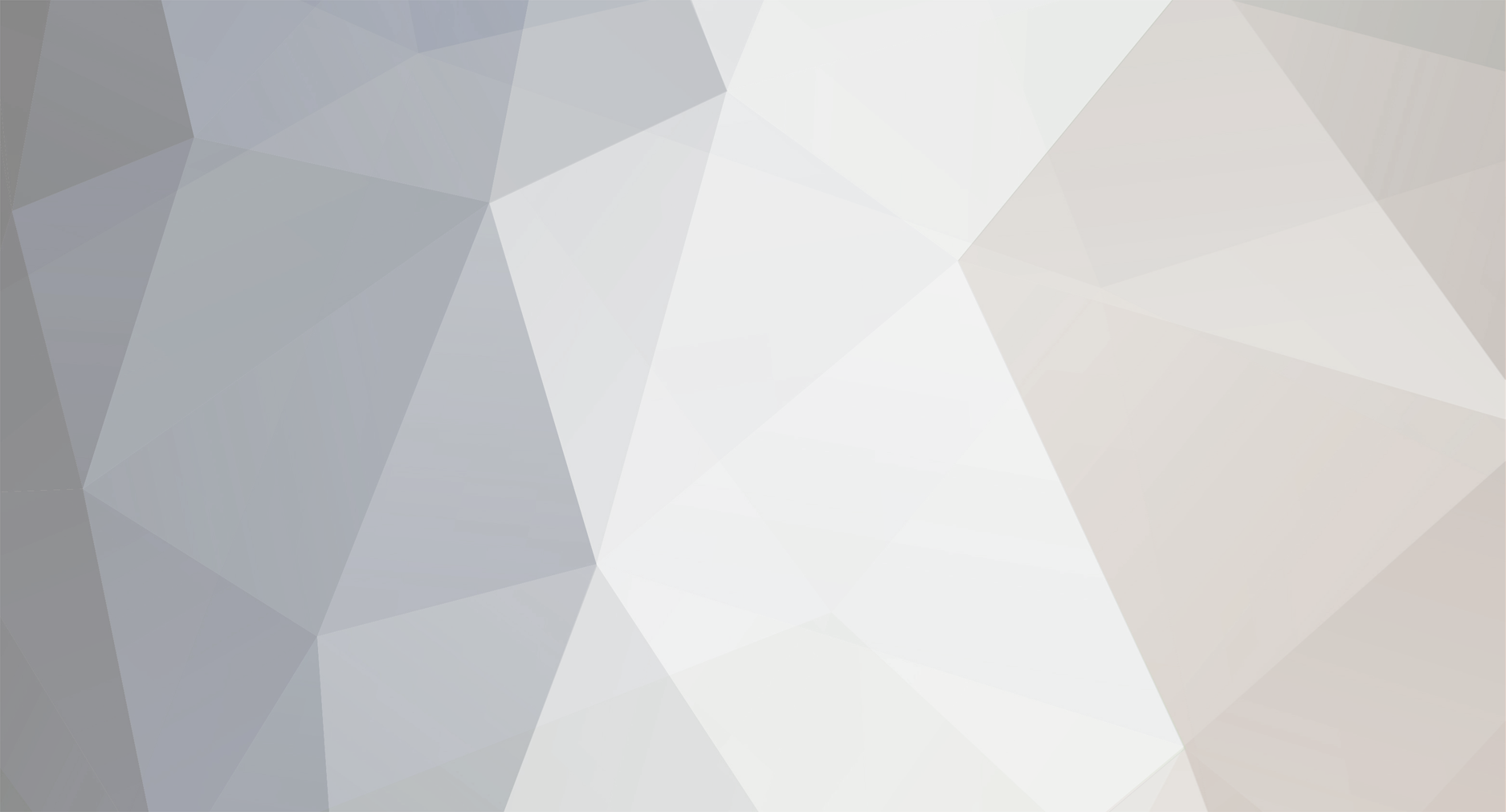
jcbones
Staff Alumni-
Posts
2,653 -
Joined
-
Last visited
-
Days Won
8
Everything posted by jcbones
-
Need a Link or Basic Script to Learn Select Functionality
jcbones replied to meOmy's topic in PHP Coding Help
Why? Data should only be stored once, in one place. Database Normalization is a must for consistent, trustworthy data. On top of that, I'm not sure this is something you would use a database for, maybe sessions is where you should be looking. Sessions should only be a backup if they don't have javascript enabled. This should really be handled via ajax.- 1 reply
-
- select
- dropdownmenu
-
(and 1 more)
Tagged with:
-
Mac_Gyver, and Maxxd got it. Both $product and $price are strings. So you need to use $products. This is set in the while loop, if you look carefully. I would also echo Mac_Gyver's post above. It would be easier to just dynamically build the page than have to change 40+ products per page.
