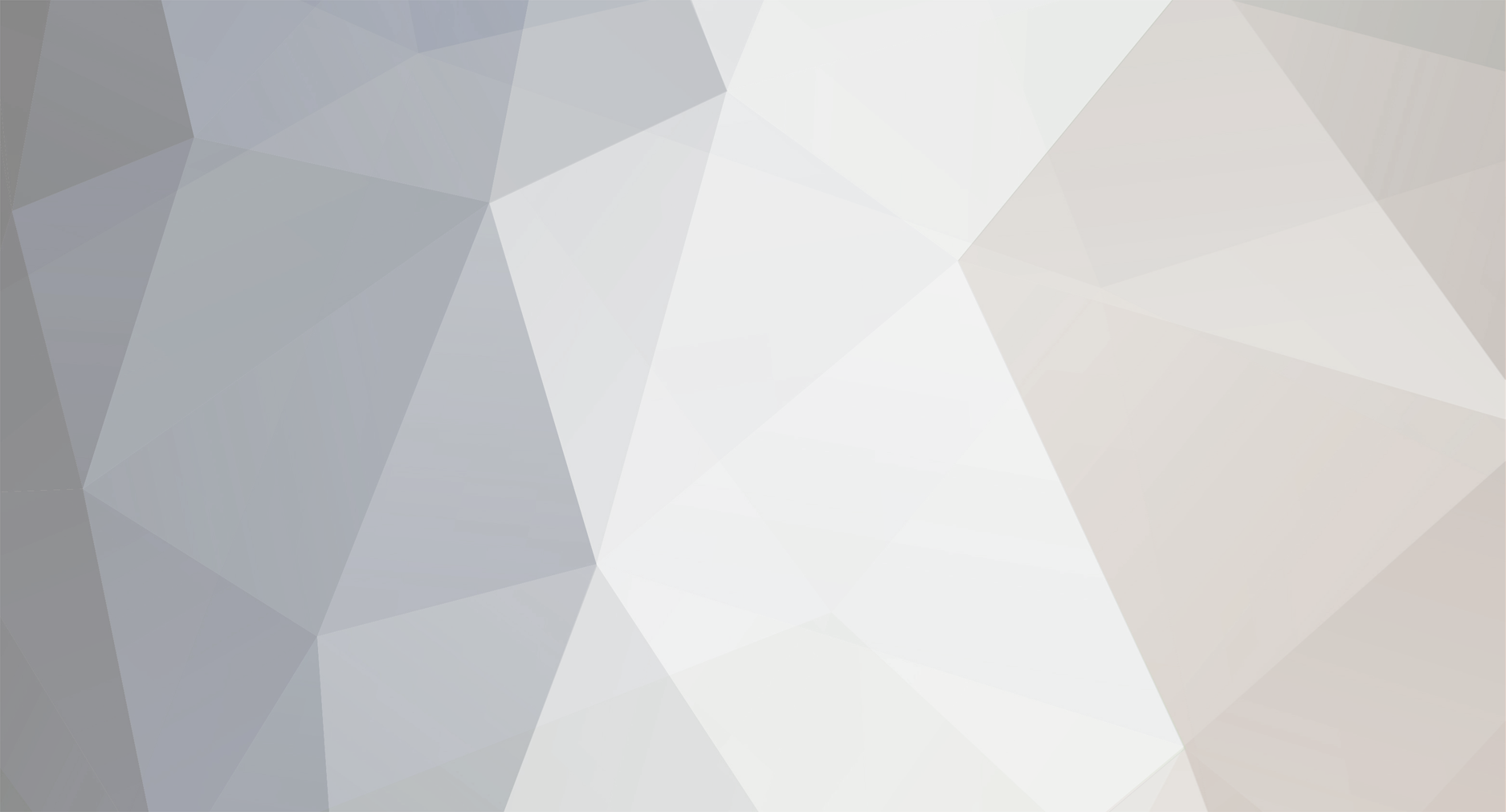
KevinM1
Moderators-
Posts
5,222 -
Joined
-
Last visited
-
Days Won
26
Everything posted by KevinM1
-
Ajax won't stop you from having to process things and validate on the back end. Remember: JavaScript can be turned off. For performance, if everything is written well, the initial page hit will be a bit bigger with Ajax than without, simply because you'll have more code coming through the pipe to be processed by the browser. Subsequent hits (like form submissions) will be better, though, because you'll only be handing that data and not having to re-render the rest of the page.
-
Exactly. Would it make more sense if it looked like: foreach ($_POST as $key => $value) { if (is_array($value)) { $temp = $value; } else { $temp = trim($value); } } ? Because that's what the ternary operator is actually doing: (condition) ? if true : if false So, in this case, you're looping through all of $_POST blindly, and can't tell from the outset if you're dealing with a single entry or a collection of them, which is what that ternary operator checks for.
