] Pagination serves two purposes. It limits the load on the MySQL server and it makes your pages more user friendly. You're performing one query to get the total number of records and another to get only those records you want. However, that first query is potentially returning [b]a lot[/b] of records. I've taken the liberty of rewriting this portion of your code: [code] <?php // Get the total count $sql = "SELECT COUNT(*) AS Num FROM shows s, venues v, zipcodes z WHERE " . "s.show_venue LIKE v.venue_id AND " . "v.venue_zip LIKE z.zipcode"; $q = mysql_query($sql); // Set $count to the number of rows if successful, or zero on failure $count = $q && is_array($q) && count($q) ? $q[0]['Num'] : 0; $page_rows = 25; $page = isset($_GET['page']) && is_int($_GET['page']) ? $_GET['page'] : 1; $total_pages = (int)($count / $page_rows + 1) $offset = ($page - 1) * $page_rows; $sql = "SELECT * FROM shows s, venues v, zipcodes z WHERE " . "s.show_venue LIKE v.venue_id AND " . "v.venue_zip LIKE z.zipcode " . "ORDER BY s.show_start " . "LIMIT {$offset},{$page_rows}"; $result = mysql_query($sql); ?> [/code]
-
I didn't bother to look at your source since it's not in code tags in addition to the fact that it looks like more than I want to go about reading. But, you should be storing any dates in one of the date fields supported by MySQL: TIMESTAMP, DATETIME, or DATE. If you're interested in the day and time you'll probably want to be using DATETIME. Let's say you have the following table: [b]events[/b] [code] CREATE TABLE events ( name VARCHAR(25) NOT NULL, description TINYTEXT, scheduled DATETIME UNIQUE( name, scheduled ) ); [/code] Here is some relevant MySQL... Select all expired events: [code]SELECT * FROM events WHERE scheduled < NOW();[/code] Select all non-expired events: [code]SELECT * FROM events WHERE scheduled >= NOW();[/code] Select all non-expired events between now and three weeks from now, order them descending by date [code]SELECT * FROM events WHERE scheduled >= NOW() AND scheduled <= (NOW() + INTERVAL 3 WEEKS) ORDER BY scheduled DESC;[/code] Select page 5 of 200, 50 records per page, of non-expired events [code]SELECT * FROM events WHERE scheduled >= NOW() ORDER BY scheduled ASC LIMIT 250,50[/code] Hope that helps you out some.
-
[quote author=wiccan8888 link=topic=114364.msg465847#msg465847 date=1163137539] men, you're both genius...how did you go that far in PHP, any tips please... [/quote] Buy a PHP programming book; read it, practice it, learn it. Buy a MySQL programming book; read it, practice it, learn it. Buy a system administration book; read it, practice it, learn it. Google for every thing else after that.
-
Using var with PHP5 will cause a warning if you have your error reporting set correctly.
-
How do you check for NULL fields in registration pages?
roopurt18 replied to diode's topic in PHP Coding Help
You should never, ever, not in a million years, rely on javascript as your [i]only[/i] form validation enforcement. Why? Because it can be turned off by the user. If you only use javascript to validate your forms, any malicious user can just turn off javascript and submit any old data they want to. You must always, forever, and every single time, validate form data on the server. It is much more difficult for a malicious user to bypass this sort of form validation than just changing a browser setting. You can use javascript validation as a convenience feature to prevent your user from having to submit the form and wait for the server to validate it to fix a field, however. As for your specific problem, use the [b]method="post"[/b] when creating your HTML form and in the validation routine check the values in $_POST. If you have a form text field named "username", it will show up in $_POST['username']. You can do something like this: [code] if(isset($_POST['username']) && strlen(trim($_POST['username']))){ // A user name was entered! }else{ // You must enter a user name! } [/code] -
Look up XAMPP through google. Download and install the windows version.
-
If you are working with a date field within MySQL, you can use the +/- INTERVAL function of MySQL. SELECT NOW() + INTERVAL 30 DAYS AS ThirtyDaysFromNow FROM tbl WHERE 1 LIMIT 1
-
User management accounts - private page per user....?
roopurt18 replied to Skeleten Neteleks's topic in PHP Coding Help
Remove the extra <?php from whatever I gave you, that was an error. That SQL query that links users to products is pulling [b]all[/b] of the possible links. In the sample home.php I gave you, the line: $Out .= $more_info_from_database; Should be replaced with something like: [code] $sql = "SELECT * FROM Users u, Products p, UserProductLinks l WHERE " . "l.user_id = u.id AND " . "l.product_id=p.id AND " . "u.User={$_SESSION['UserClean']}"; // Notice the added condition here! $result = mysql_query($sql) or die(mysql_error()); // YOU STILL NEED TO LOOP OVER THE $result // store the record of the "example" table into $row while($row = mysql_fetch_array( $result )){ // <- there was a semicolon there, // which can be removed. I guess // I fat-fingered something :D $Out .= "<p>Product Name: " . $row['Name']; . "<p>Description: " . $row['Description']; } [/code] -
User management accounts - private page per user....?
