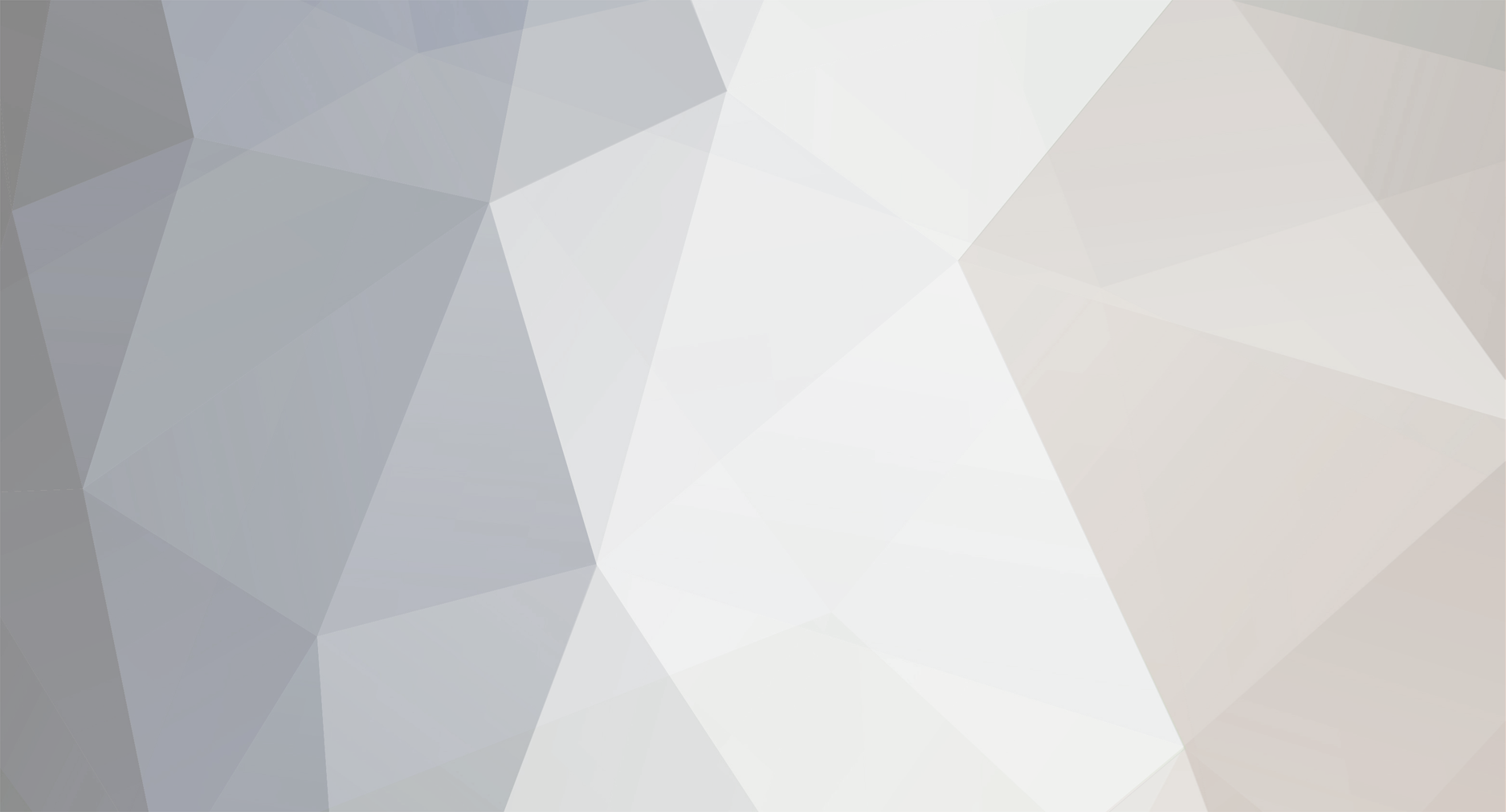
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
The problem is that the first while loop pulls the records from the first query. Then you have an inner while loop to go through the results of the second query. So, on the first iteration of the outer loop the second loop is consuming all of the records from the 2nd query. So, on the second iteration of the outer loop there are no records for the inner loop to process. But, to put it bluntly, you are doing it wrong. You should NEVER run queries within loops. In many cases, such as this one, you should be using a JOIN query. This will be much more efficient. The JOIN query below will provide a record set of all possible selections and JOIN the checked options of the user. I used a dynamically created variable "checked" that will be set to a 1 if it was checked by the user, otherwise it will be 0. //Create and run ONE query to get all the checkbox options and which ones //are checked for the users $query = "SELECT wi.id, wi.description, IF(wi.id=sw.item_id., 1, 0) AS checked FROM widget_item AS wi LEFT JOIN selected_widgets AS sw ON wi.id = sw.item_id AND sw.sessionId = '$sessionid'"; $result = mysql_query($query) or die(mysql_error()); //Process the records into output while ($row = mysql_fetch_assoc($result)) { //Set variable for whether the option is checked or not $checked = ($row['checked']) ? ' checked="checked"' : ''; $out .= "<input name='items[]' type='checkbox' value='{$row['id']}' id='{$row['id']}'{$checked} />"; $out .= "<label for='{$row['id']}'>{$row['description']}</label><br />\n"; }
-
Displaying The User Information Using Post Method Undefined Variable
Psycho replied to zenmind's topic in PHP Coding Help
Yes, I did. I also noticed that the URI for the CSS file is pointing to a folder on the University of Washington domain under a folder for a computer science course. Based upon the very basic code presented here it I think it's safe to say that the person is doing an assignment and just chose a very poor choice for the script. On the off chance that s/he is trying to do something malicious, if they can't put together a simple form processing script using just three fields I don't think we are in danger of getting scammed. -
Displaying The User Information Using Post Method Undefined Variable
Psycho replied to zenmind's topic in PHP Coding Help
And what would those problems be? Are you getting error messages? if so, what are they. Are they doing something differently than you expect? If so, what do you expect and what are they doing? -
Right, if the fields are ints (which they should be) then the VALUES() is not needed. BUt, I just realized you said this The ON DUPLICATE KEY only works if the INSERT data will cause a duplicate in a field set to be unique. Has the 'workerusername' field been set up to be unique? If so, give this a try: $workerusername = $_POST['workerusername']; $addedtogether = intval($_POST['question1']) + intval($_POST['question2']) + intval($_POST['question3']); $query = "INSERT INTO survey (username, voted, total) VALUES ('$workerusername', '$addedtogether', '30') ON DUPLICATE KEY UPDATE `voted` = `voted` + $addedtogether, `total` = `total` + 30"; mysql_query($query) or die("Query: $query<br>Error: ".mysql_error()); echo "Query run: $query"; //Leave this uncommented for debugging echo "<center>Thank you for your feedback!</center>"; Note, by not escaping the $_POST['workerusername'] you are opening yourself up to SQL Injection. Besides you should be passign an ID and not a username.
