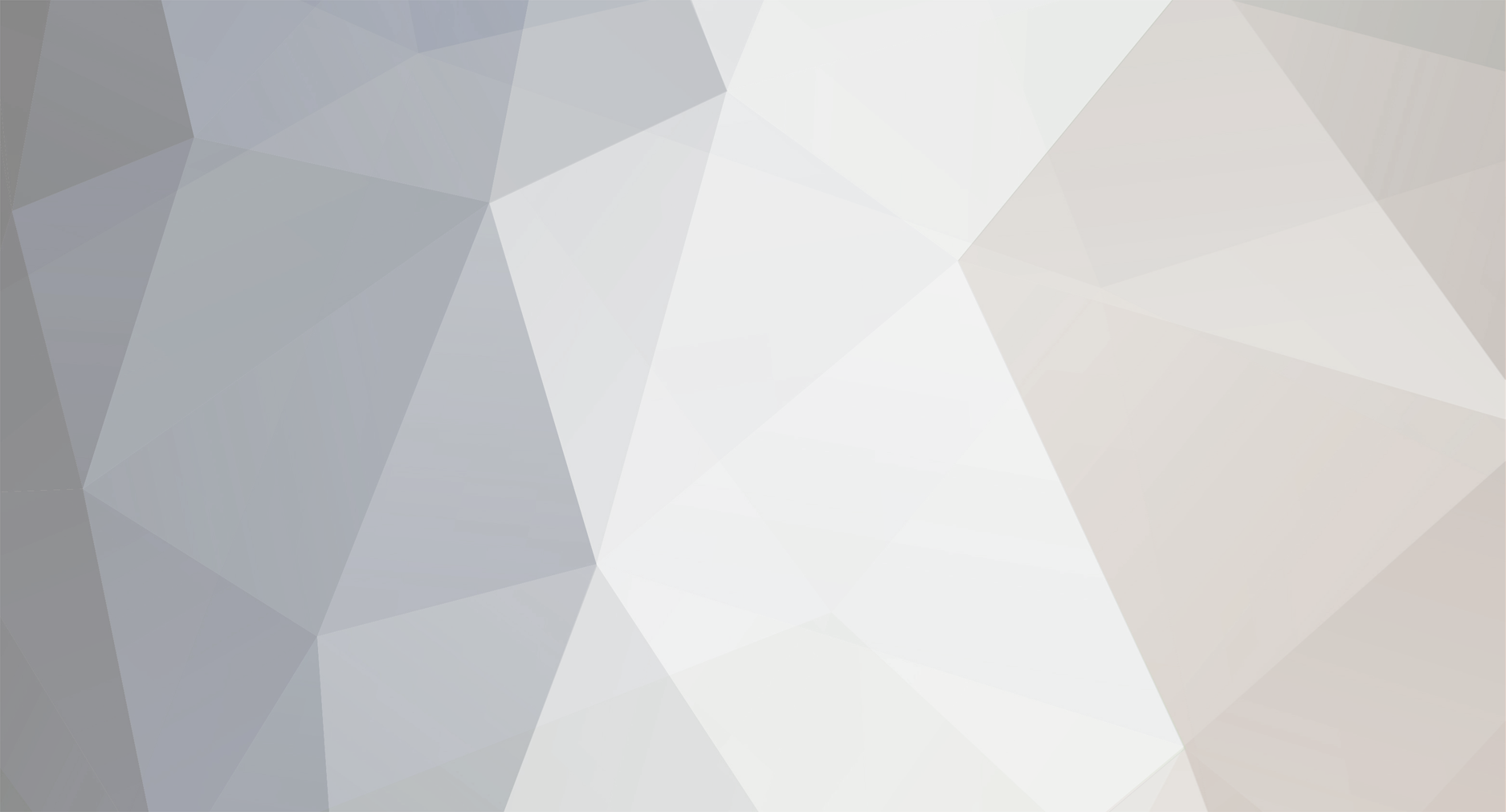
MDCode
Members-
Posts
640 -
Joined
-
Last visited
-
Days Won
1
Everything posted by MDCode
-
Ajax with PHP: click PHP result and autofill form with results
MDCode replied to kjetterman's topic in Javascript Help
I'm not exactly sure how your code relates. Your PHP seems to be searching for the person based on letter of last name and your JavaScript appears to be what you want to do with your form. Nonetheless I will provide basic outlines of something you can do. You should try organizing your search return first: /* Organized code for readability - Changed # to javascript:void(0) to get rid of the annoying page scroll Added the onclick event - Inside must be a unique database identifier (I am just assuming contact_id is) */ echo '<div id="search-results">'; echo "<ul class='letter-results'>\n"; echo "<li class='result-row'><a href='javascript:void(0)' onclick='formFill(\"{$contact_id}\");' class='testclass'>{$first_name} {$last_name}, {$church_org}</a></li>\n"; echo '</ul>'; echo '</div>'; Then your JavaScript would be something like: // Functions do not work well in document ready calls // This function will(should) fire from the onclick event we just set in the PHP function formFill(contact_id) { // You might want to clear the information in the form in case the user selects another person $("#organization").val(""); $("#firstname").val(""); $("#lastname").val(""); $("#email").val(""); $("#phone").val(""); $.ajax({ url: "fill_form.php", type: "POST", data: { "contact_id" : contact_id }, dataType: "json", success: function(data) { // Request was valid but it triggered one of our set errors if(data.hasOwnProperty('error')) { // You can choose a better option for error returning alert(data.error); return false; } // Fill the form with the information $("#organization").val(data.organization); $("#firstname").val(data.firstname); $("#lastname").val(data.lastname); $("#email").val(data.email); $("#phone").val(data.phone); }, error: function(data) { /* Add an error function if you want. Since it is in Json format, any returned text from the error will accessible in data.responseText */ }); } $(document).ready(function() { // Do whatever you are doing for searching via letter of last name }); Now: Your new PHP file (in this case fill_form.php) will have to access the database to get the information based on the contact_id /* Validate that contact_id is set Connect to the database If contact_id will always be a number, you can just use $contact_id = (int)$_POST['contact_id']; Note that removing the '' around {$contact_id} in the query if you use that method will leave you open to SQL Injection Otherwise use the below */ $contact_id = mysql_real_escape_string($_POST['contact_id']); $sql = "SELECT `first_name`, `last_name`, `church_org`, `email_address`, `phone_number` FROM `ics_data` WHERE `contact_id` = '{$contact_id}'"; //run the query against the mysql query function $result=mysql_query($sql); // Valid results if(mysql_num_rows($result) != 0) { // Because your $.ajax call has a dataType of json, you will need to json_encode everything $log = array(); $log['organization'] = $result['church_org']; $log['firstname'] = $result['first_name']; $log['lastname'] = $result['last_name']; $log['email'] = $result['email_address']; $log['phone'] = $result['phone_number']; json_encode($log); } else die(json_encode(array("error" => "No results"))); None of this was tested. Consider updating your PHP query functions to mysqli instead of mysql. You need to protect against SQL Injection and XSS in your original code as well -
window.print() does not work in opera unless the page has finished loading. Perhaps your new content is taking too long to load before it tries to print
-
Contact Form + Validator: Have to Click "Submit" Button Twice
MDCode replied to fadedrunes's topic in Javascript Help
If you still have to double-click after the JavaScript is removed, the problem is most likely your browser or something else. Pure HTML should not hang like that. -
Remove the error suppresing and see if anything happens.
-
I assume you're using JQuery Datepicker? If you can use PHP on the file and get the dates server-side, your JavaScript would be somewhat simple. You can use it on the same page and just substitue the array through PHP. Something like this perhaps (untested): <?php // Get dates into a PHP variable to be used later // $dates = ....... ?> <html> <head> <!-- I am not sure what JQuery version you use, or datepicker version/css so do not forget to add them here --> <script> $(document).ready(function() { // Substitute your dates in the var below. If you change the format, just make sure you change the formateDate below too // This array will disable October 23 - October 25 of this year var date_array = ["2014-10-23","2014-10-24","2014-10-25"]; // Substitute dates i.e. var date_array = <?php echo htmlentities($dates); ?> or something $("#datepicker").datepicker({ beforeShowDay: function(date) { // If you change date formats, change this as well var string = jQuery.datepicker.formatDate('yy-mm-dd', date); return [$.inArray(string, date_array) == -1]; } }); }); </script> </head> <body> <div class="control-group"> <label class='control-label'>Select Flyer Start Date:</label> <input type="text" name="datepicker" id="datepicker"> </div> </body> </html>
-
I'm not exactly sure what you're going for. You want a PHP array made when they click the checkboxes? Or are you trying to make an array of already modified ones?