roopurt18 replied to Skeleten Neteleks's topic in PHP Coding Help
I would recomment using sessions for your login script unless your server, for whatever reason, doesn't support them. Here's a few tips for using sessions. The PHP manual: http://www.php.net/session You [b]must[/b] call [i]session_start()[/i] at the top of every single page [b]before[/b] you send any output (i.e. calling echo, print, etc.). You store values in the autoglobal $_SESSION array. Following is a sample index.php that has the outline for a login script which, on successful login, redirects to home.php [b]index.php[/b] [code]<?php // index.php // A sample PHP script to display a login form and validate user identity // Upon successful login, we redirect to our universal home.php // I will wrap up functionalities specific to your application in functions // which you can fill out for yourself session_start(); // The first think we must do is start our session $Out = ''; // This variable is going to hold our final output for the page $Errors = Array(); // This is our errors array for the form // Now we check in $_SESSION if the user is already logged in if(isset($_SESSION['LoggedIn']) && $_SESSION['LoggedIn'] === true){ // This user is already logged in, we should just redirect to home.php header("Location: home.php"); exit(); } // We're going to count how many entries are in $_POST, if there are _any_ // entries in $_POST, then our login form must have been submitted and we // need to validate the user if(count($_POST)){ // Form has been submitted, we need to validate our user if(ValidateForm()){ // Our user is a valid user, so let's log them in and redirect $_SESSION['LoggedIn'] = true; $_SESSION['UserClean'] = CleanFormField($_POST['User']); $_SESSION['User'] = $_POST['User']; // We have set our $_SESSION parameters, so now we can redirect header("Location: home.php"); exit(); }else{ // Our form was submitted but it's invalid! This means we need to // redisplay the form $Out .= ShowForm(); } }else{ // Form not submitted, we need to show it $Out .= ShowForm(); } echo $Out; // Dump our output at the very end // ShowForm // RETURN: The html to display for the form function ShowForm(){ $Form = ''; // Start with an empty variable // First we check for errors global $Errors; if(count($Errors)){ // We have errors $Form .= 'The follow error(s) were encountered:' . '<ul><li>' . implode('</li><li>', $Errors) . '</li><ul>'; } // Set up default values for our form, using the ones from the previous // submission if one was made $defUser = isset($_POST['User']) ? $_POST['User'] : NULL; // Now display the form - we use the post method so that we can use // the $_POST array above $Form .= '<form name="login" method="post" action="">' // Create the login field, using the default . 'Login: <input type="text" name="User" value="' . $defUser . '" />' . ' ' // Create the password field, never set a default password . 'Password: <input type="password" name="Password" value="" />' . '<input type="submit" name="login" value="Login" />' . '</form>'; // Return our form return $Form; } // ValidateForm // This function validates the log in form // RETURN: true if form is valid, false otherwise function ValidateForm(){ global $Errors; // We need access to our errors array $HadErrors = false; // We initially assume our form is valid // We are going to systematically check our field for good data // Any time we find bad data, we set $HadErrors to true and add an error // message to our $Errors array // First we check if the username is valid, the condition to do so varies // based on your application. A valid username might be alphanumeric only, // or alpha only, and usually they have a length restriction. It's a good // idea to test for that here // The value $user_name_is_invalid is a dummy to represent whatever check // you might actually make if(!$user_name_is_invalid){ $HadErrors = true; // Not valid, so mark that we had errors $Errors[] = "Login name appears to be invalid."; } // Now we'll check that the user exists in our database, we make sure to // clean each of the form fields (User & Password) $Clean['User'] = CleanFormField($_POST['User']); $Clean['PW'] = CleanFormField($_POST['Password']); $sql = "SELECT COUNT(*) AS Num FROM UserTable WHERE " . "User=" . $Clean['User'] . " AND " . "Password=" . $Clean['PW']; $q = mysql_query($sql); $HaveUser = false; // Initially we have no user if($q){ // Query successful, let's make sure we have a user while($row = mysql_fetch_array($q)){ $HaveUser = $row['Num'] == 1; // Set $HaveUser to the result of the test break; } } // By now $HaveUser is true or false depending on if we have a user if(!$HaveUser){ // We have no user $HadErrors = true; // Not valid, so mark that we had errors $Errors[] = "Your account could not be found."; // It is very important that when checking an account that you NEVER // tell the user which of the fields is correct or incorrect. // This makes it harder for an attacker to determine if the login // or password they are working with are correct or not } // Now we return the NOT of $HadErrors return !