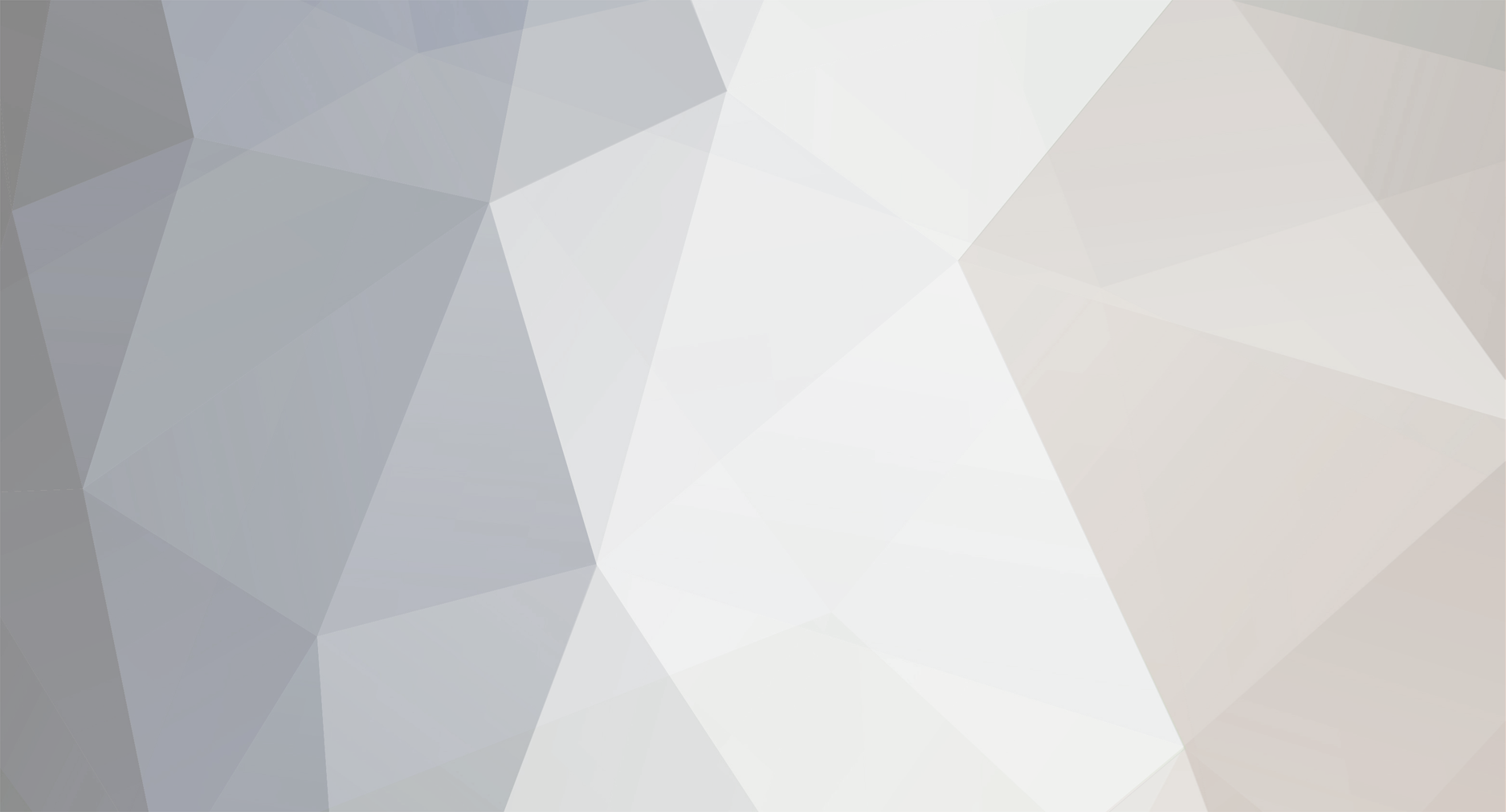
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
user selects checkbox wich columns to retrieve
Psycho replied to Klein_Kipje's topic in PHP Coding Help
Regarding the process of using the saved preferences of the user: I'm not knocking ginerjm's approach, but I would do it a little differently. I would not put logic into the query to determine which columns to fetch. If you make any mistake in properly validating the data you may create a potential hole in security. Instead I would have a single process to fetch the data that gets all the potential columns that users may want to see. Parameters I would put in such a process, if needed, would be things such as a sort order and a limit (if the user can define the page size). I would then modify the output when processing those record to determine which fields to include in the output. -
Are you storing the content and the associated footnotes as HTML? You should be storing the article content and the footnotes as separate data in the same records. Then handle the output format in the code. Then there is no need to "extract" data from formatted content. Rough exmaple <?php $query = "SELECT id, article, footnote FROM articles"; $result = $db->query($query); foreach($result as $row) { //Append article output $articles .= "<li>{$row['article']</li>\n"; //Append footnote output $footnotes .= "<blockquote> <a href=""#f1"" name=""fn1""> <span class=""superscript""> * </span></a> {$row['footnote']} </blockquote>\n"; } //Run query to get references and put into $references variable //Output the generated content ?> <div class="articles"> <?php echo $articles; ?> </div> <div class="references"> <?php echo $references; ?> </div> <div class="footnotes"> <?php echo $footnotes; ?> </div>
-
I always thought it was a Dreamweaver (which I don't use) thing that cause every single line of PHP code to have open/close tags. My preference is to first try and separate logic from presentation as much as possible. So, files tend to be mostly PHP or mostly HTML. In PHP heavy files, I will just typically put HTML code within quoted strings since the code highlighting doesn't provide a lot of value. Where I need PHP code in presentation files, I will use something similar to requinix's example above.
-
Cronix had a typo in the code he provided. Specifically there was this: }else if($browser_name = "chrome")){ echo "your browser is chrome"; That is trying to assign the value 'chrome' to the variable $browser_name (notice the single equal sign) instead of comparing them. Since the assignment always succeeds, the result is true and that condition is met. You could fix that with a double equal sign, but I think there is a better way to do the comparison that allows you to expand the list easily without writing more code. Just change the array at the top: //Create list of known browsers // -- Set key as the lowercase value found in the return value of get_browser // -- Set value to the name you would like to display $browserList = array( 'firefox'=>'Firefox', 'chromium'=>'Chromium', 'chrome'=>'Chrome', 'ie'=>'Internet Explorer' ); //returns array of browser attributes $browser = get_browser(null, true); //get the name of the browser from the $browser array, but force to lowercase for comparison $browser_name = strtolower($browser['browser']); //Check if returned browser name exists in the list of known browsers if(isset($browserList[$browser_name])) { $browserMsg = "Your browser is {$browserList[$browser_name]}"; } else { $browserMsg = "Your browser was not detected"; } echo $browserMsg;
-