-
So you are getting the authurID from the first query and using it in the second query? OR, are you using the variable $blogAuthur to populate the second query? I will go on the assumption that it is the former. "SELECT b.blogID, a.authurName FROM blogs AS b JOIN blog_authurs AS a ON b.authurID = a.authurID WHERE blogStatus = 1 "
-
Mentalist is right, you got a little confused about your structure and what is happening. I added a little clarity in this for you. //Loop through added fields foreach ( $_POST['fields'] as $key=>$value ) { //for clarity: $url = $value; $web_link = $_POST['code'][$key]; //Insert into websites table $sql_website = sprintf("INSERT INTO websites (Website_URL, web_Link) VALUES ('%s', '%s')", mysql_real_escape_string($url), mysql_real_escape_string($web_link) ); $result_website = $db->query($sql_website); $inserted_website_id = $db->last_insert_id(); //Insert into users_websites_link table $sql_users_website = sprintf("INSERT INTO users_websites_link (UserID, WebsiteID) VALUES ('%s','%s')", mysql_real_escape_string($inserted_user_id), mysql_real_escape_string($inserted_website_id) ); $result_users_website = $db->query($sql_users_website); }
-
You should also be looking for: $v['player']; $v['total']; according to your query.
-
You should be able to pull the name without a sub-query. "select player, sum(Points) as total from player_points where date_entered > '$d' Group by player order by total DESC"
-
echo $rData->id . '<br/>';
-
$r = mysql_query("select sum(points) as total from player_points where date_entered > '$d' Group by player order by total DESC") or trigger_error(mysql_error()); *Note*, You shouldn't be using mysql, it is ver` old, and a `neer do well. Stick with PDO, or Mysqli.
-
Pull all of your relevant data from the database in one query. I suppose you are using an auto_incremented ID, or some other UNIQUE key. You can store all the data in an array, and then echo out that array as the base price. If you cannot re-build the whole page to make it dynamic, this is my suggestion. Example <?php $link = mysqli_connect("localhost", "my_user", "my_password", "world"); /* check connection */ if (mysqli_connect_errno()) { printf("Connect failed: %s\n", mysqli_connect_error()); exit(); } $type = "mixers"; /* create a prepared statement */ if ($stmt = mysqli_prepare($link, "SELECT Product_Code, Base_Price FROM Product WHERE Type=?")) { /* bind parameters for markers */ mysqli_stmt_bind_param($stmt, "s", $type); /* execute query */ mysqli_stmt_execute($stmt); /* bind result variables */ mysqli_stmt_bind_result($stmt, $product, $price); /* fetch value */ while(mysqli_stmt_fetch($stmt)) { $products[$product] = $price; } /* close statement */ mysqli_stmt_close($stmt); } /* close connection */ mysqli_close($link); ?> After this, anywhere you need the price you can echo out the array. <h2 align="center" class="topper"><a href="dynamic_mixer_smx800e.html">SMX 800E <br /> Mixer</a></h2> <ul> <li>Length 27" Tube</li> <li>800 Watts </li> <li><?php echo $product['smx800e']; ?></li> </ul>
-
At this point, you need to add some de-bugging to your queries to make sure they are processing correctly. like: $q2 = mysql_query(...) or trigger_error(mysql_error()); You should really port over to mysqli at the least. mysql is very old.