-
A collection of submitted checkboxes could be an array.
-
This is a help forum. We help our members help themselves by answering specific coding questions, so they can see how the answer to that particular problem can be applied in a more global sense. We don't provide solutions to entire problems out of the gate unless they're trivial. Asking one of the team to either write a solution that you can check or rudimentary code that can be learned by visiting the online PHP manual is exactly NOT what we do here.
-
We're not going to write your code for you. That's not what we do. Now, please answer requinix's question.
-
Easiest way to think of it: Use GET to retrieve information Use POST to insert/update/delete information Under the hood they both operate similarly in that they send key/value pairs to the server. The differences being that GET's pairs are visible right in the browser's address bar whereas POST sends them behind the scenes, and you can generally send more information with POST. But, really, GET and POST are verbs. Use them as they would be used in English, and you'll be all set.
-
There's... a lot wrong with what you currently have, and it really highlights a lack of understanding about custom functions, arrays, and the difference between return and echo. $_SESSION is already an array. If you want an $errors array to be stored in $_SESSION, then you simply assign each error to the array, then assign that to $_SESSION, like so: session_start(); // IMPORTANT! You need this at the top of every file you want to access sessions in $errors = array(); // somewhere else in your code where you want to store an error $errors[] = "ERROR: You can't do what you tried to do!"; // note the []... that tells it to assign the value on the right to the // next open spot in the array on the left // even lower in your code $errors[] = "ERROR: You still can't do that!"; // assign it to $_SESSION $_SESSION['errors'] = $errors;So, on your display page, you can have: session_start(); function errors() { if (isset($_SESSION['errors'])) { // code defensively to prevent errors foreach ($_SESSION['errors'] as $error) { echo "$error<br />"; } } } // blah blah more code errors();
-
It's impossible to tell with seeing just one line of code. Are you executing it in a loop or something?
-
If you do feel like doing this yourself, get yourself some good resources: Intro: http://www.amazon.com/MySQL-Dynamic-Sites-Fourth-ebook/dp/B005GXM63U/ref=sr_sp-atf_title_1_1?s=books&ie=UTF8&qid=1374521996&sr=1-1&keywords=larry+ullman OOP: http://www.amazon.com/Objects-Patterns-Practice-Experts-ebook/dp/B004VJ2YZU/ref=sr_sp-atf_title_1_1?s=books&ie=UTF8&qid=1374522054&sr=1-1&keywords=zandstra http://www.amazon.com/Design-Patterns-Elements-Object-Oriented-ebook/dp/B000SEIBB8/ref=sr_sp-atf_title_1_1?s=books&ie=UTF8&qid=1374522078&sr=1-1&keywords=gof+design+patterns http://www.amazon.com/Enterprise-Application-Architecture-Addison-Wesley-ebook/dp/B008OHVDFM/ref=sr_sp-btf_title_1_6?s=books&ie=UTF8&qid=1374522111&sr=1-6&keywords=j2ee+design+patterns Basic security: http://www.amazon.com/Pro-PHP-Security-Implementation-ebook/dp/B004GXAGZG/ref=sr_sp-atf_title_1_1?s=books&ie=UTF8&qid=1374522172&sr=1-1&keywords=pro+php+security+2nd+edition HTML/CSS/JavaScript: https://developer.mozilla.org/en-US/ http://www.amazon.com/Pro-JavaScript-Techniques-ebook/dp/B0015R3OHI/ref=sr_sp-atf_title_1_2?ie=UTF8&qid=1374522246&sr=8-2&keywords=resig And stay far, far away from w3schools. This site will tell you why: http://www.w3fools.com/ So, like anything, you'll have to do cost analysis. Is it better to pay a professional to do the work, or is there more value in the time spent, and the resources purchased, to do it yourself? The resources above will make you about entry level competent. Maybe a little more than that with PHP itself.
-
You really, really need to use a database for this. That will allow you to easily create invoices by simply linking parts together, and then tying that collection to a user. Flat files are really not suited to what you want to do. Also, you should clarify what you mean by 'user'. If there's a difference between customers and the people working with/for you, then you'll need to be able to model that. Unfortunately, we don't do 1-on-1 mentoring here. Everyone here, including staff, are volunteers, so while we're willing to help with specific coding questions, it would take too much time for us to mentor without getting some kind of compensation in return. If you need that kind of help, feel free to post in our Freelancing section. Since this is custom work, and you don't have much/any experience with this kind of thing, you probably should hire someone. Web development is fairly easy to get started with but difficult to do well, and if you're relying on this system for your work you should have it done right the first time. That's probably not what you want to hear, but for something non-trivial, with enough complicated details to be annoying, it's really the best route to go.