You should never run queries in loops (unless absolutely necessary). A process such as SmileyBlackSheep proposes is better, but you have to be sure to use the proper functions to escape content to prevent SQL injection. Or, use prepared statements. Right now, you are open to SQL Injections. Using prepared statements in a loop isn't "as much" of a drain on system resources. You can do an "all at once" prepared statement, but creating the placeholders can be goofy. I'd also add that you need to add comments to your code. It will help you as well as others when you ask for help. Plus, format your code with indents to show structure (you may be doing this and the forum removed them). As to your problem, I have a question. I would assume each line in the file is respective to a specific entity. If you are just dumping the data separately to two tables there is no association. If two tables make sense, then I would expect that you would insert the data into one table, get the primary key, then use that key when inserting the data into the other table. Looks like the second table is for the details about a person and the first one if for an address associated with that person. If that is correct, the current process (I think) should insert the user first and, if it succeeds, then insert the address. But, I don't know your application, so I can't state that definitively. So, there are a lot of changes to be made. But, try this to see if it tells you why the records are not being inserted <?php include("core/db.php"); //Upload File if (isset($_POST['submit'])) { if (is_uploaded_file($_FILES['filename']['tmp_name'])) { echo "<h1>File {$_FILES['filename']['name']} uploaded successfully.</h1>"; echo "<h2>Displaying contents:</h2>"; echo "<pre>" . htmlentities(readfile($_FILES['filename']['tmp_name'])) . "</pre>"; } //Import uploaded file to Database $handle = fopen($_FILES['filename']['tmp_name'], "r"); while (($data = fgetcsv($handle, 1000, ",")) !== FALSE) { $query = "INSERT INTO contacts (firstname, lastname, gender, title) VALUES ('$data[4]','$data[5]','$data[6]','$data[7]')" $result = mysqli_query($con, $query); if(!$result) { //For debugging only echo "Query: {$query}<br>Error: " . mysqli_error($con); //Skip the rest of this record continue; } $query = "INSERT INTO site (name, street, city, postcode) VALUES ('$data[0]','$data[1]','$data[2]','$data[3]')" $result = mysqli_query($con, $query); if(!$result) { //For debugging only echo "Query: {$query}<br>Error: " . mysqli_error($con); } } fclose($handle); print "Import done"; } else { //view upload form print "Upload new csv by browsing to file and clicking on Upload<br />\n"; print "<form enctype='multipart/form-data' action='' method='post'>"; print "File name to import:<br />\n"; print "<input size='50' type='file' name='filename'><br />\n"; print "<input type='submit' name='submit' value='Upload'></form>"; } ?>
-
Sounds like a homework problem. Why don't you at least make an attempt and then ask for questions on where you are stumped. Or, at least provide an explanation of how you think it might be accomplished. Most people here don't mind "helping" people with homework, but we like to see the person at least try. I'll give you a little push in the right direction: 1) You show that there should be three primary queries from which you want 1 record each. You say you know how to create queries, but do you know how to, correctly, get 1 random record? Hint: don't use ORDER BY RAND() 2. You then need a secondary query to get the remaining record(s). This will be very similar to the first three queries - except you don't want to filter on the category. But, you do not want to include the records you got from the first three queries. So, start with #1. If you get it working move on to #2. If not, post back with what you've done and the problems you are experiencing.