-
1. How do you add varchars? Even though it may work, it's not good process. Change the fields to ints (or floats) 2. Since those fields are varchar's - which they shouldn't be - you would need to enclose the values in quotes. Again, change the fields to ints (or floats). 3. You have no error handling of your query. You SHOULD really change the field types. But, this would work with what you have $workerusername = mysql_real_escape_string(trim($_POST['workerusername'])); $values intval($_POST['question1']) + intval($_POST['question2']) + intval($_POST['question3'])); $query = "INSERT INTO survey (username, voted, total) VALUES ('$workerusername', '$values', '30') ON DUPLICATE KEY UPDATE `voted` = $values + VALUES(`voted`), `total` = 30 + VALUES(`total`)"; mysql_query($query) or die("Query: $query<br>Error: ".mysql_error());
-
I can think of a fairly strait-forward simple solution. But, first off you need to determine what gives one user precedence over another. If too many people selected table B as their first choice, who gets table B and who will be kicked out to another table. I don't know what data you do and do not have, but I would base it upon the timestamp of when each user made their choice. But, here is what I would do 1. Do a select query for all tables using only the ID. Process these results into an an array with the ID as the key and an empty sub-array as the value. This will be the assigned array. 2. Do a select query on the users including their choices for 1 2 and 3 3. As you process the records above you will check if a user's first selection is available in the assigned array created on step 1. If so, add them and skip to the next record. If not you check thier second and third selections. If none of the user's choices are available put the user into an unassigned array until you've finished processing all the users. 4. After all the users have been processed to put them into one of their three choices, go back through the unassigned array (users' who's first, second and third choices were at capacity) and randomly put them into the tables that have capacity. Here is a quick script that appears to work. However, I hard coded the data I would generate from a DB query for testing since I didn't feel like creating DB tables. So you will need to modify accordingly <?php //Mock DB results for tables $tablesDB = array('Table A', 'Table B', 'Table C', 'Table D'); //Mock DB results for users and their selections $userSelectionsDB = array( array('userID' => 0, 'sel_1'=>0, 'sel_2'=>1, 'sel_3'=>2), array('userID' => 1, 'sel_1'=>1, 'sel_2'=>3, 'sel_3'=>2), array('userID' => 2, 'sel_1'=>0, 'sel_2'=>1, 'sel_3'=>2), array('userID' => 3, 'sel_1'=>0, 'sel_2'=>1, 'sel_3'=>2), array('userID' => 4, 'sel_1'=>0, 'sel_2'=>1, 'sel_3'=>3), array('userID' => 5, 'sel_1'=>0, 'sel_2'=>1, 'sel_3'=>2), array('userID' => 6, 'sel_1'=>2, 'sel_2'=>1, 'sel_3'=>0), array('userID' => 7, 'sel_1'=>0, 'sel_2'=>1, 'sel_3'=>2), array('userID' => 8, 'sel_1'=>0, 'sel_2'=>1, 'sel_3'=>2), array('userID' => 9, 'sel_1'=>1, 'sel_2'=>0, 'sel_3'=>2), array('userID' => 10, 'sel_1'=>2, 'sel_2'=>1, 