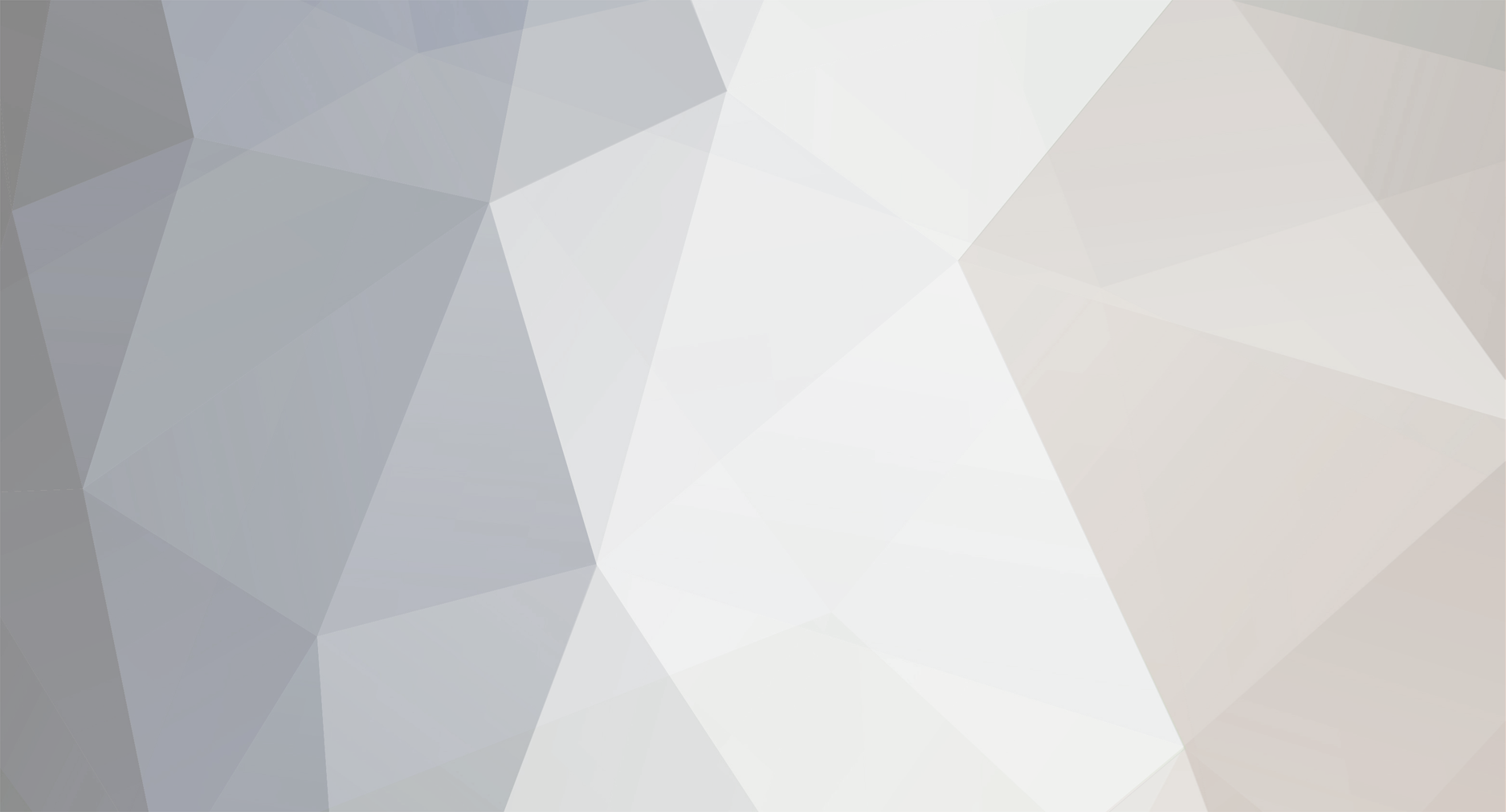
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Here's a solution that I found via Google. 'dob' represents the Date Of Birth SELECT DATE_FORMAT(NOW(), '%Y') - DATE_FORMAT(dob, '%Y') - (DATE_FORMAT(NOW(), '00-%m-%d') < DATE_FORMAT(dob, '00-%m-%d')) AS age FROM table_name
- 12 replies
-
- calculate age
- data field
-
(and 1 more)
Tagged with:
-
Well, you did state data type. What type of database are you using? MySQL and MS SQL do not store dates in the format 20.02.1989, they would use 1989-02-20
- 12 replies
-
- calculate age
- data field
-
(and 1 more)
Tagged with:
-
You should first fix the database to change that field to a date type. The database has lots of useful functions and processes for working with dates, but you can't use them on data that is not a date type. Otherwise, your best option is to read the data into PHP, parse it into a date format and then do the calculation. EDIT: My response above is based on what I know date types to by in MySQL and MS SQL. You may be using a different database that stores dates differently.