-
Checking for unique email addresses is not of much value either. With a single gmail account you can use an number of aliases. For example, if your email address is username [@gmail.com] you can also use username+1, username+2, usernaem+abc, etc. etc. I think it allows up to 5 or 6 characters after the username. And they will all be "working" email addresses that go to the same mailbox. And, as Jacques1 stated, verifying mail addresses is very problematic as well. I once added Apt. #'s to my house address to register for a service multiple times. The "best" option is to use professional services that do merge/purge/dedupe operations for mailing addresses. Although, anything that is good will cost money. Here's one resource: http://www.qas.com/address-verification.htm
-
Help needed: SELECT specific user data from MySQL in PHP
Psycho replied to raneyron's topic in MySQL Help
Correction to my previous comment. I mistakenly stated you were using mysql_ functions (as apposed to mysqli_) functions. So, you are good there. A while() loop is the typical way to iterate over the records returned from a result set when the query can potentially return multiple records. The scenario you have should only ever return 0 or 1 records. Therefore, a loop of any kind is unnecessary. Here is a slight rewrite of what you had that includes how I would fetch the results. error_reporting(E_ALL); ini_set('display_errors', 1); //Fetch User ID from session $userID = $_SESSION['user']['username']; echo "Session User ID: " . htmlentities($userID) "<br/>\n"; //Create and run query $query = "SELECT username, startDate, mealsDay, caloriesMeal, costMeal FROM diet_info WHERE username = :userID "; $statement = $db->prepare($query); $statement->bindValue(':userID', $userID); $result = $statement->execute(); //Check results if(!$result) { //Query failed echo "Query failed"; //Add debugging code } elseif(!$statement->rowCount()) { //No results returned echo "No user found for user " . htmlentities($userID); //Add debugging code } else { //A record was returned, display results $user = $statement->fetch_assoc(); echo "Start Date: {$row['startDate']}<br/>\n"; echo "Meals per Day: {$row['mealsDay']}<br/>\n"; echo "Calories per Meal: {$row['caloriesMeal']}<br/>\n"; echo "Cost per Meal: {$row['costMeal']}<br/>\n"; } $statement->closeCursor(); -
Help needed: SELECT specific user data from MySQL in PHP
Psycho replied to raneyron's topic in MySQL Help
And what are your results. What is or is not happening? I see some issues in your code, but I don't see any specific errors. Here are some things to address: 1. Don't use the mysql_ functions. They are deprecated. Use mysqli_ or, better yet, PDO. 2. Don't put user data directly into a query. Use prepared statements to protect against SQL Injection. Session values are typically safe, but it's best to be over cautious. 3. After you run the query, you don't check for errors or to even check if there were results 4. After you run the query you have a loop to get the records. You should only get one record in this scenario. You just need to see if a record was returned and get it. No need for a loop. Even so, if you have a scenario where there are multiple records, don't get the count and do a foreach loop. Just use a while loop while($row = $result->fetch_assoc() { //Do something with $row } -
Trying to program my way out of repetitive form POST capture/MySQL insert!