-
Your mysql query is failing, thereby returning false instead of a resource ID. This is likely because of a scope issue. When you have a function, any variables inside of the function must be created in the function itself, OR be passed in the arguments (I purposely steered clear of explaining globals here). Going further with the explanation, I can tell you that $dbTable, and $db are unknown variables in your function checkban(). You will either have to hard code them, or pass them in the arguments. Since you hard coded the column names, it wouldn't be to hard to hard code the table name. Then either create a db connection inside of the function (useless, unless that is the only db call), or pass it in. My suggestion would be: function checkban($mxitid,$db) {
-
Here is your script cleaned up a might, and taking into account all of the above suggestions. UN-TESTED <?php error_reporting(E_ALL); ini_set('display_errors', '1'); // some error checking /* if($_POST['reg']){ echo "form submitted"; }else{ echo "form not submitted"; } */ /////////////////////////////FAILURE TO TRIM VALUES MAY RESULT IN UN-DESIRED EXECUTION///////////////////////////// $errors = array(); //if a post has been sent to the page: if($_SERVER['REQUEST_METHOD'] == 'POST') { // echo $_POST['user']." - ".$_POST['pass']." - ".$_POST['email']; //check if either user or pass is empty: if((!empty($_POST['user']) && !empty($_POST['pass'])) { if(strlen( $_POST['user'] ) < 5 ) { //now we check for length $errors[] = "Username Must Be 5 or More Characters."; } if( strlen( $_POST['pass'] ) < 5 ) { //now we check for length $errors[] = "Password Must Be 5 or More Characters."; } if($_POST['pass'] == $_POST['user'] ) { //see if they match $errors[] = "Username And Password Can Not Be The Same."; } //now make sure pass_again isn't empty, and that the two match. if(empty($_POST['pass_again']) || $_POST['pass'] !== $_POST['pass_again'] ) { $errors[] = "Passwords must match"; } } else { //if the user or pass is empty, we catch it here, bypassing the now needless checks. $error[] = 'You must provide both a username, and a password.'; } // CREATE BETTER EMAIL CHECK //checking to make sure both emails are populated. if(!empty($_POST['email_again']) && !empty($_POST['email'])) { if(!preg_match('~^.+@[^\.].*\.[a-z]{2,}$~',$_POST['email'])) { //check to make sure it is somewhat valid expression. $errors[] = "Email must be valid."; } if($_POST['email_again'] == $_POST['email'] ) { //and that they are both the same. $errors[] = "Emails must match."; } } else { //we catch them here, if either is empty. $errors[] = 'You must provide an email address.'; } //From here on, we are just checking empty values. if(empty($_POST['address_1'])) { $errors[] = "Address cannot be empty"; } if(empty($_POST['address_2']) { $errors[] = "Address cannot be empty"; } if(empty($_POST['town']) { $errors[] = "Town cannot be empty"; } if(empty($_POST['county']) { $errors[] = "County cannot be empty"; } if(empty($_POST['postcode']) { $errors[] = "Postcode cannot be empty"; } if(empty($_POST['business']) { $errors[] = "Business cannot be empty"; } if(empty($_POST['vat_registered']) { $errors[] = "VAT Registered cannot be empty"; } if(empty($_POST['vat_number']) { $errors[] = "VAT number cannot be empty, please enter N/A if not VAT registered."; } //if errors is empty, then we are good to go on, otherwise we skip all the way to the bottom. if(empty($errors)) { require( 'database.php' ); //echo "$sqlCheckForDuplicate<br/>"; $username = mysqli_real_escape_string($con, $_POST['user']); $sqlCheckForDuplicate = "SELECT username FROM user WHERE username = '". $username ."'"; $result = mysqli_query($con, $sqlCheckForDuplicate); if(mysqli_num_rows($result) > 0) { //if there is a duplicate, we log it into errors, which is empty. $errors[] = "The Username You Have Chosen Is Already Being Used By Another User. Please Try Another One."; //echo " $username;" //this is for error checking } else { //We only add data to the database when we are sure that everything is error free. //Remove md5() function if not using encryption i.e. $password = $_POST['pass']; //note(***You should encrypt better than md5(), use the crypt() function, or password_hash(). $password = mysqli_real_escape_string($con, md5( $_POST['pass'])); $password_again = mysqli_real_escape_string($con, md5( $_POST['pass_again'])); $firstname = mysqli_real_escape_string($con, $_POST['firstname']); $lastname = mysqli_real_escape_string($con, $_POST['lastname']); $email = mysqli_real_escape_string($con, $_POST['email'] ); $email_again = mysqli_real_escape_string($con, $_POST['email_again']); $address_1 = mysqli_real_escape_string($con, $_POST['address_1']); $address_2 = mysqli_real_escape_string($con, $_POST['address_2']); $town = mysqli_real_escape_string($con, $_POST['town']); $county = mysqli_real_escape_string($con, $_POST['county']); $postcode = mysqli_real_escape_string($con, $_POST['postcode']); $business = mysqli_real_escape_string($con, $_POST['business']); $vat_registered = mysqli_real_escape_string($con, $_POST['vat_registered']); $vat_number = mysqli_real_escape_string($con, $_POST['vat_number']); //echo "No Duplicates<br/>"; $sqlRegUser = "INSERT INTO user( username, password, password_again, firstname, lastname, email, email_again, address_1, address_2, town, county, postcode, business, vat_registered, vat_number ) VALUES ( '". $username ."', '". $password ."', '". $password_again ."', '". $firstname ."', '". $lastname ."', '". $email ."', '". $email_again ."', '". $address_1 ."', '". $address_2 ."', '". $town ."', '". $county ."', '". $postcode ."', '". $business ."', '". $vat_registered ."', '". $vat_number."' )"; //echo "$sqlRegUser<br/>"; if( !mysqli_query($con, $sqlRegUser ) ) { //if for some reason the query fails after all of this, we catch it at the bottom. $errors[] = "You Could Not Register Because Of An Unexpected Error."; } else { /* Note: When using the header function, you cannot send output to the browser * before the header function is called. IF you want to echo a message to the * user before going back to your login page then you should use the HTML * Meta Refresh tag. */ //echo "You Are Registered And Can Now Login"; //echo " $username"; //this is for error checking header ('location: login.php'); //if query succeeds, then we re-direct: (NOTE, this should be a fully qualified URI). //always include an exit() function after a header(), this will end script execution: exit(); // if using echo then use meta refresh /* *?> *<meta http-equiv="refresh" content="2;url= login.php/"> *<? */ //result is always freed on exit of script, this line will never run. mysqli_free_result($result); } } //if you have gotten this far, there are only two cases: //1. you have errors: //2. registration query failed. include('includes/overall/header.php'); foreach($errors as $value) { //print all errors to page: echo $value . "<br />\n"; } include('includes/overall/footer.php'); } ?>
-
the foreach in the loop returns bool(false) though array is present
jcbones replied to littleangel's topic in PHP Coding Help
Not enough info. Post the array you are working with. There are a couple of custom functions in the code as well, we have no idea what those contain. It could all lead back to your error. -
You can get everything in one query, and then use the your: if ($row[next($row['company_id'] != $lastCompany)]) { Try using a LEFT JOIN, that way if there are no branches, then the company will still show up. You will need to check and see if there is a branch listed, but that should be easy using a: if(!empty($row['branch_id'])) { ... }
-
1. Switch to PDO 2. You should be escaping values on the input and not the output.
-
405 -> 421 break; var_dump($this->hasErr); //$hasErr is a class property, not a function variable.
-
Help with uploaded images not deleting from folder
jcbones replied to man5's topic in PHP Coding Help
Now, you wouldn't learn anything if we did it all for you. If you would read carefully what Ch0cu3r wrote, you would know why and where the issue is. He even gave you a suggestion on fixing it. The $_FILES array ONLY exists if a file is sent via a form. Since you are deleting the info, then you need to get the file path from the database BEFORE you delete the database entry. Then pass that file path to the unlink() function. -
The relevant code is the file that you are loading in your browser. Without seeing what is causing the problem, we can only guess, and be wrong.