- 12 replies
-
- calculate age
- data field
-
(and 1 more)
Tagged with:
-
How important is it to maintain the markup - for the summary? I understand you want the markup for the actual output, but why not remove tags to generate the summary?
-
My thought was to keep it available to repurposed for allowing the configurations to be changed. I believe this user has a separate post about creating a multi-step configuration setup for his site. I think it is useful to allow users to change configurations later on. The process I typically use is to have a core script that is always executed for any page request. That page would load the configuration data, among other things. That way, if the configuration data doesn't exists it would dynamically load the process to run the configuration. But, I see where you are going, the pages to set the configuration should not be generally accessible. So, if the configuration file does exist, I would only allow logged in users (with the right permissions) to rerun the configuration script. That might be a lot of overhead depending on the application.
-
$step = isset($_SESSION['step'])) ? intval($_SESSION['step']) : 0; But, I'm not going to give you a tutorial of how to set and manage session variables. Go do some research first.
-
Personally, I would use session values instead of values on the query string, but since that is what you are using: $step = isset($_GET['step'])) ? intval($_GET['step']) : 0;
-
I was bored. Here is a working example. Still needs some cleaning up. <?php $configTemp = "config.tmp"; $configFile = "config.php"; if($_SERVER['REQUEST_METHOD']=='POST') { //Get the POST values $hostname = isset($_POST['hostname']) ? trim($_POST['hostname']) : ''; $database = isset($_POST['database']) ? trim($_POST['database']) : ''; $username = isset($_POST['username']) ? trim($_POST['username']) : ''; $password = isset($_POST['password']) ? trim($_POST['password']) : ''; //Validate the data $errors = array(); // E.g. check that all fields are provided and are the right format // This is just one check if(empty($hostname) || empty($database) || empty($username) || empty($password)) { $errors[] = "All fields are required"; } //If no errors, run check to verify that values CAN be used to make a connection if(!$errors) { $dbc = @mysqli_connect($hostname, $username, $password, $database); if (mysqli_connect_errno()) { $errors[] = "Failed to connect to MySQL: " . mysqli_connect_error(); } } //If still no errors, save data to config file if(!$errors) { //If config file does not exist, create it from temp if(!is_file($configFile)) { copy($configTemp, $configFile); } //Read the contents of the current file $content = file_get_contents($configFile); //Replace the values in the content $content = preg_replace("#hostname = '[^']*'#s", "hostname = '{$hostname}'", $content); $content = preg_replace("#database = '[^']*'#s", "database = '{$database}'", $content); $content = preg_replace("#username = '[^']*'#s", "username = '{$username}'", $content); $content = preg_replace("#password = '[^']*'#s", "password = '{$password}'", $content); //Write contents back to config file file_put_contents($configFile, $content);; //Redirect to success page (followed by exit) so the form isn't loaded //Using an echo here to simulate echo "The config file was updated. Click <a href=''>here</a> to reload the page"; exit(); } } //If config file exists, read the current values so we can populate the form if(is_file($configFile)) { //Read the current values by including it include($configFile); } //If errors exists, create error message if($errors) { $errorMsg = "The following errors occured:<br><ul>\n"; foreach($errors as $error) { $errorMsg .= "<li>{$error}</li>\n"; } $errorMsg .= "</ul>\n"; } ?> <html> <head></head> <body> Provide Database Configuration<br><br> <?php echo $errorMsg; ?> <form name='form' action='' method='post'> Host: <input type='text' name='hostname' placeholder='Database host' value="<?php echo $hostname; ?>" /><br /> Database:<input type='text' name='database' placeholder='Database name' value="<?php echo $database; ?>" /><br /> Username: <input type='text' name='username' placeholder='Database username' value="<?php echo $username; ?>" /><br /> Password: <input type='password' name='password' placeholder='Database password' value="<?php echo $password; ?>" /><br /><br /> <input type='submit' name='submit' value='Change values' /> </form> </body> </html>
-
Yes, but . . . it would make more sense to re-purpose this code for the scenario of someone updating the configuration - i.e. moving a new DB. I would do this. 1. Create the default config file with a "temp" name, e.g. config.tmp. 2. On load of the page, check if the file exists 3A. If no, load the form for the user assuming they've never configured the settings. Upon providing the data (and validating it), COPY the temp file and run the logic to replace the values 3B. If the file does exist, read in the current values and display the form populating the current value. Once the user submits the new values, and you validate it, replace the values in the file The reason I would use a temp config file and copy it, as opposed to renaming it, is that it provides the user an easy way to rerun the initial setup. All they need to do is delete the config file and relaunch the application.