Psycho replied to luke3's topic in PHP Coding Help
I think your process is flawed. I too have tried to build methods to do such things automatically for any scenario. It seems like you are making things easier, but ultimately you end up painting yourself into a corner. You then end up adding more complexity to account for functionality that you need for one instance that you don't need for others. In the end, you're left with a huge mass of code that becomes difficult to maintain and can be buggy. Plus, how would you handle situations when you need to multiple records with associations? E.g. creating a client with multiple phone numbers? You should store the basic client data in one table (get the ID of that record) and then store the phone numbers in the associative table. For something such as forms which, typically, are used for different entities I think it is always best to build the logic for each one to be specific to the needs for each entity. That doesn't mean you can't build common functionality to handle certain pieces of the functionality, which is re-purposed in each form handling logic. You absolutely should do that. For example, you might build different validation functions for certain types of data (e.g. Phone, email, etc.). For example, your function assumes that the first field in the tables will always be the ID and that the last three will always be default fields. Plus, you need to match up the POST values to the DB fields. You could ensure the form fields are in the same order as the DB fields, which is a huge pain. Just changing a form to put things in a different order would require you to make an alter in the DB. Or, you could name the input fields exactly the same as the DB fields. It is a bad practice to purposefully expose any information about your DB schema. -
Yes, those were. But, you didn't mention those variables in your question. You asked You dumped a lot of code and asked why (what looks to be a class method) is not returning a value after the user is redirected via a header() function. I was not going to take 20-30 minutes to read through your code and try to decipher every line to figure out what values are specifically set where. Based on your question, my response was valid. I have no clue. I did take some time to try and follow the process flow of your code and gave up. It's too convoluted for me to digest without investing more time than I am willing. You're using "global" within a class?
-
When you do a header() redirect you will lose any variables that were set during the current page execution. It is the same as if the user had entered that url directly into their browser - the requested page has no knowledge of anything that was done on the previous page. If you need to set values that will be available on subsequent page loads you should use COOKIE or SESSION values.
-
In my opinion, you should not hide/show the menu by requiring the user to click that icon. I looked at your page and almost didn't even notice the "click for menu" prompt. I would really suggest rethinking whether the menu should be hidden at all. It doesn't take up too much vertical space. And, if that is an issue, you could see how it looks to the right of the logo. Regarding the use of the large images and mouseover images for the external pages I think it is a good, clean look. But, I would consider two changes. 1) When I mouseover an image it isn't always noticeable that the text has popped-up above because thinks like that usually appear below the action item and because the general background color is white and the background of the mouseover content is white also. I would try putting the mouseover content below the image and give it a slight tint for a background color. I'd try a 10%-20% tint of the color used for the menu icons. And, possibly an outline around the box.
-
Also, eregi() should not be used - it is deprecated. It can still work, but based on the error level set for the server it may be stopping execution due to a warning about the function being deprecated. But, since you provided no details about any errors/warnings . . .