-
Short example: <?php if(!empty($_GET['fontColor'])) { //if the form passed a color (form set to GET). setcookie('fontColor',$_GET['fontColor']); //set the cookie, of course you need to set the expiration. $_COOKIE['fontColor'] = $_GET['fontColor']; //also set the color in the cookie super global array. } //An array that holds all CSS3 colors. $colors = array("*TITLE01*" => "Reds", "Indian Red" => "#CD5C5C", "Light Coral" => "#F08080", "Salmon" => "#FA8072", "Dark Salmon" => "#E9967A", "Light Salmon" => "#FFA07A", "Crimson" => "#DC143C", "Red" => "#FF0000", "Fire Brick" => "#B22222", "Dark Red" => "#8B0000", "*TITLE02*" => "Pinks", "Pink" => "#FFC0CB", "Light Pink" => "#FFB6C1", "Hot Pink" => "#FF69B4", "Deep Pink" => "#FF1493", "Medium Violet Red" => "#C71585", "Pale Violet Red" => "#DB7093", "*TITLE03*" => "Oranges", "Light Salmon" => "#FFA07A", "Coral" => "#FF7F50", "Tomato" => "#FF6347", "Orange Red" => "#FF4500", "Dark Orange" => "#FF8C00", "Orange" => "#FFA500", "*TITLE04*" => "Yellows", "Gold" => "#FFD700", "Yellow" => "#FFFF00", "Light Yellow" => "#FFFFE0", "Lemon Chiffon" => "#FFFACD", "Light Goldenrod Yellow" => "#FAFAD2", "Papaya Whip" => "#FFEFD5", "Moccasin" => "#FFE4B5", "Peach Puff" => "#FFDAB9", "Pale Goldenrod" => "#EEE8AA", "Khaki" => "#F0E68C", "Dark Khaki" => "#BDB76B", "*TITLE05*" => "Purples", "Lavender" => "#E6E6FA", "Thistle" => "#D8BFD8", "Plum" => "#DDA0DD", "Violet" => "#EE82EE", "Orchid" => "#DA70D6", "Fuchsia" => "#FF00FF", "Magenta" => "#FF00FF", "Medium Orchid" => "#BA55D3", "Medium Purple" => "#9370DB", "Blue Violet" => "#8A2BE2", "Dark Violet" => "#9400D3", "Dark Orchid" => "#9932CC", "Dark Magenta" => "#8B008B", "Purple" => "#800080", "Indigo" => "#4B0082", "Slate Blue" => "#6A5ACD", "Dark Slate Blue" => "#483D8B", "*TITLE06*" => "Greens", "Green Yellow" => "#ADFF2F", "Chartreuse" => "#7FFF00", "Lawn Green" => "#7CFC00", "Lime" => "#00FF00", "Lime Green" => "#32CD32", "Pale Green" => "#98FB98", "Light Green" => "#90EE90", "Medium Spring Green" => "#00FA9A", "Spring Green" => "#00FF7F", "Medium Sea Green" => "#3CB371", "Sea Green" => "#2E8B57", "Forest Green" => "#228B22", "Green" => "#008000", "Dark Green" => "#006400", "Yellow Green" => "#9ACD32", "Olive Drab" => "#6B8E23", "Olive" => "#808000", "Dark Olive Green" => "#556B2F", "Medium Aquamarine" => "#66CDAA", "Dark Sea Green" => "#8FBC8F", "Light Sea Green" => "#20B2AA", "Dark Cyan" => "#008B8B", "Teal" => "#008080", "*TITLE07*" => "Blues", "Aqua" => "#00FFFF", "Cyan" => "#00FFFF", "Light Cyan" => "#E0FFFF", "Pale Turquoise" => "#AFEEEE", "Aquamarine" => "#7FFFD4", "Turquoise" => "#40E0D0", "Medium Turquoise" => "#48D1CC", "Dark Turquoise" => "#00CED1", "Cadet Blue" => "#5F9EA0", "Steel Blue" => "#4682B4", "Light Steel Blue" => "#B0C4DE", "Powder Blue" => "#B0E0E6", "Light Blue" => "#ADD8E6", "Sky Blue" => "#87CEEB", "Light Sky Blue" => "#87CEFA", "Deep Sky Blue" => "#00BFFF", "Dodger Blue" => "#1E90FF", "Cornflower Blue" => "#6495ED", "Medium Slate Blue" => "#7B68EE", "Royal Blue" => "#4169E1", "Blue" => "#0000FF", "Medium Blue" => "#0000CD", "Dark Blue" => "#00008B", "Navy" => "#000080", "Midnight