-
help on designing CBT (computer based testing) in php
Psycho replied to valdes's topic in PHP Coding Help
You really haven't provided enough information. But, I'll provide a general response. When the user starts a test you need to create a DB record for that test execution. It would only include information such as the user, date, time etc. You would have another table to store the questions and answers that the user responds with. Your tables for the questions and possible answers should already have a value to identify the correct answer - so the results tables do not need to have a value for wrong or right since that can be calculated. Anyway, once the user starts a test you need to associate them with that test. You can do this through the sessions and/or their user ID (if they are required to log in). Once a user answers a question and proceeds to the next one, you will know who they are, what test they are working on and what their results have been so far. Also, once the user initiates the action to start a test I would do the following: Query all the available questions on the test and populate the results table - leaving the answer NULL. The table could have the following fields: ID (PK), question_id, answer_id and sequence. The sequence would be so you can separately track which question the user last answered so you know which one to show them next. If the user is required to answer each question you could select the first one in the sequence order that is unanswered. Otherwise, you could allow a user to skip questions and would need to track which question to show next in session data. So, backing up, upon selecting to take a test you would run two queries: one to populate the test execution table and a second to populate the questions to be asked. Then on each page load, select the next question to show the user from the results table. You would JOIN that table on the answers table to get the available answers. When a user answers a question, store their response in the results table. If you need to show them immediately if their answer was right or wrong, you would also do a SELECT query on that answer to the answers table to see if their response was correct. I know this is just general information and I haven't provided any specifics or code, but it is not a trivial amount of work and I'm not prepared to do it at this time. -
Try this: SELECT if(type=13, '13', '40-46') as type_group, COUNT(*) as type_count FROM table_name WHERE type in (13, 40, 41, 42, 43, 44, 45)
-
It's the form handler code that needs to be modified.
-
So, you don't want the address to be displayed in a select list - that is important information. Do you have code that creates the email or are you using some 3rd party script for the email? You could start by using a separate value from the label for the options: [coed]<li><select id="shipaddress" name="shippingaddress" dir="ltr" lang="en"> <option value="80 Allstate Parkway<br />Markham ON<br />L3R 6H3">80 Allstate Parkway, Markham ON, L3R 6H3</option> <option value="30 High Meadow, Toronto ON<br />M6L 2Z5">30 High Meadow, Toronto ON, M6L 2Z5</option> <option value="4000 Victoria Park Avenue<br />Toronto ON<br />M2H 3P4">4000 Victoria Park Avenue, Toronto ON, M2H 3P4</option>[/code] If you are using a 3rd party script to generate the emails, it will likely take more effort to find and modify the right code to work properly. But, if you wrote the code to generate the email, then show the lines that generate the content from the POSTed values.
-
Didn't he just say line breaks are not supported in SELECT lists? The existence or not of a comma has nothing to do with it. If you weren't including this content in an email, you could use JQuery to build a "custom" select list object to display it however you want. But, I am pretty certain you can't run JavaScript in an HTML email. If you absolutely need to display with line breaks, you would have to provide a link into the email to open the form in a web page.