'sel_3'=>0), array('userID' => 11, 'sel_1'=>2, 'sel_2'=>1, 'sel_3'=>0), array('userID' => 12, 'sel_1'=>0, 'sel_2'=>1, 'sel_3'=>2), array('userID' => 13, 'sel_1'=>0, 'sel_2'=>1, 'sel_3'=>2), array('userID' => 14, 'sel_1'=>2, 'sel_2'=>0, 'sel_3'=>1), array('userID' => 15, 'sel_1'=>2, 'sel_2'=>0, 'sel_3'=>1), array('userID' => 16, 'sel_1'=>0, 'sel_2'=>1, 'sel_3'=>2), array('userID' => 17, 'sel_1'=>2, 'sel_2'=>0, 'sel_3'=>1), array('userID' => 18, 'sel_1'=>0, 'sel_2'=>1, 'sel_3'=>2), array('userID' => 19, 'sel_1'=>3, 'sel_2'=>1, 'sel_3'=>0) ); //Number of people that can fit at a table $tableCapacity = 5; //Create array of table assignments $assignments = array(); //This foreach loop would be replaced with a DB query and while loop foreach($tablesDB as $tableID => $tableName) { $assignments[$tableID] = array(); } //Array to temporarily assign usrs whose choices are already full $unassignedUsers = array(); //Assign users to tables based upon their preferences //This foreach loop would be replaced with a DB query and while loop foreach($userSelectionsDB as $row) { if(count($assignments[$row['sel_1']]) < $tableCapacity) { $assignments[$row['sel_1']][] = $row['userID']; } elseif(count($assignments[$row['sel_2']]) < $tableCapacity) { $assignments[$row['sel_2']][] = $row['userID']; } elseif(count($assignments[$row['sel_3']]) < $tableCapacity) { $assignments[$row['sel_3']][] = $row['userID']; } else { $unassignedUsers[] = $row['userID']; } } //After all users are placed into one of their three preferences if capacity allows //If any were not able to be placed, assign them to tables where there is capacity foreach($assignments as $tableID => $assignedUsers) { $remainingCapacity = $tableCapacity - count($assignedUsers); if($remainingCapacity > 0) { $assignments[$tableID] = array_merge($assignedUsers, array_slice($unassignedUsers, 0, $remainingCapacity)); $unassignedUsers = array_slice($unassignedUsers, $remainingCapacity); } if(!count($unassignedUsers)) { break; } } echo "<pre>" . print_r($assignments, 1) . "</pre>"; ?>
-
Have you checked what is stored in the Database? If the value in the DB is only one letter then the problem is in the saving of the data - not the display. Could be the PHP code it truncating the value or, perhaps, the DB field was set up with a length of 1. In the latter case the value will be automatically truncated when you save a record. In the code above I see the following: while($row1=mysql_fetch_array($result1)) { $name[$j]=$row1['name_with_initial']; } I don't know why you would name a field as "name_with_initial", but anyway, that loop makes no sense. If you have a while() loop I would assume the query could be returning multiple records [Note: if a query would only return one value then don't use a while() loop to extract the record]. But, that loop will only overwrite the previous value on each iteration since the value of $j is not changing. So, $name[$j] will only contain the last value when the loop completes. But, I don't even see that value used anywhere in the code that follows. So, where is the output - exactly - that is causing the problem? Note: There are a lot of other problems with that code (error handling, SQL Injection exploitable, etc.), but too much to go into here. EDIT: I also see you are running a query within a loop - you need to learn how to do JOINs in your queries.