-
Return and echo do two different things: Echo outputs directly to the browser. Return stops function execution and sends a value back to the function's invocation context. Example: function example($x) { return $x * $x; } $val = example(5); // $val now contains the value 25 (5 * 5) Since both echo and return do different things, using them correctly merely depends on what you're trying to do at that moment. Need to display data? Use echo. Need to use the value created after a function processes some data? Use return. That said, you'll likely return far more than echo. Functions are supposed to be black boxes - you pass data in, the function works on it, and ultimately gives back a new value (or set of values). Echoing generally is better used in your main script execution rather than functions.
-
Hi Dave, It's a bit difficult to steer you in the right direction because your problem is a bit vague. On the surface, it just looks like you want to pass the invoice # from page to page, which you can do either through sessions or by sending/populating a hidden form field, but I can't help but feel that that's only part of what you really want to do with your site/app. So, why don't you give us the larger view of what you want to do in its entirety, and how you see these forms fitting into it?
-
Right. I was just trying to make the weak joke that they have so many contributors because the code base sucks. ... yeah, I never said I was funny.
-
I can't help but wonder if all the Github forks mentioned in that announcement stem from people trying to fix the POS code under the hood. I don't view forks as a net good.
-
Yeah, that's what I'm thinking. They're trying to use your own ignorance against you in order to upsell.
-
I use ICDSoft, usually. And that ticket response is just another reason why I would never use GoDaddy.
-
Are you absolutely sure that's the case? Because having the ability to upload files above the web root has never been a problem with any shared hosting I've ever had.
-
...why are you asking us your interview question? Not to be a dick, but these are questions that you should actually investigate yourself before asking others. Have you tried running the code? Do you get any errors or warnings? Can you trace what it's actually doing with the database?
-
Yeah... we don't answer homework questions.
-
Use dependency injection and delegate: class Forms { private $bootstrap; public function __construct($bootstrap) { $this->bootstrap = $bootstrap; } public function input_text($name) { return $this->bootstrap->bootstrap_input_text($name); } }
-
Well, again, it depends on what you want to do. For example, with user comments, you'll likely want to either use strip_tags if you don't want HTML, or htmlentities if you do, but in a readable/non-executable format. You'll need to learn how to safely secure a user's credentials (protip: any resource that recommends MD5 as a hash algorithm should be avoided). Anything beyond that is, again, up to the particulars of your project.* *I'm not trying to be difficult, but we generally answer specific questions about code or application design. That allows us to give good answers without playing the inevitiable "I'm not doing that, but rather this" "Why didn't you say that before?" "I thought it was obvious/you didn't read it correctly" "Yes I did" "No you didn't" game. For *AMP security, there's a book titled Pro PHP Security by apress ( http://www.amazon.com/Pro-PHP-Security-Application-Implementation/dp/1430233184/ref=sr_1_2?ie=UTF8&qid=1372464328&sr=8-2&keywords=Pro+PHP+Security ) that's a pretty decent primer.
-
Security is a rather broad topic, and a lot of it depends on what a site's functionality is (or is supposed to be). So, a better way to get help would be to tell us what you're trying to build, and then we can address those particular concerns. That said, I will say that you should not use any of the old mysql_* functions. They're soft deprecated, and no longer represent best practice. Instead, use either MySQLi if you're certain your project will only use a MySQL database, or PDO as your database handler. Another general tip: since you're just starting out, if you use a resource or see a tutorial that has code like this: function blah() { global $x; // ... other code } Toss it out/ignore it/set it on fire. Use of the 'global' keyword is a bonafide symptom of doing things wrong, and any tutorial or resourse that uses it should be considered suspect.
-
What are you referring to when you mention 'code completion'? Intellisense? Actual code generation (say, dumb getters and setters)? I tend to like both, mainly because of my disabled hands. Less typing = good for me.
-
Like Christian said, we don't normally answer homework questions. So, it'll be up to you to determine if it's better. Is it?
-
If you're a freelancer, you're going to have to start small and grind out a portfolio. And, while you can do that with various experiments/demos, your best bet is to actually go around town, talk to people, and try to work on a real project. I guarantee you that there's at least one small, local business that would like some form of web presence in your area. Why bother with that? Your own projects, no matter how skillfully crafted, will be lacking in one area: human interaction. Nothing can replace the valuable experience of actually talking to and dealing with a client, figuring out where you draw the line on certain requests, etc. Freelancing in particular is a people-oriented business, as in most cases you'll need to convince your client that you're worth their investment. That's a challenge you simply can't replicate. If you can earn a client's trust, chances are they'll recommend your services to someone else. That's the kind of advertising you can't buy. And if you're good, it will snowball. After that, you can get a job wherever you want as you'll have both the portfolio, and perhaps even better, war stories re: clients, to prove your worth.