-
Check the headers of the file. The extension can be jpeg, but the headers are what gets checked.
-
You should perhaps turn error reporting on. I see a syntax error in your first array.
-
Chmod the txt file to 0640 and it won't be publicly accessible. It would take development knowledge to view it. One could even go farther and instead of requesting a txt file, request a php file that pulls the IP list from the database, or, load a txt file with stricter rules outside of the document root.
-
Blocking by IP can be bypassed by a proxy or other. If you want to do it cross domain without creating load with Remote MySQL, something like requesting a txt file could work if you have allow_url_fopen set to On If you have each IP separated by a new line in a txt file such as 1.2.3.4 2.3.4.5 3.4.5.6 You could do something like: <?php $ip_list = file_get_contents("http://yourdomain/file.txt"); $ip = explode("\n", $ip_list); if(in_array($_SERVER['REMOTE_ADDR'], $ip)) { // Do your stuff } ?>
-
1. You do not need to check isset() if you use empty(). 2. Your query will return an error because of the random 1 at the end 3. Are the vars actually set? Have you echoed $query at the end? Have you tried print_r($_POST); ?
-
Your problems (probably) start at <script type="text/javascript"> var isPaused = 0; var loopType = 'none'; var lastSong = -1; var playlist = new Array("<?php echo $songs; ?>"); <!-- Still HTML yet you start PHP out of nowhere --> $db = new mysqli(HOST.COM,USER,PASS,StreamIT); // use your credentials $sql = "SELECT Songs FROM Main"; $playlist = array(); $res = $db->query($sql); while ($row = $res->fetch_assoc()) { $playlist[] = $row['Songs']; } $songs = join('","', $playlist); ?> I stopped looking after that.
-
mysql_error()
-
^ and shouldn't $db_passwordcheck be hashed with the salt too?
-
After the line where they commented that the session_regenerate_id() deletes the session without using the delete old session parameter, I wouldn't trust it to be up to date and/or do what it says exactly. I would have someone take a good look at it.
-
Issue with readfile() and download on at least one mobile browser
MDCode replied to LLLLLLL's topic in PHP Coding Help
Just because it is cgi doesn't mean it has nothing to do with it. The issue they stated was that it loads the URL twice. Later down, it was posted that android intended for that to happen. One request to see if it is a download. If it is, send another request to the download manager. -
Issue with readfile() and download on at least one mobile browser
MDCode replied to LLLLLLL's topic in PHP Coding Help
https://code.google.com/p/android/issues/detail?id=1978 As stated about half way down, it is an intended part of the android system. It has nothing to do with not working on one server. Not sure why you are not experiencing it on another server, but it is intended. You will have to rewrite your code to comply with android. -
Issue with readfile() and download on at least one mobile browser
MDCode replied to LLLLLLL's topic in PHP Coding Help
When downloading, it can be browsers as well. Just as in older versions of FF, if you don't specify the size of the file, it returns an incorrect value. -
Issue with readfile() and download on at least one mobile browser
MDCode replied to LLLLLLL's topic in PHP Coding Help
I had a problem with that but forgot how I fixed it. All I know is it had something to do with it requesting the file twice for some reason. When it refreshed one of the session variables I had unset to query the database for the location was unset and assumed it was the file I was headering to if it was not set. -
Issue with readfile() and download on at least one mobile browser
MDCode replied to LLLLLLL's topic in PHP Coding Help
Try adding another header to specify the type of the file being downloaded. I had an issue with that before. -
Have you seen if they have a support email and emailed them? If you entered the year in the proper format, then you need to contact their support. We can't fix their API.
-
If you're using the standard php download: <?php $referrer = 1; // Along with the others header("Content-Disposition: attachment; filename=installer_{$referrer}.exe"); ?>
-
Some browsers do not even send the http referer header and it can be spoofed.
-
So you're defining the variables after already using them?
-
Yes, using apache.