-
I mean the page isn't calling the Javascript function. I've stumbled upon something while checking to see if it was maybe the session and it appears it isn't. I added another print function displaying some random string of text and it works. And I just changed the code around to where there's a inclusion of a php file which holds the session info. You need to check HTML source to see if the line of JS code was written to the page or not. If so, then this is a JS issue. If not, then the if() condition is returning false. Again, I go back to why is there no session_start() in the code for the first page.
-
Start by creating a description of the rules for the pattern as you would describe to another human. Then figure out how you will do it in the code. In this case I would describe it as follows: Starting with the number 1, perform the following process for 5 iterations: On each iteration create a number that 1) starts with the digit matching the iteration count 2) contains sequentially numbered digits up to the same number as the iteration count
-
You say it isn't working, but what does that mean - exactly? Is the page getting directed and you just aren't getting the popup (which would seem to indicate a JS problem) or is it not getting redirected at all? You also say that the code you provided for the first page is the entirety of the code for that page. Where's the session_start() statement? Also, wouldn't you want to unset that session value after echoing the javascript code?
-
Help Joining These Queries (A Cron Job That Is Destroying My Site)
Psycho replied to acidpunk's topic in PHP Coding Help
Personally, based on my understanding, I think all these update queries are completely unnecessary! As I said in my last post: And, it seems that is exactly what you are trying to do. You are populating total values for hp, attack, experience, etc. based upon all the equipped items of the users. You should avoid trying to store "summation" data based upon other data unless there is a clear reason to do so. You can run into all sorts of problems due to race conditions and data just getting out of sync. Using the query I provided previously you can dynamically determine the totals for hp, attack, experience, etc. with a single query. But, if you find that calculating those values dynamically (the best option, IMO) is too system intensive and you need to actually store those summed values, you don't even need two queries - just one will work no matter if the user added or removed a single item or multiple items. Just use the query I provided previously for the purposes of the update. This is somewhat complicated, so I can't guarantee there are no errors since I don't have your database. But, the following query would update the totals based upon all the currently equipped items. No need to do a "set max_attacks=max_attacks-'$max_attacks'" $query = "UPDATE users AS u JOIN (SELECT SUM(attack) AS attack, SUM(hp) AS hp, SUM(critical) as critical, SUM(attacks_per_hour) as attack_per_hour, SUM(max_attacks) AS max_attacks, SUM(attack_cap) as attack_cap FROM user_equipment WHERE equipped = 'Yes' AND owner = '$userID' ) as totals ON u.id = totals.owner SET u.attack = sums.attack, u.hp = sums.hp, u.critical = sums.critical, u.attacks_per_hour = sums.attack_per_hour, u.max_attacks = sums.max_attacks u.attack_cap = sums.attack_cap WHERE u.id = '$userID'"; With the above you can process multiple add/removes of equipment and then run a single query to update the totals for a user. -
Help Joining These Queries (A Cron Job That Is Destroying My Site)
Psycho replied to acidpunk's topic in PHP Coding Help
If this is a cron job I suspect you are doing more than just SELECT queries. You should probabnly provide more details on what, exactly you are tryig to achieve. Depending on what you are really doing, this process may not even be needed. At least, it's usually not necessary to calculate sums within a cron job since that can be done in real time. You also don't show what that last while loop is doing. If there are any queries in there, then you need to change that as well. Never, ever run queries in loops. In 99% of cases you can do it without looping queries. In the other 1% you're probably just doing something stupid :/ -
Help Joining These Queries (A Cron Job That Is Destroying My Site)
Psycho replied to acidpunk's topic in PHP Coding Help