Blue" => "#191970", "*TITLE08*" => "Browns", "Cornsilk" => "#FFF8DC", "Blanched Almond" => "#FFEBCD", "Bisque" => "#FFE4C4", "Navajo White" => "#FFDEAD", "Wheat" => "#F5DEB3", "Burly Wood" => "#DEB887", "Tan" => "#D2B48C", "Rosy Brown" => "#BC8F8F", "Sandy Brown" => "#F4A460", "Goldenrod" => "#DAA520", "Dark Goldenrod" => "#B8860B", "Peru" => "#CD853F", "Chocolate" => "#D2691E", "Saddle Brown" => "#8B4513", "Sienna" => "#A0522D", "Brown" => "#A52A2A", "Maroon" => "#800000", "*TITLE09*" => "Whites", "White" => "#FFFFFF", "Snow" => "#FFFAFA", "Honeydew" => "#F0FFF0", "Mint Cream" => "#F5FFFA", "Azure" => "#F0FFFF", "Alice Blue" => "#F0F8FF", "Ghost White" => "#F8F8FF", "White Smoke" => "#F5F5F5", "Seashell" => "#FFF5EE", "Beige" => "#F5F5DC", "Old Lace" => "#FDF5E6", "Floral White" => "#FFFAF0", "Ivory" => "#FFFFF0", "Antique White" => "#FAEBD7", "Linen" => "#FAF0E6", "Lavender Blush" => "#FFF0F5", "Misty Rose" => "#FFE4E1", "*TITLE10*" => "Greys", "Gainsboro" => "#DCDCDC", "Light Grey" => "#D3D3D3", "Silver" => "#C0C0C0", "Dark Gray" => "#A9A9A9", "Gray" => "#808080", "Dim Gray" => "#696969", "Light Slate Gray" => "#778899", "Slate Gray" => "#708090", "Dark Slate Gray" => "#2F4F4F", "Black" => "#000000" ); //end of processing, start output. ?> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en" lang="en"> <head> <?php if(isset($_COOKIE['fontColor'])) { //only if there is a fontColor set: //then set a css for the font color; echo '<style type="text/css"> body { color:' . $_COOKIE['fontColor'] .'; } </style>'; } ?> </head> <body> <?php // print out the form, with the select. echo '<form><label for="fontColor">Font Color</label> <select name="fontColor">'; foreach($colors as $name => $code) { //for each color if(strpos($name,'*') !== false) { //if the index contains an *, then: $name = $code; //change the index to the value; $code = '#FFFFFF'; //set the value to #FFFFFF } echo "<option style='color:{$code};' value='{$code}'>{$name}</option>"; //echo out the options (with styling). } //end the select, and the form, adding a submit button. echo '</select> <input type="submit" value="Submit" /></form> <div>THIS IS TESTING TEXT!!!</div>'; ?> </body> </html>
-
Crap! I've been asked to change my PHP ! Its been working for years!
jcbones replied to JTapp's topic in PHP Coding Help
I have no idea what API you are trying to access. I'm sure if you posted all applicable information we could get you sorted real quick. A list of things that is needed. 1. API name, or link, or list of methods. 2. Exactly what you wish to accomplish. Right now, the only thing I can give you is cURL being the proper way of doing this. <?php //data to send to API $data = "var1=what&var2=ever"; // create curl resource $ch = curl_init(); // set url curl_setopt($ch, CURLOPT_URL, "example.com"); //return the transfer as a string curl_setopt_array($ch,array( CURLOPT_RETURNTRANSFER => 1, CURLOPT_POST => 1, CURLOPT_POSTFIELDS => $data )); // $output contains the output string $output = curl_exec($ch); // close curl resource to free up system resources curl_close($ch); //Now we can use the $output in our application however we wish. ?> -
PHP contact form not working for an app I'm writing.