$HadErrors; } // CleanFormField // $fld - the input field to clean // RETURN: $fld cleaned for safe use function CleanFormField($fld){ if(is_string($fld)){ // $fld is a string so we must enclose in single quotes and escape // special characters $fld = "'" . addslashes($fld) . "'"; }else if(!is_numeric($fld)){ // We already knew it wasn't a string, but now we know it's not numeric // either, so trash it $fld = NULL; } return $fld; } ?>[/code] [b]home.php[/b] [code]<?php // home.php // This is our homepage for users session_start(); // The first think we must do is start our session $Out = ''; // This variable is going to hold our final output for the page // First check if we have a valid user if(!isset($_SESSION['LoggedIn']) || $_SESSION['LoggedIn'] !== true){ // Invalid user is trying to hack our site! $Out .= 'You do not have permission to view this page.'; }else{ // User is valid - print welcome message $Out .= "Welcome, {$_SESSION['User']}!"; // THIS IS WHERE YOU'D PULL MORE INFORMATION FROM THE DATABASE DEPENDING // ON WHICH USER HAS LOGGED IN AND DISPLAY IT TO THEM! $Out .= $more_info_from_database; // Let's also print out some debugging information $Out .= '<pre style="text-align: left;">' . print_r($_SESSION, true) . '</pre>'; } echo $Out; ?>[/code] -
User management accounts - private page per user....?
roopurt18 replied to Skeleten Neteleks's topic in PHP Coding Help
Glad to see you're working on the DB approach, the effort will pay off immensely! Now, one thing you might want to add to your Products table is a uniqueness constraint on the product name. This will prevent the same product from being entered multiple times. [code]<?php mysql_query("CREATE TABLE Products( id INT NOT NULL AUTO_INCREMENT, PRIMARY KEY(id), Name VARCHAR(50), Description TINYTEXT, // Notice the added comma UNIQUE(Name))") // And here is the uniqueness constraint or die(mysql_error()); ?>[/code] Now if you enter a product "Sam's Jelly" and later try to insert it again, the database will not insert the new record. This is dependent on if you want to prevent duplicate rows. You can do the same thing with the UserProductLinks table to ensure that each user is only associated with each unique product once. [code]<?php mysql_query("CREATE TABLE UserProductLinks( id INT NOT NULL AUTO_INCREMENT, PRIMARY KEY(id), user_id INT, product_id INT, // Note the added comma UNIQUE(user_id, product_id))") // The constraint, notice how we can enforce uniqueness across column combinations or die(mysql_error()); ?>[/code] You could use a similar constraint on the username column of your user table. The advantage to this approach is for tables that need to have unique records you no longer have to check if the record exists before inserting a new one as the constraint will prevent it from inserting duplicates in the first place. Now, for your script that is pulling data from the database: [code]<?php <?php // *** ATTENTION *** // IN YOUR POSTED CODE YOU DISPLAYED YOUR HOST, USERNAME, AND PASSWORD // EDIT THAT INFORMATION OUT ___NOW___ mysql_connect("host", "user", "password") or die(mysql_error()); mysql_select_db("table") or die(mysql_error()); /* I'm commenting out this entire block since it's unnecessary. You only need to do this if you want to display all of the users in your system, which is not what we want. // Retrieve all the data from the "example" table $result = mysql_query("SELECT * FROM Users WHERE 1") or die(mysql_error()); */ /* I'm commenting out this block for the same reason as the previous mysql_query call $result = mysql_query("SELECT * FROM Products WHERE 1") or die(mysql_error()); */ $result = mysql_query("SELECT * FROM Users u, Products p, UserProductLinks l WHERE l.user_id = u.id AND l.product_id=p.id") or die(mysql_error()); // YOU STILL NEED TO LOOP OVER THE $result // store the record of the "example" table into $row while($row = mysql_fetch_array( $result )){; echo "Your user name is: ".$row['Username']; echo "<p>Your Password: ".$row['Password']; echo "<p>Product Name: ".$row['Name']; echo "<p>Description: ".$row['Description']; } ?>[/code] That will display any users who are linked to products. What you are probably missing is entries into your UserProductLinks table. If you have 2 user accounts, their ids are probably 1 and 2. Likewise for your 2 products. Try inserting the following rows into UserProductLinks [code] +------------------+------------------+ | user_id | product_id | +------------------+------------------+ | 1 | 1 | | 1 | 2 | | 2 | 1 | | 2 | 2 | +------------------+------------------+ [/code] Try that and watch the results. Then remove rows from the UserProductLinks table and watch what happens. -
K, that's the missing piece I was looking for.