Stop querying all those fields if you don't need them. In the first query you are querying about 10 fields but only using the user ID field Assuming the list of 'slots' you have in your otiginal queries are all th epossible slot values, the below should work for you. I assume you need a name or other informatin from the users table to associate with the data, but I didn't see anything in the original query. Just add those fields to the top line of the SELECT statement: $query = "SELECT username, SUM(attack) AS attack_sum, SUM(hp) AS hp_sum, SUM(critical) as critical_sum, SUM(attacks_per_hour) as attack_per_hour_sum, SUM(max_attacks) AS max_attacks_sum, SUM(attack_cap) as attack_cap_sum FROM users LEFT JOIN user_equipment ON users.id = user_equipment.owner WHERE equipped='Yes' GROUP BY users.id"; -
No, because this would fall into one of four scenarios: 1. He knows this is a bad configuration, and he has control over it. In this case he is a moron and doesn't deserve our help. 2. He knows this is a bad configuration, and he doesn't have control over it. In this case he should have stated that fact since he does no better. So, I doubt this is likely. And he probably still doesn't deserve our help for not providing that information, thereby wasting our time. 3. He doesn't know this is a bad configuration, and he has control over it. This is the most likely case. I see people doing these types of things all the time when they start using databases. Which is what prompted my, and probably ManiacDan's, responses 4. He doesn't know this is a bad configuration, and he doesn't have control over it. This is a possibility. But, I think we are still right to point out the major flaw in the design. Maybe I was a little harsh in my response that But I still think that scenario #4 is very unlikely. If the OP can provide a statement otherwise then I might be willing to help. But, I have seen too many people post crappy logic/code/processes asking for help with a specific piece. Then when someone responds that they are taking the wrong approach they get pissy and say they just need help with that one thing. I'm not trying to be the over ambitious hall monitor for what is considered "correct" code. But, I think it is relevant of us to push people in the right directions when it is obvious they are taking approaches that are fraught with problems. Else we will be helping them the next day on another problem that could have been avoided if they did it correctly, and then the next day, . . . As I said before, I see people new to databases trying to do similar things all the time (even I did in the beginning). I only wish I had someone helping me to avoid those mistakes early on.
-
Trying To Add / Hide Certain Menu Links Using Php
Psycho replied to kjetterman's topic in PHP Coding Help
@darfreaks, Not sure what you are doing, except complicating the problem. There was nothing wrong with the original logic (except one thing). trying to introduce things such as heredoc syntax to someone who is not familiar is only going to complicate the issue. @kjetterman, The only problem I see with the code you posted in response to my post is that you are not defining $loggedIn correctly. You simply hard coded it to be a partial URL path. I noticed that in your first post you were using some logic such as if ($currentpage == $loggedin) Where $currentpage and $loggedin were the Request URI and a hard coded value. I have no idea why that would be the determining factor as to whether a user is logged in or not. Which is why I put in my code: //Set var $loggedIn to true/false I guess I should have been more explicit. You need to have a process when the user logs in to set a value. This is typically done using a session value. You would then check that session value on each page load to determine if the user is logged in or not. Using the URI makes no sense. -
I concur with kicken. Yes, it can hurt. You likely have a process to allow users to edit blogs. You may end up with multiple methods of editing a blog. You would then have to ensure each method is updating both the database and the flat file consistently. Plus, you would be using resources that would be better utilized elsewhere. Plus, editing/updating flat files is much more problematic than database records and could lead to errors. I think you wanting to do this comes from a lack of having much experience with databases. I know that in my early days of programming I preferred to store some data in a file rather than in a DB because I just "didn't get it".