jcbones replied to TimKukulka's topic in PHP Coding Help
Post the errors. If you put your code in [ code ] ... [ / code ] tags, it will preserve formatting and be easier to read. -
PHP contact form not working for an app I'm writing.
jcbones replied to TimKukulka's topic in PHP Coding Help
I don't see anything that looks out of place. I do note one thing that may keep it from working, and that is the FROM header in the mail function. Reason being (and you will have to check with your host), is that many host will not accept mail to the SMTP server (to be sent), unless the FROM header is an email account residing on the SMTP server. In these cases, I use an address from the site, and include a REPLY TO header with the senders address. Of course, I would also suggest removing error suppression (@) from in front of the mail() function as well. -
Pointing you even further in the right direction, use isset(), or empty().
-
See if this works, post back if it doesn't: (You will have to add the next/previous or page number links). Un-Tested $album_path = $this->dir_photos.$y.'/'.$album.'/'; $normalPhotos = scandir($album_path); /* $validPath = '/photos/2014/Building%20Season/test.jpg'; $exif = exif_read_data($validPath, 'IFD0', TRUE); if(isset($exif) == true){ if (@array_key_exists('DateTime', $exif)) { $strDate = $exif['DateTime']; @list( $idate, $itime ) = explode( ' ', trim($strDate) ); @list( $iyear, $imonth, $iday ) = explode( ':', $date ); $realDate = strtotime( "{$iyear}-{$imonth}-{i$day} {$itime}" ); if(filemtime($normalPhotos) != $realDate){ touch($normalPhotos,$realDate); } } } $picLink[$images.$file] = filemtime($normalPhotos); */ ######## /*$page = (isset($_REQUEST['p']) ? $_REQUEST['p'] : null); //get user p INPUT_ENV $currentpage = isset($page) ? (integer)$page : 1; //get current page.. null = 1 $totalImg = count($normalPhotos)-2; //scandir adds two items to array by default '.' and '..' $totalPages = ceil($totalImg / $this->imgPerPage); if (($currentpage > 0) && ($currentpage <= $totalPages)) { $start = ($currentpage-1) * $numperpage; for($i=$start;$i<=($numperpage+$start-1);$i++) { } }*/ ############ $offset = (!empty($_GET['offset'])) ? (int)$_GET['offset'] : 0; //get the offset from the url: echo '<div id="photosCont">'; for($i = 0,$n = $offset;$i < 12; $i++,$n++) { //changed to a for statement. if(empty($normalPhotos[$n])) { //if we hit an empty array index, break the for loop. break; //exits the for loop. } $loadedPhotos = $this->dir_cache.$y.'_'.$album.'_'.$normalPhotos[$n]; //changed to reflect array. #FORMAT: photos/load/2014_Building Season_name.jpeg $urlPhotos = $this->dir_photos.$y.'/'.$album.'/'.$normalPhotos[$n]; //changed to reflect array. #FORMAT: photos/load/2014_building season_name.jpg if($normalPhotos[$n] == '.' OR $normalPhotos[$n] == '..'){ //changed to reflect array. $normalPhotos[$n] = ''; //changed to reflext array. } else { if(!file_exists($loadedPhotos)){ $photoLink = 'image.php?y='.$y.'&a='.$album.'&i='.$normalPhotos[$n]; } else { $photoLink = $this->dir_cache.$y.'_'.$album.'_'.$normalPhotos[$n]; } echo '<div class="photosBlock">'. '<a href="'.$urlPhotos.'" target="_blank"><img src="'.$photoLink.'" alt="thumbnail"/></a></div>'; //'<span class="photoDate">'.$photos.'</span></div>'; } }//foreach //end for echo '</div>'; }
-
PHP is server side, and doesn't understand HTML, CSS, Javascript, or any other scripting language for that matter. It only understands PHP, but will receive info from HTTP, XML, JSON, etc...