-
Personally, I thing you are taking the wrong approach. Trying to backward-engineer a non-trivial sort order may never be 100% accurate. There could be nuances with specific characters that would take a lot of time and effort to verify. Just not worth the effort. I don't use Adobe Lightroom, so I can't provide specific instructions, but I think a possible better approach is to utilize AL to tell you what the sort order would be for a list of values. I would set up a page that takes a list of values as input and outputs the list using AL and apply the sort from AL. Then read the output of that page to determine how AL sorted the records. You can then apply a sort order attribute to your values. Hopefully, this is just needed one time when setting up new records and not needed every time data is displayed as that could incur unnecessary overhead.
-
This line of code is invalid list($width, $height) = getimagesize('$img_url'); If you put a variable in a double quoted string it will be parsed as the value of the variable. But, if you put a variable in a single quoted string, as shown above, the characters of the variable name will be interpreted as those literal characters! Example: $img_url = "\path\to\image\imagename.jpg"; echo "$img_url"; //Output: \path\to\image\imagename.jpg echo '$img_url'; //Output: $img_url In your usage, no quotes are even needed, just use this list($width, $height) = getimagesize($img_url);
-
Hmm, I worked on a usort solution that compares each character between words and almost finished it, but there was one problem. You don't want numbers treated as individual characters. So, "abc2" would come before "abc10". That makes it much more difficult. What if the values were: abc10def abc1def abc2def Does the "abc10def" come before "abc2def" or the other way around?
-
jquery price slider not connect with php code
Psycho replied to Hannah123456's topic in Javascript Help
When you have separate technologies (PHP & JavaScript) working together you need to test each one independently to find the problem. I would start with the PHP page. Add a line of code to output the value that should be set from the JavaScript. If the value isn't there, then you know it isn't being set by the JavaScript and you need to look in that code for the problem. If the value is there, then you know the problem is within the PHP code that processes the value. Also, it is a bad idea to put non-numeric content in a field intended for numeric content. Specifically, you are putting the '£' character into the input field with javascript and then stripping it out in the PHP. Just put the '£' as text to the left of the field. Inserting/stripping characters, especially with two different parts of code it just adding complexity for the sake of complexity with no real value. Looking further, the PHP code is flawed $values = str_replace(array(' ', '£'), '', $_POST['amount']); list($min, $max) = explode(' ', $values); That first line is replacing any spaces or pound symbols with an empty string. But,then the next line is trying to explode on the spaces character - but there won't be any. Why make this difficult. Just create two fields to hold the min/max values (without any extraneous characters) and use those in the POST fields. If you want to display the values to the user, you can still do that without adding the additional complexity you have now. -
Yes, you can do outline font in CSS, although it won't be supported in all browsers: https://css-tricks.com/adding-stroke-to-web-text/ body { font-size: 24pt; -webkit-text-fill-color: #ffffff; -webkit-text-stroke-width: 1px; -webkit-text-stroke-color: #000000; }
-
"Not working" is not helpful to solve your problem. You do state But, that doesn't make sense to me. A LIMIT has nothing to do with what row a record is in the database. It has to do with the order of the rows in the result set. One way to determine if a LIMIT is working correctly is to run the query without a limit and see what is returned. It makes no sense to use limit to determine the records for a quarter - that should be determined by a date value in the query.
-
get new num_rows count after filtering mysqli_fetch_array rows via php
Psycho replied to seany123's topic in PHP Coding Help
If you need this for pagination, you definitely need to find a solution to filter via the DB - else you are going to need to create overly complicated logic to do pagination. -
PHP & MySQL - Calculation before inserting data into table
Psycho replied to rvdveen27's topic in PHP Coding Help
Ideally, you would store those values (price, damages & cost) in the database. Then you would dynamically calculate the profit, as needed, in your SELECT queries. Only storing the calculated value results in less fidelity. Plus, what if you're asked to provide data such as profit as a percentage of costs or average damage per trip? -
If you were to add some debugging (such as testing variable values at different stages) I'm sure you would have found the problem. But, I'm too lazy right now to provide a tutorial on how you shoudl debug, so I'll just give the reason your code is not working. Well, not the reason so much, but the specific line of code that is the source of your problem. if ( ($username == "") || ($email =="") || ($password1 =="") || ($password2 =="") || ($userfirstname = "") || ($lastname == ""))
-
Is there a way to format the date output of a function?
Psycho replied to OGBugsy's topic in PHP Coding Help
I wasn't saying it did. I use PDO as I didn't like the mysqli_ extensions for prepared statements. There is no bind results comparative function in PDO. I just use the fetch method as an object or array and reference the value using the column name.