-
Trying To Add / Hide Certain Menu Links Using Php
Psycho replied to kjetterman's topic in PHP Coding Help
I'm really not following your code or your statement that "both div classes show at the same time regardless". That would be impossible based upon that if/else condition. Since I assume the links are inside the divs you need to put the logic where you output the links - not the containing div(s). //Set var $loggedIn to true/false echo "div class='two-thirds2'>"; //Standard links for all users echo "<li><a href="#">Link 1</a></li>\n" echo "<li><a href="#">Link 2</a></li>\n" echo "<li><a href="#">Link 3</a></li>\n" //Links for logged in users if($loggedIn) { echo "<li><a href="#">Link 4</a></li>\n" echo "<li><a href="#">Link 5</a></li>\n" echo "<li><a href="#">Link 6</a></li>\n" } echo "</div>\n"; -
You're right. I get PHP and Javascript formats confused some time and mistook the substr() function as valid PHP.
-
Which is exactly what you should NOT be doing. You should not create multiple tables to differentiate data between stores! You should have ONE table for all the products and have an additional column to identify which store each product is associated with. What you are trying to do will only add complexity and cause you to run into more issues as you try to develop further features. Your core_store_group table should have fields such as: store_id, store_name, etc. Then you should have one table for catalog_product_flat with all the same fields you have now, but it should have one additional field for store_id Basically, we can't (or won't) help you with the current database structure.
-
Pretty basic stuff: $query = "SELECT report_id FROM reports_table"; $result = mysql_query($query); while($row = mysql_fetch_assoc($result)) { $reportID = $row['report_id']; //Insert code to run curl process, use $reportID as needed. Example: $curlURL = "http://www.somedomain.com/get_report?id=$reportID"; } You just need to find where the report ID is used in your current code and replace with the variable you create when iterating through the results.
-
Yeah, that's PHP, he is trying to remove the last 'UNION ALL' at the end of the concatenated query that he thinks he was building. But, yeah, completely wrong approach. There should be one table not many.
-
I can't find a link to the forum rules since the site changed, but I know that one of them if that any requests to have code written for you should be posted in the freelance section. ManiacDan provided some guidance on things you need to check. So, what have you done with that information other than respond back asking for someone to do the work for you? We are here to help, but we want the person receiving the help to learn in the process. So, I'll give you this. Whenever running a query I suggest always creating the query as a string variable (which you are doing) so that you can echo the query to the page for debugging purposes when there are problems (which you are not doing). If you echo'd the query to the page you would see several problems - there are syntax errors and you are not generating the query you think you are. You are overwriting $sql on each iteration of the loop - not concatenating the queries as I think you intended. But, you need to take a step back. There should be no reason to have tables such as catalog_product_flat_1, catalog_product_flat_2, catalog_product_flat_3, etc. You should have just 1 table for that data. You can add an additional field in that table to further categorize/separate the data by core_store_group if that is what you need. If you set it up correctly you only need one single query to get the information you need. So, instead of fixing the code above I would highly suggest fixing your database structure first. Otherwise you are just creating more problems as you progress further.
-
I see nothing to be gained from storing the same content in the DB as well as in a flat file. It would take more overhead and create greater opportunity for the content to get out of sync between the two. Besides, if you can't rely upon whatever storage method you are using then you are using the wrong method. As to what field type you use is dependent upon how much and what type of content you would be storing. Longtext would work (supporting up to 4,294,967,295 characters), but that might be overkill to me. I would think that a medium text (supporting up to 16,777,215) characters would more than suffice. That would be more than 4,000 pages of content on a normal double-spaced printed page! By comparison a "normal" text/blob field (65,535 characters) would provide room for up to 16 pages of content on a double-spaced printed page. There are performance/storage costs to using larger fields than needed. But, to be honest, I'm not sure of all the ramifications. So, I would use the smallest field type that is larger than anything you would ever expect to have to save.
-
While I agree with Jessica's response as to why it doesn't work and how you can make it work with array_pop(), there is no reason to explode the string into an array. You can accomplish this with a simple string function: strrchr() echo strrchr($occurrence->Description, ' ');
-
Beginner In Php Need Help With A Music Playlist App
Psycho replied to zenmind's topic in PHP Coding Help
OK, so what did I tell you before? Do one thing, make sure it works, then do the next thing. So, now you know you have the data in an array. Now loop over the array to echo the file name (which I assume to be the same as the song name) to the page. Then you'll need to create a link to each file so the user can play them. You'll need to make these links relative to the file from the root of the web folder, not the C drive.