- 8 replies
-
- php
- contact form
-
(and 1 more)
Tagged with:
-
A few things: 1. Don't use SELECT * unless you really need everything from those tables. Requesting all the columns takes more resources to run the query and will not scale well. 2. Don't use a LEFT/RIGHT JOIN unless it is necessary. Again, it uses more resources than needed. I would assume that every stream has an association with a user - if so, no LEFT JOIN would be needed to the users table. 3. Are you really using the username as the primary and foreign key? Normally an INT type field would be used and be indexed for performance.
-
foreach($xml->gms->g as $games) { //Skip if either team name is empty if(empty($games['htn']) || empty($games['vtn'])) { continue; } //If both scores are 0, don't show them (game hasn't been played) if($games['hs']==0 && $games['hs']==0) { $games['hs'] = ''; $games['vs'] = ''; } echo "<div class='button blue'>"; echo "{$games['htn']} {$games['hs']} VS {$games['vtn']} {$games['vs']} on {$games['d']} at {$games['t']}"; echo "</div>"; }
-
Not sure which function you plan on using this with, but the RegEx for what you want would look like this: "(\d+)=\\1"
-
So, you hash the password during registration then on login you compare the unhashed value the user submits to the hashed value in the DB? See the problem?
-
Um, no. The figure was $600MM. But, it is now at $840MM. (http://www.cbsnews.com/news/healthcare-gov-has-already-cost-840-million-report/) It is things like this that really piss me off as a tax payer. The $6MM figure you quoted would still have been more than adequate to build what they ended up with.
-
Why would you want to scrape their sites as opposed to using one of the APIs they provide? If they make any changes to the format of their pages your code will break as opposed to using one of their provided APIs which they have a vested interest in maintaining. Here are a couple links to get you started. http://go.developer.ebay.com/developers/ebay/php-perl-and-python-developer-center http://aws.amazon.com/sdk-for-php/
-
For non logged in users, you would not want to use IP addresses since you can have multiple people behind the same IP address. You would want to use unique sessions. You could then use that functionality for both logged in users and guests. When user requests a page save session ID, User ID (if logged in user) and a timestamp. Then, as Frank_b stated before, you would query the unique users that were active in the last X minutes and do a JOIN out to the user table to get the names for the logged in users.
-
Um, yeah. I guess I need to get a refund on my speed reading class. So, it probably is a type on the table name in one of those instances.
-
I was thinking they were supposed to be the same table as well. But, if that was the case, there would be no need for either of the IN conditions since the first one would be returning ALL of the possible post numbers. Therefore, the second IN condition would be unnecessary since those numbers (if they existed at all) would be returned from the first IN condition. But, even that one would not be needed since it would be selecting all of then anyway.
-
Off topic: Creating a variable that is only used once (unless it is based on a complicated calculation) is a waste. It only adds complexity where none needs to exist. In your if() condition you have two variables that were created just before the condition check. For example, you define $_Email as the same value as the session value. Then define $EmailLen as the length of that variable. That's two lines of code that are unnecessary. Also, stripslashes() is unnecessary unless you are on a server running a very old version of PHP. Plus, I'd suggest not creating variables before you need them. It makes it difficult when you revisit the code to understand why the variable is being created. Lastly, do not use the mysql_ functions, they are deprecated. Your current code is open to SQL injection. You should use prepared statements using mysqlI_ or, better yet, PDO. Rough example: <?php // Library SMS3S Version 1.0.0 15-03-2014 Desmond O'Toole. include ("../secure/SecureFunctions.php"); session_start(); Session_Init(); $emailPost = trim($_POST['Email']); $_SESSION['Error_1'] = ""; if(strlen($emailPost) && !validEmail($emailPost)) { $_SESSION['Error_1'] = "Invalid Email Address"; $_SESSION["Email"] = $emailPost; header('Location: EditUserDetails.php?ID={$_SESSION['ID']}'); exit(); } connectDB(CURRENT_DB); $User = crossref($_SESSION['ID']); $sqlUpdateUser = "UPDATE LIBusersX SET Email = '$emailPost' WHERE User = '$User'"; //$query = mysql_query ($sqlUpdateUser); header('Location: edituser.php'); ?>